Figure Factory Tables in Python/v3
How to make tables in Python with Plotly's Figure Factory.
Note: this page is part of the documentation for version 3 of Plotly.py, which is not the most recent version.
See our Version 4 Migration Guide for information about how to upgrade.
See our Version 4 Migration Guide for information about how to upgrade.
New to Plotly?¶
Plotly's Python library is free and open source! Get started by downloading the client and reading the primer.
You can set up Plotly to work in online or offline mode, or in jupyter notebooks.
We also have a quick-reference cheatsheet (new!) to help you get started!
Version Check¶
Note: Tables are available in version 1.9.2+
Run pip install plotly --upgrade
to update your Plotly version
In [3]:
import plotly
plotly.__version__
Out[3]:
Simple Table¶
In [4]:
import plotly.plotly as py
import plotly.figure_factory as ff
data_matrix = [['Country', 'Year', 'Population'],
['United States', 2000, 282200000],
['Canada', 2000, 27790000],
['United States', 2005, 295500000],
['Canada', 2005, 32310000],
['United States', 2010, 309000000],
['Canada', 2010, 34000000]]
table = ff.create_table(data_matrix)
py.iplot(table, filename='simple_table')
Out[4]:
Add Links¶
In [5]:
import plotly.plotly as py
import plotly.figure_factory as ff
data_matrix = [['User', 'Language', 'Chart Type', '# of Views'],
['<a href="https://plotly.com/~empet/folder/home">empet</a>',
'<a href="https://plotly.com/python/">Python</a>',
'<a href="https://plotly.com/~empet/8614/">Network Graph</a>',
298],
['<a href="https://plotly.com/~Grondo/folder/home">Grondo</a>',
'<a href="https://plotly.com/matlab/">Matlab</a>',
'<a href="https://plotly.com/~Grondo/42/">Subplots</a>',
356],
['<a href="https://plotly.com/~Dreamshot/folder/home">Dreamshot</a>',
'<a href="https://help.plot.ly/tutorials/">Web App</a>',
'<a href="https://plotly.com/~Dreamshot/6575/_2014-us-city-populations/">Bubble Map</a>',
262],
['<a href="https://plotly.com/~FiveThirtyEight/folder/home">FiveThirtyEight</a>',
'<a href="https://help.plot.ly/tutorials/">Web App</a>',
'<a href="https://plotly.com/~FiveThirtyEight/30/">Scatter</a>',
692],
['<a href="https://plotly.com/~cpsievert/folder/home">cpsievert</a>',
'<a href="https://plotly.com/r/">R</a>',
'<a href="https://plotly.com/~cpsievert/1130/">Surface</a>',
302]]
table = ff.create_table(data_matrix)
py.iplot(table, filename='linked_table')
Out[5]:
Use LaTeX¶
In [6]:
import plotly.plotly as py
import plotly.figure_factory as ff
data_matrix = [['Name', 'Equation'],
['Pythagorean Theorem', '$a^{2}+b^{2}=c^{2}$'],
['Euler\'s Formula', '$F-E+V=2$'],
['The Origin of Complex Numbers', '$i^{2}=-1$'],
['Einstein\'s Theory of Relativity', '$E=m c^{2}$']]
table = ff.create_table(data_matrix)
py.iplot(table, filename='latex_table')
Out[6]:
Use a Panda's Dataframe¶
In [7]:
import plotly.plotly as py
import plotly.figure_factory as ff
import pandas as pd
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/master/gapminderDataFiveYear.csv')
df_sample = df[100:120]
table = ff.create_table(df_sample)
py.iplot(table, filename='pandas_table')
Out[7]:
Modify Row Height¶
The default row height is 30 pixels. Set height_constant
if you'd like to change the height of each row.
