Plotly FigureWidget Overview in Python
Introduction to the new Plotly FigureWidget
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Create a Simple FigureWidget¶
Create an empty FigureWidget and then view it.
import plotly.graph_objects as go
f = go.FigureWidget()
f
Add traces or update the layout and then watch the output above update in real time.
f.add_scatter(y=[2, 1, 4, 3]);
f.add_bar(y=[1, 4, 3, 2]);
f.layout.title = 'Hello FigureWidget'
Update the Data and the Layout¶
# update scatter data
scatter = f.data[0]
scatter.y = [3, 1, 4, 3]
# update bar data
bar = f.data[1]
bar.y = [5, 3, 2, 8]
f.layout.title.text = 'This is a new title'
Construct a FigureWidget from a Figure graph object¶
A standard Figure
object can be passed to the FigureWidget
constructor.
import plotly.graph_objects as go
trace = go.Heatmap(z=[[1, 20, 30, 50, 1], [20, 1, 60, 80, 30], [30, 60, 1, -10, 20]],
x=['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'],
y=['Morning', 'Afternoon', 'Evening'])
data=[trace]
layout = go.Layout(title='Activity Heatmap')
figure = go.Figure(data=data, layout=layout)
f2 = go.FigureWidget(figure)
f2
Reference¶
See these Jupyter notebooks for even more FigureWidget examples.
help(go.FigureWidget)
Help on class FigureWidget in module plotly.graph_objs._figurewidget: class FigureWidget(plotly.basewidget.BaseFigureWidget) | FigureWidget(data=None, layout=None, frames=None, skip_invalid=False, **kwargs) | | Method resolution order: | FigureWidget | plotly.basewidget.BaseFigureWidget | plotly.basedatatypes.BaseFigure | ipywidgets.widgets.domwidget.DOMWidget | ipywidgets.widgets.widget.Widget | ipywidgets.widgets.widget.LoggingHasTraits | traitlets.traitlets.HasTraits | traitlets.traitlets.HasDescriptors | builtins.object | | Methods defined here: | | __init__(self, data=None, layout=None, frames=None, skip_invalid=False, **kwargs) | Create a new :class:FigureWidget instance | | Parameters | ---------- | data | The 'data' property is a tuple of trace instances | that may be specified as: | - A list or tuple of trace instances | (e.g. [Scatter(...), Bar(...)]) | - A single trace instance | (e.g. Scatter(...), Bar(...), etc.) | - A list or tuple of dicts of string/value properties where: | - The 'type' property specifies the trace type | One of: ['bar', 'barpolar', 'box', 'candlestick', | 'carpet', 'choropleth', 'choroplethmap', | 'choroplethmapbox', 'cone', 'contour', | 'contourcarpet', 'densitymap', | 'densitymapbox', 'funnel', 'funnelarea', | 'heatmap', 'heatmapgl', 'histogram', | 'histogram2d', 'histogram2dcontour', 'icicle', | 'image', 'indicator', 'isosurface', 'mesh3d', | 'ohlc', 'parcats', 'parcoords', 'pie', | 'pointcloud', 'sankey', 'scatter', | 'scatter3d', 'scattercarpet', 'scattergeo', | 'scattergl', 'scattermap', 'scattermapbox', | 'scatterpolar', 'scatterpolargl', | 'scattersmith', 'scatterternary', 'splom', | 'streamtube', 'sunburst', 'surface', 'table', | 'treemap', 'violin', 'volume', 'waterfall'] | | - All remaining properties are passed to the constructor of | the specified trace type | | (e.g. [{'type': 'scatter', ...}, {'type': 'bar, ...}]) | | layout | The 'layout' property is an instance of Layout | that may be specified as: | - An instance of :class:`plotly.graph_objs.Layout` | - A dict of string/value properties that will be passed | to the Layout constructor | | Supported dict properties: | | activeselection | :class:`plotly.graph_objects.layout.Activeselec | tion` instance or dict with compatible | properties | activeshape | :class:`plotly.graph_objects.layout.Activeshape | ` instance or dict with compatible properties | annotations | A tuple of | :class:`plotly.graph_objects.layout.Annotation` | instances or dicts with compatible properties | annotationdefaults | When used in a template (as | layout.template.layout.annotationdefaults), | sets the default property values to use for | elements of layout.annotations | autosize | Determines whether or not a layout width or | height that has been left undefined by the user | is initialized on each relayout. Note that, | regardless of this attribute, an undefined | layout width or height is always initialized on | the first call to plot. | autotypenumbers | Using "strict" a numeric string in trace data | is not converted to a number. Using *convert | types* a numeric string in trace data may be | treated as a number during automatic axis | `type` detection. This is the default value; | however it could be overridden for individual | axes. | barcornerradius | Sets the rounding of bar corners. May be an | integer number of pixels, or a percentage of | bar width (as a string ending in %). | bargap | Sets the gap (in plot fraction) between bars of | adjacent location coordinates. | bargroupgap | Sets the gap (in plot fraction) between bars of | the same location coordinate. | barmode | Determines how bars at the same location | coordinate are displayed on the graph. With | "stack", the bars are stacked on top of one | another With "relative", the bars are stacked | on top of one another, with negative values | below the axis, positive values above With | "group", the bars are plotted next to one | another centered around the shared location. | With "overlay", the bars are plotted over one | another, you might need to reduce "opacity" to | see multiple bars. | barnorm | Sets the normalization for bar traces on the | graph. With "fraction", the value of each bar | is divided by the sum of all values at that | location coordinate. "percent" is the same but | multiplied by 100 to show percentages. | boxgap | Sets the gap (in plot fraction) between boxes | of adjacent location coordinates. Has no effect | on traces that have "width" set. | boxgroupgap | Sets the gap (in plot fraction) between boxes | of the same location coordinate. Has no effect | on traces that have "width" set. | boxmode | Determines how boxes at the same location | coordinate are displayed on the graph. If | "group", the boxes are plotted next to one | another centered around the shared location. If | "overlay", the boxes are plotted over one | another, you might need to set "opacity" to see | them multiple boxes. Has no effect on traces | that have "width" set. | calendar | Sets the default calendar system to use for | interpreting and displaying dates throughout | the plot. | clickmode | Determines the mode of single click | interactions. "event" is the default value and | emits the `plotly_click` event. In addition | this mode emits the `plotly_selected` event in | drag modes "lasso" and "select", but with no | event data attached (kept for compatibility | reasons). The "select" flag enables selecting | single data points via click. This mode also | supports persistent selections, meaning that | pressing Shift while clicking, adds to / | subtracts from an existing selection. "select" | with `hovermode`: "x" can be confusing, | consider explicitly setting `hovermode`: | "closest" when using this feature. Selection | events are sent accordingly as long as "event" | flag is set as well. When the "event" flag is | missing, `plotly_click` and `plotly_selected` | events are not fired. | coloraxis | :class:`plotly.graph_objects.layout.Coloraxis` | instance or dict with compatible properties | colorscale | :class:`plotly.graph_objects.layout.Colorscale` | instance or dict with compatible properties | colorway | Sets the default trace colors. | computed | Placeholder for exporting automargin-impacting | values namely `margin.t`, `margin.b`, | `margin.l` and `margin.r` in "full-json" mode. | datarevision | If provided, a changed value tells | `Plotly.react` that one or more data arrays has | changed. This way you can modify arrays in- | place rather than making a complete new copy | for an incremental change. If NOT provided, | `Plotly.react` assumes that data arrays are | being treated as immutable, thus any data array | with a different identity from its predecessor | contains new data. | dragmode | Determines the mode of drag interactions. | "select" and "lasso" apply only to scatter | traces with markers or text. "orbit" and | "turntable" apply only to 3D scenes. | editrevision | Controls persistence of user-driven changes in | `editable: true` configuration, other than | trace names and axis titles. Defaults to | `layout.uirevision`. | extendfunnelareacolors | If `true`, the funnelarea slice colors (whether | given by `funnelareacolorway` or inherited from | `colorway`) will be extended to three times its | original length by first repeating every color | 20% lighter then each color 20% darker. This is | intended to reduce the likelihood of reusing | the same color when you have many slices, but | you can set `false` to disable. Colors provided | in the trace, using `marker.colors`, are never | extended. | extendiciclecolors | If `true`, the icicle slice colors (whether | given by `iciclecolorway` or inherited from | `colorway`) will be extended to three times its | original length by first repeating every color | 20% lighter then each color 20% darker. This is | intended to reduce the likelihood of reusing | the same color when you have many slices, but | you can set `false` to disable. Colors provided | in the trace, using `marker.colors`, are never | extended. | extendpiecolors | If `true`, the pie slice colors (whether given | by `piecolorway` or inherited from `colorway`) | will be extended to three times its original | length by first repeating every color 20% | lighter then each color 20% darker. This is | intended to reduce the likelihood of reusing | the same color when you have many slices, but | you can set `false` to disable. Colors provided | in the trace, using `marker.colors`, are never | extended. | extendsunburstcolors | If `true`, the sunburst slice colors (whether | given by `sunburstcolorway` or inherited from | `colorway`) will be extended to three times its | original length by first repeating every color | 20% lighter then each color 20% darker. This is | intended to reduce the likelihood of reusing | the same color when you have many slices, but | you can set `false` to disable. Colors provided | in the trace, using `marker.colors`, are never | extended. | extendtreemapcolors | If `true`, the treemap slice colors (whether | given by `treemapcolorway` or inherited from | `colorway`) will be extended to three times its | original length by first repeating every color | 20% lighter then each color 20% darker. This is | intended to reduce the likelihood of reusing | the same color when you have many slices, but | you can set `false` to disable. Colors provided | in the trace, using `marker.colors`, are never | extended. | font | Sets the global font. Note that fonts used in | traces and other layout components inherit from | the global font. | funnelareacolorway | Sets the default funnelarea slice colors. | Defaults to the main `colorway` used for trace | colors. If you specify a new list here it can | still be extended with lighter and darker | colors, see `extendfunnelareacolors`. | funnelgap | Sets the gap (in plot fraction) between bars of | adjacent location coordinates. | funnelgroupgap | Sets the gap (in plot fraction) between bars of | the same location coordinate. | funnelmode | Determines how bars at the same location | coordinate are displayed on the graph. With | "stack", the bars are stacked on top of one | another With "group", the bars are plotted next | to one another centered around the shared | location. With "overlay", the bars are plotted | over one another, you might need to reduce | "opacity" to see multiple bars. | geo | :class:`plotly.graph_objects.layout.Geo` | instance or dict with compatible properties | grid | :class:`plotly.graph_objects.layout.Grid` | instance or dict with compatible properties | height | Sets the plot's height (in px). | hiddenlabels | hiddenlabels is the funnelarea & pie chart | analog of visible:'legendonly' but it can | contain many labels, and can simultaneously | hide slices from several pies/funnelarea charts | hiddenlabelssrc | Sets the source reference on Chart Studio Cloud | for `hiddenlabels`. | hidesources | Determines whether or not a text link citing | the data source is placed at the bottom-right | cored of the figure. Has only an effect only on | graphs that have been generated via forked | graphs from the Chart Studio Cloud (at | https://chart-studio.plotly.com or on-premise). | hoverdistance | Sets the default distance (in pixels) to look | for data to add hover labels (-1 means no | cutoff, 0 means no looking for data). This is | only a real distance for hovering on point-like | objects, like scatter points. For area-like | objects (bars, scatter fills, etc) hovering is | on inside the area and off outside, but these | objects will not supersede hover on point-like | objects in case of conflict. | hoverlabel | :class:`plotly.graph_objects.layout.Hoverlabel` | instance or dict with compatible properties | hovermode | Determines the mode of hover interactions. If | "closest", a single hoverlabel will appear for | the "closest" point within the `hoverdistance`. | If "x" (or "y"), multiple hoverlabels will | appear for multiple points at the "closest" x- | (or y-) coordinate within the `hoverdistance`, | with the caveat that no more than one | hoverlabel will appear per trace. If *x | unified* (or *y unified*), a single hoverlabel | will appear multiple points at the closest x- | (or y-) coordinate within the `hoverdistance` | with the caveat that no more than one | hoverlabel will appear per trace. In this mode, | spikelines are enabled by default perpendicular | to the specified axis. If false, hover | interactions are disabled. | hoversubplots | Determines expansion of hover effects to other | subplots If "single" just the axis pair of the | primary point is included without overlaying | subplots. If "overlaying" all subplots using | the main axis and occupying the same space are | included. If "axis", also include stacked | subplots using the same axis when `hovermode` | is set to "x", *x unified*, "y" or *y unified*. | iciclecolorway | Sets the default icicle slice colors. Defaults | to the main `colorway` used for trace colors. | If you specify a new list here it can still be | extended with lighter and darker colors, see | `extendiciclecolors`. | images | A tuple of | :class:`plotly.graph_objects.layout.Image` | instances or dicts with compatible properties | imagedefaults | When used in a template (as | layout.template.layout.imagedefaults), sets the | default property values to use for elements of | layout.images | legend | :class:`plotly.graph_objects.layout.Legend` | instance or dict with compatible properties | map | :class:`plotly.graph_objects.layout.Map` | instance or dict with compatible properties | mapbox | :class:`plotly.graph_objects.layout.Mapbox` | instance or dict with compatible properties | margin | :class:`plotly.graph_objects.layout.Margin` | instance or dict with compatible properties | meta | Assigns extra meta information that can be used | in various `text` attributes. Attributes such | as the graph, axis and colorbar `title.text`, | annotation `text` `trace.name` in legend items, | `rangeselector`, `updatemenus` and `sliders` | `label` text all support `meta`. One can access | `meta` fields using template strings: | `%{meta[i]}` where `i` is the index of the | `meta` item in question. `meta` can also be an | object for example `{key: value}` which can be | accessed %{meta[key]}. | metasrc | Sets the source reference on Chart Studio Cloud | for `meta`. | minreducedheight | Minimum height of the plot with | margin.automargin applied (in px) | minreducedwidth | Minimum width of the plot with | margin.automargin applied (in px) | modebar | :class:`plotly.graph_objects.layout.Modebar` | instance or dict with compatible properties | newselection | :class:`plotly.graph_objects.layout.Newselectio | n` instance or dict with compatible properties | newshape | :class:`plotly.graph_objects.layout.Newshape` | instance or dict with compatible properties | paper_bgcolor | Sets the background color of the paper where | the graph is drawn. | piecolorway | Sets the default pie slice colors. Defaults to | the main `colorway` used for trace colors. If | you specify a new list here it can still be | extended with lighter and darker colors, see | `extendpiecolors`. | plot_bgcolor | Sets the background color of the plotting area | in-between x and y axes. | polar | :class:`plotly.graph_objects.layout.Polar` | instance or dict with compatible properties | scattergap | Sets the gap (in plot fraction) between scatter | points of adjacent location coordinates. | Defaults to `bargap`. | scattermode | Determines how scatter points at the same | location coordinate are displayed on the graph. | With "group", the scatter points are plotted | next to one another centered around the shared | location. With "overlay", the scatter points | are plotted over one another, you might need to | reduce "opacity" to see multiple scatter | points. | scene | :class:`plotly.graph_objects.layout.Scene` | instance or dict with compatible properties | selectdirection | When `dragmode` is set to "select", this limits | the selection of the drag to horizontal, | vertical or diagonal. "h" only allows | horizontal selection, "v" only vertical, "d" | only diagonal and "any" sets no limit. | selectionrevision | Controls persistence of user-driven changes in | selected points from all traces. | selections | A tuple of | :class:`plotly.graph_objects.layout.Selection` | instances or dicts with compatible properties | selectiondefaults | When used in a template (as | layout.template.layout.selectiondefaults), sets | the default property values to use for elements | of layout.selections | separators | Sets the decimal and thousand separators. For | example, *. * puts a '.' before decimals and a | space between thousands. In English locales, | dflt is ".," but other locales may alter this | default. | shapes | A tuple of | :class:`plotly.graph_objects.layout.Shape` | instances or dicts with compatible properties | shapedefaults | When used in a template (as | layout.template.layout.shapedefaults), sets the | default property values to use for elements of | layout.shapes | showlegend | Determines whether or not a legend is drawn. | Default is `true` if there is a trace to show | and any of these: a) Two or more traces would | by default be shown in the legend. b) One pie | trace is shown in the legend. c) One trace is | explicitly given with `showlegend: true`. | sliders | A tuple of | :class:`plotly.graph_objects.layout.Slider` | instances or dicts with compatible properties | sliderdefaults | When used in a template (as | layout.template.layout.sliderdefaults), sets | the default property values to use for elements | of layout.sliders | smith | :class:`plotly.graph_objects.layout.Smith` | instance or dict with compatible properties | spikedistance | Sets the default distance (in pixels) to look | for data to draw spikelines to (-1 means no | cutoff, 0 means no looking for data). As with | hoverdistance, distance does not apply to area- | like objects. In addition, some objects can be | hovered on but will not generate spikelines, | such as scatter fills. | sunburstcolorway | Sets the default sunburst slice colors. | Defaults to the main `colorway` used for trace | colors. If you specify a new list here it can | still be extended with lighter and darker | colors, see `extendsunburstcolors`. | template | Default attributes to be applied to the plot. | This should be a dict with format: `{'layout': | layoutTemplate, 'data': {trace_type: | [traceTemplate, ...], ...}}` where | `layoutTemplate` is a dict matching the | structure of `figure.layout` and | `traceTemplate` is a dict matching the | structure of the trace with type `trace_type` | (e.g. 'scatter'). Alternatively, this may be | specified as an instance of | plotly.graph_objs.layout.Template. Trace | templates are applied cyclically to traces of | each type. Container arrays (eg `annotations`) | have special handling: An object ending in | `defaults` (eg `annotationdefaults`) is applied | to each array item. But if an item has a | `templateitemname` key we look in the template | array for an item with matching `name` and | apply that instead. If no matching `name` is | found we mark the item invisible. Any named | template item not referenced is appended to the | end of the array, so this can be used to add a | watermark annotation or a logo image, for | example. To omit one of these items on the | plot, make an item with matching | `templateitemname` and `visible: false`. | ternary | :class:`plotly.graph_objects.layout.Ternary` | instance or dict with compatible properties | title | :class:`plotly.graph_objects.layout.Title` | instance or dict with compatible properties | titlefont | Deprecated: Please use layout.title.font | instead. Sets the title font. Note that the | title's font used to be customized by the now | deprecated `titlefont` attribute. | transition | Sets transition options used during | Plotly.react updates. | treemapcolorway | Sets the default treemap slice colors. Defaults | to the main `colorway` used for trace colors. | If you specify a new list here it can still be | extended with lighter and darker colors, see | `extendtreemapcolors`. | uirevision | Used to allow user interactions with the plot | to persist after `Plotly.react` calls that are | unaware of these interactions. If `uirevision` | is omitted, or if it is given and it changed | from the previous `Plotly.react` call, the | exact new figure is used. If `uirevision` is | truthy and did NOT change, any attribute that | has been affected by user interactions and did | not receive a different value in the new figure | will keep the interaction value. | `layout.uirevision` attribute serves as the | default for `uirevision` attributes in various | sub-containers. For finer control you can set | these sub-attributes directly. For example, if | your app separately controls the data on the x | and y axes you might set | `xaxis.uirevision=*time*` and | `yaxis.uirevision=*cost*`. Then if only the y | data is changed, you can update | `yaxis.uirevision=*quantity*` and the y axis | range will reset but the x axis range will | retain any user-driven zoom. | uniformtext | :class:`plotly.graph_objects.layout.Uniformtext | ` instance or dict with compatible properties | updatemenus | A tuple of | :class:`plotly.graph_objects.layout.Updatemenu` | instances or dicts with compatible properties | updatemenudefaults | When used in a template (as | layout.template.layout.updatemenudefaults), | sets the default property values to use for | elements of layout.updatemenus | violingap | Sets the gap (in plot fraction) between violins | of adjacent location coordinates. Has no effect | on traces that have "width" set. | violingroupgap | Sets the gap (in plot fraction) between violins | of the same location coordinate. Has no effect | on traces that have "width" set. | violinmode | Determines how violins at the same location | coordinate are displayed on the graph. If | "group", the violins are plotted next to one | another centered around the shared location. If | "overlay", the violins are plotted over one | another, you might need to set "opacity" to see | them multiple violins. Has no effect on traces | that have "width" set. | waterfallgap | Sets the gap (in plot fraction) between bars of | adjacent location coordinates. | waterfallgroupgap | Sets the gap (in plot fraction) between bars of | the same location coordinate. | waterfallmode | Determines how bars at the same location | coordinate are displayed on the graph. With | "group", the bars are plotted next to one | another centered around the shared location. | With "overlay", the bars are plotted over one | another, you might need to reduce "opacity" to | see multiple bars. | width | Sets the plot's width (in px). | xaxis | :class:`plotly.graph_objects.layout.XAxis` | instance or dict with compatible properties | yaxis | :class:`plotly.graph_objects.layout.YAxis` | instance or dict with compatible properties | | frames | The 'frames' property is a tuple of instances of | Frame that may be specified as: | - A list or tuple of instances of plotly.graph_objs.Frame | - A list or tuple of dicts of string/value properties that | will be passed to the Frame constructor | | Supported dict properties: | | baseframe | The name of the frame into which this frame's | properties are merged before applying. This is | used to unify properties and avoid needing to | specify the same values for the same properties | in multiple frames. | data | A list of traces this frame modifies. The | format is identical to the normal trace | definition. | group | An identifier that specifies the group to which | the frame belongs, used by animate to select a | subset of frames. | layout | Layout properties which this frame modifies. | The format is identical to the normal layout | definition. | name | A label by which to identify the frame | traces | A list of trace indices that identify the | respective traces in the data attribute | | skip_invalid: bool | If True, invalid properties in the figure specification will be | skipped silently. If False (default) invalid properties in the | figure specification will result in a ValueError | | Raises | ------ | ValueError | if a property in the specification of data, layout, or frames | is invalid AND skip_invalid is False | | add_annotation(self, arg=None, align=None, arrowcolor=None, arrowhead=None, arrowside=None, arrowsize=None, arrowwidth=None, ax=None, axref=None, ay=None, ayref=None, bgcolor=None, bordercolor=None, borderpad=None, borderwidth=None, captureevents=None, clicktoshow=None, font=None, height=None, hoverlabel=None, hovertext=None, name=None, opacity=None, showarrow=None, standoff=None, startarrowhead=None, startarrowsize=None, startstandoff=None, templateitemname=None, text=None, textangle=None, valign=None, visible=None, width=None, x=None, xanchor=None, xclick=None, xref=None, xshift=None, y=None, yanchor=None, yclick=None, yref=None, yshift=None, row=None, col=None, secondary_y=None, exclude_empty_subplots=None, **kwargs) -> 'FigureWidget' | Create and add a new annotation to the figure's layout | | Parameters | ---------- | arg | instance of Annotation or dict with compatible | properties | align | Sets the horizontal alignment of the `text` within the | box. Has an effect only if `text` spans two or more | lines (i.e. `text` contains one or more <br> HTML tags) | or if an explicit width is set to override the text | width. | arrowcolor | Sets the color of the annotation arrow. | arrowhead | Sets the end annotation arrow head style. | arrowside | Sets the annotation arrow head position. | arrowsize | Sets the size of the end annotation arrow head, | relative to `arrowwidth`. A value of 1 (default) gives | a head about 3x as wide as the line. | arrowwidth | Sets the width (in px) of annotation arrow line. | ax | Sets the x component of the arrow tail about the arrow | head. If `axref` is `pixel`, a positive (negative) | component corresponds to an arrow pointing from right | to left (left to right). If `axref` is not `pixel` and | is exactly the same as `xref`, this is an absolute | value on that axis, like `x`, specified in the same | coordinates as `xref`. | axref | Indicates in what coordinates the tail of the | annotation (ax,ay) is specified. If set to a x axis id | (e.g. "x" or "x2"), the `x` position refers to a x | coordinate. If set to "paper", the `x` position refers | to the distance from the left of the plotting area in | normalized coordinates where 0 (1) corresponds to the | left (right). If set to a x axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the left of the | domain of that axis: e.g., *x2 domain* refers to the | domain of the second x axis and a x position of 0.5 | refers to the point between the left and the right of | the domain of the second x axis. In order for absolute | positioning of the arrow to work, "axref" must be | exactly the same as "xref", otherwise "axref" will | revert to "pixel" (explained next). For relative | positioning, "axref" can be set to "pixel", in which | case the "ax" value is specified in pixels relative to | "x". Absolute positioning is useful for trendline | annotations which should continue to indicate the | correct trend when zoomed. Relative positioning is | useful for specifying the text offset for an annotated | point. | ay | Sets the y component of the arrow tail about the arrow | head. If `ayref` is `pixel`, a positive (negative) | component corresponds to an arrow pointing from bottom | to top (top to bottom). If `ayref` is not `pixel` and | is exactly the same as `yref`, this is an absolute | value on that axis, like `y`, specified in the same | coordinates as `yref`. | ayref | Indicates in what coordinates the tail of the | annotation (ax,ay) is specified. If set to a y axis id | (e.g. "y" or "y2"), the `y` position refers to a y | coordinate. If set to "paper", the `y` position refers | to the distance from the bottom of the plotting area in | normalized coordinates where 0 (1) corresponds to the | bottom (top). If set to a y axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the bottom of the | domain of that axis: e.g., *y2 domain* refers to the | domain of the second y axis and a y position of 0.5 | refers to the point between the bottom and the top of | the domain of the second y axis. In order for absolute | positioning of the arrow to work, "ayref" must be | exactly the same as "yref", otherwise "ayref" will | revert to "pixel" (explained next). For relative | positioning, "ayref" can be set to "pixel", in which | case the "ay" value is specified in pixels relative to | "y". Absolute positioning is useful for trendline | annotations which should continue to indicate the | correct trend when zoomed. Relative positioning is | useful for specifying the text offset for an annotated | point. | bgcolor | Sets the background color of the annotation. | bordercolor | Sets the color of the border enclosing the annotation | `text`. | borderpad | Sets the padding (in px) between the `text` and the | enclosing border. | borderwidth | Sets the width (in px) of the border enclosing the | annotation `text`. | captureevents | Determines whether the annotation text box captures | mouse move and click events, or allows those events to | pass through to data points in the plot that may be | behind the annotation. By default `captureevents` is | False unless `hovertext` is provided. If you use the | event `plotly_clickannotation` without `hovertext` you | must explicitly enable `captureevents`. | clicktoshow | Makes this annotation respond to clicks on the plot. If | you click a data point that exactly matches the `x` and | `y` values of this annotation, and it is hidden | (visible: false), it will appear. In "onoff" mode, you | must click the same point again to make it disappear, | so if you click multiple points, you can show multiple | annotations. In "onout" mode, a click anywhere else in | the plot (on another data point or not) will hide this | annotation. If you need to show/hide this annotation in | response to different `x` or `y` values, you can set | `xclick` and/or `yclick`. This is useful for example to | label the side of a bar. To label markers though, | `standoff` is preferred over `xclick` and `yclick`. | font | Sets the annotation text font. | height | Sets an explicit height for the text box. null | (default) lets the text set the box height. Taller text | will be clipped. | hoverlabel | :class:`plotly.graph_objects.layout.annotation.Hoverlab | el` instance or dict with compatible properties | hovertext | Sets text to appear when hovering over this annotation. | If omitted or blank, no hover label will appear. | name | When used in a template, named items are created in the | output figure in addition to any items the figure | already has in this array. You can modify these items | in the output figure by making your own item with | `templateitemname` matching this `name` alongside your | modifications (including `visible: false` or `enabled: | false` to hide it). Has no effect outside of a | template. | opacity | Sets the opacity of the annotation (text + arrow). | showarrow | Determines whether or not the annotation is drawn with | an arrow. If True, `text` is placed near the arrow's | tail. If False, `text` lines up with the `x` and `y` | provided. | standoff | Sets a distance, in pixels, to move the end arrowhead | away from the position it is pointing at, for example | to point at the edge of a marker independent of zoom. | Note that this shortens the arrow from the `ax` / `ay` | vector, in contrast to `xshift` / `yshift` which moves | everything by this amount. | startarrowhead | Sets the start annotation arrow head style. | startarrowsize | Sets the size of the start annotation arrow head, | relative to `arrowwidth`. A value of 1 (default) gives | a head about 3x as wide as the line. | startstandoff | Sets a distance, in pixels, to move the start arrowhead | away from the position it is pointing at, for example | to point at the edge of a marker independent of zoom. | Note that this shortens the arrow from the `ax` / `ay` | vector, in contrast to `xshift` / `yshift` which moves | everything by this amount. | templateitemname | Used to refer to a named item in this array in the | template. Named items from the template will be created | even without a matching item in the input figure, but | you can modify one by making an item with | `templateitemname` matching its `name`, alongside your | modifications (including `visible: false` or `enabled: | false` to hide it). If there is no template or no | matching item, this item will be hidden unless you | explicitly show it with `visible: true`. | text | Sets the text associated with this annotation. Plotly | uses a subset of HTML tags to do things like newline | (<br>), bold (<b></b>), italics (<i></i>), hyperlinks | (<a href='...'></a>). Tags <em>, <sup>, <sub>, <s>, <u> | <span> are also supported. | textangle | Sets the angle at which the `text` is drawn with | respect to the horizontal. | valign | Sets the vertical alignment of the `text` within the | box. Has an effect only if an explicit height is set to | override the text height. | visible | Determines whether or not this annotation is visible. | width | Sets an explicit width for the text box. null (default) | lets the text set the box width. Wider text will be | clipped. There is no automatic wrapping; use <br> to | start a new line. | x | Sets the annotation's x position. If the axis `type` is | "log", then you must take the log of your desired | range. If the axis `type` is "date", it should be date | strings, like date data, though Date objects and unix | milliseconds will be accepted and converted to strings. | If the axis `type` is "category", it should be numbers, | using the scale where each category is assigned a | serial number from zero in the order it appears. | xanchor | Sets the text box's horizontal position anchor This | anchor binds the `x` position to the "left", "center" | or "right" of the annotation. For example, if `x` is | set to 1, `xref` to "paper" and `xanchor` to "right" | then the right-most portion of the annotation lines up | with the right-most edge of the plotting area. If | "auto", the anchor is equivalent to "center" for data- | referenced annotations or if there is an arrow, whereas | for paper-referenced with no arrow, the anchor picked | corresponds to the closest side. | xclick | Toggle this annotation when clicking a data point whose | `x` value is `xclick` rather than the annotation's `x` | value. | xref | Sets the annotation's x coordinate axis. If set to a x | axis id (e.g. "x" or "x2"), the `x` position refers to | a x coordinate. If set to "paper", the `x` position | refers to the distance from the left of the plotting | area in normalized coordinates where 0 (1) corresponds | to the left (right). If set to a x axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the left of the | domain of that axis: e.g., *x2 domain* refers to the | domain of the second x axis and a x position of 0.5 | refers to the point between the left and the right of | the domain of the second x axis. | xshift | Shifts the position of the whole annotation and arrow | to the right (positive) or left (negative) by this many | pixels. | y | Sets the annotation's y position. If the axis `type` is | "log", then you must take the log of your desired | range. If the axis `type` is "date", it should be date | strings, like date data, though Date objects and unix | milliseconds will be accepted and converted to strings. | If the axis `type` is "category", it should be numbers, | using the scale where each category is assigned a | serial number from zero in the order it appears. | yanchor | Sets the text box's vertical position anchor This | anchor binds the `y` position to the "top", "middle" or | "bottom" of the annotation. For example, if `y` is set | to 1, `yref` to "paper" and `yanchor` to "top" then the | top-most portion of the annotation lines up with the | top-most edge of the plotting area. If "auto", the | anchor is equivalent to "middle" for data-referenced | annotations or if there is an arrow, whereas for paper- | referenced with no arrow, the anchor picked corresponds | to the closest side. | yclick | Toggle this annotation when clicking a data point whose | `y` value is `yclick` rather than the annotation's `y` | value. | yref | Sets the annotation's y coordinate axis. If set to a y | axis id (e.g. "y" or "y2"), the `y` position refers to | a y coordinate. If set to "paper", the `y` position | refers to the distance from the bottom of the plotting | area in normalized coordinates where 0 (1) corresponds | to the bottom (top). If set to a y axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the bottom of the | domain of that axis: e.g., *y2 domain* refers to the | domain of the second y axis and a y position of 0.5 | refers to the point between the bottom and the top of | the domain of the second y axis. | yshift | Shifts the position of the whole annotation and arrow | up (positive) or down (negative) by this many pixels. | row | Subplot row for annotation. If 'all', addresses all | rows in the specified column(s). | col | Subplot column for annotation. If 'all', addresses all | columns in the specified row(s). | secondary_y | Whether to add annotation to secondary y-axis | exclude_empty_subplots | If True, annotation will not be added to subplots | without traces. | | Returns | ------- | FigureWidget | | add_bar(self, alignmentgroup=None, base=None, basesrc=None, cliponaxis=None, constraintext=None, customdata=None, customdatasrc=None, dx=None, dy=None, error_x=None, error_y=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, insidetextanchor=None, insidetextfont=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, offset=None, offsetgroup=None, offsetsrc=None, opacity=None, orientation=None, outsidetextfont=None, selected=None, selectedpoints=None, showlegend=None, stream=None, text=None, textangle=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, width=None, widthsrc=None, x=None, x0=None, xaxis=None, xcalendar=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, y=None, y0=None, yaxis=None, ycalendar=None, yhoverformat=None, yperiod=None, yperiod0=None, yperiodalignment=None, ysrc=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Bar trace | | The data visualized by the span of the bars is set in `y` if | `orientation` is set to "v" (the default) and the labels are | set in `x`. By setting `orientation` to "h", the roles are | interchanged. | | Parameters | ---------- | alignmentgroup | Set several traces linked to the same position axis or | matching axes to the same alignmentgroup. This controls | whether bars compute their positional range dependently | or independently. | base | Sets where the bar base is drawn (in position axis | units). In "stack" or "relative" barmode, traces that | set "base" will be excluded and drawn in "overlay" mode | instead. | basesrc | Sets the source reference on Chart Studio Cloud for | `base`. | cliponaxis | Determines whether the text nodes are clipped about the | subplot axes. To show the text nodes above axis lines | and tick labels, make sure to set `xaxis.layer` and | `yaxis.layer` to *below traces*. | constraintext | Constrain the size of text inside or outside a bar to | be no larger than the bar itself. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Sets the x coordinate step. See `x0` for more info. | dy | Sets the y coordinate step. See `y0` for more info. | error_x | :class:`plotly.graph_objects.bar.ErrorX` instance or | dict with compatible properties | error_y | :class:`plotly.graph_objects.bar.ErrorY` instance or | dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.bar.Hoverlabel` instance | or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `value` and `label`. Anything contained in | tag `<extra>` is displayed in the secondary box, for | example "<extra>{fullData.name}</extra>". To hide the | secondary box completely, use an empty tag | `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (x,y) | pair. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | insidetextanchor | Determines if texts are kept at center or start/end | points in `textposition` "inside" mode. | insidetextfont | Sets the font used for `text` lying inside the bar. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.bar.