Getting Started with Plotly in Python
Getting Started with Plotly for Python.
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Overview¶
The plotly
Python library is an interactive, open-source plotting library that supports over 40 unique chart types covering a wide range of statistical, financial, geographic, scientific, and 3-dimensional use-cases.
Built on top of the Plotly JavaScript library (plotly.js), plotly
enables Python users to create beautiful interactive web-based visualizations that can be displayed in Jupyter notebooks, saved to standalone HTML files, or served as part of pure Python-built web applications using Dash. The plotly
Python library is sometimes referred to as "plotly.py" to differentiate it from the JavaScript library.
Thanks to deep integration with our Kaleido image export utility, plotly
also provides great support for non-web contexts including desktop editors (e.g. QtConsole, Spyder, PyCharm) and static document publishing (e.g. exporting notebooks to PDF with high-quality vector images).
This Getting Started guide explains how to install plotly
and related optional pages. Once you've installed, you can use our documentation in three main ways:
- You jump right in to examples of how to make basic charts, statistical charts, scientific charts, financial charts, maps, and 3-dimensional charts.
- If you prefer to learn about the fundamentals of the library first, you can read about the structure of figures, how to create and update figures, how to display figures, how to theme figures with templates, how to export figures to various formats and about Plotly Express, the high-level API for doing all of the above.
- You can check out our exhaustive reference guides: the Python API reference or the Figure Reference
For information on using Python to build web applications containing plotly figures, see the Dash User Guide.
We also encourage you to join the Plotly Community Forum if you want help with anything related to plotly
.
Installation¶
plotly
may be installed using pip
:
or conda
:
This package contains everything you need to write figures to standalone HTML files.
Note: No internet connection, account, or payment is required to use plotly.py. Prior to version 4, this library could operate in either an "online" or "offline" mode. The documentation tended to emphasize the online mode, where graphs get published to the Chart Studio web service. In version 4, all "online" functionality was removed from the
plotly
package and is now available as the separate, optional,chart-studio
package (See below). plotly.py version 4 is "offline" only, and does not include any functionality for uploading figures or data to cloud services.
import plotly.express as px
fig = px.bar(x=["a", "b", "c"], y=[1, 3, 2])
fig.write_html('first_figure.html', auto_open=True)
Plotly charts in Dash¶
Dash is the best way to build analytical apps in Python using Plotly figures. To run the app below, run pip install dash
, click "Download" to get the code and run python app.py
.
Get started with the official Dash docs and learn how to effortlessly style & deploy apps like this with Dash Enterprise.
Sign up for Dash Club → Free cheat sheets plus updates from Chris Parmer and Adam Schroeder delivered to your inbox every two months. Includes tips and tricks, community apps, and deep dives into the Dash architecture. Join now.
JupyterLab Support¶
For use in JupyterLab, install the jupyterlab
and ipywidgets
packages using pip
:
or conda
:
You'll need jupyter-dash
to add widgets such as sliders, dropdowns, and buttons to Plotly charts in JupyterLab.
Install jupyter-dash
using pip
:
or conda
:
These packages contain everything you need to run JupyterLab...
and display plotly figures inline using the plotly_mimetype
renderer...
import plotly.express as px
fig = px.bar(x=["a", "b", "c"], y=[1, 3, 2])
fig.show()
or using FigureWidget
objects.
import plotly.express as px
fig = px.bar(x=["a", "b", "c"], y=[1, 3, 2])
import plotly.graph_objects as go
fig_widget = go.FigureWidget(fig)
fig_widget
The instructions above apply to JupyterLab 3.x. For JupyterLab 2 or earlier, run the following commands to install the required JupyterLab extensions (note that this will require node
to be installed):
Please check out our Troubleshooting guide if you run into any problems with JupyterLab, particularly if you are using multiple python environments inside Jupyter.
See Displaying Figures in Python for more information on the renderers framework, and see Plotly FigureWidget Overview for more information on using FigureWidget
.