In [8]:
import plotly.plotly as py
import plotly.figure_factory as ff
data_matrix = [['Country', 'Year', 'Population'],
['United States', 2000, 282200000],
['Canada', 2000, 27790000],
['United States', 2005, 295500000],
['Canada', 2005, 32310000],
['United States', 2010, 309000000],
['Canada', 2010, 34000000]]
table = ff.create_table(data_matrix, height_constant=20)
py.iplot(table, filename='size_row_table')
Out[8]:
Custom Table Colors¶
A custom colorscale should be a list[list]
:
[[0, 'Header_Color'],[.5, 'Odd_Row_Color'],[1, 'Even_Row_Color']]
In [9]:
import plotly.plotly as py
import plotly.figure_factory as ff
import pandas as pd
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/master/gapminderDataFiveYear.csv')
df_sample = df[400:410]
colorscale = [[0, '#4d004c'],[.5, '#f2e5ff'],[1, '#ffffff']]
table = ff.create_table(df_sample, colorscale=colorscale)
py.iplot(table, filename='color_table')
Out[9]:
Custom Font Colors¶
In [10]:
import plotly.plotly as py
import plotly.figure_factory as ff
text = [['Team', 'Rank'], ['A', 1], ['B', 2], ['C', 3], ['D', 4], ['E', 5], ['F', 6]]
colorscale = [[0, '#272D31'],[.5, '#ffffff'],[1, '#ffffff']]
font=['#FCFCFC', '#00EE00', '#008B00', '#004F00', '#660000', '#CD0000', '#FF3030']
table = ff.create_table(text, colorscale=colorscale, font_colors=font)
table.layout.width=250
py.iplot(table, filename='font_table')
Out[10]:
Change Font Size¶
In [11]:
import plotly.plotly as py
import plotly.figure_factory as ff
data_matrix = [['Country', 'Year', 'Population'],
['United States', 2000, 282200000],
['Canada', 2000, 27790000],
['United States', 2005, 295500000],
['Canada', 2005, 32310000],
['United States', 2010, 309000000],
['Canada', 2010, 34000000]]
table = ff.create_table(data_matrix, index=True)
# Make text size larger
for i in range(len(table.layout.annotations)):
table.layout.annotations[i].font.size = 20
py.iplot(table, filename='index_table')
Out[11]:
Tables with Graphs¶
In [12]:
import plotly.plotly as py
import plotly.graph_objs as go
import plotly.figure_factory as ff
# Add table data
table_data = [['Team', 'Wins', 'Losses', 'Ties'],
['Montréal<br>Canadiens', 18, 4, 0],
['Dallas Stars', 18, 5, 0],
['NY Rangers', 16, 5, 0],
['Boston<br>Bruins', 13, 8, 0],
['Chicago<br>Blackhawks', 13, 8, 0],
['LA Kings', 13, 8, 0],
['Ottawa<br>Senators', 12, 5, 0]]
# Initialize a figure with FF.create_table(table_data)
figure = ff.create_table(table_data, height_constant=60)
# Add graph data
teams = ['Montréal Canadiens', 'Dallas Stars', 'NY Rangers',
'Boston Bruins', 'Chicago Blackhawks', 'LA Kings', 'Ottawa Senators']
GFPG = [3.54, 3.48, 3.0, 3.27, 2.83, 2.45, 3.18]
GAPG = [2.17, 2.57, 2.0, 2.91, 2.57, 2.14, 2.77]
# Make traces for graph
trace1 = go.Scatter(x=teams, y=GFPG,
marker=dict(color='#0099ff'),
name='Goals For<br>Per Game',
xaxis='x2', yaxis='y2')
trace2 = go.Scatter(x=teams, y=GAPG,
marker=dict(color='#404040'),
name='Goals Against<br>Per Game',
xaxis='x2', yaxis='y2')
# Add trace data to figure
figure['data'].extend(go.Data([trace1, trace2]))
# Edit layout for subplots
figure.layout.xaxis.update({'domain': [0, .5]})
figure.layout.xaxis2.update({'domain': [0.6, 1.]})
# The graph's yaxis MUST BE anchored to the graph's xaxis
figure.layout.yaxis2.update({'anchor': 'x2'})
figure.layout.yaxis2.update({'title': 'Goals'})
# Update the margins to add a title and see graph x-labels.
figure.layout.margin.update({'t':50, 'b':100})
figure.layout.update({'title': '2016 Hockey Stats'})
# Plot!
py.iplot(figure, filename='subplot_table')
Out[12]:
In [13]:
import plotly.plotly as py
import plotly.graph_objs as go
import plotly.figure_factory as FF
# Add table data
table_data = [['Team', 'Wins', 'Losses', 'Ties'],
['Montréal<br>Canadiens', 18, 4, 0],
['Dallas Stars', 18, 5, 0],
['NY Rangers', 16, 5, 0],
['Boston<br>Bruins', 13, 8, 0],
['Chicago<br>Blackhawks', 13, 8, 0],
['Ottawa<br>Senators', 12, 5, 0]]
# Initialize a figure with FF.create_table(table_data)
figure = FF.create_table(table_data, height_constant=60)
# Add graph data
teams = ['Montréal Canadiens', 'Dallas Stars', 'NY Rangers',
'Boston Bruins', 'Chicago Blackhawks', 'Ottawa Senators']
GFPG = [3.54, 3.48, 3.0, 3.27, 2.83, 3.18]
GAPG = [2.17, 2.57, 2.0, 2.91, 2.57, 2.77]
# Make traces for graph
trace1 = go.Bar(x=teams, y=GFPG, xaxis='x2', yaxis='y2',
marker=dict(color='#0099ff'),
name='Goals For<br>Per Game')
trace2 = go.Bar(x=teams, y=GAPG, xaxis='x2', yaxis='y2',
marker=dict(color='#404040'),
name='Goals Against<br>Per Game')
# Add trace data to figure
figure['data'].extend(go.Data([trace1, trace2]))
# Edit layout for subplots
figure.layout.yaxis.update({'domain': [0, .45]})
figure.layout.yaxis2.update({'domain': [.6, 1]})
# The graph's yaxis2 MUST BE anchored to the graph's xaxis2 and vice versa
figure.layout.yaxis2.update({'anchor': 'x2'})
figure.layout.xaxis2.update({'anchor': 'y2'})
figure.layout.yaxis2.update({'title': 'Goals'})