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.bar.Marker` instance or | dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | offset | Shifts the position where the bar is drawn (in position | axis units). In "group" barmode, traces that set | "offset" will be excluded and drawn in "overlay" mode | instead. | offsetgroup | Set several traces linked to the same position axis or | matching axes to the same offsetgroup where bars of the | same position coordinate will line up. | offsetsrc | Sets the source reference on Chart Studio Cloud for | `offset`. | opacity | Sets the opacity of the trace. | orientation | Sets the orientation of the bars. With "v" ("h"), the | value of the each bar spans along the vertical | (horizontal). | outsidetextfont | Sets the font used for `text` lying outside the bar. | selected | :class:`plotly.graph_objects.bar.Selected` instance or | dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.bar.Stream` instance or | dict with compatible properties | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textangle | Sets the angle of the tick labels with respect to the | bar. For example, a `tickangle` of -90 draws the tick | labels vertically. With "auto" the texts may | automatically be rotated to fit with the maximum size | in bars. | textfont | Sets the font used for `text`. | textposition | Specifies the location of the `text`. "inside" | positions `text` inside, next to the bar end (rotated | and scaled if needed). "outside" positions `text` | outside, next to the bar end (scaled if needed), unless | there is another bar stacked on this one, then the text | gets pushed inside. "auto" tries to position `text` | inside the bar, but if the bar is too small and no bar | is stacked on this one the text is moved outside. If | "none", no text appears. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `value` and `label`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.bar.Unselected` instance | or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | width | Sets the bar width (in position axis units). | widthsrc | Sets the source reference on Chart Studio Cloud for | `width`. | x | Sets the x coordinates. | x0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates. | y0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | yperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the y | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | yperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the y0 axis. When `y0period` is round number | of weeks, the `y0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | yperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the y axis. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_barpolar(self, base=None, basesrc=None, customdata=None, customdatasrc=None, dr=None, dtheta=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, offset=None, offsetsrc=None, opacity=None, r=None, r0=None, rsrc=None, selected=None, selectedpoints=None, showlegend=None, stream=None, subplot=None, text=None, textsrc=None, theta=None, theta0=None, thetasrc=None, thetaunit=None, uid=None, uirevision=None, unselected=None, visible=None, width=None, widthsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Barpolar trace | | The data visualized by the radial span of the bars is set in | `r` | | Parameters | ---------- | base | Sets where the bar base is drawn (in radial axis | units). In "stack" barmode, traces that set "base" will | be excluded and drawn in "overlay" mode instead. | basesrc | Sets the source reference on Chart Studio Cloud for | `base`. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dr | Sets the r coordinate step. | dtheta | Sets the theta coordinate step. By default, the | `dtheta` step equals the subplot's period divided by | the length of the `r` coordinates. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.barpolar.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.barpolar.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.barpolar.Marker` instance | or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | offset | Shifts the angular position where the bar is drawn (in | "thetatunit" units). | offsetsrc | Sets the source reference on Chart Studio Cloud for | `offset`. | opacity | Sets the opacity of the trace. | r | Sets the radial coordinates | r0 | Alternate to `r`. Builds a linear space of r | coordinates. Use with `dr` where `r0` is the starting | coordinate and `dr` the step. | rsrc | Sets the source reference on Chart Studio Cloud for | `r`. | selected | :class:`plotly.graph_objects.barpolar.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.barpolar.Stream` instance | or dict with compatible properties | subplot | Sets a reference between this trace's data coordinates | and a polar subplot. If "polar" (the default value), | the data refer to `layout.polar`. If "polar2", the data | refer to `layout.polar2`, and so on. | text | Sets hover text elements associated with each bar. If a | single string, the same string appears over all bars. | If an array of string, the items are mapped in order to | the this trace's coordinates. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | theta | Sets the angular coordinates | theta0 | Alternate to `theta`. Builds a linear space of theta | coordinates. Use with `dtheta` where `theta0` is the | starting coordinate and `dtheta` the step. | thetasrc | Sets the source reference on Chart Studio Cloud for | `theta`. | thetaunit | Sets the unit of input "theta" values. Has an effect | only when on "linear" angular axes. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.barpolar.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | width | Sets the bar angular width (in "thetaunit" units). | widthsrc | Sets the source reference on Chart Studio Cloud for | `width`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_box(self, alignmentgroup=None, boxmean=None, boxpoints=None, customdata=None, customdatasrc=None, dx=None, dy=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoveron=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, jitter=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, lowerfence=None, lowerfencesrc=None, marker=None, mean=None, meansrc=None, median=None, mediansrc=None, meta=None, metasrc=None, name=None, notched=None, notchspan=None, notchspansrc=None, notchwidth=None, offsetgroup=None, opacity=None, orientation=None, pointpos=None, q1=None, q1src=None, q3=None, q3src=None, quartilemethod=None, sd=None, sdmultiple=None, sdsrc=None, selected=None, selectedpoints=None, showlegend=None, showwhiskers=None, sizemode=None, stream=None, text=None, textsrc=None, uid=None, uirevision=None, unselected=None, upperfence=None, upperfencesrc=None, visible=None, whiskerwidth=None, width=None, x=None, x0=None, xaxis=None, xcalendar=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, y=None, y0=None, yaxis=None, ycalendar=None, yhoverformat=None, yperiod=None, yperiod0=None, yperiodalignment=None, ysrc=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Box trace | | Each box spans from quartile 1 (Q1) to quartile 3 (Q3). The | second quartile (Q2, i.e. the median) is marked by a line | inside the box. The fences grow outward from the boxes' edges, | by default they span +/- 1.5 times the interquartile range | (IQR: Q3-Q1), The sample mean and standard deviation as well as | notches and the sample, outlier and suspected outliers points | can be optionally added to the box plot. The values and | positions corresponding to each boxes can be input using two | signatures. The first signature expects users to supply the | sample values in the `y` data array for vertical boxes (`x` for | horizontal boxes). By supplying an `x` (`y`) array, one box per | distinct `x` (`y`) value is drawn If no `x` (`y`) list is | provided, a single box is drawn. In this case, the box is | positioned with the trace `name` or with `x0` (`y0`) if | provided. The second signature expects users to supply the | boxes corresponding Q1, median and Q3 statistics in the `q1`, | `median` and `q3` data arrays respectively. Other box features | relying on statistics namely `lowerfence`, `upperfence`, | `notchspan` can be set directly by the users. To have plotly | compute them or to show sample points besides the boxes, users | can set the `y` data array for vertical boxes (`x` for | horizontal boxes) to a 2D array with the outer length | corresponding to the number of boxes in the traces and the | inner length corresponding the sample size. | | Parameters | ---------- | alignmentgroup | Set several traces linked to the same position axis or | matching axes to the same alignmentgroup. This controls | whether bars compute their positional range dependently | or independently. | boxmean | If True, the mean of the box(es)' underlying | distribution is drawn as a dashed line inside the | box(es). If "sd" the standard deviation is also drawn. | Defaults to True when `mean` is set. Defaults to "sd" | when `sd` is set Otherwise defaults to False. | boxpoints | If "outliers", only the sample points lying outside the | whiskers are shown If "suspectedoutliers", the outlier | points are shown and points either less than 4*Q1-3*Q3 | or greater than 4*Q3-3*Q1 are highlighted (see | `outliercolor`) If "all", all sample points are shown | If False, only the box(es) are shown with no sample | points Defaults to "suspectedoutliers" when | `marker.outliercolor` or `marker.line.outliercolor` is | set. Defaults to "all" under the q1/median/q3 | signature. Otherwise defaults to "outliers". | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Sets the x coordinate step for multi-box traces set | using q1/median/q3. | dy | Sets the y coordinate step for multi-box traces set | using q1/median/q3. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.box.Hoverlabel` instance | or dict with compatible properties | hoveron | Do the hover effects highlight individual boxes or | sample points or both? | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | jitter | Sets the amount of jitter in the sample points drawn. | If 0, the sample points align along the distribution | axis. If 1, the sample points are drawn in a random | jitter of width equal to the width of the box(es). | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.box.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.box.Line` instance or dict | with compatible properties | lowerfence | Sets the lower fence values. There should be as many | items as the number of boxes desired. This attribute | has effect only under the q1/median/q3 signature. If | `lowerfence` is not provided but a sample (in `y` or | `x`) is set, we compute the lower as the last sample | point below 1.5 times the IQR. | lowerfencesrc | Sets the source reference on Chart Studio Cloud for | `lowerfence`. | marker | :class:`plotly.graph_objects.box.Marker` instance or | dict with compatible properties | mean | Sets the mean values. There should be as many items as | the number of boxes desired. This attribute has effect | only under the q1/median/q3 signature. If `mean` is not | provided but a sample (in `y` or `x`) is set, we | compute the mean for each box using the sample values. | meansrc | Sets the source reference on Chart Studio Cloud for | `mean`. | median | Sets the median values. There should be as many items | as the number of boxes desired. | mediansrc | Sets the source reference on Chart Studio Cloud for | `median`. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. For box traces, the name will | also be used for the position coordinate, if `x` and | `x0` (`y` and `y0` if horizontal) are missing and the | position axis is categorical | notched | Determines whether or not notches are drawn. Notches | displays a confidence interval around the median. We | compute the confidence interval as median +/- 1.57 * | IQR / sqrt(N), where IQR is the interquartile range and | N is the sample size. If two boxes' notches do not | overlap there is 95% confidence their medians differ. | See https://sites.google.com/site/davidsstatistics/home | /notched-box-plots for more info. Defaults to False | unless `notchwidth` or `notchspan` is set. | notchspan | Sets the notch span from the boxes' `median` values. | There should be as many items as the number of boxes | desired. This attribute has effect only under the | q1/median/q3 signature. If `notchspan` is not provided | but a sample (in `y` or `x`) is set, we compute it as | 1.57 * IQR / sqrt(N), where N is the sample size. | notchspansrc | Sets the source reference on Chart Studio Cloud for | `notchspan`. | notchwidth | Sets the width of the notches relative to the box' | width. For example, with 0, the notches are as wide as | the box(es). | offsetgroup | Set several traces linked to the same position axis or | matching axes to the same offsetgroup where bars of the | same position coordinate will line up. | opacity | Sets the opacity of the trace. | orientation | Sets the orientation of the box(es). If "v" ("h"), the | distribution is visualized along the vertical | (horizontal). | pointpos | Sets the position of the sample points in relation to | the box(es). If 0, the sample points are places over | the center of the box(es). Positive (negative) values | correspond to positions to the right (left) for | vertical boxes and above (below) for horizontal boxes | q1 | Sets the Quartile 1 values. There should be as many | items as the number of boxes desired. | q1src | Sets the source reference on Chart Studio Cloud for | `q1`. | q3 | Sets the Quartile 3 values. There should be as many | items as the number of boxes desired. | q3src | Sets the source reference on Chart Studio Cloud for | `q3`. | quartilemethod | Sets the method used to compute the sample's Q1 and Q3 | quartiles. The "linear" method uses the 25th percentile | for Q1 and 75th percentile for Q3 as computed using | method #10 (listed on | http://jse.amstat.org/v14n3/langford.html). The | "exclusive" method uses the median to divide the | ordered dataset into two halves if the sample is odd, | it does not include the median in either half - Q1 is | then the median of the lower half and Q3 the median of | the upper half. The "inclusive" method also uses the | median to divide the ordered dataset into two halves | but if the sample is odd, it includes the median in | both halves - Q1 is then the median of the lower half | and Q3 the median of the upper half. | sd | Sets the standard deviation values. There should be as | many items as the number of boxes desired. This | attribute has effect only under the q1/median/q3 | signature. If `sd` is not provided but a sample (in `y` | or `x`) is set, we compute the standard deviation for | each box using the sample values. | sdmultiple | Scales the box size when sizemode=sd Allowing boxes to | be drawn across any stddev range For example 1-stddev, | 3-stddev, 5-stddev | sdsrc | Sets the source reference on Chart Studio Cloud for | `sd`. | selected | :class:`plotly.graph_objects.box.Selected` instance or | dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showwhiskers | Determines whether or not whiskers are visible. | Defaults to true for `sizemode` "quartiles", false for | "sd". | sizemode | Sets the upper and lower bound for the boxes quartiles | means box is drawn between Q1 and Q3 SD means the box | is drawn between Mean +- Standard Deviation Argument | sdmultiple (default 1) to scale the box size So it | could be drawn 1-stddev, 3-stddev etc | stream | :class:`plotly.graph_objects.box.Stream` instance or | dict with compatible properties | text | Sets the text elements associated with each sample | value. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.box.Unselected` instance | or dict with compatible properties | upperfence | Sets the upper fence values. There should be as many | items as the number of boxes desired. This attribute | has effect only under the q1/median/q3 signature. If | `upperfence` is not provided but a sample (in `y` or | `x`) is set, we compute the upper as the last sample | point above 1.5 times the IQR. | upperfencesrc | Sets the source reference on Chart Studio Cloud for | `upperfence`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | whiskerwidth | Sets the width of the whiskers relative to the box' | width. For example, with 1, the whiskers are as wide as | the box(es). | width | Sets the width of the box in data coordinate If 0 | (default value) the width is automatically selected | based on the positions of other box traces in the same | subplot. | x | Sets the x sample data or coordinates. See overview for | more info. | x0 | Sets the x coordinate for single-box traces or the | starting coordinate for multi-box traces set using | q1/median/q3. See overview for more info. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y sample data or coordinates. See overview for | more info. | y0 | Sets the y coordinate for single-box traces or the | starting coordinate for multi-box traces set using | q1/median/q3. See overview for more info. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | yperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the y | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | yperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the y0 axis. When `y0period` is round number | of weeks, the `y0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | yperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the y axis. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_candlestick(self, close=None, closesrc=None, customdata=None, customdatasrc=None, decreasing=None, high=None, highsrc=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, increasing=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, low=None, lowsrc=None, meta=None, metasrc=None, name=None, opacity=None, open=None, opensrc=None, selectedpoints=None, showlegend=None, stream=None, text=None, textsrc=None, uid=None, uirevision=None, visible=None, whiskerwidth=None, x=None, xaxis=None, xcalendar=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, yaxis=None, yhoverformat=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Candlestick trace | | The candlestick is a style of financial chart describing open, | high, low and close for a given `x` coordinate (most likely | time). The boxes represent the spread between the `open` and | `close` values and the lines represent the spread between the | `low` and `high` values Sample points where the close value is | higher (lower) then the open value are called increasing | (decreasing). By default, increasing candles are drawn in green | whereas decreasing are drawn in red. | | Parameters | ---------- | close | Sets the close values. | closesrc | Sets the source reference on Chart Studio Cloud for | `close`. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | decreasing | :class:`plotly.graph_objects.candlestick.Decreasing` | instance or dict with compatible properties | high | Sets the high values. | highsrc | Sets the source reference on Chart Studio Cloud for | `high`. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.candlestick.Hoverlabel` | instance or dict with compatible properties | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | increasing | :class:`plotly.graph_objects.candlestick.Increasing` | instance or dict with compatible properties | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.candlestick.Legendgrouptit | le` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.candlestick.Line` instance | or dict with compatible properties | low | Sets the low values. | lowsrc | Sets the source reference on Chart Studio Cloud for | `low`. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | open | Sets the open values. | opensrc | Sets the source reference on Chart Studio Cloud for | `open`. | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.candlestick.Stream` | instance or dict with compatible properties | text | Sets hover text elements associated with each sample | point. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to this trace's sample points. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | whiskerwidth | Sets the width of the whiskers relative to the box' | width. For example, with 1, the whiskers are as wide as | the box(es). | x | Sets the x coordinates. If absent, linear coordinate | will be generated. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_carpet(self, a=None, a0=None, aaxis=None, asrc=None, b=None, b0=None, baxis=None, bsrc=None, carpet=None, cheaterslope=None, color=None, customdata=None, customdatasrc=None, da=None, db=None, font=None, ids=None, idssrc=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, meta=None, metasrc=None, name=None, opacity=None, stream=None, uid=None, uirevision=None, visible=None, x=None, xaxis=None, xsrc=None, y=None, yaxis=None, ysrc=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Carpet trace | | The data describing carpet axis layout is set in `y` and | (optionally) also `x`. If only `y` is present, `x` the plot is | interpreted as a cheater plot and is filled in using the `y` | values. `x` and `y` may either be 2D arrays matching with each | dimension matching that of `a` and `b`, or they may be 1D | arrays with total length equal to that of `a` and `b`. | | Parameters | ---------- | a | An array containing values of the first parameter value | a0 | Alternate to `a`. Builds a linear space of a | coordinates. Use with `da` where `a0` is the starting | coordinate and `da` the step. | aaxis | :class:`plotly.graph_objects.carpet.Aaxis` instance or | dict with compatible properties | asrc | Sets the source reference on Chart Studio Cloud for | `a`. | b | A two dimensional array of y coordinates at each carpet | point. | b0 | Alternate to `b`. Builds a linear space of a | coordinates. Use with `db` where `b0` is the starting | coordinate and `db` the step. | baxis | :class:`plotly.graph_objects.carpet.Baxis` instance or | dict with compatible properties | bsrc | Sets the source reference on Chart Studio Cloud for | `b`. | carpet | An identifier for this carpet, so that `scattercarpet` | and `contourcarpet` traces can specify a carpet plot on | which they lie | cheaterslope | The shift applied to each successive row of data in | creating a cheater plot. Only used if `x` is been | omitted. | color | Sets default for all colors associated with this axis | all at once: line, font, tick, and grid colors. Grid | color is lightened by blending this with the plot | background Individual pieces can override this. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | da | Sets the a coordinate step. See `a0` for more info. | db | Sets the b coordinate step. See `b0` for more info. | font | The default font used for axis & tick labels on this | carpet | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.carpet.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | stream | :class:`plotly.graph_objects.carpet.Stream` instance or | dict with compatible properties | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | A two dimensional array of x coordinates at each carpet | point. If omitted, the plot is a cheater plot and the | xaxis is hidden by default. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | A two dimensional array of y coordinates at each carpet | point. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_choropleth(self, autocolorscale=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, featureidkey=None, geo=None, geojson=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, locationmode=None, locations=None, locationssrc=None, marker=None, meta=None, metasrc=None, name=None, reversescale=None, selected=None, selectedpoints=None, showlegend=None, showscale=None, stream=None, text=None, textsrc=None, uid=None, uirevision=None, unselected=None, visible=None, z=None, zauto=None, zmax=None, zmid=None, zmin=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Choropleth trace | | The data that describes the choropleth value-to-color mapping | is set in `z`. The geographic locations corresponding to each | value in `z` are set in `locations`. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.choropleth.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | featureidkey | Sets the key in GeoJSON features which is used as id to | match the items included in the `locations` array. Only | has an effect when `geojson` is set. Support nested | property, for example "properties.name". | geo | Sets a reference between this trace's geospatial | coordinates and a geographic map. If "geo" (the default | value), the geospatial coordinates refer to | `layout.geo`. If "geo2", the geospatial coordinates | refer to `layout.geo2`, and so on. | geojson | Sets optional GeoJSON data associated with this trace. | If not given, the features on the base map are used. It | can be set as a valid GeoJSON object or as a URL | string. Note that we only accept GeoJSONs of type | "FeatureCollection" or "Feature" with geometries of | type "Polygon" or "MultiPolygon". | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.choropleth.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.choropleth.Legendgrouptitl | e` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | locationmode | Determines the set of locations used to match entries | in `locations` to regions on the map. Values "ISO-3", | "USA-states", *country names* correspond to features on | the base map and value "geojson-id" corresponds to | features from a custom GeoJSON linked to the `geojson` | attribute. | locations | Sets the coordinates via location IDs or names. See | `locationmode` for more info. | locationssrc | Sets the source reference on Chart Studio Cloud for | `locations`. | marker | :class:`plotly.graph_objects.choropleth.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | selected | :class:`plotly.graph_objects.choropleth.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.choropleth.Stream` | instance or dict with compatible properties | text | Sets the text elements associated with each location. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.choropleth.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | z | Sets the color values. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_choroplethmap(self, autocolorscale=None, below=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, featureidkey=None, geojson=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, locations=None, locationssrc=None, marker=None, meta=None, metasrc=None, name=None, reversescale=None, selected=None, selectedpoints=None, showlegend=None, showscale=None, stream=None, subplot=None, text=None, textsrc=None, uid=None, uirevision=None, unselected=None, visible=None, z=None, zauto=None, zmax=None, zmid=None, zmin=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Choroplethmap trace | | GeoJSON features to be filled are set in `geojson` The data | that describes the choropleth value-to-color mapping is set in | `locations` and `z`. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | below | Determines if the choropleth polygons will be inserted | before the layer with the specified ID. By default, | choroplethmap traces are placed above the water layers. | If set to '', the layer will be inserted above every | existing layer. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.choroplethmap.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | featureidkey | Sets the key in GeoJSON features which is used as id to | match the items included in the `locations` array. | Support nested property, for example "properties.name". | geojson | Sets the GeoJSON data associated with this trace. It | can be set as a valid GeoJSON object or as a URL | string. Note that we only accept GeoJSONs of type | "FeatureCollection" or "Feature" with geometries of | type "Polygon" or "MultiPolygon". | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.choroplethmap.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variable `properties` Anything contained in tag | `<extra>` is displayed in the secondary box, for | example "<extra>{fullData.name}</extra>". To hide the | secondary box completely, use an empty tag | `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.choroplethmap.Legendgroupt | itle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | locations | Sets which features found in "geojson" to plot using | their feature `id` field. | locationssrc | Sets the source reference on Chart Studio Cloud for | `locations`. | marker | :class:`plotly.graph_objects.choroplethmap.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | selected | :class:`plotly.graph_objects.choroplethmap.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.choroplethmap.Stream` | instance or dict with compatible properties | subplot | Sets a reference between this trace's data coordinates | and a map subplot. If "map" (the default value), the | data refer to `layout.map`. If "map2", the data refer | to `layout.map2`, and so on. | text | Sets the text elements associated with each location. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.choroplethmap.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | z | Sets the color values. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_choroplethmapbox(self, autocolorscale=None, below=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, featureidkey=None, geojson=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, locations=None, locationssrc=None, marker=None, meta=None, metasrc=None, name=None, reversescale=None, selected=None, selectedpoints=None, showlegend=None, showscale=None, stream=None, subplot=None, text=None, textsrc=None, uid=None, uirevision=None, unselected=None, visible=None, z=None, zauto=None, zmax=None, zmid=None, zmin=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Choroplethmapbox trace | | "choroplethmapbox" trace is deprecated! Please consider | switching to the "choroplethmap" trace type and `map` subplots. | Learn more at: https://plotly.com/javascript/maplibre- | migration/ GeoJSON features to be filled are set in `geojson` | The data that describes the choropleth value-to-color mapping | is set in `locations` and `z`. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | below | Determines if the choropleth polygons will be inserted | before the layer with the specified ID. By default, | choroplethmapbox traces are placed above the water | layers. If set to '', the layer will be inserted above | every existing layer. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.choroplethmapbox.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | featureidkey | Sets the key in GeoJSON features which is used as id to | match the items included in the `locations` array. | Support nested property, for example "properties.name". | geojson | Sets the GeoJSON data associated with this trace. It | can be set as a valid GeoJSON object or as a URL | string. Note that we only accept GeoJSONs of type | "FeatureCollection" or "Feature" with geometries of | type "Polygon" or "MultiPolygon". | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.choroplethmapbox.Hoverlabe | l` instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variable `properties` Anything contained in tag | `<extra>` is displayed in the secondary box, for | example "<extra>{fullData.name}</extra>". To hide the | secondary box completely, use an empty tag | `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.choroplethmapbox.Legendgro | uptitle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | locations | Sets which features found in "geojson" to plot using | their feature `id` field. | locationssrc | Sets the source reference on Chart Studio Cloud for | `locations`. | marker | :class:`plotly.graph_objects.choroplethmapbox.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | selected | :class:`plotly.graph_objects.choroplethmapbox.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.choroplethmapbox.Stream` | instance or dict with compatible properties | subplot | mapbox subplots and traces are deprecated! Please | consider switching to `map` subplots and traces. Learn | more at: https://plotly.com/javascript/maplibre- | migration/ Sets a reference between this trace's data | coordinates and a mapbox subplot. If "mapbox" (the | default value), the data refer to `layout.mapbox`. If | "mapbox2", the data refer to `layout.mapbox2`, and so | on. | text | Sets the text elements associated with each location. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.choroplethmapbox.Unselecte | d` instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | z | Sets the color values. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_cone(self, anchor=None, autocolorscale=None, cauto=None, cmax=None, cmid=None, cmin=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, lighting=None, lightposition=None, meta=None, metasrc=None, name=None, opacity=None, reversescale=None, scene=None, showlegend=None, showscale=None, sizemode=None, sizeref=None, stream=None, text=None, textsrc=None, u=None, uhoverformat=None, uid=None, uirevision=None, usrc=None, v=None, vhoverformat=None, visible=None, vsrc=None, w=None, whoverformat=None, wsrc=None, x=None, xhoverformat=None, xsrc=None, y=None, yhoverformat=None, ysrc=None, z=None, zhoverformat=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Cone trace | | Use cone traces to visualize vector fields. Specify a vector | field using 6 1D arrays, 3 position arrays `x`, `y` and `z` and | 3 vector component arrays `u`, `v`, `w`. The cones are drawn | exactly at the positions given by `x`, `y` and `z`. | | Parameters | ---------- | anchor | Sets the cones' anchor with respect to their x/y/z | positions. Note that "cm" denote the cone's center of | mass which corresponds to 1/4 from the tail to tip. | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | cauto | Determines whether or not the color domain is computed | with respect to the input data (here u/v/w norm) or the | bounds set in `cmin` and `cmax` Defaults to `false` | when `cmin` and `cmax` are set by the user. | cmax | Sets the upper bound of the color domain. Value should | have the same units as u/v/w norm and if set, `cmin` | must be set as well. | cmid | Sets the mid-point of the color domain by scaling | `cmin` and/or `cmax` to be equidistant to this point. | Value should have the same units as u/v/w norm. Has no | effect when `cauto` is `false`. | cmin | Sets the lower bound of the color domain. Value should | have the same units as u/v/w norm and if set, `cmax` | must be set as well. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.cone.ColorBar` instance or | dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `cmin` and `cmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.cone.Hoverlabel` instance | or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variable `norm` Anything contained in tag `<extra>` | is displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.cone.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | lighting | :class:`plotly.graph_objects.cone.Lighting` instance or | dict with compatible properties | lightposition | :class:`plotly.graph_objects.cone.Lightposition` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the surface. Please note that in | the case of using high `opacity` values for example a | value greater than or equal to 0.5 on two surfaces (and | 0.25 with four surfaces), an overlay of multiple | transparent surfaces may not perfectly be sorted in | depth by the webgl API. This behavior may be improved | in the near future and is subject to change. | reversescale | Reverses the color mapping if true. If true, `cmin` | will correspond to the last color in the array and | `cmax` will correspond to the first color. | scene | Sets a reference between this trace's 3D coordinate | system and a 3D scene. If "scene" (the default value), | the (x,y,z) coordinates refer to `layout.scene`. If | "scene2", the (x,y,z) coordinates refer to | `layout.scene2`, and so on. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | sizemode | Determines whether `sizeref` is set as a "scaled" (i.e | unitless) scalar (normalized by the max u/v/w norm in | the vector field) or as "absolute" value (in the same | units as the vector field). To display sizes in actual | vector length use "raw". | sizeref | Adjusts the cone size scaling. The size of the cones is | determined by their u/v/w norm multiplied a factor and | `sizeref`. This factor (computed internally) | corresponds to the minimum "time" to travel across two | successive x/y/z positions at the average velocity of | those two successive positions. All cones in a given | trace use the same factor. With `sizemode` set to | "raw", its default value is 1. With `sizemode` set to | "scaled", `sizeref` is unitless, its default value is | 0.5. With `sizemode` set to "absolute", `sizeref` has | the same units as the u/v/w vector field, its the | default value is half the sample's maximum vector norm. | stream | :class:`plotly.graph_objects.cone.Stream` instance or | dict with compatible properties | text | Sets the text elements associated with the cones. If | trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | u | Sets the x components of the vector field. | uhoverformat | Sets the hover text formatting rulefor `u` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | usrc | Sets the source reference on Chart Studio Cloud for | `u`. | v | Sets the y components of the vector field. | vhoverformat | Sets the hover text formatting rulefor `v` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | vsrc | Sets the source reference on Chart Studio Cloud for | `v`. | w | Sets the z components of the vector field. | whoverformat | Sets the hover text formatting rulefor `w` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | wsrc | Sets the source reference on Chart Studio Cloud for | `w`. | x | Sets the x coordinates of the vector field and of the | displayed cones. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates of the vector field and of the | displayed cones. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the z coordinates of the vector field and of the | displayed cones. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `zaxis.hoverformat`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_contour(self, autocolorscale=None, autocontour=None, coloraxis=None, colorbar=None, colorscale=None, connectgaps=None, contours=None, customdata=None, customdatasrc=None, dx=None, dy=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoverongaps=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, meta=None, metasrc=None, name=None, ncontours=None, opacity=None, reversescale=None, showlegend=None, showscale=None, stream=None, text=None, textfont=None, textsrc=None, texttemplate=None, transpose=None, uid=None, uirevision=None, visible=None, x=None, x0=None, xaxis=None, xcalendar=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, xtype=None, y=None, y0=None, yaxis=None, ycalendar=None, yhoverformat=None, yperiod=None, yperiod0=None, yperiodalignment=None, ysrc=None, ytype=None, z=None, zauto=None, zhoverformat=None, zmax=None, zmid=None, zmin=None, zorder=None, zsrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Contour trace | | The data from which contour lines are computed is set in `z`. | Data in `z` must be a 2D list of numbers. Say that `z` has N | rows and M columns, then by default, these N rows correspond to | N y coordinates (set in `y` or auto-generated) and the M | columns correspond to M x coordinates (set in `x` or auto- | generated). By setting `transpose` to True, the above behavior | is flipped. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | autocontour | Determines whether or not the contour level attributes | are picked by an algorithm. If True, the number of | contour levels can be set in `ncontours`. If False, set | the contour level attributes in `contours`. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.contour.ColorBar` instance | or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the `z` data are filled in. It is defaulted | to true if `z` is a one dimensional array otherwise it | is defaulted to false. | contours | :class:`plotly.graph_objects.contour.Contours` instance | or dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Sets the x coordinate step. See `x0` for more info. | dy | Sets the y coordinate step. See `y0` for more info. | fillcolor | Sets the fill color if `contours.type` is "constraint". | Defaults to a half-transparent variant of the line | color, marker color, or marker line color, whichever is | available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.contour.Hoverlabel` | instance or dict with compatible properties | hoverongaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the `z` data have hover labels associated | with them. | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.contour.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.contour.Line` instance or | dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | ncontours | Sets the maximum number of contour levels. The actual | number of contours will be chosen automatically to be | less than or equal to the value of `ncontours`. Has an | effect only if `autocontour` is True or if | `contours.size` is missing. | opacity | Sets the opacity of the trace. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.contour.Stream` instance | or dict with compatible properties | text | Sets the text elements associated with each z value. | textfont | For this trace it only has an effect if `coloring` is | set to "heatmap". Sets the text font. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | For this trace it only has an effect if `coloring` is | set to "heatmap". Template string used for rendering | the information text that appear on points. Note that | this will override `textinfo`. Variables are inserted | using %{variable}, for example "y: %{y}". Numbers are | formatted using d3-format's syntax | %{variable:d3-format}, for example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `x`, `y`, `z` and `text`. | transpose | Transposes the z data. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. | x0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | xtype | If "array", the heatmap's x coordinates are given by | "x" (the default behavior when `x` is provided). If | "scaled", the heatmap's x coordinates are given by "x0" | and "dx" (the default behavior when `x` is not | provided). | y | Sets the y coordinates. | y0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | yperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the y | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | yperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the y0 axis. When `y0period` is round number | of weeks, the `y0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | yperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the y axis. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | ytype | If "array", the heatmap's y coordinates are given by | "y" (the default behavior when `y` is provided) If | "scaled", the heatmap's y coordinates are given by "y0" | and "dy" (the default behavior when `y` is not | provided) | z | Sets the z data. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_contourcarpet(self, a=None, a0=None, asrc=None, atype=None, autocolorscale=None, autocontour=None, b=None, b0=None, bsrc=None, btype=None, carpet=None, coloraxis=None, colorbar=None, colorscale=None, contours=None, customdata=None, customdatasrc=None, da=None, db=None, fillcolor=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, meta=None, metasrc=None, name=None, ncontours=None, opacity=None, reversescale=None, showlegend=None, showscale=None, stream=None, text=None, textsrc=None, transpose=None, uid=None, uirevision=None, visible=None, xaxis=None, yaxis=None, z=None, zauto=None, zmax=None, zmid=None, zmin=None, zorder=None, zsrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Contourcarpet trace | | Plots contours on either the first carpet axis or the carpet | axis with a matching `carpet` attribute. Data `z` is | interpreted as matching that of the corresponding carpet axis. | | Parameters | ---------- | a | Sets the x coordinates. | a0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | asrc | Sets the source reference on Chart Studio Cloud for | `a`. | atype | If "array", the heatmap's x coordinates are given by | "x" (the default behavior when `x` is provided). If | "scaled", the heatmap's x coordinates are given by "x0" | and "dx" (the default behavior when `x` is not | provided). | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | autocontour | Determines whether or not the contour level attributes | are picked by an algorithm. If True, the number of | contour levels can be set in `ncontours`. If False, set | the contour level attributes in `contours`. | b | Sets the y coordinates. | b0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | bsrc | Sets the source reference on Chart Studio Cloud for | `b`. | btype | If "array", the heatmap's y coordinates are given by | "y" (the default behavior when `y` is provided) If | "scaled", the heatmap's y coordinates are given by "y0" | and "dy" (the default behavior when `y` is not | provided) | carpet | The `carpet` of the carpet axes on which this contour | trace lies | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.contourcarpet.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | contours | :class:`plotly.graph_objects.contourcarpet.Contours` | instance or dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | da | Sets the x coordinate step. See `x0` for more info. | db | Sets the y coordinate step. See `y0` for more info. | fillcolor | Sets the fill color if `contours.type` is "constraint". | Defaults to a half-transparent variant of the line | color, marker color, or marker line color, whichever is | available. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.contourcarpet.Legendgroupt | itle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.contourcarpet.Line` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | ncontours | Sets the maximum number of contour levels. The actual | number of contours will be chosen automatically to be | less than or equal to the value of `ncontours`. Has an | effect only if `autocontour` is True or if | `contours.size` is missing. | opacity | Sets the opacity of the trace. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.contourcarpet.Stream` | instance or dict with compatible properties | text | Sets the text elements associated with each z value. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | transpose | Transposes the z data. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | z | Sets the z data. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_densitymap(self, autocolorscale=None, below=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, lat=None, latsrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, lon=None, lonsrc=None, meta=None, metasrc=None, name=None, opacity=None, radius=None, radiussrc=None, reversescale=None, showlegend=None, showscale=None, stream=None, subplot=None, text=None, textsrc=None, uid=None, uirevision=None, visible=None, z=None, zauto=None, zmax=None, zmid=None, zmin=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Densitymap trace | | Draws a bivariate kernel density estimation with a Gaussian | kernel from `lon` and `lat` coordinates and optional `z` values | using a colorscale. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | below | Determines if the densitymap trace will be inserted | before the layer with the specified ID. By default, | densitymap traces are placed below the first layer of | type symbol If set to '', the layer will be inserted | above every existing layer. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.densitymap.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.densitymap.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (lon,lat) | pair If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (lon,lat) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | lat | Sets the latitude coordinates (in degrees North). | latsrc | Sets the source reference on Chart Studio Cloud for | `lat`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.densitymap.Legendgrouptitl | e` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | lon | Sets the longitude coordinates (in degrees East). | lonsrc | Sets the source reference on Chart Studio Cloud for | `lon`. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | radius | Sets the radius of influence of one `lon` / `lat` point | in pixels. Increasing the value makes the densitymap | trace smoother, but less detailed. | radiussrc | Sets the source reference on Chart Studio Cloud for | `radius`. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.densitymap.Stream` | instance or dict with compatible properties | subplot | Sets a reference between this trace's data coordinates | and a map subplot. If "map" (the default value), the | data refer to `layout.map`. If "map2", the data refer | to `layout.map2`, and so on. | text | Sets text elements associated with each (lon,lat) pair | If a single string, the same string appears over all | the data points. If an array of string, the items are | mapped in order to the this trace's (lon,lat) | coordinates. If trace `hoverinfo` contains a "text" | flag and "hovertext" is not set, these elements will be | seen in the hover labels. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | z | Sets the points' weight. For example, a value of 10 | would be equivalent to having 10 points of weight 1 in | the same spot | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_densitymapbox(self, autocolorscale=None, below=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, lat=None, latsrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, lon=None, lonsrc=None, meta=None, metasrc=None, name=None, opacity=None, radius=None, radiussrc=None, reversescale=None, showlegend=None, showscale=None, stream=None, subplot=None, text=None, textsrc=None, uid=None, uirevision=None, visible=None, z=None, zauto=None, zmax=None, zmid=None, zmin=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Densitymapbox trace | | "densitymapbox" trace is deprecated! Please consider switching | to the "densitymap" trace type and `map` subplots. Learn more | at: https://plotly.com/javascript/maplibre-migration/ Draws a | bivariate kernel density estimation with a Gaussian kernel from | `lon` and `lat` coordinates and optional `z` values using a | colorscale. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | below | Determines if the densitymapbox trace will be inserted | before the layer with the specified ID. By default, | densitymapbox traces are placed below the first layer | of type symbol If set to '', the layer will be inserted | above every existing layer. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.densitymapbox.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.densitymapbox.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (lon,lat) | pair If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (lon,lat) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | lat | Sets the latitude coordinates (in degrees North). | latsrc | Sets the source reference on Chart Studio Cloud for | `lat`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.densitymapbox.Legendgroupt | itle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | lon | Sets the longitude coordinates (in degrees East). | lonsrc | Sets the source reference on Chart Studio Cloud for | `lon`. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | radius | Sets the radius of influence of one `lon` / `lat` point | in pixels. Increasing the value makes the densitymapbox | trace smoother, but less detailed. | radiussrc | Sets the source reference on Chart Studio Cloud for | `radius`. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.densitymapbox.Stream` | instance or dict with compatible properties | subplot | mapbox subplots and traces are deprecated! Please | consider switching to `map` subplots and traces. Learn | more at: https://plotly.com/javascript/maplibre- | migration/ Sets a reference between this trace's data | coordinates and a mapbox subplot. If "mapbox" (the | default value), the data refer to `layout.mapbox`. If | "mapbox2", the data refer to `layout.mapbox2`, and so | on. | text | Sets text elements associated with each (lon,lat) pair | If a single string, the same string appears over all | the data points. If an array of string, the items are | mapped in order to the this trace's (lon,lat) | coordinates. If trace `hoverinfo` contains a "text" | flag and "hovertext" is not set, these elements will be | seen in the hover labels. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | z | Sets the points' weight. For example, a value of 10 | would be equivalent to having 10 points of weight 1 in | the same spot | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_funnel(self, alignmentgroup=None, cliponaxis=None, connector=None, constraintext=None, customdata=None, customdatasrc=None, dx=None, dy=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, insidetextanchor=None, insidetextfont=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, offset=None, offsetgroup=None, opacity=None, orientation=None, outsidetextfont=None, selectedpoints=None, showlegend=None, stream=None, text=None, textangle=None, textfont=None, textinfo=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, visible=None, width=None, x=None, x0=None, xaxis=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, y=None, y0=None, yaxis=None, yhoverformat=None, yperiod=None, yperiod0=None, yperiodalignment=None, ysrc=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Funnel trace | | Visualize stages in a process using length-encoded bars. This | trace can be used to show data in either a part-to-whole | representation wherein each item appears in a single stage, or | in a "drop-off" representation wherein each item appears in | each stage it traversed. See also the "funnelarea" trace type | for a different approach to visualizing funnel data. | | Parameters | ---------- | alignmentgroup | Set several traces linked to the same position axis or | matching axes to the same alignmentgroup. This controls | whether bars compute their positional range dependently | or independently. | cliponaxis | Determines whether the text nodes are clipped about the | subplot axes. To show the text nodes above axis lines | and tick labels, make sure to set `xaxis.layer` and | `yaxis.layer` to *below traces*. | connector | :class:`plotly.graph_objects.funnel.Connector` instance | or dict with compatible properties | constraintext | Constrain the size of text inside or outside a bar to | be no larger than the bar itself. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Sets the x coordinate step. See `x0` for more info. | dy | Sets the y coordinate step. See `y0` for more info. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.funnel.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `percentInitial`, `percentPrevious` and | `percentTotal`. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (x,y) | pair. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | insidetextanchor | Determines if texts are kept at center or start/end | points in `textposition` "inside" mode. | insidetextfont | Sets the font used for `text` lying inside the bar. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.funnel.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.funnel.Marker` instance or | dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | offset | Shifts the position where the bar is drawn (in position | axis units). In "group" barmode, traces that set | "offset" will be excluded and drawn in "overlay" mode | instead. | offsetgroup | Set several traces linked to the same position axis or | matching axes to the same offsetgroup where bars of the | same position coordinate will line up. | opacity | Sets the opacity of the trace. | orientation | Sets the orientation of the funnels. With "v" ("h"), | the value of the each bar spans along the vertical | (horizontal). By default funnels are tend to be | oriented horizontally; unless only "y" array is | presented or orientation is set to "v". Also regarding | graphs including only 'horizontal' funnels, "autorange" | on the "y-axis" are set to "reversed". | outsidetextfont | Sets the font used for `text` lying outside the bar. | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.funnel.Stream` instance or | dict with compatible properties | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textangle | Sets the angle of the tick labels with respect to the | bar. For example, a `tickangle` of -90 draws the tick | labels vertically. With "auto" the texts may | automatically be rotated to fit with the maximum size | in bars. | textfont | Sets the font used for `text`. | textinfo | Determines which trace information appear on the graph. | In the case of having multiple funnels, percentages & | totals are computed separately (per trace). | textposition | Specifies the location of the `text`. "inside" | positions `text` inside, next to the bar end (rotated | and scaled if needed). "outside" positions `text` | outside, next to the bar end (scaled if needed), unless | there is another bar stacked on this one, then the text | gets pushed inside. "auto" tries to position `text` | inside the bar, but if the bar is too small and no bar | is stacked on this one the text is moved outside. If | "none", no text appears. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `percentInitial`, `percentPrevious`, | `percentTotal`, `label` and `value`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | width | Sets the bar width (in position axis units). | x | Sets the x coordinates. | x0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates. | y0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | yperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the y | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | yperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the y0 axis. When `y0period` is round number | of weeks, the `y0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | yperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the y axis. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_funnelarea(self, aspectratio=None, baseratio=None, customdata=None, customdatasrc=None, dlabel=None, domain=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, insidetextfont=None, label0=None, labels=None, labelssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, opacity=None, scalegroup=None, showlegend=None, stream=None, text=None, textfont=None, textinfo=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, title=None, uid=None, uirevision=None, values=None, valuessrc=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Funnelarea trace | | Visualize stages in a process using area-encoded trapezoids. | This trace can be used to show data in a part-to-whole | representation similar to a "pie" trace, wherein each item | appears in a single stage. See also the "funnel" trace type for | a different approach to visualizing funnel data. | | Parameters | ---------- | aspectratio | Sets the ratio between height and width | baseratio | Sets the ratio between bottom length and maximum top | length. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dlabel | Sets the label step. See `label0` for more info. | domain | :class:`plotly.graph_objects.funnelarea.Domain` | instance or dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.funnelarea.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `label`, `color`, `value`, `text` and | `percent`. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each sector. | If a single string, the same string appears for all | data points. If an array of string, the items are | mapped in order of this trace's sectors. To be seen, | trace `hoverinfo` must contain a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | insidetextfont | Sets the font used for `textinfo` lying inside the | sector. | label0 | Alternate to `labels`. Builds a numeric set of labels. | Use with `dlabel` where `label0` is the starting label | and `dlabel` the step. | labels | Sets the sector labels. If `labels` entries are | duplicated, we sum associated `values` or simply count | occurrences if `values` is not provided. For other | array attributes (including color) we use the first | non-empty entry among all occurrences of the label. | labelssrc | Sets the source reference on Chart Studio Cloud for | `labels`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.funnelarea.Legendgrouptitl | e` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.funnelarea.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | scalegroup | If there are multiple funnelareas that should be sized | according to their totals, link them by providing a | non-empty group id here shared by every trace in the | same group. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.funnelarea.Stream` | instance or dict with compatible properties | text | Sets text elements associated with each sector. If | trace `textinfo` contains a "text" flag, these elements | will be seen on the chart. If trace `hoverinfo` | contains a "text" flag and "hovertext" is not set, | these elements will be seen in the hover labels. | textfont | Sets the font used for `textinfo`. | textinfo | Determines which trace information appear on the graph. | textposition | Specifies the location of the `textinfo`. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `label`, `color`, `value`, `text` and | `percent`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | title | :class:`plotly.graph_objects.funnelarea.Title` instance | or dict with compatible properties | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | values | Sets the values of the sectors. If omitted, we count | occurrences of each label. | valuessrc | Sets the source reference on Chart Studio Cloud for | `values`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_heatmap(self, autocolorscale=None, coloraxis=None, colorbar=None, colorscale=None, connectgaps=None, customdata=None, customdatasrc=None, dx=None, dy=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoverongaps=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, meta=None, metasrc=None, name=None, opacity=None, reversescale=None, showlegend=None, showscale=None, stream=None, text=None, textfont=None, textsrc=None, texttemplate=None, transpose=None, uid=None, uirevision=None, visible=None, x=None, x0=None, xaxis=None, xcalendar=None, xgap=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, xtype=None, y=None, y0=None, yaxis=None, ycalendar=None, ygap=None, yhoverformat=None, yperiod=None, yperiod0=None, yperiodalignment=None, ysrc=None, ytype=None, z=None, zauto=None, zhoverformat=None, zmax=None, zmid=None, zmin=None, zorder=None, zsmooth=None, zsrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Heatmap trace | | The data that describes the heatmap value-to-color mapping is | set in `z`. Data in `z` can either be a 2D list of values | (ragged or not) or a 1D array of values. In the case where `z` | is a 2D list, say that `z` has N rows and M columns. Then, by | default, the resulting heatmap will have N partitions along the | y axis and M partitions along the x axis. In other words, the | i-th row/ j-th column cell in `z` is mapped to the i-th | partition of the y axis (starting from the bottom of the plot) | and the j-th partition of the x-axis (starting from the left of | the plot). This behavior can be flipped by using `transpose`. | Moreover, `x` (`y`) can be provided with M or M+1 (N or N+1) | elements. If M (N), then the coordinates correspond to the | center of the heatmap cells and the cells have equal width. If | M+1 (N+1), then the coordinates correspond to the edges of the | heatmap cells. In the case where `z` is a 1D list, the x and y | coordinates must be provided in `x` and `y` respectively to | form data triplets. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.heatmap.ColorBar` instance | or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the `z` data are filled in. It is defaulted | to true if `z` is a one dimensional array and `zsmooth` | is not false; otherwise it is defaulted to false. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Sets the x coordinate step. See `x0` for more info. | dy | Sets the y coordinate step. See `y0` for more info. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.heatmap.Hoverlabel` | instance or dict with compatible properties | hoverongaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the `z` data have hover labels associated | with them. | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.heatmap.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.heatmap.Stream` instance | or dict with compatible properties | text | Sets the text elements associated with each z value. | textfont | Sets the text font. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `x`, `y`, `z` and `text`. | transpose | Transposes the z data. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. | x0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xcalendar | Sets the calendar system to use with `x` date data. | xgap | Sets the horizontal gap (in pixels) between bricks. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | xtype | If "array", the heatmap's x coordinates are given by | "x" (the default behavior when `x` is provided). If | "scaled", the heatmap's x coordinates are given by "x0" | and "dx" (the default behavior when `x` is not | provided). | y | Sets the y coordinates. | y0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ycalendar | Sets the calendar system to use with `y` date data. | ygap | Sets the vertical gap (in pixels) between bricks. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | yperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the y | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | yperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the y0 axis. When `y0period` is round number | of weeks, the `y0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | yperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the y axis. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | ytype | If "array", the heatmap's y coordinates are given by | "y" (the default behavior when `y` is provided) If | "scaled", the heatmap's y coordinates are given by "y0" | and "dy" (the default behavior when `y` is not | provided) | z | Sets the z data. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | zsmooth | Picks a smoothing algorithm use to smooth `z` data. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_heatmapgl(self, autocolorscale=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, dx=None, dy=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, ids=None, idssrc=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, meta=None, metasrc=None, name=None, opacity=None, reversescale=None, showscale=None, stream=None, text=None, textsrc=None, transpose=None, uid=None, uirevision=None, visible=None, x=None, x0=None, xaxis=None, xsrc=None, xtype=None, y=None, y0=None, yaxis=None, ysrc=None, ytype=None, z=None, zauto=None, zmax=None, zmid=None, zmin=None, zsmooth=None, zsrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Heatmapgl trace | | "heatmapgl" trace is deprecated! Please consider switching to | the "heatmap" or "image" trace types. Alternatively you could | contribute/sponsor rewriting this trace type based on cartesian | features and using regl framework. WebGL version of the heatmap | trace type. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.heatmapgl.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Sets the x coordinate step. See `x0` for more info. | dy | Sets the y coordinate step. See `y0` for more info. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.heatmapgl.Hoverlabel` | instance or dict with compatible properties | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.heatmapgl.Legendgrouptitle | ` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.heatmapgl.Stream` instance | or dict with compatible properties | text | Sets the text elements associated with each z value. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | transpose | Transposes the z data. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. | x0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | xtype | If "array", the heatmap's x coordinates are given by | "x" (the default behavior when `x` is provided). If | "scaled", the heatmap's x coordinates are given by "x0" | and "dx" (the default behavior when `x` is not | provided). | y | Sets the y coordinates. | y0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | ytype | If "array", the heatmap's y coordinates are given by | "y" (the default behavior when `y` is provided) If | "scaled", the heatmap's y coordinates are given by "y0" | and "dy" (the default behavior when `y` is not | provided) | z | Sets the z data. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zsmooth | Picks a smoothing algorithm use to smooth `z` data. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_histogram(self, alignmentgroup=None, autobinx=None, autobiny=None, bingroup=None, cliponaxis=None, constraintext=None, cumulative=None, customdata=None, customdatasrc=None, error_x=None, error_y=None, histfunc=None, histnorm=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, insidetextanchor=None, insidetextfont=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, nbinsx=None, nbinsy=None, offsetgroup=None, opacity=None, orientation=None, outsidetextfont=None, selected=None, selectedpoints=None, showlegend=None, stream=None, text=None, textangle=None, textfont=None, textposition=None, textsrc=None, texttemplate=None, uid=None, uirevision=None, unselected=None, visible=None, x=None, xaxis=None, xbins=None, xcalendar=None, xhoverformat=None, xsrc=None, y=None, yaxis=None, ybins=None, ycalendar=None, yhoverformat=None, ysrc=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Histogram trace | | The sample data from which statistics are computed is set in | `x` for vertically spanning histograms and in `y` for | horizontally spanning histograms. Binning options are set | `xbins` and `ybins` respectively if no aggregation data is | provided. | | Parameters | ---------- | alignmentgroup | Set several traces linked to the same position axis or | matching axes to the same alignmentgroup. This controls | whether bars compute their positional range dependently | or independently. | autobinx | Obsolete: since v1.42 each bin attribute is auto- | determined separately and `autobinx` is not needed. | However, we accept `autobinx: true` or `false` and will | update `xbins` accordingly before deleting `autobinx` | from the trace. | autobiny | Obsolete: since v1.42 each bin attribute is auto- | determined separately and `autobiny` is not needed. | However, we accept `autobiny: true` or `false` and will | update `ybins` accordingly before deleting `autobiny` | from the trace. | bingroup | Set a group of histogram traces which will have | compatible bin settings. Note that traces on the same | subplot and with the same "orientation" under `barmode` | "stack", "relative" and "group" are forced into the | same bingroup, Using `bingroup`, traces under `barmode` | "overlay" and on different axes (of the same axis type) | can have compatible bin settings. Note that histogram | and histogram2d* trace can share the same `bingroup` | cliponaxis | Determines whether the text nodes are clipped about the | subplot axes. To show the text nodes above axis lines | and tick labels, make sure to set `xaxis.layer` and | `yaxis.layer` to *below traces*. | constraintext | Constrain the size of text inside or outside a bar to | be no larger than the bar itself. | cumulative | :class:`plotly.graph_objects.histogram.Cumulative` | instance or dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | error_x | :class:`plotly.graph_objects.histogram.ErrorX` instance | or dict with compatible properties | error_y | :class:`plotly.graph_objects.histogram.ErrorY` instance | or dict with compatible properties | histfunc | Specifies the binning function used for this histogram | trace. If "count", the histogram values are computed by | counting the number of values lying inside each bin. If | "sum", "avg", "min", "max", the histogram values are | computed using the sum, the average, the minimum or the | maximum of the values lying inside each bin | respectively. | histnorm | Specifies the type of normalization used for this | histogram trace. If "", the span of each bar | corresponds to the number of occurrences (i.e. the | number of data points lying inside the bins). If | "percent" / "probability", the span of each bar | corresponds to the percentage / fraction of occurrences | with respect to the total number of sample points | (here, the sum of all bin HEIGHTS equals 100% / 1). If | "density", the span of each bar corresponds to the | number of occurrences in a bin divided by the size of | the bin interval (here, the sum of all bin AREAS equals | the total number of sample points). If *probability | density*, the area of each bar corresponds to the | probability that an event will fall into the | corresponding bin (here, the sum of all bin AREAS | equals 1). | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.histogram.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variable `binNumber` Anything contained in tag | `<extra>` is displayed in the secondary box, for | example "<extra>{fullData.name}</extra>". To hide the | secondary box completely, use an empty tag | `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | insidetextanchor | Determines if texts are kept at center or start/end | points in `textposition` "inside" mode. | insidetextfont | Sets the font used for `text` lying inside the bar. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.histogram.Legendgrouptitle | ` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.histogram.Marker` instance | or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | nbinsx | Specifies the maximum number of desired bins. This | value will be used in an algorithm that will decide the | optimal bin size such that the histogram best | visualizes the distribution of the data. Ignored if | `xbins.size` is provided. | nbinsy | Specifies the maximum number of desired bins. This | value will be used in an algorithm that will decide the | optimal bin size such that the histogram best | visualizes the distribution of the data. Ignored if | `ybins.size` is provided. | offsetgroup | Set several traces linked to the same position axis or | matching axes to the same offsetgroup where bars of the | same position coordinate will line up. | opacity | Sets the opacity of the trace. | orientation | Sets the orientation of the bars. With "v" ("h"), the | value of the each bar spans along the vertical | (horizontal). | outsidetextfont | Sets the font used for `text` lying outside the bar. | selected | :class:`plotly.graph_objects.histogram.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.histogram.Stream` instance | or dict with compatible properties | text | Sets hover text elements associated with each bar. If a | single string, the same string appears over all bars. | If an array of string, the items are mapped in order to | the this trace's coordinates. | textangle | Sets the angle of the tick labels with respect to the | bar. For example, a `tickangle` of -90 draws the tick | labels vertically. With "auto" the texts may | automatically be rotated to fit with the maximum size | in bars. | textfont | Sets the text font. | textposition | Specifies the location of the `text`. "inside" | positions `text` inside, next to the bar end (rotated | and scaled if needed). "outside" positions `text` | outside, next to the bar end (scaled if needed), unless | there is another bar stacked on this one, then the text | gets pushed inside. "auto" tries to position `text` | inside the bar, but if the bar is too small and no bar | is stacked on this one the text is moved outside. If | "none", no text appears. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `label` and `value`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.histogram.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the sample data to be binned on the x axis. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xbins | :class:`plotly.graph_objects.histogram.XBins` instance | or dict with compatible properties | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the sample data to be binned on the y axis. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ybins | :class:`plotly.graph_objects.histogram.YBins` instance | or dict with compatible properties | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_histogram2d(self, autobinx=None, autobiny=None, autocolorscale=None, bingroup=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, histfunc=None, histnorm=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, nbinsx=None, nbinsy=None, opacity=None, reversescale=None, showlegend=None, showscale=None, stream=None, textfont=None, texttemplate=None, uid=None, uirevision=None, visible=None, x=None, xaxis=None, xbingroup=None, xbins=None, xcalendar=None, xgap=None, xhoverformat=None, xsrc=None, y=None, yaxis=None, ybingroup=None, ybins=None, ycalendar=None, ygap=None, yhoverformat=None, ysrc=None, z=None, zauto=None, zhoverformat=None, zmax=None, zmid=None, zmin=None, zsmooth=None, zsrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Histogram2d trace | | The sample data from which statistics are computed is set in | `x` and `y` (where `x` and `y` represent marginal | distributions, binning is set in `xbins` and `ybins` in this | case) or `z` (where `z` represent the 2D distribution and | binning set, binning is set by `x` and `y` in this case). The | resulting distribution is visualized as a heatmap. | | Parameters | ---------- | autobinx | Obsolete: since v1.42 each bin attribute is auto- | determined separately and `autobinx` is not needed. | However, we accept `autobinx: true` or `false` and will | update `xbins` accordingly before deleting `autobinx` | from the trace. | autobiny | Obsolete: since v1.42 each bin attribute is auto- | determined separately and `autobiny` is not needed. | However, we accept `autobiny: true` or `false` and will | update `ybins` accordingly before deleting `autobiny` | from the trace. | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | bingroup | Set the `xbingroup` and `ybingroup` default prefix For | example, setting a `bingroup` of 1 on two histogram2d | traces will make them their x-bins and y-bins match | separately. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.histogram2d.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | histfunc | Specifies the binning function used for this histogram | trace. If "count", the histogram values are computed by | counting the number of values lying inside each bin. If | "sum", "avg", "min", "max", the histogram values are | computed using the sum, the average, the minimum or the | maximum of the values lying inside each bin | respectively. | histnorm | Specifies the type of normalization used for this | histogram trace. If "", the span of each bar | corresponds to the number of occurrences (i.e. the | number of data points lying inside the bins). If | "percent" / "probability", the span of each bar | corresponds to the percentage / fraction of occurrences | with respect to the total number of sample points | (here, the sum of all bin HEIGHTS equals 100% / 1). If | "density", the span of each bar corresponds to the | number of occurrences in a bin divided by the size of | the bin interval (here, the sum of all bin AREAS equals | the total number of sample points). If *probability | density*, the area of each bar corresponds to the | probability that an event will fall into the | corresponding bin (here, the sum of all bin AREAS | equals 1). | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.histogram2d.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variable `z` Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.histogram2d.Legendgrouptit | le` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.histogram2d.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | nbinsx | Specifies the maximum number of desired bins. This | value will be used in an algorithm that will decide the | optimal bin size such that the histogram best | visualizes the distribution of the data. Ignored if | `xbins.size` is provided. | nbinsy | Specifies the maximum number of desired bins. This | value will be used in an algorithm that will decide the | optimal bin size such that the histogram best | visualizes the distribution of the data. Ignored if | `ybins.size` is provided. | opacity | Sets the opacity of the trace. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.histogram2d.Stream` | instance or dict with compatible properties | textfont | Sets the text font. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variable `z` | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the sample data to be binned on the x axis. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xbingroup | Set a group of histogram traces which will have | compatible x-bin settings. Using `xbingroup`, | histogram2d and histogram2dcontour traces (on axes of | the same axis type) can have compatible x-bin settings. | Note that the same `xbingroup` value can be used to set | (1D) histogram `bingroup` | xbins | :class:`plotly.graph_objects.histogram2d.XBins` | instance or dict with compatible properties | xcalendar | Sets the calendar system to use with `x` date data. | xgap | Sets the horizontal gap (in pixels) between bricks. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the sample data to be binned on the y axis. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ybingroup | Set a group of histogram traces which will have | compatible y-bin settings. Using `ybingroup`, | histogram2d and histogram2dcontour traces (on axes of | the same axis type) can have compatible y-bin settings. | Note that the same `ybingroup` value can be used to set | (1D) histogram `bingroup` | ybins | :class:`plotly.graph_objects.histogram2d.YBins` | instance or dict with compatible properties | ycalendar | Sets the calendar system to use with `y` date data. | ygap | Sets the vertical gap (in pixels) between bricks. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the aggregation data. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zsmooth | Picks a smoothing algorithm use to smooth `z` data. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_histogram2dcontour(self, autobinx=None, autobiny=None, autocolorscale=None, autocontour=None, bingroup=None, coloraxis=None, colorbar=None, colorscale=None, contours=None, customdata=None, customdatasrc=None, histfunc=None, histnorm=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, name=None, nbinsx=None, nbinsy=None, ncontours=None, opacity=None, reversescale=None, showlegend=None, showscale=None, stream=None, textfont=None, texttemplate=None, uid=None, uirevision=None, visible=None, x=None, xaxis=None, xbingroup=None, xbins=None, xcalendar=None, xhoverformat=None, xsrc=None, y=None, yaxis=None, ybingroup=None, ybins=None, ycalendar=None, yhoverformat=None, ysrc=None, z=None, zauto=None, zhoverformat=None, zmax=None, zmid=None, zmin=None, zsrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Histogram2dContour trace | | The sample data from which statistics are computed is set in | `x` and `y` (where `x` and `y` represent marginal | distributions, binning is set in `xbins` and `ybins` in this | case) or `z` (where `z` represent the 2D distribution and | binning set, binning is set by `x` and `y` in this case). The | resulting distribution is visualized as a contour plot. | | Parameters | ---------- | autobinx | Obsolete: since v1.42 each bin attribute is auto- | determined separately and `autobinx` is not needed. | However, we accept `autobinx: true` or `false` and will | update `xbins` accordingly before deleting `autobinx` | from the trace. | autobiny | Obsolete: since v1.42 each bin attribute is auto- | determined separately and `autobiny` is not needed. | However, we accept `autobiny: true` or `false` and will | update `ybins` accordingly before deleting `autobiny` | from the trace. | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | autocontour | Determines whether or not the contour level attributes | are picked by an algorithm. If True, the number of | contour levels can be set in `ncontours`. If False, set | the contour level attributes in `contours`. | bingroup | Set the `xbingroup` and `ybingroup` default prefix For | example, setting a `bingroup` of 1 on two histogram2d | traces will make them their x-bins and y-bins match | separately. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.histogram2dcontour.ColorBa | r` instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `zmin` and `zmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | contours | :class:`plotly.graph_objects.histogram2dcontour.Contour | s` instance or dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | histfunc | Specifies the binning function used for this histogram | trace. If "count", the histogram values are computed by | counting the number of values lying inside each bin. If | "sum", "avg", "min", "max", the histogram values are | computed using the sum, the average, the minimum or the | maximum of the values lying inside each bin | respectively. | histnorm | Specifies the type of normalization used for this | histogram trace. If "", the span of each bar | corresponds to the number of occurrences (i.e. the | number of data points lying inside the bins). If | "percent" / "probability", the span of each bar | corresponds to the percentage / fraction of occurrences | with respect to the total number of sample points | (here, the sum of all bin HEIGHTS equals 100% / 1). If | "density", the span of each bar corresponds to the | number of occurrences in a bin divided by the size of | the bin interval (here, the sum of all bin AREAS equals | the total number of sample points). If *probability | density*, the area of each bar corresponds to the | probability that an event will fall into the | corresponding bin (here, the sum of all bin AREAS | equals 1). | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.histogram2dcontour.Hoverla | bel` instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variable `z` Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.histogram2dcontour.Legendg | rouptitle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.histogram2dcontour.Line` | instance or dict with compatible properties | marker | :class:`plotly.graph_objects.histogram2dcontour.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | nbinsx | Specifies the maximum number of desired bins. This | value will be used in an algorithm that will decide the | optimal bin size such that the histogram best | visualizes the distribution of the data. Ignored if | `xbins.size` is provided. | nbinsy | Specifies the maximum number of desired bins. This | value will be used in an algorithm that will decide the | optimal bin size such that the histogram best | visualizes the distribution of the data. Ignored if | `ybins.size` is provided. | ncontours | Sets the maximum number of contour levels. The actual | number of contours will be chosen automatically to be | less than or equal to the value of `ncontours`. Has an | effect only if `autocontour` is True or if | `contours.size` is missing. | opacity | Sets the opacity of the trace. | reversescale | Reverses the color mapping if true. If true, `zmin` | will correspond to the last color in the array and | `zmax` will correspond to the first color. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.histogram2dcontour.Stream` | instance or dict with compatible properties | textfont | For this trace it only has an effect if `coloring` is | set to "heatmap". Sets the text font. | texttemplate | For this trace it only has an effect if `coloring` is | set to "heatmap". Template string used for rendering | the information text that appear on points. Note that | this will override `textinfo`. Variables are inserted | using %{variable}, for example "y: %{y}". Numbers are | formatted using d3-format's syntax | %{variable:d3-format}, for example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `x`, `y`, `z` and `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the sample data to be binned on the x axis. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xbingroup | Set a group of histogram traces which will have | compatible x-bin settings. Using `xbingroup`, | histogram2d and histogram2dcontour traces (on axes of | the same axis type) can have compatible x-bin settings. | Note that the same `xbingroup` value can be used to set | (1D) histogram `bingroup` | xbins | :class:`plotly.graph_objects.histogram2dcontour.XBins` | instance or dict with compatible properties | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the sample data to be binned on the y axis. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ybingroup | Set a group of histogram traces which will have | compatible y-bin settings. Using `ybingroup`, | histogram2d and histogram2dcontour traces (on axes of | the same axis type) can have compatible y-bin settings. | Note that the same `ybingroup` value can be used to set | (1D) histogram `bingroup` | ybins | :class:`plotly.graph_objects.histogram2dcontour.YBins` | instance or dict with compatible properties | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the aggregation data. | zauto | Determines whether or not the color domain is computed | with respect to the input data (here in `z`) or the | bounds set in `zmin` and `zmax` Defaults to `false` | when `zmin` and `zmax` are set by the user. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | zmax | Sets the upper bound of the color domain. Value should | have the same units as in `z` and if set, `zmin` must | be set as well. | zmid | Sets the mid-point of the color domain by scaling | `zmin` and/or `zmax` to be equidistant to this point. | Value should have the same units as in `z`. Has no | effect when `zauto` is `false`. | zmin | Sets the lower bound of the color domain. Value should | have the same units as in `z` and if set, `zmax` must | be set as well. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_hline(self, y, row='all', col='all', exclude_empty_subplots=True, annotation=None, **kwargs) -> 'FigureWidget' | Add a horizontal line to a plot or subplot that extends infinitely in the | x-dimension. | | Parameters | ---------- | y: float or int | A number representing the y coordinate of the horizontal line. | exclude_empty_subplots: Boolean | If True (default) do not place the shape on subplots that have no data | plotted on them. | row: None, int or 'all' | Subplot row for shape indexed starting at 1. If 'all', addresses all rows in | the specified column(s). If both row and col are None, addresses the | first subplot if subplots exist, or the only plot. By default is "all". | col: None, int or 'all' | Subplot column for shape indexed starting at 1. If 'all', addresses all rows in | the specified column(s). If both row and col are None, addresses the | first subplot if subplots exist, or the only plot. By default is "all". | annotation: dict or plotly.graph_objects.layout.Annotation. If dict(), | it is interpreted as describing an annotation. The annotation is | placed relative to the shape based on annotation_position (see | below) unless its x or y value has been specified for the annotation | passed here. xref and yref are always the same as for the added | shape and cannot be overridden. | annotation_position: a string containing optionally ["top", "bottom"] | and ["left", "right"] specifying where the text should be anchored | to on the line. Example positions are "bottom left", "right top", | "right", "bottom". If an annotation is added but annotation_position is | not specified, this defaults to "top right". | annotation_*: any parameters to go.layout.Annotation can be passed as | keywords by prefixing them with "annotation_". For example, to specify the | annotation text "example" you can pass annotation_text="example" as a | keyword argument. | **kwargs: | Any named function parameters that can be passed to 'add_shape', | except for x0, x1, y0, y1 or type. | | add_hrect(self, y0, y1, row='all', col='all', exclude_empty_subplots=True, annotation=None, **kwargs) -> 'FigureWidget' | Add a rectangle to a plot or subplot that extends infinitely in the | x-dimension. | | Parameters | ---------- | y0: float or int | A number representing the y coordinate of one side of the rectangle. | y1: float or int | A number representing the y coordinate of the other side of the rectangle. | exclude_empty_subplots: Boolean | If True (default) do not place the shape on subplots that have no data | plotted on them. | row: None, int or 'all' | Subplot row for shape indexed starting at 1. If 'all', addresses all rows in | the specified column(s). If both row and col are None, addresses the | first subplot if subplots exist, or the only plot. By default is "all". | col: None, int or 'all' | Subplot column for shape indexed starting at 1. If 'all', addresses all rows in | the specified column(s). If both row and col are None, addresses the | first subplot if subplots exist, or the only plot. By default is "all". | annotation: dict or plotly.graph_objects.layout.Annotation. If dict(), | it is interpreted as describing an annotation. The annotation is | placed relative to the shape based on annotation_position (see | below) unless its x or y value has been specified for the annotation | passed here. xref and yref are always the same as for the added | shape and cannot be overridden. | annotation_position: a string containing optionally ["inside", "outside"], ["top", "bottom"] | and ["left", "right"] specifying where the text should be anchored | to on the rectangle. Example positions are "outside top left", "inside | bottom", "right", "inside left", "inside" ("outside" is not supported). If | an annotation is added but annotation_position is not specified this | defaults to "inside top right". | annotation_*: any parameters to go.layout.Annotation can be passed as | keywords by prefixing them with "annotation_". For example, to specify the | annotation text "example" you can pass annotation_text="example" as a | keyword argument. | **kwargs: | Any named function parameters that can be passed to 'add_shape', | except for x0, x1, y0, y1 or type. | | add_icicle(self, branchvalues=None, count=None, customdata=None, customdatasrc=None, domain=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, insidetextfont=None, labels=None, labelssrc=None, leaf=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, level=None, marker=None, maxdepth=None, meta=None, metasrc=None, name=None, opacity=None, outsidetextfont=None, parents=None, parentssrc=None, pathbar=None, root=None, sort=None, stream=None, text=None, textfont=None, textinfo=None, textposition=None, textsrc=None, texttemplate=None, texttemplatesrc=None, tiling=None, uid=None, uirevision=None, values=None, valuessrc=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Icicle trace | | Visualize hierarchal data from leaves (and/or outer branches) | towards root with rectangles. The icicle sectors are determined | by the entries in "labels" or "ids" and in "parents". | | Parameters | ---------- | branchvalues | Determines how the items in `values` are summed. When | set to "total", items in `values` are taken to be value | of all its descendants. When set to "remainder", items | in `values` corresponding to the root and the branches | sectors are taken to be the extra part not part of the | sum of the values at their leaves. | count | Determines default for `values` when it is not | provided, by inferring a 1 for each of the "leaves" | and/or "branches", otherwise 0. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | domain | :class:`plotly.graph_objects.icicle.Domain` instance or | dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.icicle.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `currentPath`, `root`, `entry`, | `percentRoot`, `percentEntry` and `percentParent`. | Anything contained in tag `<extra>` is displayed in the | secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each sector. | If a single string, the same string appears for all | data points. If an array of string, the items are | mapped in order of this trace's sectors. To be seen, | trace `hoverinfo` must contain a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | insidetextfont | Sets the font used for `textinfo` lying inside the | sector. | labels | Sets the labels of each of the sectors. | labelssrc | Sets the source reference on Chart Studio Cloud for | `labels`. | leaf | :class:`plotly.graph_objects.icicle.Leaf` instance or | dict with compatible properties | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.icicle.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | level | Sets the level from which this trace hierarchy is | rendered. Set `level` to `''` to start from the root | node in the hierarchy. Must be an "id" if `ids` is | filled in, otherwise plotly attempts to find a matching | item in `labels`. | marker | :class:`plotly.graph_objects.icicle.Marker` instance or | dict with compatible properties | maxdepth | Sets the number of rendered sectors from any given | `level`. Set `maxdepth` to "-1" to render all the | levels in the hierarchy. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | outsidetextfont | Sets the font used for `textinfo` lying outside the | sector. This option refers to the root of the hierarchy | presented on top left corner of a treemap graph. Please | note that if a hierarchy has multiple root nodes, this | option won't have any effect and `insidetextfont` would | be used. | parents | Sets the parent sectors for each of the sectors. Empty | string items '' are understood to reference the root | node in the hierarchy. If `ids` is filled, `parents` | items are understood to be "ids" themselves. When `ids` | is not set, plotly attempts to find matching items in | `labels`, but beware they must be unique. | parentssrc | Sets the source reference on Chart Studio Cloud for | `parents`. | pathbar | :class:`plotly.graph_objects.icicle.Pathbar` instance | or dict with compatible properties | root | :class:`plotly.graph_objects.icicle.Root` instance or | dict with compatible properties | sort | Determines whether or not the sectors are reordered | from largest to smallest. | stream | :class:`plotly.graph_objects.icicle.Stream` instance or | dict with compatible properties | text | Sets text elements associated with each sector. If | trace `textinfo` contains a "text" flag, these elements | will be seen on the chart. If trace `hoverinfo` | contains a "text" flag and "hovertext" is not set, | these elements will be seen in the hover labels. | textfont | Sets the font used for `textinfo`. | textinfo | Determines which trace information appear on the graph. | textposition | Sets the positions of the `text` elements. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `currentPath`, `root`, `entry`, | `percentRoot`, `percentEntry`, `percentParent`, `label` | and `value`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | tiling | :class:`plotly.graph_objects.icicle.Tiling` instance or | dict with compatible properties | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | values | Sets the values associated with each of the sectors. | Use with `branchvalues` to determine how the values are | summed. | valuessrc | Sets the source reference on Chart Studio Cloud for | `values`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_image(self, colormodel=None, customdata=None, customdatasrc=None, dx=None, dy=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, meta=None, metasrc=None, name=None, opacity=None, source=None, stream=None, text=None, textsrc=None, uid=None, uirevision=None, visible=None, x0=None, xaxis=None, y0=None, yaxis=None, z=None, zmax=None, zmin=None, zorder=None, zsmooth=None, zsrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Image trace | | Display an image, i.e. data on a 2D regular raster. By default, | when an image is displayed in a subplot, its y axis will be | reversed (ie. `autorange: 'reversed'`), constrained to the | domain (ie. `constrain: 'domain'`) and it will have the same | scale as its x axis (ie. `scaleanchor: 'x,`) in order for | pixels to be rendered as squares. | | Parameters | ---------- | colormodel | Color model used to map the numerical color components | described in `z` into colors. If `source` is specified, | this attribute will be set to `rgba256` otherwise it | defaults to `rgb`. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Set the pixel's horizontal size. | dy | Set the pixel's vertical size | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.image.Hoverlabel` instance | or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `z`, `color` and `colormodel`. Anything | contained in tag `<extra>` is displayed in the | secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.image.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | source | Specifies the data URI of the image to be visualized. | The URI consists of "data:image/[<media | subtype>][;base64],<data>" | stream | :class:`plotly.graph_objects.image.Stream` instance or | dict with compatible properties | text | Sets the text elements associated with each z value. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x0 | Set the image's x position. The left edge of the image | (or the right edge if the x axis is reversed or dx is | negative) will be found at xmin=x0-dx/2 | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | y0 | Set the image's y position. The top edge of the image | (or the bottom edge if the y axis is NOT reversed or if | dy is negative) will be found at ymin=y0-dy/2. By | default when an image trace is included, the y axis | will be reversed so that the image is right-side-up, | but you can disable this by setting | yaxis.autorange=true or by providing an explicit y axis | range. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | z | A 2-dimensional array in which each element is an array | of 3 or 4 numbers representing a color. | zmax | Array defining the higher bound for each color | component. Note that the default value will depend on | the colormodel. For the `rgb` colormodel, it is [255, | 255, 255]. For the `rgba` colormodel, it is [255, 255, | 255, 1]. For the `rgba256` colormodel, it is [255, 255, | 255, 255]. For the `hsl` colormodel, it is [360, 100, | 100]. For the `hsla` colormodel, it is [360, 100, 100, | 1]. | zmin | Array defining the lower bound for each color | component. Note that the default value will depend on | the colormodel. For the `rgb` colormodel, it is [0, 0, | 0]. For the `rgba` colormodel, it is [0, 0, 0, 0]. For | the `rgba256` colormodel, it is [0, 0, 0, 0]. For the | `hsl` colormodel, it is [0, 0, 0]. For the `hsla` | colormodel, it is [0, 0, 0, 0]. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | zsmooth | Picks a smoothing algorithm used to smooth `z` data. | This only applies for image traces that use the | `source` attribute. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_indicator(self, align=None, customdata=None, customdatasrc=None, delta=None, domain=None, gauge=None, ids=None, idssrc=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, meta=None, metasrc=None, mode=None, name=None, number=None, stream=None, title=None, uid=None, uirevision=None, value=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Indicator trace | | An indicator is used to visualize a single `value` along with | some contextual information such as `steps` or a `threshold`, | using a combination of three visual elements: a number, a | delta, and/or a gauge. Deltas are taken with respect to a | `reference`. Gauges can be either angular or bullet (aka | linear) gauges. | | Parameters | ---------- | align | Sets the horizontal alignment of the `text` within the | box. Note that this attribute has no effect if an | angular gauge is displayed: in this case, it is always | centered | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | delta | :class:`plotly.graph_objects.indicator.Delta` instance | or dict with compatible properties | domain | :class:`plotly.graph_objects.indicator.Domain` instance | or dict with compatible properties | gauge | The gauge of the Indicator plot. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.indicator.Legendgrouptitle | ` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines how the value is displayed on the graph. | `number` displays the value numerically in text. | `delta` displays the difference to a reference value in | text. Finally, `gauge` displays the value graphically | on an axis. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | number | :class:`plotly.graph_objects.indicator.Number` instance | or dict with compatible properties | stream | :class:`plotly.graph_objects.indicator.Stream` instance | or dict with compatible properties | title | :class:`plotly.graph_objects.indicator.Title` instance | or dict with compatible properties | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | value | Sets the number to be displayed. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_isosurface(self, autocolorscale=None, caps=None, cauto=None, cmax=None, cmid=None, cmin=None, coloraxis=None, colorbar=None, colorscale=None, contour=None, customdata=None, customdatasrc=None, flatshading=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, isomax=None, isomin=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, lighting=None, lightposition=None, meta=None, metasrc=None, name=None, opacity=None, reversescale=None, scene=None, showlegend=None, showscale=None, slices=None, spaceframe=None, stream=None, surface=None, text=None, textsrc=None, uid=None, uirevision=None, value=None, valuehoverformat=None, valuesrc=None, visible=None, x=None, xhoverformat=None, xsrc=None, y=None, yhoverformat=None, ysrc=None, z=None, zhoverformat=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Isosurface trace | | Draws isosurfaces between iso-min and iso-max values with | coordinates given by four 1-dimensional arrays containing the | `value`, `x`, `y` and `z` of every vertex of a uniform or non- | uniform 3-D grid. Horizontal or vertical slices, caps as well | as spaceframe between iso-min and iso-max values could also be | drawn using this trace. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | caps | :class:`plotly.graph_objects.isosurface.Caps` instance | or dict with compatible properties | cauto | Determines whether or not the color domain is computed | with respect to the input data (here `value`) or the | bounds set in `cmin` and `cmax` Defaults to `false` | when `cmin` and `cmax` are set by the user. | cmax | Sets the upper bound of the color domain. Value should | have the same units as `value` and if set, `cmin` must | be set as well. | cmid | Sets the mid-point of the color domain by scaling | `cmin` and/or `cmax` to be equidistant to this point. | Value should have the same units as `value`. Has no | effect when `cauto` is `false`. | cmin | Sets the lower bound of the color domain. Value should | have the same units as `value` and if set, `cmax` must | be set as well. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.isosurface.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `cmin` and `cmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | contour | :class:`plotly.graph_objects.isosurface.Contour` | instance or dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | flatshading | Determines whether or not normal smoothing is applied | to the meshes, creating meshes with an angular, low- | poly look via flat reflections. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.isosurface.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | isomax | Sets the maximum boundary for iso-surface plot. | isomin | Sets the minimum boundary for iso-surface plot. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.isosurface.Legendgrouptitl | e` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | lighting | :class:`plotly.graph_objects.isosurface.Lighting` | instance or dict with compatible properties | lightposition | :class:`plotly.graph_objects.isosurface.Lightposition` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the surface. Please note that in | the case of using high `opacity` values for example a | value greater than or equal to 0.5 on two surfaces (and | 0.25 with four surfaces), an overlay of multiple | transparent surfaces may not perfectly be sorted in | depth by the webgl API. This behavior may be improved | in the near future and is subject to change. | reversescale | Reverses the color mapping if true. If true, `cmin` | will correspond to the last color in the array and | `cmax` will correspond to the first color. | scene | Sets a reference between this trace's 3D coordinate | system and a 3D scene. If "scene" (the default value), | the (x,y,z) coordinates refer to `layout.scene`. If | "scene2", the (x,y,z) coordinates refer to | `layout.scene2`, and so on. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | slices | :class:`plotly.graph_objects.isosurface.Slices` | instance or dict with compatible properties | spaceframe | :class:`plotly.graph_objects.isosurface.Spaceframe` | instance or dict with compatible properties | stream | :class:`plotly.graph_objects.isosurface.Stream` | instance or dict with compatible properties | surface | :class:`plotly.graph_objects.isosurface.Surface` | instance or dict with compatible properties | text | Sets the text elements associated with the vertices. If | trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | value | Sets the 4th dimension (value) of the vertices. | valuehoverformat | Sets the hover text formatting rulefor `value` using | d3 formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | valuesrc | Sets the source reference on Chart Studio Cloud for | `value`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the X coordinates of the vertices on X axis. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the Y coordinates of the vertices on Y axis. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the Z coordinates of the vertices on Z axis. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `zaxis.hoverformat`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_layout_image(self, arg=None, layer=None, name=None, opacity=None, sizex=None, sizey=None, sizing=None, source=None, templateitemname=None, visible=None, x=None, xanchor=None, xref=None, y=None, yanchor=None, yref=None, row=None, col=None, secondary_y=None, exclude_empty_subplots=None, **kwargs) -> 'FigureWidget' | Create and add a new image to the figure's layout | | Parameters | ---------- | arg | instance of Image or dict with compatible properties | layer | Specifies whether images are drawn below or above | traces. When `xref` and `yref` are both set to `paper`, | image is drawn below the entire plot area. | name | When used in a template, named items are created in the | output figure in addition to any items the figure | already has in this array. You can modify these items | in the output figure by making your own item with | `templateitemname` matching this `name` alongside your | modifications (including `visible: false` or `enabled: | false` to hide it). Has no effect outside of a | template. | opacity | Sets the opacity of the image. | sizex | Sets the image container size horizontally. The image | will be sized based on the `position` value. When | `xref` is set to `paper`, units are sized relative to | the plot width. When `xref` ends with ` domain`, units | are sized relative to the axis width. | sizey | Sets the image container size vertically. The image | will be sized based on the `position` value. When | `yref` is set to `paper`, units are sized relative to | the plot height. When `yref` ends with ` domain`, units | are sized relative to the axis height. | sizing | Specifies which dimension of the image to constrain. | source | Specifies the URL of the image to be used. The URL must | be accessible from the domain where the plot code is | run, and can be either relative or absolute. | templateitemname | Used to refer to a named item in this array in the | template. Named items from the template will be created | even without a matching item in the input figure, but | you can modify one by making an item with | `templateitemname` matching its `name`, alongside your | modifications (including `visible: false` or `enabled: | false` to hide it). If there is no template or no | matching item, this item will be hidden unless you | explicitly show it with `visible: true`. | visible | Determines whether or not this image is visible. | x | Sets the image's x position. When `xref` is set to | `paper`, units are sized relative to the plot height. | See `xref` for more info | xanchor | Sets the anchor for the x position | xref | Sets the images's x coordinate axis. If set to a x axis | id (e.g. "x" or "x2"), the `x` position refers to a x | coordinate. If set to "paper", the `x` position refers | to the distance from the left of the plotting area in | normalized coordinates where 0 (1) corresponds to the | left (right). If set to a x axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the left of the | domain of that axis: e.g., *x2 domain* refers to the | domain of the second x axis and a x position of 0.5 | refers to the point between the left and the right of | the domain of the second x axis. | y | Sets the image's y position. When `yref` is set to | `paper`, units are sized relative to the plot height. | See `yref` for more info | yanchor | Sets the anchor for the y position. | yref | Sets the images's y coordinate axis. If set to a y axis | id (e.g. "y" or "y2"), the `y` position refers to a y | coordinate. If set to "paper", the `y` position refers | to the distance from the bottom of the plotting area in | normalized coordinates where 0 (1) corresponds to the | bottom (top). If set to a y axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the bottom of the | domain of that axis: e.g., *y2 domain* refers to the | domain of the second y axis and a y position of 0.5 | refers to the point between the bottom and the top of | the domain of the second y axis. | row | Subplot row for image. If 'all', addresses all rows in | the specified column(s). | col | Subplot column for image. If 'all', addresses all | columns in the specified row(s). | secondary_y | Whether to add image to secondary y-axis | exclude_empty_subplots | If True, image will not be added to subplots without | traces. | | Returns | ------- | FigureWidget | | add_mesh3d(self, alphahull=None, autocolorscale=None, cauto=None, cmax=None, cmid=None, cmin=None, color=None, coloraxis=None, colorbar=None, colorscale=None, contour=None, customdata=None, customdatasrc=None, delaunayaxis=None, facecolor=None, facecolorsrc=None, flatshading=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, i=None, ids=None, idssrc=None, intensity=None, intensitymode=None, intensitysrc=None, isrc=None, j=None, jsrc=None, k=None, ksrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, lighting=None, lightposition=None, meta=None, metasrc=None, name=None, opacity=None, reversescale=None, scene=None, showlegend=None, showscale=None, stream=None, text=None, textsrc=None, uid=None, uirevision=None, vertexcolor=None, vertexcolorsrc=None, visible=None, x=None, xcalendar=None, xhoverformat=None, xsrc=None, y=None, ycalendar=None, yhoverformat=None, ysrc=None, z=None, zcalendar=None, zhoverformat=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Mesh3d trace | | Draws sets of triangles with coordinates given by three | 1-dimensional arrays in `x`, `y`, `z` and (1) a sets of `i`, | `j`, `k` indices (2) Delaunay triangulation or (3) the Alpha- | shape algorithm or (4) the Convex-hull algorithm | | Parameters | ---------- | alphahull | Determines how the mesh surface triangles are derived | from the set of vertices (points) represented by the | `x`, `y` and `z` arrays, if the `i`, `j`, `k` arrays | are not supplied. For general use of `mesh3d` it is | preferred that `i`, `j`, `k` are supplied. If "-1", | Delaunay triangulation is used, which is mainly | suitable if the mesh is a single, more or less layer | surface that is perpendicular to `delaunayaxis`. In | case the `delaunayaxis` intersects the mesh surface at | more than one point it will result triangles that are | very long in the dimension of `delaunayaxis`. If ">0", | the alpha-shape algorithm is used. In this case, the | positive `alphahull` value signals the use of the | alpha-shape algorithm, _and_ its value acts as the | parameter for the mesh fitting. If 0, the convex-hull | algorithm is used. It is suitable for convex bodies or | if the intention is to enclose the `x`, `y` and `z` | point set into a convex hull. | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | cauto | Determines whether or not the color domain is computed | with respect to the input data (here `intensity`) or | the bounds set in `cmin` and `cmax` Defaults to `false` | when `cmin` and `cmax` are set by the user. | cmax | Sets the upper bound of the color domain. Value should | have the same units as `intensity` and if set, `cmin` | must be set as well. | cmid | Sets the mid-point of the color domain by scaling | `cmin` and/or `cmax` to be equidistant to this point. | Value should have the same units as `intensity`. Has no | effect when `cauto` is `false`. | cmin | Sets the lower bound of the color domain. Value should | have the same units as `intensity` and if set, `cmax` | must be set as well. | color | Sets the color of the whole mesh | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.mesh3d.ColorBar` instance | or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `cmin` and `cmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | contour | :class:`plotly.graph_objects.mesh3d.Contour` instance | or dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | delaunayaxis | Sets the Delaunay axis, which is the axis that is | perpendicular to the surface of the Delaunay | triangulation. It has an effect if `i`, `j`, `k` are | not provided and `alphahull` is set to indicate | Delaunay triangulation. | facecolor | Sets the color of each face Overrides "color" and | "vertexcolor". | facecolorsrc | Sets the source reference on Chart Studio Cloud for | `facecolor`. | flatshading | Determines whether or not normal smoothing is applied | to the meshes, creating meshes with an angular, low- | poly look via flat reflections. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.mesh3d.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | i | A vector of vertex indices, i.e. integer values between | 0 and the length of the vertex vectors, representing | the "first" vertex of a triangle. For example, `{i[m], | j[m], k[m]}` together represent face m (triangle m) in | the mesh, where `i[m] = n` points to the triplet | `{x[n], y[n], z[n]}` in the vertex arrays. Therefore, | each element in `i` represents a point in space, which | is the first vertex of a triangle. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | intensity | Sets the intensity values for vertices or cells as | defined by `intensitymode`. It can be used for plotting | fields on meshes. | intensitymode | Determines the source of `intensity` values. | intensitysrc | Sets the source reference on Chart Studio Cloud for | `intensity`. | isrc | Sets the source reference on Chart Studio Cloud for | `i`. | j | A vector of vertex indices, i.e. integer values between | 0 and the length of the vertex vectors, representing | the "second" vertex of a triangle. For example, `{i[m], | j[m], k[m]}` together represent face m (triangle m) in | the mesh, where `j[m] = n` points to the triplet | `{x[n], y[n], z[n]}` in the vertex arrays. Therefore, | each element in `j` represents a point in space, which | is the second vertex of a triangle. | jsrc | Sets the source reference on Chart Studio Cloud for | `j`. | k | A vector of vertex indices, i.e. integer values between | 0 and the length of the vertex vectors, representing | the "third" vertex of a triangle. For example, `{i[m], | j[m], k[m]}` together represent face m (triangle m) in | the mesh, where `k[m] = n` points to the triplet | `{x[n], y[n], z[n]}` in the vertex arrays. Therefore, | each element in `k` represents a point in space, which | is the third vertex of a triangle. | ksrc | Sets the source reference on Chart Studio Cloud for | `k`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.mesh3d.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | lighting | :class:`plotly.graph_objects.mesh3d.Lighting` instance | or dict with compatible properties | lightposition | :class:`plotly.graph_objects.mesh3d.Lightposition` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the surface. Please note that in | the case of using high `opacity` values for example a | value greater than or equal to 0.5 on two surfaces (and | 0.25 with four surfaces), an overlay of multiple | transparent surfaces may not perfectly be sorted in | depth by the webgl API. This behavior may be improved | in the near future and is subject to change. | reversescale | Reverses the color mapping if true. If true, `cmin` | will correspond to the last color in the array and | `cmax` will correspond to the first color. | scene | Sets a reference between this trace's 3D coordinate | system and a 3D scene. If "scene" (the default value), | the (x,y,z) coordinates refer to `layout.scene`. If | "scene2", the (x,y,z) coordinates refer to | `layout.scene2`, and so on. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.mesh3d.Stream` instance or | dict with compatible properties | text | Sets the text elements associated with the vertices. If | trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | vertexcolor | Sets the color of each vertex Overrides "color". While | Red, green and blue colors are in the range of 0 and | 255; in the case of having vertex color data in RGBA | format, the alpha color should be normalized to be | between 0 and 1. | vertexcolorsrc | Sets the source reference on Chart Studio Cloud for | `vertexcolor`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the X coordinates of the vertices. The nth element | of vectors `x`, `y` and `z` jointly represent the X, Y | and Z coordinates of the nth vertex. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the Y coordinates of the vertices. The nth element | of vectors `x`, `y` and `z` jointly represent the X, Y | and Z coordinates of the nth vertex. | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the Z coordinates of the vertices. The nth element | of vectors `x`, `y` and `z` jointly represent the X, Y | and Z coordinates of the nth vertex. | zcalendar | Sets the calendar system to use with `z` date data. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `zaxis.hoverformat`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_ohlc(self, close=None, closesrc=None, customdata=None, customdatasrc=None, decreasing=None, high=None, highsrc=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, increasing=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, low=None, lowsrc=None, meta=None, metasrc=None, name=None, opacity=None, open=None, opensrc=None, selectedpoints=None, showlegend=None, stream=None, text=None, textsrc=None, tickwidth=None, uid=None, uirevision=None, visible=None, x=None, xaxis=None, xcalendar=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, yaxis=None, yhoverformat=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Ohlc trace | | The ohlc (short for Open-High-Low-Close) is a style of | financial chart describing open, high, low and close for a | given `x` coordinate (most likely time). The tip of the lines | represent the `low` and `high` values and the horizontal | segments represent the `open` and `close` values. Sample points | where the close value is higher (lower) then the open value are | called increasing (decreasing). By default, increasing items | are drawn in green whereas decreasing are drawn in red. | | Parameters | ---------- | close | Sets the close values. | closesrc | Sets the source reference on Chart Studio Cloud for | `close`. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | decreasing | :class:`plotly.graph_objects.ohlc.Decreasing` instance | or dict with compatible properties | high | Sets the high values. | highsrc | Sets the source reference on Chart Studio Cloud for | `high`. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.ohlc.Hoverlabel` instance | or dict with compatible properties | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | increasing | :class:`plotly.graph_objects.ohlc.Increasing` instance | or dict with compatible properties | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.ohlc.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.ohlc.Line` instance or | dict with compatible properties | low | Sets the low values. | lowsrc | Sets the source reference on Chart Studio Cloud for | `low`. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | open | Sets the open values. | opensrc | Sets the source reference on Chart Studio Cloud for | `open`. | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.ohlc.Stream` instance or | dict with compatible properties | text | Sets hover text elements associated with each sample | point. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to this trace's sample points. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | tickwidth | Sets the width of the open/close tick marks relative to | the "x" minimal interval. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. If absent, linear coordinate | will be generated. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_parcats(self, arrangement=None, bundlecolors=None, counts=None, countssrc=None, dimensions=None, dimensiondefaults=None, domain=None, hoverinfo=None, hoveron=None, hovertemplate=None, labelfont=None, legendgrouptitle=None, legendwidth=None, line=None, meta=None, metasrc=None, name=None, sortpaths=None, stream=None, tickfont=None, uid=None, uirevision=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Parcats trace | | Parallel categories diagram for multidimensional categorical | data. | | Parameters | ---------- | arrangement | Sets the drag interaction mode for categories and | dimensions. If `perpendicular`, the categories can only | move along a line perpendicular to the paths. If | `freeform`, the categories can freely move on the | plane. If `fixed`, the categories and dimensions are | stationary. | bundlecolors | Sort paths so that like colors are bundled together | within each category. | counts | The number of observations represented by each state. | Defaults to 1 so that each state represents one | observation | countssrc | Sets the source reference on Chart Studio Cloud for | `counts`. | dimensions | The dimensions (variables) of the parallel categories | diagram. | dimensiondefaults | When used in a template (as | layout.template.data.parcats.dimensiondefaults), sets | the default property values to use for elements of | parcats.dimensions | domain | :class:`plotly.graph_objects.parcats.Domain` instance | or dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoveron | Sets the hover interaction mode for the parcats | diagram. If `category`, hover interaction take place | per category. If `color`, hover interactions take place | per color per category. If `dimension`, hover | interactions take place across all categories per | dimension. | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. This value here applies when hovering | over dimensions. Note that `*categorycount`, | "colorcount" and "bandcolorcount" are only available | when `hoveron` contains the "color" flagFinally, the | template string has access to variables `count`, | `probability`, `category`, `categorycount`, | `colorcount` and `bandcolorcount`. Anything contained | in tag `<extra>` is displayed in the secondary box, for | example "<extra>{fullData.name}</extra>". To hide the | secondary box completely, use an empty tag | `<extra></extra>`. | labelfont | Sets the font for the `dimension` labels. | legendgrouptitle | :class:`plotly.graph_objects.parcats.Legendgrouptitle` | instance or dict with compatible properties | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.parcats.Line` instance or | dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | sortpaths | Sets the path sorting algorithm. If `forward`, sort | paths based on dimension categories from left to right. | If `backward`, sort paths based on dimensions | categories from right to left. | stream | :class:`plotly.graph_objects.parcats.Stream` instance | or dict with compatible properties | tickfont | Sets the font for the `category` labels. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_parcoords(self, customdata=None, customdatasrc=None, dimensions=None, dimensiondefaults=None, domain=None, ids=None, idssrc=None, labelangle=None, labelfont=None, labelside=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, meta=None, metasrc=None, name=None, rangefont=None, stream=None, tickfont=None, uid=None, uirevision=None, unselected=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Parcoords trace | | Parallel coordinates for multidimensional exploratory data | analysis. The samples are specified in `dimensions`. The colors | are set in `line.color`. | | Parameters | ---------- | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dimensions | The dimensions (variables) of the parallel coordinates | chart. 2..60 dimensions are supported. | dimensiondefaults | When used in a template (as | layout.template.data.parcoords.dimensiondefaults), sets | the default property values to use for elements of | parcoords.dimensions | domain | :class:`plotly.graph_objects.parcoords.Domain` instance | or dict with compatible properties | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | labelangle | Sets the angle of the labels with respect to the | horizontal. For example, a `tickangle` of -90 draws the | labels vertically. Tilted labels with "labelangle" may | be positioned better inside margins when | `labelposition` is set to "bottom". | labelfont | Sets the font for the `dimension` labels. | labelside | Specifies the location of the `label`. "top" positions | labels above, next to the title "bottom" positions | labels below the graph Tilted labels with "labelangle" | may be positioned better inside margins when | `labelposition` is set to "bottom". | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.parcoords.Legendgrouptitle | ` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.parcoords.Line` instance | or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | rangefont | Sets the font for the `dimension` range values. | stream | :class:`plotly.graph_objects.parcoords.Stream` instance | or dict with compatible properties | tickfont | Sets the font for the `dimension` tick values. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.parcoords.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_pie(self, automargin=None, customdata=None, customdatasrc=None, direction=None, dlabel=None, domain=None, hole=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, insidetextfont=None, insidetextorientation=None, label0=None, labels=None, labelssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, opacity=None, outsidetextfont=None, pull=None, pullsrc=None, rotation=None, scalegroup=None, showlegend=None, sort=None, stream=None, text=None, textfont=None, textinfo=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, title=None, titlefont=None, titleposition=None, uid=None, uirevision=None, values=None, valuessrc=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Pie trace | | A data visualized by the sectors of the pie is set in `values`. | The sector labels are set in `labels`. The sector colors are | set in `marker.colors` | | Parameters | ---------- | automargin | Determines whether outside text labels can push the | margins. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | direction | Specifies the direction at which succeeding sectors | follow one another. | dlabel | Sets the label step. See `label0` for more info. | domain | :class:`plotly.graph_objects.pie.Domain` instance or | dict with compatible properties | hole | Sets the fraction of the radius to cut out of the pie. | Use this to make a donut chart. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.pie.Hoverlabel` instance | or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `label`, `color`, `value`, `percent` and | `text`. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each sector. | If a single string, the same string appears for all | data points. If an array of string, the items are | mapped in order of this trace's sectors. To be seen, | trace `hoverinfo` must contain a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | insidetextfont | Sets the font used for `textinfo` lying inside the | sector. | insidetextorientation | Controls the orientation of the text inside chart | sectors. When set to "auto", text may be oriented in | any direction in order to be as big as possible in the | middle of a sector. The "horizontal" option orients | text to be parallel with the bottom of the chart, and | may make text smaller in order to achieve that goal. | The "radial" option orients text along the radius of | the sector. The "tangential" option orients text | perpendicular to the radius of the sector. | label0 | Alternate to `labels`. Builds a numeric set of labels. | Use with `dlabel` where `label0` is the starting label | and `dlabel` the step. | labels | Sets the sector labels. If `labels` entries are | duplicated, we sum associated `values` or simply count | occurrences if `values` is not provided. For other | array attributes (including color) we use the first | non-empty entry among all occurrences of the label. | labelssrc | Sets the source reference on Chart Studio Cloud for | `labels`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.pie.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.pie.Marker` instance or | dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | outsidetextfont | Sets the font used for `textinfo` lying outside the | sector. | pull | Sets the fraction of larger radius to pull the sectors | out from the center. This can be a constant to pull all | slices apart from each other equally or an array to | highlight one or more slices. | pullsrc | Sets the source reference on Chart Studio Cloud for | `pull`. | rotation | Instead of the first slice starting at 12 o'clock, | rotate to some other angle. | scalegroup | If there are multiple pie charts that should be sized | according to their totals, link them by providing a | non-empty group id here shared by every trace in the | same group. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | sort | Determines whether or not the sectors are reordered | from largest to smallest. | stream | :class:`plotly.graph_objects.pie.Stream` instance or | dict with compatible properties | text | Sets text elements associated with each sector. If | trace `textinfo` contains a "text" flag, these elements | will be seen on the chart. If trace `hoverinfo` | contains a "text" flag and "hovertext" is not set, | these elements will be seen in the hover labels. | textfont | Sets the font used for `textinfo`. | textinfo | Determines which trace information appear on the graph. | textposition | Specifies the location of the `textinfo`. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `label`, `color`, `value`, `percent` and | `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | title | :class:`plotly.graph_objects.pie.Title` instance or | dict with compatible properties | titlefont | Deprecated: Please use pie.title.font instead. Sets the | font used for `title`. Note that the title's font used | to be set by the now deprecated `titlefont` attribute. | titleposition | Deprecated: Please use pie.title.position instead. | Specifies the location of the `title`. Note that the | title's position used to be set by the now deprecated | `titleposition` attribute. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | values | Sets the values of the sectors. If omitted, we count | occurrences of each label. | valuessrc | Sets the source reference on Chart Studio Cloud for | `values`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_pointcloud(self, customdata=None, customdatasrc=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, ids=None, idssrc=None, indices=None, indicessrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, opacity=None, showlegend=None, stream=None, text=None, textsrc=None, uid=None, uirevision=None, visible=None, x=None, xaxis=None, xbounds=None, xboundssrc=None, xsrc=None, xy=None, xysrc=None, y=None, yaxis=None, ybounds=None, yboundssrc=None, ysrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Pointcloud trace | | "pointcloud" trace is deprecated! Please consider switching to | the "scattergl" trace type. The data visualized as a point | cloud set in `x` and `y` using the WebGl plotting engine. | | Parameters | ---------- | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.pointcloud.Hoverlabel` | instance or dict with compatible properties | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | indices | A sequential value, 0..n, supply it to avoid creating | this array inside plotting. If specified, it must be a | typed `Int32Array` array. Its length must be equal to | or greater than the number of points. For the best | performance and memory use, create one large `indices` | typed array that is guaranteed to be at least as long | as the largest number of points during use, and reuse | it on each `Plotly.restyle()` call. | indicessrc | Sets the source reference on Chart Studio Cloud for | `indices`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.pointcloud.Legendgrouptitl | e` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.pointcloud.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.pointcloud.Stream` | instance or dict with compatible properties | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xbounds | Specify `xbounds` in the shape of `[xMin, xMax] to | avoid looping through the `xy` typed array. Use it in | conjunction with `xy` and `ybounds` for the performance | benefits. | xboundssrc | Sets the source reference on Chart Studio Cloud for | `xbounds`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | xy | Faster alternative to specifying `x` and `y` | separately. If supplied, it must be a typed | `Float32Array` array that represents points such that | `xy[i * 2] = x[i]` and `xy[i * 2 + 1] = y[i]` | xysrc | Sets the source reference on Chart Studio Cloud for | `xy`. | y | Sets the y coordinates. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ybounds | Specify `ybounds` in the shape of `[yMin, yMax] to | avoid looping through the `xy` typed array. Use it in | conjunction with `xy` and `xbounds` for the performance | benefits. | yboundssrc | Sets the source reference on Chart Studio Cloud for | `ybounds`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_sankey(self, arrangement=None, customdata=None, customdatasrc=None, domain=None, hoverinfo=None, hoverlabel=None, ids=None, idssrc=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, link=None, meta=None, metasrc=None, name=None, node=None, orientation=None, selectedpoints=None, stream=None, textfont=None, uid=None, uirevision=None, valueformat=None, valuesuffix=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Sankey trace | | Sankey plots for network flow data analysis. The nodes are | specified in `nodes` and the links between sources and targets | in `links`. The colors are set in `nodes[i].color` and | `links[i].color`, otherwise defaults are used. | | Parameters | ---------- | arrangement | If value is `snap` (the default), the node arrangement | is assisted by automatic snapping of elements to | preserve space between nodes specified via `nodepad`. | If value is `perpendicular`, the nodes can only move | along a line perpendicular to the flow. If value is | `freeform`, the nodes can freely move on the plane. If | value is `fixed`, the nodes are stationary. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | domain | :class:`plotly.graph_objects.sankey.Domain` instance or | dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. Note that this attribute is | superseded by `node.hoverinfo` and `node.hoverinfo` for | nodes and links respectively. | hoverlabel | :class:`plotly.graph_objects.sankey.Hoverlabel` | instance or dict with compatible properties | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.sankey.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | link | The links of the Sankey plot. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | node | The nodes of the Sankey plot. | orientation | Sets the orientation of the Sankey diagram. | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | stream | :class:`plotly.graph_objects.sankey.Stream` instance or | dict with compatible properties | textfont | Sets the font for node labels | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | valueformat | Sets the value formatting rule using d3 formatting | mini-languages which are very similar to those in | Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | valuesuffix | Adds a unit to follow the value in the hover tooltip. | Add a space if a separation is necessary from the | value. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_scatter(self, alignmentgroup=None, cliponaxis=None, connectgaps=None, customdata=None, customdatasrc=None, dx=None, dy=None, error_x=None, error_y=None, fill=None, fillcolor=None, fillgradient=None, fillpattern=None, groupnorm=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoveron=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, mode=None, name=None, offsetgroup=None, opacity=None, orientation=None, selected=None, selectedpoints=None, showlegend=None, stackgaps=None, stackgroup=None, stream=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, x=None, x0=None, xaxis=None, xcalendar=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, y=None, y0=None, yaxis=None, ycalendar=None, yhoverformat=None, yperiod=None, yperiod0=None, yperiodalignment=None, ysrc=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Scatter trace | | The scatter trace type encompasses line charts, scatter charts, | text charts, and bubble charts. The data visualized as scatter | point or lines is set in `x` and `y`. Text (appearing either on | the chart or on hover only) is via `text`. Bubble charts are | achieved by setting `marker.size` and/or `marker.color` to | numerical arrays. | | Parameters | ---------- | alignmentgroup | Set several traces linked to the same position axis or | matching axes to the same alignmentgroup. This controls | whether bars compute their positional range dependently | or independently. | cliponaxis | Determines whether or not markers and text nodes are | clipped about the subplot axes. To show markers and | text nodes above axis lines and tick labels, make sure | to set `xaxis.layer` and `yaxis.layer` to *below | traces*. | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Sets the x coordinate step. See `x0` for more info. | dy | Sets the y coordinate step. See `y0` for more info. | error_x | :class:`plotly.graph_objects.scatter.ErrorX` instance | or dict with compatible properties | error_y | :class:`plotly.graph_objects.scatter.ErrorY` instance | or dict with compatible properties | fill | Sets the area to fill with a solid color. Defaults to | "none" unless this trace is stacked, then it gets | "tonexty" ("tonextx") if `orientation` is "v" ("h") Use | with `fillcolor` if not "none". "tozerox" and "tozeroy" | fill to x=0 and y=0 respectively. "tonextx" and | "tonexty" fill between the endpoints of this trace and | the endpoints of the trace before it, connecting those | endpoints with straight lines (to make a stacked area | graph); if there is no trace before it, they behave | like "tozerox" and "tozeroy". "toself" connects the | endpoints of the trace (or each segment of the trace if | it has gaps) into a closed shape. "tonext" fills the | space between two traces if one completely encloses the | other (eg consecutive contour lines), and behaves like | "toself" if there is no trace before it. "tonext" | should not be used if one trace does not enclose the | other. Traces in a `stackgroup` will only fill to (or | be filled to) other traces in the same group. With | multiple `stackgroup`s or some traces stacked and some | not, if fill-linked traces are not already consecutive, | the later ones will be pushed down in the drawing | order. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. If fillgradient is | specified, fillcolor is ignored except for setting the | background color of the hover label, if any. | fillgradient | Sets a fill gradient. If not specified, the fillcolor | is used instead. | fillpattern | Sets the pattern within the marker. | groupnorm | Only relevant when `stackgroup` is used, and only the | first `groupnorm` found in the `stackgroup` will be | used - including if `visible` is "legendonly" but not | if it is `false`. Sets the normalization for the sum of | this `stackgroup`. With "fraction", the value of each | trace at each location is divided by the sum of all | trace values at that location. "percent" is the same | but multiplied by 100 to show percentages. If there are | multiple subplots, or multiple `stackgroup`s on one | subplot, each will be normalized within its own set. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scatter.Hoverlabel` | instance or dict with compatible properties | hoveron | Do the hover effects highlight individual points | (markers or line points) or do they highlight filled | regions? If the fill is "toself" or "tonext" and there | are no markers or text, then the default is "fills", | otherwise it is "points". | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (x,y) | pair. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scatter.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scatter.Line` instance or | dict with compatible properties | marker | :class:`plotly.graph_objects.scatter.Marker` instance | or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. If there are less than | 20 points and the trace is not stacked then the default | is "lines+markers". Otherwise, "lines". | name | Sets the trace name. The trace name appears as the | legend item and on hover. | offsetgroup | Set several traces linked to the same position axis or | matching axes to the same offsetgroup where bars of the | same position coordinate will line up. | opacity | Sets the opacity of the trace. | orientation | Only relevant in the following cases: 1. when | `scattermode` is set to "group". 2. when `stackgroup` | is used, and only the first `orientation` found in the | `stackgroup` will be used - including if `visible` is | "legendonly" but not if it is `false`. Sets the | stacking direction. With "v" ("h"), the y (x) values of | subsequent traces are added. Also affects the default | value of `fill`. | selected | :class:`plotly.graph_objects.scatter.Selected` instance | or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stackgaps | Only relevant when `stackgroup` is used, and only the | first `stackgaps` found in the `stackgroup` will be | used - including if `visible` is "legendonly" but not | if it is `false`. Determines how we handle locations at | which other traces in this group have data but this one | does not. With *infer zero* we insert a zero at these | locations. With "interpolate" we linearly interpolate | between existing values, and extrapolate a constant | beyond the existing values. | stackgroup | Set several scatter traces (on the same subplot) to the | same stackgroup in order to add their y values (or | their x values if `orientation` is "h"). If blank or | omitted this trace will not be stacked. Stacking also | turns `fill` on by default, using "tonexty" ("tonextx") | if `orientation` is "h" ("v") and sets the default | `mode` to "lines" irrespective of point count. You can | only stack on a numeric (linear or log) axis. Traces in | a `stackgroup` will only fill to (or be filled to) | other traces in the same group. With multiple | `stackgroup`s or some traces stacked and some not, if | fill-linked traces are not already consecutive, the | later ones will be pushed down in the drawing order. | stream | :class:`plotly.graph_objects.scatter.Stream` instance | or dict with compatible properties | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scatter.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. | x0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates. | y0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | yperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the y | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | yperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the y0 axis. When `y0period` is round number | of weeks, the `y0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | yperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the y axis. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_scatter3d(self, connectgaps=None, customdata=None, customdatasrc=None, error_x=None, error_y=None, error_z=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, projection=None, scene=None, showlegend=None, stream=None, surfaceaxis=None, surfacecolor=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, visible=None, x=None, xcalendar=None, xhoverformat=None, xsrc=None, y=None, ycalendar=None, yhoverformat=None, ysrc=None, z=None, zcalendar=None, zhoverformat=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Scatter3d trace | | The data visualized as scatter point or lines in 3D dimension | is set in `x`, `y`, `z`. Text (appearing either on the chart or | on hover only) is via `text`. Bubble charts are achieved by | setting `marker.size` and/or `marker.color` Projections are | achieved via `projection`. Surface fills are achieved via | `surfaceaxis`. | | Parameters | ---------- | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | error_x | :class:`plotly.graph_objects.scatter3d.ErrorX` instance | or dict with compatible properties | error_y | :class:`plotly.graph_objects.scatter3d.ErrorY` instance | or dict with compatible properties | error_z | :class:`plotly.graph_objects.scatter3d.ErrorZ` instance | or dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scatter3d.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets text elements associated with each (x,y,z) | triplet. If a single string, the same string appears | over all the data points. If an array of string, the | items are mapped in order to the this trace's (x,y,z) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scatter3d.Legendgrouptitle | ` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scatter3d.Line` instance | or dict with compatible properties | marker | :class:`plotly.graph_objects.scatter3d.Marker` instance | or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. If there are less than | 20 points and the trace is not stacked then the default | is "lines+markers". Otherwise, "lines". | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | projection | :class:`plotly.graph_objects.scatter3d.Projection` | instance or dict with compatible properties | scene | Sets a reference between this trace's 3D coordinate | system and a 3D scene. If "scene" (the default value), | the (x,y,z) coordinates refer to `layout.scene`. If | "scene2", the (x,y,z) coordinates refer to | `layout.scene2`, and so on. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scatter3d.Stream` instance | or dict with compatible properties | surfaceaxis | If "-1", the scatter points are not fill with a surface | If 0, 1, 2, the scatter points are filled with a | Delaunay surface about the x, y, z respectively. | surfacecolor | Sets the surface fill color. | text | Sets text elements associated with each (x,y,z) | triplet. If a single string, the same string appears | over all the data points. If an array of string, the | items are mapped in order to the this trace's (x,y,z) | coordinates. If trace `hoverinfo` contains a "text" | flag and "hovertext" is not set, these elements will be | seen in the hover labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates. | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the z coordinates. | zcalendar | Sets the calendar system to use with `z` date data. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `zaxis.hoverformat`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_scattercarpet(self, a=None, asrc=None, b=None, bsrc=None, carpet=None, connectgaps=None, customdata=None, customdatasrc=None, fill=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoveron=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, selected=None, selectedpoints=None, showlegend=None, stream=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, xaxis=None, yaxis=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Scattercarpet trace | | Plots a scatter trace on either the first carpet axis or the | carpet axis with a matching `carpet` attribute. | | Parameters | ---------- | a | Sets the a-axis coordinates. | asrc | Sets the source reference on Chart Studio Cloud for | `a`. | b | Sets the b-axis coordinates. | bsrc | Sets the source reference on Chart Studio Cloud for | `b`. | carpet | An identifier for this carpet, so that `scattercarpet` | and `contourcarpet` traces can specify a carpet plot on | which they lie | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | fill | Sets the area to fill with a solid color. Use with | `fillcolor` if not "none". scatterternary has a subset | of the options available to scatter. "toself" connects | the endpoints of the trace (or each segment of the | trace if it has gaps) into a closed shape. "tonext" | fills the space between two traces if one completely | encloses the other (eg consecutive contour lines), and | behaves like "toself" if there is no trace before it. | "tonext" should not be used if one trace does not | enclose the other. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scattercarpet.Hoverlabel` | instance or dict with compatible properties | hoveron | Do the hover effects highlight individual points | (markers or line points) or do they highlight filled | regions? If the fill is "toself" or "tonext" and there | are no markers or text, then the default is "fills", | otherwise it is "points". | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (a,b) | point. If a single string, the same string appears over | all the data points. If an array of strings, the items | are mapped in order to the the data points in (a,b). To | be seen, trace `hoverinfo` must contain a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scattercarpet.Legendgroupt | itle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scattercarpet.Line` | instance or dict with compatible properties | marker | :class:`plotly.graph_objects.scattercarpet.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. If there are less than | 20 points and the trace is not stacked then the default | is "lines+markers". Otherwise, "lines". | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | selected | :class:`plotly.graph_objects.scattercarpet.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scattercarpet.Stream` | instance or dict with compatible properties | text | Sets text elements associated with each (a,b) point. If | a single string, the same string appears over all the | data points. If an array of strings, the items are | mapped in order to the the data points in (a,b). If | trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `a`, `b` and `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scattercarpet.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_scattergeo(self, connectgaps=None, customdata=None, customdatasrc=None, featureidkey=None, fill=None, fillcolor=None, geo=None, geojson=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, lat=None, latsrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, locationmode=None, locations=None, locationssrc=None, lon=None, lonsrc=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, selected=None, selectedpoints=None, showlegend=None, stream=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Scattergeo trace | | The data visualized as scatter point or lines on a geographic | map is provided either by longitude/latitude pairs in `lon` and | `lat` respectively or by geographic location IDs or names in | `locations`. | | Parameters | ---------- | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | featureidkey | Sets the key in GeoJSON features which is used as id to | match the items included in the `locations` array. Only | has an effect when `geojson` is set. Support nested | property, for example "properties.name". | fill | Sets the area to fill with a solid color. Use with | `fillcolor` if not "none". "toself" connects the | endpoints of the trace (or each segment of the trace if | it has gaps) into a closed shape. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | geo | Sets a reference between this trace's geospatial | coordinates and a geographic map. If "geo" (the default | value), the geospatial coordinates refer to | `layout.geo`. If "geo2", the geospatial coordinates | refer to `layout.geo2`, and so on. | geojson | Sets optional GeoJSON data associated with this trace. | If not given, the features on the base map are used | when `locations` is set. It can be set as a valid | GeoJSON object or as a URL string. Note that we only | accept GeoJSONs of type "FeatureCollection" or | "Feature" with geometries of type "Polygon" or | "MultiPolygon". | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scattergeo.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (lon,lat) | pair or item in `locations`. If a single string, the | same string appears over all the data points. If an | array of string, the items are mapped in order to the | this trace's (lon,lat) or `locations` coordinates. To | be seen, trace `hoverinfo` must contain a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | lat | Sets the latitude coordinates (in degrees North). | latsrc | Sets the source reference on Chart Studio Cloud for | `lat`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scattergeo.Legendgrouptitl | e` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scattergeo.Line` instance | or dict with compatible properties | locationmode | Determines the set of locations used to match entries | in `locations` to regions on the map. Values "ISO-3", | "USA-states", *country names* correspond to features on | the base map and value "geojson-id" corresponds to | features from a custom GeoJSON linked to the `geojson` | attribute. | locations | Sets the coordinates via location IDs or names. | Coordinates correspond to the centroid of each location | given. See `locationmode` for more info. | locationssrc | Sets the source reference on Chart Studio Cloud for | `locations`. | lon | Sets the longitude coordinates (in degrees East). | lonsrc | Sets the source reference on Chart Studio Cloud for | `lon`. | marker | :class:`plotly.graph_objects.scattergeo.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. If there are less than | 20 points and the trace is not stacked then the default | is "lines+markers". Otherwise, "lines". | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | selected | :class:`plotly.graph_objects.scattergeo.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scattergeo.Stream` | instance or dict with compatible properties | text | Sets text elements associated with each (lon,lat) pair | or item in `locations`. If a single string, the same | string appears over all the data points. If an array of | string, the items are mapped in order to the this | trace's (lon,lat) or `locations` coordinates. If trace | `hoverinfo` contains a "text" flag and "hovertext" is | not set, these elements will be seen in the hover | labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `lat`, `lon`, `location` and `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scattergeo.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_scattergl(self, connectgaps=None, customdata=None, customdatasrc=None, dx=None, dy=None, error_x=None, error_y=None, fill=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, selected=None, selectedpoints=None, showlegend=None, stream=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, x=None, x0=None, xaxis=None, xcalendar=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, y=None, y0=None, yaxis=None, ycalendar=None, yhoverformat=None, yperiod=None, yperiod0=None, yperiodalignment=None, ysrc=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Scattergl trace | | The data visualized as scatter point or lines is set in `x` and | `y` using the WebGL plotting engine. Bubble charts are achieved | by setting `marker.size` and/or `marker.color` to a numerical | arrays. | | Parameters | ---------- | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dx | Sets the x coordinate step. See `x0` for more info. | dy | Sets the y coordinate step. See `y0` for more info. | error_x | :class:`plotly.graph_objects.scattergl.ErrorX` instance | or dict with compatible properties | error_y | :class:`plotly.graph_objects.scattergl.ErrorY` instance | or dict with compatible properties | fill | Sets the area to fill with a solid color. Defaults to | "none" unless this trace is stacked, then it gets | "tonexty" ("tonextx") if `orientation` is "v" ("h") Use | with `fillcolor` if not "none". "tozerox" and "tozeroy" | fill to x=0 and y=0 respectively. "tonextx" and | "tonexty" fill between the endpoints of this trace and | the endpoints of the trace before it, connecting those | endpoints with straight lines (to make a stacked area | graph); if there is no trace before it, they behave | like "tozerox" and "tozeroy". "toself" connects the | endpoints of the trace (or each segment of the trace if | it has gaps) into a closed shape. "tonext" fills the | space between two traces if one completely encloses the | other (eg consecutive contour lines), and behaves like | "toself" if there is no trace before it. "tonext" | should not be used if one trace does not enclose the | other. Traces in a `stackgroup` will only fill to (or | be filled to) other traces in the same group. With | multiple `stackgroup`s or some traces stacked and some | not, if fill-linked traces are not already consecutive, | the later ones will be pushed down in the drawing | order. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scattergl.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (x,y) | pair. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scattergl.Legendgrouptitle | ` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scattergl.Line` instance | or dict with compatible properties | marker | :class:`plotly.graph_objects.scattergl.Marker` instance | or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | selected | :class:`plotly.graph_objects.scattergl.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scattergl.Stream` instance | or dict with compatible properties | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scattergl.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. | x0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates. | y0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | yperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the y | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | yperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the y0 axis. When `y0period` is round number | of weeks, the `y0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | yperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the y axis. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_scattermap(self, below=None, cluster=None, connectgaps=None, customdata=None, customdatasrc=None, fill=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, lat=None, latsrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, lon=None, lonsrc=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, selected=None, selectedpoints=None, showlegend=None, stream=None, subplot=None, text=None, textfont=None, textposition=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Scattermap trace | | The data visualized as scatter point, lines or marker symbols | on a MapLibre GL geographic map is provided by | longitude/latitude pairs in `lon` and `lat`. | | Parameters | ---------- | below | Determines if this scattermap trace's layers are to be | inserted before the layer with the specified ID. By | default, scattermap layers are inserted above all the | base layers. To place the scattermap layers above every | other layer, set `below` to "''". | cluster | :class:`plotly.graph_objects.scattermap.Cluster` | instance or dict with compatible properties | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | fill | Sets the area to fill with a solid color. Use with | `fillcolor` if not "none". "toself" connects the | endpoints of the trace (or each segment of the trace if | it has gaps) into a closed shape. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scattermap.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (lon,lat) | pair If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (lon,lat) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | lat | Sets the latitude coordinates (in degrees North). | latsrc | Sets the source reference on Chart Studio Cloud for | `lat`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scattermap.Legendgrouptitl | e` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scattermap.Line` instance | or dict with compatible properties | lon | Sets the longitude coordinates (in degrees East). | lonsrc | Sets the source reference on Chart Studio Cloud for | `lon`. | marker | :class:`plotly.graph_objects.scattermap.