Jupyter Notebook Support¶
For use in the classic Jupyter Notebook, install the notebook
and ipywidgets
packages using pip
:
or conda
:
These packages contain everything you need to run a Jupyter notebook...
and display plotly figures inline using the notebook renderer...
import plotly.express as px
fig = px.bar(x=["a", "b", "c"], y=[1, 3, 2])
fig.show()
or using FigureWidget
objects.
import plotly.express as px
fig = px.bar(x=["a", "b", "c"], y=[1, 3, 2])
import plotly.graph_objects as go
fig_widget = go.FigureWidget(fig)
fig_widget
See Displaying Figures in Python for more information on the renderers framework, and see Plotly FigureWidget Overview for more information on using FigureWidget
.
or conda.
Orca¶
While Kaleido is now the recommended image export approach because it is easier to install
and more widely compatible, static image export
can also be supported
by the legacy orca command line utility and the
psutil
Python package.
These dependencies can both be installed using conda:
Or, psutil
can be installed using pip...
and orca can be installed according to the instructions in the orca README.
Extended Geo Support¶
Some plotly.py features rely on fairly large geographic shape files. The county
choropleth figure factory is one such example. These shape files are distributed as a
separate plotly-geo
package. This package can be installed using pip...
or conda.
See USA County Choropleth Maps in Python for more information on the county choropleth figure factory.
Chart Studio Support¶
The chart-studio
package can be used to upload plotly figures to Plotly's Chart
Studio Cloud or On-Prem services. This package can be installed using pip...
or conda.
Note: This package is optional, and if it is not installed it is not possible for figures to be uploaded to the Chart Studio cloud service.
Where to next?¶
Once you've installed, you can use our documentation in three main ways:
- You jump right in to examples of how to make basic charts, statistical charts, scientific charts, financial charts, maps, and 3-dimensional charts.
- If you prefer to learn about the fundamentals of the library first, you can read about the structure of figures, how to create and update figures, how to display figures, how to theme figures with templates, how to export figures to various formats and about Plotly Express, the high-level API for doing all of the above.
- You can check out our exhaustive reference guides: the Python API reference or the Figure Reference
For information on using Python to build web applications containing plotly figures, see the Dash User Guide.
We also encourage you to join the Plotly Community Forum if you want help with anything related to plotly
.
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
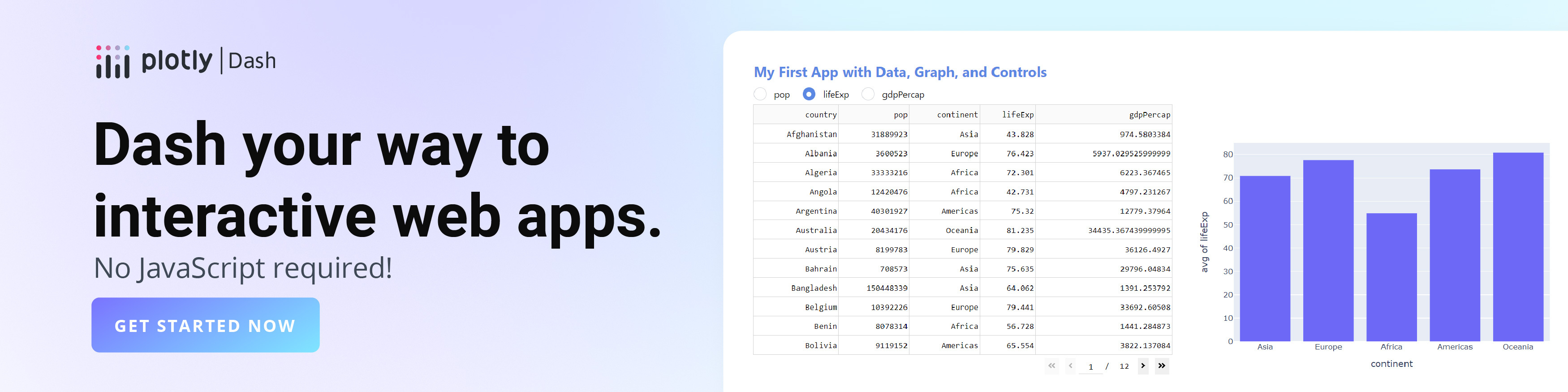