# Update the margins to add a title and see graph x-labels.
figure.layout.margin.update({'t':75, 'l':50})
figure.layout.update({'title': '2016 Hockey Stats'})
# Update the height because adding a graph vertically will interact with
# the plot height calculated for the table
figure.layout.update({'height':800})
# Plot!
py.iplot(figure, filename='subplot_table_vertical')
Out[13]:
In [14]:
import plotly.plotly as py
import plotly.graph_objs as go
import plotly.figure_factory as ff
# Add table data
table_data = [['Prominence', 'Percent', 'RGB Value'],
[1, '38%', 'rgb(56, 75, 126)'],
[2, '27%', 'rgb(18, 36, 37)'],
[3, '18%', 'rgb(34, 53, 101)'],
[4, '10%', 'rgb(36, 55, 57)'],
[5, '7%', 'rgb(6, 4, 4)']]
# Initialize a figure with ff.create_table(table_data)
figure = ff.create_table(table_data, height_constant=60)
# Add graph data
trace1={'labels': ['1st', '2nd', '3rd', '4th', '5th'],
'values': [38, 27, 18, 10, 7],
'type': 'pie',
'name': 'Starry Night',
'marker': {'colors': ['rgb(56, 75, 126)',
'rgb(18, 36, 37)',
'rgb(34, 53, 101)',
'rgb(36, 55, 57)',
'rgb(6, 4, 4)']},
'domain': {'x': [0, 1],
'y': [.4, 1]},
'hoverinfo':'label+percent+name',
'textinfo':'none'
}
# Add trace data to figure
figure['data'].extend(go.Data([trace1]))
# Edit layout for subplots
figure.layout.yaxis.update({'domain': [0, .30]})
# The graph's yaxis2 MUST BE anchored to the graph's xaxis2 and vice versa
# Update the margins to add a title and see graph x-labels.
figure.layout.margin.update({'t':75, 'l':50})
figure.layout.update({'title': 'Starry Night'})
# Update the height because adding a graph vertically will interact with
# the plot height calculated for the table
figure.layout.update({'height':800})
# Plot!
py.iplot(figure)
Out[14]:
Reference¶
In [15]:
help(ff.create_table)
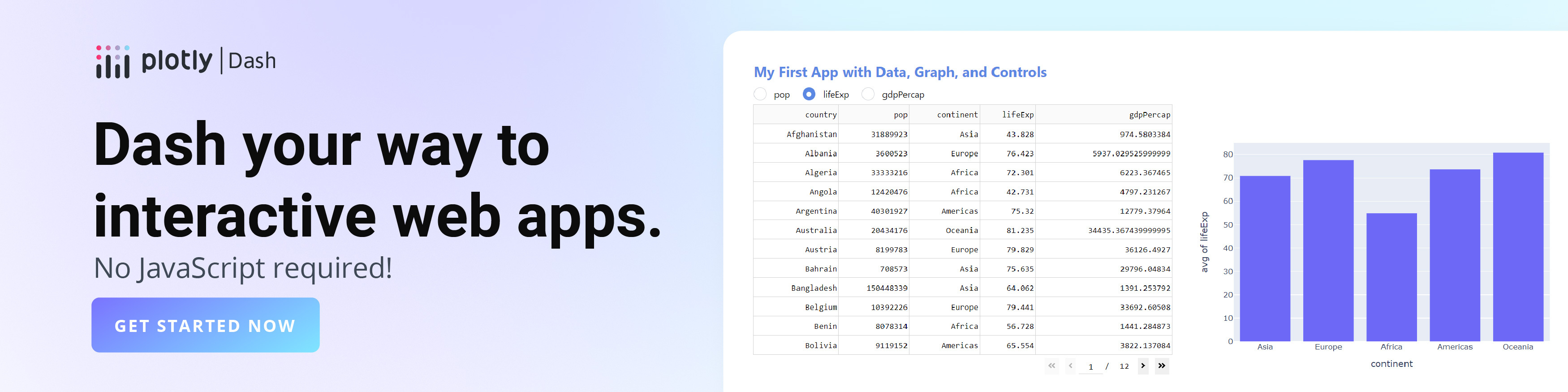