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | selected | :class:`plotly.graph_objects.scattermap.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scattermap.Stream` | instance or dict with compatible properties | subplot | Sets a reference between this trace's data coordinates | and a map subplot. If "map" (the default value), the | data refer to `layout.map`. If "map2", the data refer | to `layout.map2`, and so on. | text | Sets text elements associated with each (lon,lat) pair | If a single string, the same string appears over all | the data points. If an array of string, the items are | mapped in order to the this trace's (lon,lat) | coordinates. If trace `hoverinfo` contains a "text" | flag and "hovertext" is not set, these elements will be | seen in the hover labels. | textfont | Sets the icon text font (color=map.layer.paint.text- | color, size=map.layer.layout.text-size). Has an effect | only when `type` is set to "symbol". | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `lat`, `lon` and `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scattermap.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_scattermapbox(self, below=None, cluster=None, connectgaps=None, customdata=None, customdatasrc=None, fill=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, lat=None, latsrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, lon=None, lonsrc=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, selected=None, selectedpoints=None, showlegend=None, stream=None, subplot=None, text=None, textfont=None, textposition=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Scattermapbox trace | | "scattermapbox" trace is deprecated! Please consider switching | to the "scattermap" trace type and `map` subplots. Learn more | at: https://plotly.com/javascript/maplibre-migration/ The data | visualized as scatter point, lines or marker symbols on a | Mapbox GL geographic map is provided by longitude/latitude | pairs in `lon` and `lat`. | | Parameters | ---------- | below | Determines if this scattermapbox trace's layers are to | be inserted before the layer with the specified ID. By | default, scattermapbox layers are inserted above all | the base layers. To place the scattermapbox layers | above every other layer, set `below` to "''". | cluster | :class:`plotly.graph_objects.scattermapbox.Cluster` | instance or dict with compatible properties | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | fill | Sets the area to fill with a solid color. Use with | `fillcolor` if not "none". "toself" connects the | endpoints of the trace (or each segment of the trace if | it has gaps) into a closed shape. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scattermapbox.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (lon,lat) | pair If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (lon,lat) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | lat | Sets the latitude coordinates (in degrees North). | latsrc | Sets the source reference on Chart Studio Cloud for | `lat`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scattermapbox.Legendgroupt | itle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scattermapbox.Line` | instance or dict with compatible properties | lon | Sets the longitude coordinates (in degrees East). | lonsrc | Sets the source reference on Chart Studio Cloud for | `lon`. | marker | :class:`plotly.graph_objects.scattermapbox.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | selected | :class:`plotly.graph_objects.scattermapbox.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scattermapbox.Stream` | instance or dict with compatible properties | subplot | mapbox subplots and traces are deprecated! Please | consider switching to `map` subplots and traces. Learn | more at: https://plotly.com/javascript/maplibre- | migration/ Sets a reference between this trace's data | coordinates and a mapbox subplot. If "mapbox" (the | default value), the data refer to `layout.mapbox`. If | "mapbox2", the data refer to `layout.mapbox2`, and so | on. | text | Sets text elements associated with each (lon,lat) pair | If a single string, the same string appears over all | the data points. If an array of string, the items are | mapped in order to the this trace's (lon,lat) | coordinates. If trace `hoverinfo` contains a "text" | flag and "hovertext" is not set, these elements will be | seen in the hover labels. | textfont | Sets the icon text font (color=mapbox.layer.paint.text- | color, size=mapbox.layer.layout.text-size). Has an | effect only when `type` is set to "symbol". | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `lat`, `lon` and `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scattermapbox.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_scatterpolar(self, cliponaxis=None, connectgaps=None, customdata=None, customdatasrc=None, dr=None, dtheta=None, fill=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoveron=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, r=None, r0=None, rsrc=None, selected=None, selectedpoints=None, showlegend=None, stream=None, subplot=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, theta=None, theta0=None, thetasrc=None, thetaunit=None, uid=None, uirevision=None, unselected=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Scatterpolar trace | | The scatterpolar trace type encompasses line charts, scatter | charts, text charts, and bubble charts in polar coordinates. | The data visualized as scatter point or lines is set in `r` | (radial) and `theta` (angular) coordinates Text (appearing | either on the chart or on hover only) is via `text`. Bubble | charts are achieved by setting `marker.size` and/or | `marker.color` to numerical arrays. | | Parameters | ---------- | cliponaxis | Determines whether or not markers and text nodes are | clipped about the subplot axes. To show markers and | text nodes above axis lines and tick labels, make sure | to set `xaxis.layer` and `yaxis.layer` to *below | traces*. | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dr | Sets the r coordinate step. | dtheta | Sets the theta coordinate step. By default, the | `dtheta` step equals the subplot's period divided by | the length of the `r` coordinates. | fill | Sets the area to fill with a solid color. Use with | `fillcolor` if not "none". scatterpolar has a subset of | the options available to scatter. "toself" connects the | endpoints of the trace (or each segment of the trace if | it has gaps) into a closed shape. "tonext" fills the | space between two traces if one completely encloses the | other (eg consecutive contour lines), and behaves like | "toself" if there is no trace before it. "tonext" | should not be used if one trace does not enclose the | other. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scatterpolar.Hoverlabel` | instance or dict with compatible properties | hoveron | Do the hover effects highlight individual points | (markers or line points) or do they highlight filled | regions? If the fill is "toself" or "tonext" and there | are no markers or text, then the default is "fills", | otherwise it is "points". | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (x,y) | pair. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scatterpolar.Legendgroupti | tle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scatterpolar.Line` | instance or dict with compatible properties | marker | :class:`plotly.graph_objects.scatterpolar.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. If there are less than | 20 points and the trace is not stacked then the default | is "lines+markers". Otherwise, "lines". | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | r | Sets the radial coordinates | r0 | Alternate to `r`. Builds a linear space of r | coordinates. Use with `dr` where `r0` is the starting | coordinate and `dr` the step. | rsrc | Sets the source reference on Chart Studio Cloud for | `r`. | selected | :class:`plotly.graph_objects.scatterpolar.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scatterpolar.Stream` | instance or dict with compatible properties | subplot | Sets a reference between this trace's data coordinates | and a polar subplot. If "polar" (the default value), | the data refer to `layout.polar`. If "polar2", the data | refer to `layout.polar2`, and so on. | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `r`, `theta` and `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | theta | Sets the angular coordinates | theta0 | Alternate to `theta`. Builds a linear space of theta | coordinates. Use with `dtheta` where `theta0` is the | starting coordinate and `dtheta` the step. | thetasrc | Sets the source reference on Chart Studio Cloud for | `theta`. | thetaunit | Sets the unit of input "theta" values. Has an effect | only when on "linear" angular axes. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scatterpolar.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_scatterpolargl(self, connectgaps=None, customdata=None, customdatasrc=None, dr=None, dtheta=None, fill=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, r=None, r0=None, rsrc=None, selected=None, selectedpoints=None, showlegend=None, stream=None, subplot=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, theta=None, theta0=None, thetasrc=None, thetaunit=None, uid=None, uirevision=None, unselected=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Scatterpolargl trace | | The scatterpolargl trace type encompasses line charts, scatter | charts, and bubble charts in polar coordinates using the WebGL | plotting engine. The data visualized as scatter point or lines | is set in `r` (radial) and `theta` (angular) coordinates Bubble | charts are achieved by setting `marker.size` and/or | `marker.color` to numerical arrays. | | Parameters | ---------- | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | dr | Sets the r coordinate step. | dtheta | Sets the theta coordinate step. By default, the | `dtheta` step equals the subplot's period divided by | the length of the `r` coordinates. | fill | Sets the area to fill with a solid color. Defaults to | "none" unless this trace is stacked, then it gets | "tonexty" ("tonextx") if `orientation` is "v" ("h") Use | with `fillcolor` if not "none". "tozerox" and "tozeroy" | fill to x=0 and y=0 respectively. "tonextx" and | "tonexty" fill between the endpoints of this trace and | the endpoints of the trace before it, connecting those | endpoints with straight lines (to make a stacked area | graph); if there is no trace before it, they behave | like "tozerox" and "tozeroy". "toself" connects the | endpoints of the trace (or each segment of the trace if | it has gaps) into a closed shape. "tonext" fills the | space between two traces if one completely encloses the | other (eg consecutive contour lines), and behaves like | "toself" if there is no trace before it. "tonext" | should not be used if one trace does not enclose the | other. Traces in a `stackgroup` will only fill to (or | be filled to) other traces in the same group. With | multiple `stackgroup`s or some traces stacked and some | not, if fill-linked traces are not already consecutive, | the later ones will be pushed down in the drawing | order. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scatterpolargl.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (x,y) | pair. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scatterpolargl.Legendgroup | title` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scatterpolargl.Line` | instance or dict with compatible properties | marker | :class:`plotly.graph_objects.scatterpolargl.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. If there are less than | 20 points and the trace is not stacked then the default | is "lines+markers". Otherwise, "lines". | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | r | Sets the radial coordinates | r0 | Alternate to `r`. Builds a linear space of r | coordinates. Use with `dr` where `r0` is the starting | coordinate and `dr` the step. | rsrc | Sets the source reference on Chart Studio Cloud for | `r`. | selected | :class:`plotly.graph_objects.scatterpolargl.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scatterpolargl.Stream` | instance or dict with compatible properties | subplot | Sets a reference between this trace's data coordinates | and a polar subplot. If "polar" (the default value), | the data refer to `layout.polar`. If "polar2", the data | refer to `layout.polar2`, and so on. | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `r`, `theta` and `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | theta | Sets the angular coordinates | theta0 | Alternate to `theta`. Builds a linear space of theta | coordinates. Use with `dtheta` where `theta0` is the | starting coordinate and `dtheta` the step. | thetasrc | Sets the source reference on Chart Studio Cloud for | `theta`. | thetaunit | Sets the unit of input "theta" values. Has an effect | only when on "linear" angular axes. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scatterpolargl.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_scattersmith(self, cliponaxis=None, connectgaps=None, customdata=None, customdatasrc=None, fill=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoveron=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, imag=None, imagsrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, real=None, realsrc=None, selected=None, selectedpoints=None, showlegend=None, stream=None, subplot=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Scattersmith trace | | The scattersmith trace type encompasses line charts, scatter | charts, text charts, and bubble charts in smith coordinates. | The data visualized as scatter point or lines is set in `real` | and `imag` (imaginary) coordinates Text (appearing either on | the chart or on hover only) is via `text`. Bubble charts are | achieved by setting `marker.size` and/or `marker.color` to | numerical arrays. | | Parameters | ---------- | cliponaxis | Determines whether or not markers and text nodes are | clipped about the subplot axes. To show markers and | text nodes above axis lines and tick labels, make sure | to set `xaxis.layer` and `yaxis.layer` to *below | traces*. | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | fill | Sets the area to fill with a solid color. Use with | `fillcolor` if not "none". scattersmith has a subset of | the options available to scatter. "toself" connects the | endpoints of the trace (or each segment of the trace if | it has gaps) into a closed shape. "tonext" fills the | space between two traces if one completely encloses the | other (eg consecutive contour lines), and behaves like | "toself" if there is no trace before it. "tonext" | should not be used if one trace does not enclose the | other. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scattersmith.Hoverlabel` | instance or dict with compatible properties | hoveron | Do the hover effects highlight individual points | (markers or line points) or do they highlight filled | regions? If the fill is "toself" or "tonext" and there | are no markers or text, then the default is "fills", | otherwise it is "points". | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (x,y) | pair. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | imag | Sets the imaginary component of the data, in units of | normalized impedance such that real=1, imag=0 is the | center of the chart. | imagsrc | Sets the source reference on Chart Studio Cloud for | `imag`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scattersmith.Legendgroupti | tle` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scattersmith.Line` | instance or dict with compatible properties | marker | :class:`plotly.graph_objects.scattersmith.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. If there are less than | 20 points and the trace is not stacked then the default | is "lines+markers". Otherwise, "lines". | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | real | Sets the real component of the data, in units of | normalized impedance such that real=1, imag=0 is the | center of the chart. | realsrc | Sets the source reference on Chart Studio Cloud for | `real`. | selected | :class:`plotly.graph_objects.scattersmith.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scattersmith.Stream` | instance or dict with compatible properties | subplot | Sets a reference between this trace's data coordinates | and a smith subplot. If "smith" (the default value), | the data refer to `layout.smith`. If "smith2", the data | refer to `layout.smith2`, and so on. | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `real`, `imag` and `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scattersmith.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_scatterternary(self, a=None, asrc=None, b=None, bsrc=None, c=None, cliponaxis=None, connectgaps=None, csrc=None, customdata=None, customdatasrc=None, fill=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoveron=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meta=None, metasrc=None, mode=None, name=None, opacity=None, selected=None, selectedpoints=None, showlegend=None, stream=None, subplot=None, sum=None, text=None, textfont=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, unselected=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Scatterternary trace | | Provides similar functionality to the "scatter" type but on a | ternary phase diagram. The data is provided by at least two | arrays out of `a`, `b`, `c` triplets. | | Parameters | ---------- | a | Sets the quantity of component `a` in each data point. | If `a`, `b`, and `c` are all provided, they need not be | normalized, only the relative values matter. If only | two arrays are provided they must be normalized to | match `ternary<i>.sum`. | asrc | Sets the source reference on Chart Studio Cloud for | `a`. | b | Sets the quantity of component `a` in each data point. | If `a`, `b`, and `c` are all provided, they need not be | normalized, only the relative values matter. If only | two arrays are provided they must be normalized to | match `ternary<i>.sum`. | bsrc | Sets the source reference on Chart Studio Cloud for | `b`. | c | Sets the quantity of component `a` in each data point. | If `a`, `b`, and `c` are all provided, they need not be | normalized, only the relative values matter. If only | two arrays are provided they must be normalized to | match `ternary<i>.sum`. | cliponaxis | Determines whether or not markers and text nodes are | clipped about the subplot axes. To show markers and | text nodes above axis lines and tick labels, make sure | to set `xaxis.layer` and `yaxis.layer` to *below | traces*. | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the provided data arrays are connected. | csrc | Sets the source reference on Chart Studio Cloud for | `c`. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | fill | Sets the area to fill with a solid color. Use with | `fillcolor` if not "none". scatterternary has a subset | of the options available to scatter. "toself" connects | the endpoints of the trace (or each segment of the | trace if it has gaps) into a closed shape. "tonext" | fills the space between two traces if one completely | encloses the other (eg consecutive contour lines), and | behaves like "toself" if there is no trace before it. | "tonext" should not be used if one trace does not | enclose the other. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.scatterternary.Hoverlabel` | instance or dict with compatible properties | hoveron | Do the hover effects highlight individual points | (markers or line points) or do they highlight filled | regions? If the fill is "toself" or "tonext" and there | are no markers or text, then the default is "fills", | otherwise it is "points". | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (a,b,c) | point. If a single string, the same string appears over | all the data points. If an array of strings, the items | are mapped in order to the the data points in (a,b,c). | To be seen, trace `hoverinfo` must contain a "text" | flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.scatterternary.Legendgroup | title` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.scatterternary.Line` | instance or dict with compatible properties | marker | :class:`plotly.graph_objects.scatterternary.Marker` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | mode | Determines the drawing mode for this scatter trace. If | the provided `mode` includes "text" then the `text` | elements appear at the coordinates. Otherwise, the | `text` elements appear on hover. If there are less than | 20 points and the trace is not stacked then the default | is "lines+markers". Otherwise, "lines". | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | selected | :class:`plotly.graph_objects.scatterternary.Selected` | instance or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.scatterternary.Stream` | instance or dict with compatible properties | subplot | Sets a reference between this trace's data coordinates | and a ternary subplot. If "ternary" (the default | value), the data refer to `layout.ternary`. If | "ternary2", the data refer to `layout.ternary2`, and so | on. | sum | The number each triplet should sum to, if only two of | `a`, `b`, and `c` are provided. This overrides | `ternary<i>.sum` to normalize this specific trace, but | does not affect the values displayed on the axes. 0 (or | missing) means to use ternary<i>.sum | text | Sets text elements associated with each (a,b,c) point. | If a single string, the same string appears over all | the data points. If an array of strings, the items are | mapped in order to the the data points in (a,b,c). If | trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textfont | Sets the text font. | textposition | Sets the positions of the `text` elements with respects | to the (x,y) coordinates. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `a`, `b`, `c` and `text`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.scatterternary.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_selection(self, arg=None, line=None, name=None, opacity=None, path=None, templateitemname=None, type=None, x0=None, x1=None, xref=None, y0=None, y1=None, yref=None, row=None, col=None, secondary_y=None, exclude_empty_subplots=None, **kwargs) -> 'FigureWidget' | Create and add a new selection to the figure's layout | | Parameters | ---------- | arg | instance of Selection or dict with compatible | properties | line | :class:`plotly.graph_objects.layout.selection.Line` | instance or dict with compatible properties | name | When used in a template, named items are created in the | output figure in addition to any items the figure | already has in this array. You can modify these items | in the output figure by making your own item with | `templateitemname` matching this `name` alongside your | modifications (including `visible: false` or `enabled: | false` to hide it). Has no effect outside of a | template. | opacity | Sets the opacity of the selection. | path | For `type` "path" - a valid SVG path similar to | `shapes.path` in data coordinates. Allowed segments | are: M, L and Z. | templateitemname | Used to refer to a named item in this array in the | template. Named items from the template will be created | even without a matching item in the input figure, but | you can modify one by making an item with | `templateitemname` matching its `name`, alongside your | modifications (including `visible: false` or `enabled: | false` to hide it). If there is no template or no | matching item, this item will be hidden unless you | explicitly show it with `visible: true`. | type | Specifies the selection type to be drawn. If "rect", a | rectangle is drawn linking (`x0`,`y0`), (`x1`,`y0`), | (`x1`,`y1`) and (`x0`,`y1`). If "path", draw a custom | SVG path using `path`. | x0 | Sets the selection's starting x position. | x1 | Sets the selection's end x position. | xref | Sets the selection's x coordinate axis. If set to a x | axis id (e.g. "x" or "x2"), the `x` position refers to | a x coordinate. If set to "paper", the `x` position | refers to the distance from the left of the plotting | area in normalized coordinates where 0 (1) corresponds | to the left (right). If set to a x axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the left of the | domain of that axis: e.g., *x2 domain* refers to the | domain of the second x axis and a x position of 0.5 | refers to the point between the left and the right of | the domain of the second x axis. | y0 | Sets the selection's starting y position. | y1 | Sets the selection's end y position. | yref | Sets the selection's x coordinate axis. If set to a y | axis id (e.g. "y" or "y2"), the `y` position refers to | a y coordinate. If set to "paper", the `y` position | refers to the distance from the bottom of the plotting | area in normalized coordinates where 0 (1) corresponds | to the bottom (top). If set to a y axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the bottom of the | domain of that axis: e.g., *y2 domain* refers to the | domain of the second y axis and a y position of 0.5 | refers to the point between the bottom and the top of | the domain of the second y axis. | row | Subplot row for selection. If 'all', addresses all rows | in the specified column(s). | col | Subplot column for selection. If 'all', addresses all | columns in the specified row(s). | secondary_y | Whether to add selection to secondary y-axis | exclude_empty_subplots | If True, selection will not be added to subplots | without traces. | | Returns | ------- | FigureWidget | | add_shape(self, arg=None, editable=None, fillcolor=None, fillrule=None, label=None, layer=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, name=None, opacity=None, path=None, showlegend=None, templateitemname=None, type=None, visible=None, x0=None, x0shift=None, x1=None, x1shift=None, xanchor=None, xref=None, xsizemode=None, y0=None, y0shift=None, y1=None, y1shift=None, yanchor=None, yref=None, ysizemode=None, row=None, col=None, secondary_y=None, exclude_empty_subplots=None, **kwargs) -> 'FigureWidget' | Create and add a new shape to the figure's layout | | Parameters | ---------- | arg | instance of Shape or dict with compatible properties | editable | Determines whether the shape could be activated for | edit or not. Has no effect when the older editable | shapes mode is enabled via `config.editable` or | `config.edits.shapePosition`. | fillcolor | Sets the color filling the shape's interior. Only | applies to closed shapes. | fillrule | Determines which regions of complex paths constitute | the interior. For more info please visit | https://developer.mozilla.org/en- | US/docs/Web/SVG/Attribute/fill-rule | label | :class:`plotly.graph_objects.layout.shape.Label` | instance or dict with compatible properties | layer | Specifies whether shapes are drawn below gridlines | ("below"), between gridlines and traces ("between") or | above traces ("above"). | legend | Sets the reference to a legend to show this shape in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this shape. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.layout.shape.Legendgroupti | tle` instance or dict with compatible properties | legendrank | Sets the legend rank for this shape. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this shape. | line | :class:`plotly.graph_objects.layout.shape.Line` | instance or dict with compatible properties | name | When used in a template, named items are created in the | output figure in addition to any items the figure | already has in this array. You can modify these items | in the output figure by making your own item with | `templateitemname` matching this `name` alongside your | modifications (including `visible: false` or `enabled: | false` to hide it). Has no effect outside of a | template. | opacity | Sets the opacity of the shape. | path | For `type` "path" - a valid SVG path with the pixel | values replaced by data values in | `xsizemode`/`ysizemode` being "scaled" and taken | unmodified as pixels relative to `xanchor` and | `yanchor` in case of "pixel" size mode. There are a few | restrictions / quirks only absolute instructions, not | relative. So the allowed segments are: M, L, H, V, Q, | C, T, S, and Z arcs (A) are not allowed because radius | rx and ry are relative. In the future we could consider | supporting relative commands, but we would have to | decide on how to handle date and log axes. Note that | even as is, Q and C Bezier paths that are smooth on | linear axes may not be smooth on log, and vice versa. | no chained "polybezier" commands - specify the segment | type for each one. On category axes, values are numbers | scaled to the serial numbers of categories because | using the categories themselves there would be no way | to describe fractional positions On data axes: because | space and T are both normal components of path strings, | we can't use either to separate date from time parts. | Therefore we'll use underscore for this purpose: | 2015-02-21_13:45:56.789 | showlegend | Determines whether or not this shape is shown in the | legend. | templateitemname | Used to refer to a named item in this array in the | template. Named items from the template will be created | even without a matching item in the input figure, but | you can modify one by making an item with | `templateitemname` matching its `name`, alongside your | modifications (including `visible: false` or `enabled: | false` to hide it). If there is no template or no | matching item, this item will be hidden unless you | explicitly show it with `visible: true`. | type | Specifies the shape type to be drawn. If "line", a line | is drawn from (`x0`,`y0`) to (`x1`,`y1`) with respect | to the axes' sizing mode. If "circle", a circle is | drawn from ((`x0`+`x1`)/2, (`y0`+`y1`)/2)) with radius | (|(`x0`+`x1`)/2 - `x0`|, |(`y0`+`y1`)/2 -`y0`)|) with | respect to the axes' sizing mode. If "rect", a | rectangle is drawn linking (`x0`,`y0`), (`x1`,`y0`), | (`x1`,`y1`), (`x0`,`y1`), (`x0`,`y0`) with respect to | the axes' sizing mode. If "path", draw a custom SVG | path using `path`. with respect to the axes' sizing | mode. | visible | Determines whether or not this shape is visible. If | "legendonly", the shape is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x0 | Sets the shape's starting x position. See `type` and | `xsizemode` for more info. | x0shift | Shifts `x0` away from the center of the category when | `xref` is a "category" or "multicategory" axis. -0.5 | corresponds to the start of the category and 0.5 | corresponds to the end of the category. | x1 | Sets the shape's end x position. See `type` and | `xsizemode` for more info. | x1shift | Shifts `x1` away from the center of the category when | `xref` is a "category" or "multicategory" axis. -0.5 | corresponds to the start of the category and 0.5 | corresponds to the end of the category. | xanchor | Only relevant in conjunction with `xsizemode` set to | "pixel". Specifies the anchor point on the x axis to | which `x0`, `x1` and x coordinates within `path` are | relative to. E.g. useful to attach a pixel sized shape | to a certain data value. No effect when `xsizemode` not | set to "pixel". | xref | Sets the shape's x coordinate axis. If set to a x axis | id (e.g. "x" or "x2"), the `x` position refers to a x | coordinate. If set to "paper", the `x` position refers | to the distance from the left of the plotting area in | normalized coordinates where 0 (1) corresponds to the | left (right). If set to a x axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the left of the | domain of that axis: e.g., *x2 domain* refers to the | domain of the second x axis and a x position of 0.5 | refers to the point between the left and the right of | the domain of the second x axis. | xsizemode | Sets the shapes's sizing mode along the x axis. If set | to "scaled", `x0`, `x1` and x coordinates within `path` | refer to data values on the x axis or a fraction of the | plot area's width (`xref` set to "paper"). If set to | "pixel", `xanchor` specifies the x position in terms of | data or plot fraction but `x0`, `x1` and x coordinates | within `path` are pixels relative to `xanchor`. This | way, the shape can have a fixed width while maintaining | a position relative to data or plot fraction. | y0 | Sets the shape's starting y position. See `type` and | `ysizemode` for more info. | y0shift | Shifts `y0` away from the center of the category when | `yref` is a "category" or "multicategory" axis. -0.5 | corresponds to the start of the category and 0.5 | corresponds to the end of the category. | y1 | Sets the shape's end y position. See `type` and | `ysizemode` for more info. | y1shift | Shifts `y1` away from the center of the category when | `yref` is a "category" or "multicategory" axis. -0.5 | corresponds to the start of the category and 0.5 | corresponds to the end of the category. | yanchor | Only relevant in conjunction with `ysizemode` set to | "pixel". Specifies the anchor point on the y axis to | which `y0`, `y1` and y coordinates within `path` are | relative to. E.g. useful to attach a pixel sized shape | to a certain data value. No effect when `ysizemode` not | set to "pixel". | yref | Sets the shape's y coordinate axis. If set to a y axis | id (e.g. "y" or "y2"), the `y` position refers to a y | coordinate. If set to "paper", the `y` position refers | to the distance from the bottom of the plotting area in | normalized coordinates where 0 (1) corresponds to the | bottom (top). If set to a y axis ID followed by | "domain" (separated by a space), the position behaves | like for "paper", but refers to the distance in | fractions of the domain length from the bottom of the | domain of that axis: e.g., *y2 domain* refers to the | domain of the second y axis and a y position of 0.5 | refers to the point between the bottom and the top of | the domain of the second y axis. | ysizemode | Sets the shapes's sizing mode along the y axis. If set | to "scaled", `y0`, `y1` and y coordinates within `path` | refer to data values on the y axis or a fraction of the | plot area's height (`yref` set to "paper"). If set to | "pixel", `yanchor` specifies the y position in terms of | data or plot fraction but `y0`, `y1` and y coordinates | within `path` are pixels relative to `yanchor`. This | way, the shape can have a fixed height while | maintaining a position relative to data or plot | fraction. | row | Subplot row for shape. If 'all', addresses all rows in | the specified column(s). | col | Subplot column for shape. If 'all', addresses all | columns in the specified row(s). | secondary_y | Whether to add shape to secondary y-axis | exclude_empty_subplots | If True, shape will not be added to subplots without | traces. | | Returns | ------- | FigureWidget | | add_splom(self, customdata=None, customdatasrc=None, diagonal=None, dimensions=None, dimensiondefaults=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, marker=None, meta=None, metasrc=None, name=None, opacity=None, selected=None, selectedpoints=None, showlegend=None, showlowerhalf=None, showupperhalf=None, stream=None, text=None, textsrc=None, uid=None, uirevision=None, unselected=None, visible=None, xaxes=None, xhoverformat=None, yaxes=None, yhoverformat=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Splom trace | | Splom traces generate scatter plot matrix visualizations. Each | splom `dimensions` items correspond to a generated axis. Values | for each of those dimensions are set in `dimensions[i].values`. | Splom traces support all `scattergl` marker style attributes. | Specify `layout.grid` attributes and/or layout x-axis and | y-axis attributes for more control over the axis positioning | and style. | | Parameters | ---------- | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | diagonal | :class:`plotly.graph_objects.splom.Diagonal` instance | or dict with compatible properties | dimensions | A tuple of | :class:`plotly.graph_objects.splom.Dimension` instances | or dicts with compatible properties | dimensiondefaults | When used in a template (as | layout.template.data.splom.dimensiondefaults), sets the | default property values to use for elements of | splom.dimensions | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.splom.Hoverlabel` instance | or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.splom.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | marker | :class:`plotly.graph_objects.splom.Marker` instance or | dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | selected | :class:`plotly.graph_objects.splom.Selected` instance | or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showlowerhalf | Determines whether or not subplots on the lower half | from the diagonal are displayed. | showupperhalf | Determines whether or not subplots on the upper half | from the diagonal are displayed. | stream | :class:`plotly.graph_objects.splom.Stream` instance or | dict with compatible properties | text | Sets text elements associated with each (x,y) pair to | appear on hover. If a single string, the same string | appears over all the data points. If an array of | string, the items are mapped in order to the this | trace's (x,y) coordinates. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.splom.Unselected` instance | or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | xaxes | Sets the list of x axes corresponding to dimensions of | this splom trace. By default, a splom will match the | first N xaxes where N is the number of input | dimensions. Note that, in case where `diagonal.visible` | is false and `showupperhalf` or `showlowerhalf` is | false, this splom trace will generate one less x-axis | and one less y-axis. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | yaxes | Sets the list of y axes corresponding to dimensions of | this splom trace. By default, a splom will match the | first N yaxes where N is the number of input | dimensions. Note that, in case where `diagonal.visible` | is false and `showupperhalf` or `showlowerhalf` is | false, this splom trace will generate one less x-axis | and one less y-axis. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_streamtube(self, autocolorscale=None, cauto=None, cmax=None, cmid=None, cmin=None, coloraxis=None, colorbar=None, colorscale=None, customdata=None, customdatasrc=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, lighting=None, lightposition=None, maxdisplayed=None, meta=None, metasrc=None, name=None, opacity=None, reversescale=None, scene=None, showlegend=None, showscale=None, sizeref=None, starts=None, stream=None, text=None, u=None, uhoverformat=None, uid=None, uirevision=None, usrc=None, v=None, vhoverformat=None, visible=None, vsrc=None, w=None, whoverformat=None, wsrc=None, x=None, xhoverformat=None, xsrc=None, y=None, yhoverformat=None, ysrc=None, z=None, zhoverformat=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Streamtube trace | | Use a streamtube trace to visualize flow in a vector field. | Specify a vector field using 6 1D arrays of equal length, 3 | position arrays `x`, `y` and `z` and 3 vector component arrays | `u`, `v`, and `w`. By default, the tubes' starting positions | will be cut from the vector field's x-z plane at its minimum y | value. To specify your own starting position, use attributes | `starts.x`, `starts.y` and `starts.z`. The color is encoded by | the norm of (u, v, w), and the local radius by the divergence | of (u, v, w). | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | cauto | Determines whether or not the color domain is computed | with respect to the input data (here u/v/w norm) or the | bounds set in `cmin` and `cmax` Defaults to `false` | when `cmin` and `cmax` are set by the user. | cmax | Sets the upper bound of the color domain. Value should | have the same units as u/v/w norm and if set, `cmin` | must be set as well. | cmid | Sets the mid-point of the color domain by scaling | `cmin` and/or `cmax` to be equidistant to this point. | Value should have the same units as u/v/w norm. Has no | effect when `cauto` is `false`. | cmin | Sets the lower bound of the color domain. Value should | have the same units as u/v/w norm and if set, `cmax` | must be set as well. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.streamtube.ColorBar` | instance or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `cmin` and `cmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.streamtube.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `tubex`, `tubey`, `tubez`, `tubeu`, | `tubev`, `tubew`, `norm` and `divergence`. Anything | contained in tag `<extra>` is displayed in the | secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.streamtube.Legendgrouptitl | e` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | lighting | :class:`plotly.graph_objects.streamtube.Lighting` | instance or dict with compatible properties | lightposition | :class:`plotly.graph_objects.streamtube.Lightposition` | instance or dict with compatible properties | maxdisplayed | The maximum number of displayed segments in a | streamtube. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the surface. Please note that in | the case of using high `opacity` values for example a | value greater than or equal to 0.5 on two surfaces (and | 0.25 with four surfaces), an overlay of multiple | transparent surfaces may not perfectly be sorted in | depth by the webgl API. This behavior may be improved | in the near future and is subject to change. | reversescale | Reverses the color mapping if true. If true, `cmin` | will correspond to the last color in the array and | `cmax` will correspond to the first color. | scene | Sets a reference between this trace's 3D coordinate | system and a 3D scene. If "scene" (the default value), | the (x,y,z) coordinates refer to `layout.scene`. If | "scene2", the (x,y,z) coordinates refer to | `layout.scene2`, and so on. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | sizeref | The scaling factor for the streamtubes. The default is | 1, which avoids two max divergence tubes from touching | at adjacent starting positions. | starts | :class:`plotly.graph_objects.streamtube.Starts` | instance or dict with compatible properties | stream | :class:`plotly.graph_objects.streamtube.Stream` | instance or dict with compatible properties | text | Sets a text element associated with this trace. If | trace `hoverinfo` contains a "text" flag, this text | element will be seen in all hover labels. Note that | streamtube traces do not support array `text` values. | u | Sets the x components of the vector field. | uhoverformat | Sets the hover text formatting rulefor `u` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | usrc | Sets the source reference on Chart Studio Cloud for | `u`. | v | Sets the y components of the vector field. | vhoverformat | Sets the hover text formatting rulefor `v` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | vsrc | Sets the source reference on Chart Studio Cloud for | `v`. | w | Sets the z components of the vector field. | whoverformat | Sets the hover text formatting rulefor `w` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | wsrc | Sets the source reference on Chart Studio Cloud for | `w`. | x | Sets the x coordinates of the vector field. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates of the vector field. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the z coordinates of the vector field. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `zaxis.hoverformat`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_sunburst(self, branchvalues=None, count=None, customdata=None, customdatasrc=None, domain=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, insidetextfont=None, insidetextorientation=None, labels=None, labelssrc=None, leaf=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, level=None, marker=None, maxdepth=None, meta=None, metasrc=None, name=None, opacity=None, outsidetextfont=None, parents=None, parentssrc=None, root=None, rotation=None, sort=None, stream=None, text=None, textfont=None, textinfo=None, textsrc=None, texttemplate=None, texttemplatesrc=None, uid=None, uirevision=None, values=None, valuessrc=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Sunburst trace | | Visualize hierarchal data spanning outward radially from root | to leaves. The sunburst sectors are determined by the entries | in "labels" or "ids" and in "parents". | | Parameters | ---------- | branchvalues | Determines how the items in `values` are summed. When | set to "total", items in `values` are taken to be value | of all its descendants. When set to "remainder", items | in `values` corresponding to the root and the branches | sectors are taken to be the extra part not part of the | sum of the values at their leaves. | count | Determines default for `values` when it is not | provided, by inferring a 1 for each of the "leaves" | and/or "branches", otherwise 0. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | domain | :class:`plotly.graph_objects.sunburst.Domain` instance | or dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.sunburst.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `currentPath`, `root`, `entry`, | `percentRoot`, `percentEntry` and `percentParent`. | Anything contained in tag `<extra>` is displayed in the | secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each sector. | If a single string, the same string appears for all | data points. If an array of string, the items are | mapped in order of this trace's sectors. To be seen, | trace `hoverinfo` must contain a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | insidetextfont | Sets the font used for `textinfo` lying inside the | sector. | insidetextorientation | Controls the orientation of the text inside chart | sectors. When set to "auto", text may be oriented in | any direction in order to be as big as possible in the | middle of a sector. The "horizontal" option orients | text to be parallel with the bottom of the chart, and | may make text smaller in order to achieve that goal. | The "radial" option orients text along the radius of | the sector. The "tangential" option orients text | perpendicular to the radius of the sector. | labels | Sets the labels of each of the sectors. | labelssrc | Sets the source reference on Chart Studio Cloud for | `labels`. | leaf | :class:`plotly.graph_objects.sunburst.Leaf` instance or | dict with compatible properties | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.sunburst.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | level | Sets the level from which this trace hierarchy is | rendered. Set `level` to `''` to start from the root | node in the hierarchy. Must be an "id" if `ids` is | filled in, otherwise plotly attempts to find a matching | item in `labels`. | marker | :class:`plotly.graph_objects.sunburst.Marker` instance | or dict with compatible properties | maxdepth | Sets the number of rendered sectors from any given | `level`. Set `maxdepth` to "-1" to render all the | levels in the hierarchy. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | outsidetextfont | Sets the font used for `textinfo` lying outside the | sector. This option refers to the root of the hierarchy | presented at the center of a sunburst graph. Please | note that if a hierarchy has multiple root nodes, this | option won't have any effect and `insidetextfont` would | be used. | parents | Sets the parent sectors for each of the sectors. Empty | string items '' are understood to reference the root | node in the hierarchy. If `ids` is filled, `parents` | items are understood to be "ids" themselves. When `ids` | is not set, plotly attempts to find matching items in | `labels`, but beware they must be unique. | parentssrc | Sets the source reference on Chart Studio Cloud for | `parents`. | root | :class:`plotly.graph_objects.sunburst.Root` instance or | dict with compatible properties | rotation | Rotates the whole diagram counterclockwise by some | angle. By default the first slice starts at 3 o'clock. | sort | Determines whether or not the sectors are reordered | from largest to smallest. | stream | :class:`plotly.graph_objects.sunburst.Stream` instance | or dict with compatible properties | text | Sets text elements associated with each sector. If | trace `textinfo` contains a "text" flag, these elements | will be seen on the chart. If trace `hoverinfo` | contains a "text" flag and "hovertext" is not set, | these elements will be seen in the hover labels. | textfont | Sets the font used for `textinfo`. | textinfo | Determines which trace information appear on the graph. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `currentPath`, `root`, `entry`, | `percentRoot`, `percentEntry`, `percentParent`, `label` | and `value`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | values | Sets the values associated with each of the sectors. | Use with `branchvalues` to determine how the values are | summed. | valuessrc | Sets the source reference on Chart Studio Cloud for | `values`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_surface(self, autocolorscale=None, cauto=None, cmax=None, cmid=None, cmin=None, coloraxis=None, colorbar=None, colorscale=None, connectgaps=None, contours=None, customdata=None, customdatasrc=None, hidesurface=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, lighting=None, lightposition=None, meta=None, metasrc=None, name=None, opacity=None, opacityscale=None, reversescale=None, scene=None, showlegend=None, showscale=None, stream=None, surfacecolor=None, surfacecolorsrc=None, text=None, textsrc=None, uid=None, uirevision=None, visible=None, x=None, xcalendar=None, xhoverformat=None, xsrc=None, y=None, ycalendar=None, yhoverformat=None, ysrc=None, z=None, zcalendar=None, zhoverformat=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Surface trace | | The data the describes the coordinates of the surface is set in | `z`. Data in `z` should be a 2D list. Coordinates in `x` and | `y` can either be 1D lists or 2D lists (e.g. to graph | parametric surfaces). If not provided in `x` and `y`, the x and | y coordinates are assumed to be linear starting at 0 with a | unit step. The color scale corresponds to the `z` values by | default. For custom color scales, use `surfacecolor` which | should be a 2D list, where its bounds can be controlled using | `cmin` and `cmax`. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | cauto | Determines whether or not the color domain is computed | with respect to the input data (here z or surfacecolor) | or the bounds set in `cmin` and `cmax` Defaults to | `false` when `cmin` and `cmax` are set by the user. | cmax | Sets the upper bound of the color domain. Value should | have the same units as z or surfacecolor and if set, | `cmin` must be set as well. | cmid | Sets the mid-point of the color domain by scaling | `cmin` and/or `cmax` to be equidistant to this point. | Value should have the same units as z or surfacecolor. | Has no effect when `cauto` is `false`. | cmin | Sets the lower bound of the color domain. Value should | have the same units as z or surfacecolor and if set, | `cmax` must be set as well. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.surface.ColorBar` instance | or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `cmin` and `cmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | connectgaps | Determines whether or not gaps (i.e. {nan} or missing | values) in the `z` data are filled in. | contours | :class:`plotly.graph_objects.surface.Contours` instance | or dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | hidesurface | Determines whether or not a surface is drawn. For | example, set `hidesurface` to False `contours.x.show` | to True and `contours.y.show` to True to draw a wire | frame plot. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.surface.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.surface.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | lighting | :class:`plotly.graph_objects.surface.Lighting` instance | or dict with compatible properties | lightposition | :class:`plotly.graph_objects.surface.Lightposition` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the surface. Please note that in | the case of using high `opacity` values for example a | value greater than or equal to 0.5 on two surfaces (and | 0.25 with four surfaces), an overlay of multiple | transparent surfaces may not perfectly be sorted in | depth by the webgl API. This behavior may be improved | in the near future and is subject to change. | opacityscale | Sets the opacityscale. The opacityscale must be an | array containing arrays mapping a normalized value to | an opacity value. At minimum, a mapping for the lowest | (0) and highest (1) values are required. For example, | `[[0, 1], [0.5, 0.2], [1, 1]]` means that higher/lower | values would have higher opacity values and those in | the middle would be more transparent Alternatively, | `opacityscale` may be a palette name string of the | following list: 'min', 'max', 'extremes' and 'uniform'. | The default is 'uniform'. | reversescale | Reverses the color mapping if true. If true, `cmin` | will correspond to the last color in the array and | `cmax` will correspond to the first color. | scene | Sets a reference between this trace's 3D coordinate | system and a 3D scene. If "scene" (the default value), | the (x,y,z) coordinates refer to `layout.scene`. If | "scene2", the (x,y,z) coordinates refer to | `layout.scene2`, and so on. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | stream | :class:`plotly.graph_objects.surface.Stream` instance | or dict with compatible properties | surfacecolor | Sets the surface color values, used for setting a color | scale independent of `z`. | surfacecolorsrc | Sets the source reference on Chart Studio Cloud for | `surfacecolor`. | text | Sets the text elements associated with each z value. If | trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the x coordinates. | xcalendar | Sets the calendar system to use with `x` date data. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates. | ycalendar | Sets the calendar system to use with `y` date data. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the z coordinates. | zcalendar | Sets the calendar system to use with `z` date data. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `zaxis.hoverformat`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_table(self, cells=None, columnorder=None, columnordersrc=None, columnwidth=None, columnwidthsrc=None, customdata=None, customdatasrc=None, domain=None, header=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, ids=None, idssrc=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, meta=None, metasrc=None, name=None, stream=None, uid=None, uirevision=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Table trace | | Table view for detailed data viewing. The data are arranged in | a grid of rows and columns. Most styling can be specified for | columns, rows or individual cells. Table is using a column- | major order, ie. the grid is represented as a vector of column | vectors. | | Parameters | ---------- | cells | :class:`plotly.graph_objects.table.Cells` instance or | dict with compatible properties | columnorder | Specifies the rendered order of the data columns; for | example, a value `2` at position `0` means that column | index `0` in the data will be rendered as the third | column, as columns have an index base of zero. | columnordersrc | Sets the source reference on Chart Studio Cloud for | `columnorder`. | columnwidth | The width of columns expressed as a ratio. Columns fill | the available width in proportion of their specified | column widths. | columnwidthsrc | Sets the source reference on Chart Studio Cloud for | `columnwidth`. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | domain | :class:`plotly.graph_objects.table.Domain` instance or | dict with compatible properties | header | :class:`plotly.graph_objects.table.Header` instance or | dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.table.Hoverlabel` instance | or dict with compatible properties | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.table.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | stream | :class:`plotly.graph_objects.table.Stream` instance or | dict with compatible properties | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_trace(self, trace, row=None, col=None, secondary_y=None, exclude_empty_subplots=False) -> 'FigureWidget' | Add a trace to the figure | | Parameters | ---------- | trace : BaseTraceType or dict | Either: | - An instances of a trace classe from the plotly.graph_objs | package (e.g plotly.graph_objs.Scatter, plotly.graph_objs.Bar) | - or a dicts where: | | - The 'type' property specifies the trace type (e.g. | 'scatter', 'bar', 'area', etc.). If the dict has no 'type' | property then 'scatter' is assumed. | - All remaining properties are passed to the constructor | of the specified trace type. | | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`. | If 'all', addresses all rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`. | If 'all', addresses all columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | * The trace argument is a 2D cartesian trace | (scatter, bar, etc.) | exclude_empty_subplots: boolean | If True, the trace will not be added to subplots that don't already | have traces. | Returns | ------- | BaseFigure | The Figure that add_trace was called on | | Examples | -------- | | >>> from plotly import subplots | >>> import plotly.graph_objs as go | | Add two Scatter traces to a figure | | >>> fig = go.Figure() | >>> fig.add_trace(go.Scatter(x=[1,2,3], y=[2,1,2])) # doctest: +ELLIPSIS | Figure(...) | >>> fig.add_trace(go.Scatter(x=[1,2,3], y=[2,1,2])) # doctest: +ELLIPSIS | Figure(...) | | | Add two Scatter traces to vertically stacked subplots | | >>> fig = subplots.make_subplots(rows=2) | >>> fig.add_trace(go.Scatter(x=[1,2,3], y=[2,1,2]), row=1, col=1) # doctest: +ELLIPSIS | Figure(...) | >>> fig.add_trace(go.Scatter(x=[1,2,3], y=[2,1,2]), row=2, col=1) # doctest: +ELLIPSIS | Figure(...) | | add_traces(self, data, rows=None, cols=None, secondary_ys=None, exclude_empty_subplots=False) -> 'FigureWidget' | Add traces to the figure | | Parameters | ---------- | data : list[BaseTraceType or dict] | A list of trace specifications to be added. | Trace specifications may be either: | | - Instances of trace classes from the plotly.graph_objs | package (e.g plotly.graph_objs.Scatter, plotly.graph_objs.Bar) | - Dicts where: | | - The 'type' property specifies the trace type (e.g. | 'scatter', 'bar', 'area', etc.). If the dict has no 'type' | property then 'scatter' is assumed. | - All remaining properties are passed to the constructor | of the specified trace type. | | rows : None, list[int], or int (default None) | List of subplot row indexes (starting from 1) for the traces to be | added. Only valid if figure was created using | `plotly.tools.make_subplots` | If a single integer is passed, all traces will be added to row number | | cols : None or list[int] (default None) | List of subplot column indexes (starting from 1) for the traces | to be added. Only valid if figure was created using | `plotly.tools.make_subplots` | If a single integer is passed, all traces will be added to column number | | | secondary_ys: None or list[boolean] (default None) | List of secondary_y booleans for traces to be added. See the | docstring for `add_trace` for more info. | | exclude_empty_subplots: boolean | If True, the trace will not be added to subplots that don't already | have traces. | | Returns | ------- | BaseFigure | The Figure that add_traces was called on | | Examples | -------- | | >>> from plotly import subplots | >>> import plotly.graph_objs as go | | Add two Scatter traces to a figure | | >>> fig = go.Figure() | >>> fig.add_traces([go.Scatter(x=[1,2,3], y=[2,1,2]), | ... go.Scatter(x=[1,2,3], y=[2,1,2])]) # doctest: +ELLIPSIS | Figure(...) | | Add two Scatter traces to vertically stacked subplots | | >>> fig = subplots.make_subplots(rows=2) | >>> fig.add_traces([go.Scatter(x=[1,2,3], y=[2,1,2]), | ... go.Scatter(x=[1,2,3], y=[2,1,2])], | ... rows=[1, 2], cols=[1, 1]) # doctest: +ELLIPSIS | Figure(...) | | add_treemap(self, branchvalues=None, count=None, customdata=None, customdatasrc=None, domain=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, insidetextfont=None, labels=None, labelssrc=None, legend=None, legendgrouptitle=None, legendrank=None, legendwidth=None, level=None, marker=None, maxdepth=None, meta=None, metasrc=None, name=None, opacity=None, outsidetextfont=None, parents=None, parentssrc=None, pathbar=None, root=None, sort=None, stream=None, text=None, textfont=None, textinfo=None, textposition=None, textsrc=None, texttemplate=None, texttemplatesrc=None, tiling=None, uid=None, uirevision=None, values=None, valuessrc=None, visible=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Treemap trace | | Visualize hierarchal data from leaves (and/or outer branches) | towards root with rectangles. The treemap sectors are | determined by the entries in "labels" or "ids" and in | "parents". | | Parameters | ---------- | branchvalues | Determines how the items in `values` are summed. When | set to "total", items in `values` are taken to be value | of all its descendants. When set to "remainder", items | in `values` corresponding to the root and the branches | sectors are taken to be the extra part not part of the | sum of the values at their leaves. | count | Determines default for `values` when it is not | provided, by inferring a 1 for each of the "leaves" | and/or "branches", otherwise 0. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | domain | :class:`plotly.graph_objects.treemap.Domain` instance | or dict with compatible properties | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.treemap.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `currentPath`, `root`, `entry`, | `percentRoot`, `percentEntry` and `percentParent`. | Anything contained in tag `<extra>` is displayed in the | secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each sector. | If a single string, the same string appears for all | data points. If an array of string, the items are | mapped in order of this trace's sectors. To be seen, | trace `hoverinfo` must contain a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | insidetextfont | Sets the font used for `textinfo` lying inside the | sector. | labels | Sets the labels of each of the sectors. | labelssrc | Sets the source reference on Chart Studio Cloud for | `labels`. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgrouptitle | :class:`plotly.graph_objects.treemap.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | level | Sets the level from which this trace hierarchy is | rendered. Set `level` to `''` to start from the root | node in the hierarchy. Must be an "id" if `ids` is | filled in, otherwise plotly attempts to find a matching | item in `labels`. | marker | :class:`plotly.graph_objects.treemap.Marker` instance | or dict with compatible properties | maxdepth | Sets the number of rendered sectors from any given | `level`. Set `maxdepth` to "-1" to render all the | levels in the hierarchy. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the trace. | outsidetextfont | Sets the font used for `textinfo` lying outside the | sector. This option refers to the root of the hierarchy | presented on top left corner of a treemap graph. Please | note that if a hierarchy has multiple root nodes, this | option won't have any effect and `insidetextfont` would | be used. | parents | Sets the parent sectors for each of the sectors. Empty | string items '' are understood to reference the root | node in the hierarchy. If `ids` is filled, `parents` | items are understood to be "ids" themselves. When `ids` | is not set, plotly attempts to find matching items in | `labels`, but beware they must be unique. | parentssrc | Sets the source reference on Chart Studio Cloud for | `parents`. | pathbar | :class:`plotly.graph_objects.treemap.Pathbar` instance | or dict with compatible properties | root | :class:`plotly.graph_objects.treemap.Root` instance or | dict with compatible properties | sort | Determines whether or not the sectors are reordered | from largest to smallest. | stream | :class:`plotly.graph_objects.treemap.Stream` instance | or dict with compatible properties | text | Sets text elements associated with each sector. If | trace `textinfo` contains a "text" flag, these elements | will be seen on the chart. If trace `hoverinfo` | contains a "text" flag and "hovertext" is not set, | these elements will be seen in the hover labels. | textfont | Sets the font used for `textinfo`. | textinfo | Determines which trace information appear on the graph. | textposition | Sets the positions of the `text` elements. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `currentPath`, `root`, `entry`, | `percentRoot`, `percentEntry`, `percentParent`, `label` | and `value`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | tiling | :class:`plotly.graph_objects.treemap.Tiling` instance | or dict with compatible properties | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | values | Sets the values associated with each of the sectors. | Use with `branchvalues` to determine how the values are | summed. | valuessrc | Sets the source reference on Chart Studio Cloud for | `values`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_violin(self, alignmentgroup=None, bandwidth=None, box=None, customdata=None, customdatasrc=None, fillcolor=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hoveron=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, jitter=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, line=None, marker=None, meanline=None, meta=None, metasrc=None, name=None, offsetgroup=None, opacity=None, orientation=None, pointpos=None, points=None, quartilemethod=None, scalegroup=None, scalemode=None, selected=None, selectedpoints=None, showlegend=None, side=None, span=None, spanmode=None, stream=None, text=None, textsrc=None, uid=None, uirevision=None, unselected=None, visible=None, width=None, x=None, x0=None, xaxis=None, xhoverformat=None, xsrc=None, y=None, y0=None, yaxis=None, yhoverformat=None, ysrc=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Violin trace | | In vertical (horizontal) violin plots, statistics are computed | using `y` (`x`) values. By supplying an `x` (`y`) array, one | violin per distinct x (y) value is drawn If no `x` (`y`) list | is provided, a single violin is drawn. That violin position is | then positioned with with `name` or with `x0` (`y0`) if | provided. | | Parameters | ---------- | alignmentgroup | Set several traces linked to the same position axis or | matching axes to the same alignmentgroup. This controls | whether bars compute their positional range dependently | or independently. | bandwidth | Sets the bandwidth used to compute the kernel density | estimate. By default, the bandwidth is determined by | Silverman's rule of thumb. | box | :class:`plotly.graph_objects.violin.Box` instance or | dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | fillcolor | Sets the fill color. Defaults to a half-transparent | variant of the line color, marker color, or marker line | color, whichever is available. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.violin.Hoverlabel` | instance or dict with compatible properties | hoveron | Do the hover effects highlight individual violins or | sample points or the kernel density estimate or any | combination of them? | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | jitter | Sets the amount of jitter in the sample points drawn. | If 0, the sample points align along the distribution | axis. If 1, the sample points are drawn in a random | jitter of width equal to the width of the violins. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.violin.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | line | :class:`plotly.graph_objects.violin.Line` instance or | dict with compatible properties | marker | :class:`plotly.graph_objects.violin.Marker` instance or | dict with compatible properties | meanline | :class:`plotly.graph_objects.violin.Meanline` instance | or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. For violin traces, the name | will also be used for the position coordinate, if `x` | and `x0` (`y` and `y0` if horizontal) are missing and | the position axis is categorical. Note that the trace | name is also used as a default value for attribute | `scalegroup` (please see its description for details). | offsetgroup | Set several traces linked to the same position axis or | matching axes to the same offsetgroup where bars of the | same position coordinate will line up. | opacity | Sets the opacity of the trace. | orientation | Sets the orientation of the violin(s). If "v" ("h"), | the distribution is visualized along the vertical | (horizontal). | pointpos | Sets the position of the sample points in relation to | the violins. If 0, the sample points are places over | the center of the violins. Positive (negative) values | correspond to positions to the right (left) for | vertical violins and above (below) for horizontal | violins. | points | If "outliers", only the sample points lying outside the | whiskers are shown If "suspectedoutliers", the outlier | points are shown and points either less than 4*Q1-3*Q3 | or greater than 4*Q3-3*Q1 are highlighted (see | `outliercolor`) If "all", all sample points are shown | If False, only the violins are shown with no sample | points. Defaults to "suspectedoutliers" when | `marker.outliercolor` or `marker.line.outliercolor` is | set, otherwise defaults to "outliers". | quartilemethod | Sets the method used to compute the sample's Q1 and Q3 | quartiles. The "linear" method uses the 25th percentile | for Q1 and 75th percentile for Q3 as computed using | method #10 (listed on | http://jse.amstat.org/v14n3/langford.html). The | "exclusive" method uses the median to divide the | ordered dataset into two halves if the sample is odd, | it does not include the median in either half - Q1 is | then the median of the lower half and Q3 the median of | the upper half. The "inclusive" method also uses the | median to divide the ordered dataset into two halves | but if the sample is odd, it includes the median in | both halves - Q1 is then the median of the lower half | and Q3 the median of the upper half. | scalegroup | If there are multiple violins that should be sized | according to to some metric (see `scalemode`), link | them by providing a non-empty group id here shared by | every trace in the same group. If a violin's `width` is | undefined, `scalegroup` will default to the trace's | name. In this case, violins with the same names will be | linked together | scalemode | Sets the metric by which the width of each violin is | determined. "width" means each violin has the same | (max) width "count" means the violins are scaled by the | number of sample points making up each violin. | selected | :class:`plotly.graph_objects.violin.Selected` instance | or dict with compatible properties | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | side | Determines on which side of the position value the | density function making up one half of a violin is | plotted. Useful when comparing two violin traces under | "overlay" mode, where one trace has `side` set to | "positive" and the other to "negative". | span | Sets the span in data space for which the density | function will be computed. Has an effect only when | `spanmode` is set to "manual". | spanmode | Sets the method by which the span in data space where | the density function will be computed. "soft" means the | span goes from the sample's minimum value minus two | bandwidths to the sample's maximum value plus two | bandwidths. "hard" means the span goes from the | sample's minimum to its maximum value. For custom span | settings, use mode "manual" and fill in the `span` | attribute. | stream | :class:`plotly.graph_objects.violin.Stream` instance or | dict with compatible properties | text | Sets the text elements associated with each sample | value. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | unselected | :class:`plotly.graph_objects.violin.Unselected` | instance or dict with compatible properties | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | width | Sets the width of the violin in data coordinates. If 0 | (default value) the width is automatically selected | based on the positions of other violin traces in the | same subplot. | x | Sets the x sample data or coordinates. See overview for | more info. | x0 | Sets the x coordinate for single-box traces or the | starting coordinate for multi-box traces set using | q1/median/q3. See overview for more info. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y sample data or coordinates. See overview for | more info. | y0 | Sets the y coordinate for single-box traces or the | starting coordinate for multi-box traces set using | q1/median/q3. See overview for more info. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | add_vline(self, x, row='all', col='all', exclude_empty_subplots=True, annotation=None, **kwargs) -> 'FigureWidget' | Add a vertical line to a plot or subplot that extends infinitely in the | y-dimension. | | Parameters | ---------- | x: float or int | A number representing the x coordinate of the vertical line. | exclude_empty_subplots: Boolean | If True (default) do not place the shape on subplots that have no data | plotted on them. | row: None, int or 'all' | Subplot row for shape indexed starting at 1. If 'all', addresses all rows in | the specified column(s). If both row and col are None, addresses the | first subplot if subplots exist, or the only plot. By default is "all". | col: None, int or 'all' | Subplot column for shape indexed starting at 1. If 'all', addresses all rows in | the specified column(s). If both row and col are None, addresses the | first subplot if subplots exist, or the only plot. By default is "all". | annotation: dict or plotly.graph_objects.layout.Annotation. If dict(), | it is interpreted as describing an annotation. The annotation is | placed relative to the shape based on annotation_position (see | below) unless its x or y value has been specified for the annotation | passed here. xref and yref are always the same as for the added | shape and cannot be overridden. | annotation_position: a string containing optionally ["top", "bottom"] | and ["left", "right"] specifying where the text should be anchored | to on the line. Example positions are "bottom left", "right top", | "right", "bottom". If an annotation is added but annotation_position is | not specified, this defaults to "top right". | annotation_*: any parameters to go.layout.Annotation can be passed as | keywords by prefixing them with "annotation_". For example, to specify the | annotation text "example" you can pass annotation_text="example" as a | keyword argument. | **kwargs: | Any named function parameters that can be passed to 'add_shape', | except for x0, x1, y0, y1 or type. | | add_volume(self, autocolorscale=None, caps=None, cauto=None, cmax=None, cmid=None, cmin=None, coloraxis=None, colorbar=None, colorscale=None, contour=None, customdata=None, customdatasrc=None, flatshading=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, isomax=None, isomin=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, lighting=None, lightposition=None, meta=None, metasrc=None, name=None, opacity=None, opacityscale=None, reversescale=None, scene=None, showlegend=None, showscale=None, slices=None, spaceframe=None, stream=None, surface=None, text=None, textsrc=None, uid=None, uirevision=None, value=None, valuehoverformat=None, valuesrc=None, visible=None, x=None, xhoverformat=None, xsrc=None, y=None, yhoverformat=None, ysrc=None, z=None, zhoverformat=None, zsrc=None, row=None, col=None, **kwargs) -> 'FigureWidget' | Add a new Volume trace | | Draws volume trace between iso-min and iso-max values with | coordinates given by four 1-dimensional arrays containing the | `value`, `x`, `y` and `z` of every vertex of a uniform or non- | uniform 3-D grid. Horizontal or vertical slices, caps as well | as spaceframe between iso-min and iso-max values could also be | drawn using this trace. | | Parameters | ---------- | autocolorscale | Determines whether the colorscale is a default palette | (`autocolorscale: true`) or the palette determined by | `colorscale`. In case `colorscale` is unspecified or | `autocolorscale` is true, the default palette will be | chosen according to whether numbers in the `color` | array are all positive, all negative or mixed. | caps | :class:`plotly.graph_objects.volume.Caps` instance or | dict with compatible properties | cauto | Determines whether or not the color domain is computed | with respect to the input data (here `value`) or the | bounds set in `cmin` and `cmax` Defaults to `false` | when `cmin` and `cmax` are set by the user. | cmax | Sets the upper bound of the color domain. Value should | have the same units as `value` and if set, `cmin` must | be set as well. | cmid | Sets the mid-point of the color domain by scaling | `cmin` and/or `cmax` to be equidistant to this point. | Value should have the same units as `value`. Has no | effect when `cauto` is `false`. | cmin | Sets the lower bound of the color domain. Value should | have the same units as `value` and if set, `cmax` must | be set as well. | coloraxis | Sets a reference to a shared color axis. References to | these shared color axes are "coloraxis", "coloraxis2", | "coloraxis3", etc. Settings for these shared color axes | are set in the layout, under `layout.coloraxis`, | `layout.coloraxis2`, etc. Note that multiple color | scales can be linked to the same color axis. | colorbar | :class:`plotly.graph_objects.volume.ColorBar` instance | or dict with compatible properties | colorscale | Sets the colorscale. The colorscale must be an array | containing arrays mapping a normalized value to an rgb, | rgba, hex, hsl, hsv, or named color string. At minimum, | a mapping for the lowest (0) and highest (1) values are | required. For example, `[[0, 'rgb(0,0,255)'], [1, | 'rgb(255,0,0)']]`. To control the bounds of the | colorscale in color space, use `cmin` and `cmax`. | Alternatively, `colorscale` may be a palette name | string of the following list: Blackbody,Bluered,Blues,C | ividis,Earth,Electric,Greens,Greys,Hot,Jet,Picnic,Portl | and,Rainbow,RdBu,Reds,Viridis,YlGnBu,YlOrRd. | contour | :class:`plotly.graph_objects.volume.Contour` instance | or dict with compatible properties | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | flatshading | Determines whether or not normal smoothing is applied | to the meshes, creating meshes with an angular, low- | poly look via flat reflections. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.volume.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Anything contained in tag `<extra>` is | displayed in the secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Same as `text`. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | isomax | Sets the maximum boundary for iso-surface plot. | isomin | Sets the minimum boundary for iso-surface plot. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.volume.Legendgrouptitle` | instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | lighting | :class:`plotly.graph_objects.volume.Lighting` instance | or dict with compatible properties | lightposition | :class:`plotly.graph_objects.volume.Lightposition` | instance or dict with compatible properties | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | opacity | Sets the opacity of the surface. Please note that in | the case of using high `opacity` values for example a | value greater than or equal to 0.5 on two surfaces (and | 0.25 with four surfaces), an overlay of multiple | transparent surfaces may not perfectly be sorted in | depth by the webgl API. This behavior may be improved | in the near future and is subject to change. | opacityscale | Sets the opacityscale. The opacityscale must be an | array containing arrays mapping a normalized value to | an opacity value. At minimum, a mapping for the lowest | (0) and highest (1) values are required. For example, | `[[0, 1], [0.5, 0.2], [1, 1]]` means that higher/lower | values would have higher opacity values and those in | the middle would be more transparent Alternatively, | `opacityscale` may be a palette name string of the | following list: 'min', 'max', 'extremes' and 'uniform'. | The default is 'uniform'. | reversescale | Reverses the color mapping if true. If true, `cmin` | will correspond to the last color in the array and | `cmax` will correspond to the first color. | scene | Sets a reference between this trace's 3D coordinate | system and a 3D scene. If "scene" (the default value), | the (x,y,z) coordinates refer to `layout.scene`. If | "scene2", the (x,y,z) coordinates refer to | `layout.scene2`, and so on. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | showscale | Determines whether or not a colorbar is displayed for | this trace. | slices | :class:`plotly.graph_objects.volume.Slices` instance or | dict with compatible properties | spaceframe | :class:`plotly.graph_objects.volume.Spaceframe` | instance or dict with compatible properties | stream | :class:`plotly.graph_objects.volume.Stream` instance or | dict with compatible properties | surface | :class:`plotly.graph_objects.volume.Surface` instance | or dict with compatible properties | text | Sets the text elements associated with the vertices. If | trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | value | Sets the 4th dimension (value) of the vertices. | valuehoverformat | Sets the hover text formatting rulefor `value` using | d3 formatting mini-languages which are very similar to | those in Python. For numbers, see: https://github.com/d | 3/d3-format/tree/v1.4.5#d3-format.By default the values | are formatted using generic number format. | valuesrc | Sets the source reference on Chart Studio Cloud for | `value`. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | x | Sets the X coordinates of the vertices on X axis. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the Y coordinates of the vertices on Y axis. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | z | Sets the Z coordinates of the vertices on Z axis. | zhoverformat | Sets the hover text formatting rulefor `z` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `zaxis.hoverformat`. | zsrc | Sets the source reference on Chart Studio Cloud for | `z`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | | Returns | ------- | FigureWidget | | add_vrect(self, x0, x1, row='all', col='all', exclude_empty_subplots=True, annotation=None, **kwargs) -> 'FigureWidget' | Add a rectangle to a plot or subplot that extends infinitely in the | y-dimension. | | Parameters | ---------- | x0: float or int | A number representing the x coordinate of one side of the rectangle. | x1: float or int | A number representing the x coordinate of the other side of the rectangle. | exclude_empty_subplots: Boolean | If True (default) do not place the shape on subplots that have no data | plotted on them. | row: None, int or 'all' | Subplot row for shape indexed starting at 1. If 'all', addresses all rows in | the specified column(s). If both row and col are None, addresses the | first subplot if subplots exist, or the only plot. By default is "all". | col: None, int or 'all' | Subplot column for shape indexed starting at 1. If 'all', addresses all rows in | the specified column(s). If both row and col are None, addresses the | first subplot if subplots exist, or the only plot. By default is "all". | annotation: dict or plotly.graph_objects.layout.Annotation. If dict(), | it is interpreted as describing an annotation. The annotation is | placed relative to the shape based on annotation_position (see | below) unless its x or y value has been specified for the annotation | passed here. xref and yref are always the same as for the added | shape and cannot be overridden. | annotation_position: a string containing optionally ["inside", "outside"], ["top", "bottom"] | and ["left", "right"] specifying where the text should be anchored | to on the rectangle. Example positions are "outside top left", "inside | bottom", "right", "inside left", "inside" ("outside" is not supported). If | an annotation is added but annotation_position is not specified this | defaults to "inside top right". | annotation_*: any parameters to go.layout.Annotation can be passed as | keywords by prefixing them with "annotation_". For example, to specify the | annotation text "example" you can pass annotation_text="example" as a | keyword argument. | **kwargs: | Any named function parameters that can be passed to 'add_shape', | except for x0, x1, y0, y1 or type. | | add_waterfall(self, alignmentgroup=None, base=None, cliponaxis=None, connector=None, constraintext=None, customdata=None, customdatasrc=None, decreasing=None, dx=None, dy=None, hoverinfo=None, hoverinfosrc=None, hoverlabel=None, hovertemplate=None, hovertemplatesrc=None, hovertext=None, hovertextsrc=None, ids=None, idssrc=None, increasing=None, insidetextanchor=None, insidetextfont=None, legend=None, legendgroup=None, legendgrouptitle=None, legendrank=None, legendwidth=None, measure=None, measuresrc=None, meta=None, metasrc=None, name=None, offset=None, offsetgroup=None, offsetsrc=None, opacity=None, orientation=None, outsidetextfont=None, selectedpoints=None, showlegend=None, stream=None, text=None, textangle=None, textfont=None, textinfo=None, textposition=None, textpositionsrc=None, textsrc=None, texttemplate=None, texttemplatesrc=None, totals=None, uid=None, uirevision=None, visible=None, width=None, widthsrc=None, x=None, x0=None, xaxis=None, xhoverformat=None, xperiod=None, xperiod0=None, xperiodalignment=None, xsrc=None, y=None, y0=None, yaxis=None, yhoverformat=None, yperiod=None, yperiod0=None, yperiodalignment=None, ysrc=None, zorder=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Add a new Waterfall trace | | Draws waterfall trace which is useful graph to displays the | contribution of various elements (either positive or negative) | in a bar chart. The data visualized by the span of the bars is | set in `y` if `orientation` is set to "v" (the default) and the | labels are set in `x`. By setting `orientation` to "h", the | roles are interchanged. | | Parameters | ---------- | alignmentgroup | Set several traces linked to the same position axis or | matching axes to the same alignmentgroup. This controls | whether bars compute their positional range dependently | or independently. | base | Sets where the bar base is drawn (in position axis | units). | cliponaxis | Determines whether the text nodes are clipped about the | subplot axes. To show the text nodes above axis lines | and tick labels, make sure to set `xaxis.layer` and | `yaxis.layer` to *below traces*. | connector | :class:`plotly.graph_objects.waterfall.Connector` | instance or dict with compatible properties | constraintext | Constrain the size of text inside or outside a bar to | be no larger than the bar itself. | customdata | Assigns extra data each datum. This may be useful when | listening to hover, click and selection events. Note | that, "scatter" traces also appends customdata items in | the markers DOM elements | customdatasrc | Sets the source reference on Chart Studio Cloud for | `customdata`. | decreasing | :class:`plotly.graph_objects.waterfall.Decreasing` | instance or dict with compatible properties | dx | Sets the x coordinate step. See `x0` for more info. | dy | Sets the y coordinate step. See `y0` for more info. | hoverinfo | Determines which trace information appear on hover. If | `none` or `skip` are set, no information is displayed | upon hovering. But, if `none` is set, click and hover | events are still fired. | hoverinfosrc | Sets the source reference on Chart Studio Cloud for | `hoverinfo`. | hoverlabel | :class:`plotly.graph_objects.waterfall.Hoverlabel` | instance or dict with compatible properties | hovertemplate | Template string used for rendering the information that | appear on hover box. Note that this will override | `hoverinfo`. Variables are inserted using %{variable}, | for example "y: %{y}" as well as %{xother}, {%_xother}, | {%_xother_}, {%xother_}. When showing info for several | points, "xother" will be added to those with different | x positions from the first point. An underscore before | or after "(x|y)other" will add a space on that side, | only when this field is shown. Numbers are formatted | using d3-format's syntax %{variable:d3-format}, for | example "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. The variables available in | `hovertemplate` are the ones emitted as event data | described at this link | https://plotly.com/javascript/plotlyjs-events/#event- | data. Additionally, every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `initial`, `delta` and `final`. Anything | contained in tag `<extra>` is displayed in the | secondary box, for example | "<extra>{fullData.name}</extra>". To hide the secondary | box completely, use an empty tag `<extra></extra>`. | hovertemplatesrc | Sets the source reference on Chart Studio Cloud for | `hovertemplate`. | hovertext | Sets hover text elements associated with each (x,y) | pair. If a single string, the same string appears over | all the data points. If an array of string, the items | are mapped in order to the this trace's (x,y) | coordinates. To be seen, trace `hoverinfo` must contain | a "text" flag. | hovertextsrc | Sets the source reference on Chart Studio Cloud for | `hovertext`. | ids | Assigns id labels to each datum. These ids for object | constancy of data points during animation. Should be an | array of strings, not numbers or any other type. | idssrc | Sets the source reference on Chart Studio Cloud for | `ids`. | increasing | :class:`plotly.graph_objects.waterfall.Increasing` | instance or dict with compatible properties | insidetextanchor | Determines if texts are kept at center or start/end | points in `textposition` "inside" mode. | insidetextfont | Sets the font used for `text` lying inside the bar. | legend | Sets the reference to a legend to show this trace in. | References to these legends are "legend", "legend2", | "legend3", etc. Settings for these legends are set in | the layout, under `layout.legend`, `layout.legend2`, | etc. | legendgroup | Sets the legend group for this trace. Traces and shapes | part of the same legend group hide/show at the same | time when toggling legend items. | legendgrouptitle | :class:`plotly.graph_objects.waterfall.Legendgrouptitle | ` instance or dict with compatible properties | legendrank | Sets the legend rank for this trace. Items and groups | with smaller ranks are presented on top/left side while | with "reversed" `legend.traceorder` they are on | bottom/right side. The default legendrank is 1000, so | that you can use ranks less than 1000 to place certain | items before all unranked items, and ranks greater than | 1000 to go after all unranked items. When having | unranked or equal rank items shapes would be displayed | after traces i.e. according to their order in data and | layout. | legendwidth | Sets the width (in px or fraction) of the legend for | this trace. | measure | An array containing types of values. By default the | values are considered as 'relative'. However; it is | possible to use 'total' to compute the sums. Also | 'absolute' could be applied to reset the computed total | or to declare an initial value where needed. | measuresrc | Sets the source reference on Chart Studio Cloud for | `measure`. | meta | Assigns extra meta information associated with this | trace that can be used in various text attributes. | Attributes such as trace `name`, graph, axis and | colorbar `title.text`, annotation `text` | `rangeselector`, `updatemenues` and `sliders` `label` | text all support `meta`. To access the trace `meta` | values in an attribute in the same trace, simply use | `%{meta[i]}` where `i` is the index or key of the | `meta` item in question. To access trace `meta` in | layout attributes, use `%{data[n[.meta[i]}` where `i` | is the index or key of the `meta` and `n` is the trace | index. | metasrc | Sets the source reference on Chart Studio Cloud for | `meta`. | name | Sets the trace name. The trace name appears as the | legend item and on hover. | offset | Shifts the position where the bar is drawn (in position | axis units). In "group" barmode, traces that set | "offset" will be excluded and drawn in "overlay" mode | instead. | offsetgroup | Set several traces linked to the same position axis or | matching axes to the same offsetgroup where bars of the | same position coordinate will line up. | offsetsrc | Sets the source reference on Chart Studio Cloud for | `offset`. | opacity | Sets the opacity of the trace. | orientation | Sets the orientation of the bars. With "v" ("h"), the | value of the each bar spans along the vertical | (horizontal). | outsidetextfont | Sets the font used for `text` lying outside the bar. | selectedpoints | Array containing integer indices of selected points. | Has an effect only for traces that support selections. | Note that an empty array means an empty selection where | the `unselected` are turned on for all points, whereas, | any other non-array values means no selection all where | the `selected` and `unselected` styles have no effect. | showlegend | Determines whether or not an item corresponding to this | trace is shown in the legend. | stream | :class:`plotly.graph_objects.waterfall.Stream` instance | or dict with compatible properties | text | Sets text elements associated with each (x,y) pair. If | a single string, the same string appears over all the | data points. If an array of string, the items are | mapped in order to the this trace's (x,y) coordinates. | If trace `hoverinfo` contains a "text" flag and | "hovertext" is not set, these elements will be seen in | the hover labels. | textangle | Sets the angle of the tick labels with respect to the | bar. For example, a `tickangle` of -90 draws the tick | labels vertically. With "auto" the texts may | automatically be rotated to fit with the maximum size | in bars. | textfont | Sets the font used for `text`. | textinfo | Determines which trace information appear on the graph. | In the case of having multiple waterfalls, totals are | computed separately (per trace). | textposition | Specifies the location of the `text`. "inside" | positions `text` inside, next to the bar end (rotated | and scaled if needed). "outside" positions `text` | outside, next to the bar end (scaled if needed), unless | there is another bar stacked on this one, then the text | gets pushed inside. "auto" tries to position `text` | inside the bar, but if the bar is too small and no bar | is stacked on this one the text is moved outside. If | "none", no text appears. | textpositionsrc | Sets the source reference on Chart Studio Cloud for | `textposition`. | textsrc | Sets the source reference on Chart Studio Cloud for | `text`. | texttemplate | Template string used for rendering the information text | that appear on points. Note that this will override | `textinfo`. Variables are inserted using %{variable}, | for example "y: %{y}". Numbers are formatted using | d3-format's syntax %{variable:d3-format}, for example | "Price: %{y:$.2f}". | https://github.com/d3/d3-format/tree/v1.4.5#d3-format | for details on the formatting syntax. Dates are | formatted using d3-time-format's syntax | %{variable|d3-time-format}, for example "Day: | %{2019-01-01|%A}". https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format for details on the | date formatting syntax. Every attributes that can be | specified per-point (the ones that are `arrayOk: true`) | are available. Finally, the template string has access | to variables `initial`, `delta`, `final` and `label`. | texttemplatesrc | Sets the source reference on Chart Studio Cloud for | `texttemplate`. | totals | :class:`plotly.graph_objects.waterfall.Totals` instance | or dict with compatible properties | uid | Assign an id to this trace, Use this to provide object | constancy between traces during animations and | transitions. | uirevision | Controls persistence of some user-driven changes to the | trace: `constraintrange` in `parcoords` traces, as well | as some `editable: true` modifications such as `name` | and `colorbar.title`. Defaults to `layout.uirevision`. | Note that other user-driven trace attribute changes are | controlled by `layout` attributes: `trace.visible` is | controlled by `layout.legend.uirevision`, | `selectedpoints` is controlled by | `layout.selectionrevision`, and `colorbar.(x|y)` | (accessible with `config: {editable: true}`) is | controlled by `layout.editrevision`. Trace changes are | tracked by `uid`, which only falls back on trace index | if no `uid` is provided. So if your app can add/remove | traces before the end of the `data` array, such that | the same trace has a different index, you can still | preserve user-driven changes if you give each trace a | `uid` that stays with it as it moves. | visible | Determines whether or not this trace is visible. If | "legendonly", the trace is not drawn, but can appear as | a legend item (provided that the legend itself is | visible). | width | Sets the bar width (in position axis units). | widthsrc | Sets the source reference on Chart Studio Cloud for | `width`. | x | Sets the x coordinates. | x0 | Alternate to `x`. Builds a linear space of x | coordinates. Use with `dx` where `x0` is the starting | coordinate and `dx` the step. | xaxis | Sets a reference between this trace's x coordinates and | a 2D cartesian x axis. If "x" (the default value), the | x coordinates refer to `layout.xaxis`. If "x2", the x | coordinates refer to `layout.xaxis2`, and so on. | xhoverformat | Sets the hover text formatting rulefor `x` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `xaxis.hoverformat`. | xperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the x | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | xperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the x0 axis. When `x0period` is round number | of weeks, the `x0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | xperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the x axis. | xsrc | Sets the source reference on Chart Studio Cloud for | `x`. | y | Sets the y coordinates. | y0 | Alternate to `y`. Builds a linear space of y | coordinates. Use with `dy` where `y0` is the starting | coordinate and `dy` the step. | yaxis | Sets a reference between this trace's y coordinates and | a 2D cartesian y axis. If "y" (the default value), the | y coordinates refer to `layout.yaxis`. If "y2", the y | coordinates refer to `layout.yaxis2`, and so on. | yhoverformat | Sets the hover text formatting rulefor `y` using d3 | formatting mini-languages which are very similar to | those in Python. For numbers, see: | https://github.com/d3/d3-format/tree/v1.4.5#d3-format. | And for dates see: https://github.com/d3/d3-time- | format/tree/v2.2.3#locale_format. We add two items to | d3's date formatter: "%h" for half of the year as a | decimal number as well as "%{n}f" for fractional | seconds with n digits. For example, *2016-10-13 | 09:15:23.456* with tickformat "%H~%M~%S.%2f" would | display *09~15~23.46*By default the values are | formatted using `yaxis.hoverformat`. | yperiod | Only relevant when the axis `type` is "date". Sets the | period positioning in milliseconds or "M<n>" on the y | axis. Special values in the form of "M<n>" could be | used to declare the number of months. In this case `n` | must be a positive integer. | yperiod0 | Only relevant when the axis `type` is "date". Sets the | base for period positioning in milliseconds or date | string on the y0 axis. When `y0period` is round number | of weeks, the `y0period0` by default would be on a | Sunday i.e. 2000-01-02, otherwise it would be at | 2000-01-01. | yperiodalignment | Only relevant when the axis `type` is "date". Sets the | alignment of data points on the y axis. | ysrc | Sets the source reference on Chart Studio Cloud for | `y`. | zorder | Sets the layer on which this trace is displayed, | relative to other SVG traces on the same subplot. SVG | traces with higher `zorder` appear in front of those | with lower `zorder`. | row : 'all', int or None (default) | Subplot row index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | rows in the specified column(s). | col : 'all', int or None (default) | Subplot col index (starting from 1) for the trace to be | added. Only valid if figure was created using | `plotly.tools.make_subplots`.If 'all', addresses all | columns in the specified row(s). | secondary_y: boolean or None (default None) | If True, associate this trace with the secondary y-axis of the | subplot at the specified row and col. Only valid if all of the | following conditions are satisfied: | * The figure was created using `plotly.subplots.make_subplots`. | * The row and col arguments are not None | * The subplot at the specified row and col has type xy | (which is the default) and secondary_y True. These | properties are specified in the specs argument to | make_subplots. See the make_subplots docstring for more info. | | Returns | ------- | FigureWidget | | for_each_annotation(self, fn, selector=None, row=None, col=None, secondary_y=None) | Apply a function to all annotations that satisfy the specified selection | criteria | | Parameters | ---------- | fn: | Function that inputs a single annotation object. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all annotations are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each annotation and those for which the function returned True | will be in the selection. If an int N, the Nth annotation matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of annotations to select. | To select annotations by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | annotations that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all annotations are selected. | secondary_y: boolean or None (default None) | * If True, only select annotations associated with the secondary | y-axis of the subplot. | * If False, only select annotations associated with the primary | y-axis of the subplot. | * If None (the default), do not filter annotations based on secondary | y-axis. | | To select annotations by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_coloraxis(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all coloraxis objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single coloraxis object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | coloraxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all coloraxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | coloraxis and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of coloraxis objects to select. | To select coloraxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all coloraxis objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_geo(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all geo objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single geo object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | geo objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all geo objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | geo and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of geo objects to select. | To select geo objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all geo objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_layout_image(self, fn, selector=None, row=None, col=None, secondary_y=None) | Apply a function to all images that satisfy the specified selection | criteria | | Parameters | ---------- | fn: | Function that inputs a single image object. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all images are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each image and those for which the function returned True | will be in the selection. If an int N, the Nth image matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of images to select. | To select images by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | images that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all images are selected. | secondary_y: boolean or None (default None) | * If True, only select images associated with the secondary | y-axis of the subplot. | * If False, only select images associated with the primary | y-axis of the subplot. | * If None (the default), do not filter images based on secondary | y-axis. | | To select images by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_legend(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all legend objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single legend object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | legend objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all legend objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | legend and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of legend objects to select. | To select legend objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all legend objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_map(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all map objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single map object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | map objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all map objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | map and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of map objects to select. | To select map objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all map objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_mapbox(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all mapbox objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single mapbox object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | mapbox objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all mapbox objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | mapbox and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of mapbox objects to select. | To select mapbox objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all mapbox objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_polar(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all polar objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single polar object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | polar objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all polar objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | polar and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of polar objects to select. | To select polar objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all polar objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_scene(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all scene objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single scene object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | scene objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all scene objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | scene and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of scene objects to select. | To select scene objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all scene objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_selection(self, fn, selector=None, row=None, col=None, secondary_y=None) | Apply a function to all selections that satisfy the specified selection | criteria | | Parameters | ---------- | fn: | Function that inputs a single selection object. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all selections are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each selection and those for which the function returned True | will be in the selection. If an int N, the Nth selection matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of selections to select. | To select selections by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | selections that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all selections are selected. | secondary_y: boolean or None (default None) | * If True, only select selections associated with the secondary | y-axis of the subplot. | * If False, only select selections associated with the primary | y-axis of the subplot. | * If None (the default), do not filter selections based on secondary | y-axis. | | To select selections by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_shape(self, fn, selector=None, row=None, col=None, secondary_y=None) | Apply a function to all shapes that satisfy the specified selection | criteria | | Parameters | ---------- | fn: | Function that inputs a single shape object. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all shapes are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each shape and those for which the function returned True | will be in the selection. If an int N, the Nth shape matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of shapes to select. | To select shapes by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | shapes that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all shapes are selected. | secondary_y: boolean or None (default None) | * If True, only select shapes associated with the secondary | y-axis of the subplot. | * If False, only select shapes associated with the primary | y-axis of the subplot. | * If None (the default), do not filter shapes based on secondary | y-axis. | | To select shapes by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_smith(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all smith objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single smith object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | smith objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all smith objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | smith and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of smith objects to select. | To select smith objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all smith objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_ternary(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all ternary objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single ternary object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | ternary objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all ternary objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | ternary and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of ternary objects to select. | To select ternary objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all ternary objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_trace(self, fn, selector=None, row=None, col=None, secondary_y=None) -> 'FigureWidget' | Apply a function to all traces that satisfy the specified selection | criteria | | Parameters | ---------- | fn: | Function that inputs a single trace object. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all traces are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each trace and those for which the function returned True | will be in the selection. If an int N, the Nth trace matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of traces to select. | To select traces by row and column, the Figure must have been | created using plotly.subplots.make_subplots. If None | (the default), all traces are selected. | secondary_y: boolean or None (default None) | * If True, only select traces associated with the secondary | y-axis of the subplot. | * If False, only select traces associated with the primary | y-axis of the subplot. | * If None (the default), do not filter traces based on secondary | y-axis. | | To select traces by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | self | Returns the Figure object that the method was called on | | for_each_xaxis(self, fn, selector=None, row=None, col=None) -> 'FigureWidget' | Apply a function to all xaxis objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single xaxis object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | xaxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all xaxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | xaxis and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of xaxis objects to select. | To select xaxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all xaxis objects are selected. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | for_each_yaxis(self, fn, selector=None, row=None, col=None, secondary_y=None) -> 'FigureWidget' | Apply a function to all yaxis objects that satisfy the | specified selection criteria | | Parameters | ---------- | fn: | Function that inputs a single yaxis object. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | yaxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all yaxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | yaxis and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of yaxis objects to select. | To select yaxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all yaxis objects are selected. | secondary_y: boolean or None (default None) | * If True, only select yaxis objects associated with the secondary | y-axis of the subplot. | * If False, only select yaxis objects associated with the primary | y-axis of the subplot. | * If None (the default), do not filter yaxis objects based on | a secondary y-axis condition. | | To select yaxis objects by secondary y-axis, the Figure must | have been created using plotly.subplots.make_subplots. See | the docstring for the specs argument to make_subplots for more | info on creating subplots with secondary y-axes. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | select_annotations(self, selector=None, row=None, col=None, secondary_y=None) | Select annotations from a particular subplot cell and/or annotations | that satisfy custom selection criteria. | | Parameters | ---------- | selector: dict, function, int, str, or None (default None) | Dict to use as selection criteria. | Annotations will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all annotations are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each annotation and those for which the function returned True | will be in the selection. If an int N, the Nth annotation matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of annotations to select. | To select annotations by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | annotation that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all annotations are selected. | secondary_y: boolean or None (default None) | * If True, only select annotations associated with the secondary | y-axis of the subplot. | * If False, only select annotations associated with the primary | y-axis of the subplot. | * If None (the default), do not filter annotations based on secondary | y-axis. | | To select annotations by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | generator | Generator that iterates through all of the annotations that satisfy | all of the specified selection criteria | | select_coloraxes(self, selector=None, row=None, col=None) | Select coloraxis subplot objects from a particular subplot cell | and/or coloraxis subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | coloraxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all coloraxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | coloraxis and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of coloraxis objects to select. | To select coloraxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all coloraxis objects are selected. | Returns | ------- | generator | Generator that iterates through all of the coloraxis | objects that satisfy all of the specified selection criteria | | select_geos(self, selector=None, row=None, col=None) | Select geo subplot objects from a particular subplot cell | and/or geo subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | geo objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all geo objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | geo and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of geo objects to select. | To select geo objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all geo objects are selected. | Returns | ------- | generator | Generator that iterates through all of the geo | objects that satisfy all of the specified selection criteria | | select_layout_images(self, selector=None, row=None, col=None, secondary_y=None) | Select images from a particular subplot cell and/or images | that satisfy custom selection criteria. | | Parameters | ---------- | selector: dict, function, int, str, or None (default None) | Dict to use as selection criteria. | Annotations will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all images are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each image and those for which the function returned True | will be in the selection. If an int N, the Nth image matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of images to select. | To select images by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | image that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all images are selected. | secondary_y: boolean or None (default None) | * If True, only select images associated with the secondary | y-axis of the subplot. | * If False, only select images associated with the primary | y-axis of the subplot. | * If None (the default), do not filter images based on secondary | y-axis. | | To select images by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | generator | Generator that iterates through all of the images that satisfy | all of the specified selection criteria | | select_legends(self, selector=None, row=None, col=None) | Select legend subplot objects from a particular subplot cell | and/or legend subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | legend objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all legend objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | legend and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of legend objects to select. | To select legend objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all legend objects are selected. | Returns | ------- | generator | Generator that iterates through all of the legend | objects that satisfy all of the specified selection criteria | | select_mapboxes(self, selector=None, row=None, col=None) | Select mapbox subplot objects from a particular subplot cell | and/or mapbox subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | mapbox objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all mapbox objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | mapbox and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of mapbox objects to select. | To select mapbox objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all mapbox objects are selected. | Returns | ------- | generator | Generator that iterates through all of the mapbox | objects that satisfy all of the specified selection criteria | | select_maps(self, selector=None, row=None, col=None) | Select map subplot objects from a particular subplot cell | and/or map subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | map objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all map objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | map and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of map objects to select. | To select map objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all map objects are selected. | Returns | ------- | generator | Generator that iterates through all of the map | objects that satisfy all of the specified selection criteria | | select_polars(self, selector=None, row=None, col=None) | Select polar subplot objects from a particular subplot cell | and/or polar subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | polar objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all polar objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | polar and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of polar objects to select. | To select polar objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all polar objects are selected. | Returns | ------- | generator | Generator that iterates through all of the polar | objects that satisfy all of the specified selection criteria | | select_scenes(self, selector=None, row=None, col=None) | Select scene subplot objects from a particular subplot cell | and/or scene subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | scene objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all scene objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | scene and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of scene objects to select. | To select scene objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all scene objects are selected. | Returns | ------- | generator | Generator that iterates through all of the scene | objects that satisfy all of the specified selection criteria | | select_selections(self, selector=None, row=None, col=None, secondary_y=None) | Select selections from a particular subplot cell and/or selections | that satisfy custom selection criteria. | | Parameters | ---------- | selector: dict, function, int, str, or None (default None) | Dict to use as selection criteria. | Annotations will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all selections are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each selection and those for which the function returned True | will be in the selection. If an int N, the Nth selection matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of selections to select. | To select selections by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | selection that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all selections are selected. | secondary_y: boolean or None (default None) | * If True, only select selections associated with the secondary | y-axis of the subplot. | * If False, only select selections associated with the primary | y-axis of the subplot. | * If None (the default), do not filter selections based on secondary | y-axis. | | To select selections by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | generator | Generator that iterates through all of the selections that satisfy | all of the specified selection criteria | | select_shapes(self, selector=None, row=None, col=None, secondary_y=None) | Select shapes from a particular subplot cell and/or shapes | that satisfy custom selection criteria. | | Parameters | ---------- | selector: dict, function, int, str, or None (default None) | Dict to use as selection criteria. | Annotations will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all shapes are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each shape and those for which the function returned True | will be in the selection. If an int N, the Nth shape matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of shapes to select. | To select shapes by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | shape that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all shapes are selected. | secondary_y: boolean or None (default None) | * If True, only select shapes associated with the secondary | y-axis of the subplot. | * If False, only select shapes associated with the primary | y-axis of the subplot. | * If None (the default), do not filter shapes based on secondary | y-axis. | | To select shapes by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | generator | Generator that iterates through all of the shapes that satisfy | all of the specified selection criteria | | select_smiths(self, selector=None, row=None, col=None) | Select smith subplot objects from a particular subplot cell | and/or smith subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | smith objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all smith objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | smith and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of smith objects to select. | To select smith objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all smith objects are selected. | Returns | ------- | generator | Generator that iterates through all of the smith | objects that satisfy all of the specified selection criteria | | select_ternaries(self, selector=None, row=None, col=None) | Select ternary subplot objects from a particular subplot cell | and/or ternary subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | ternary objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all ternary objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | ternary and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of ternary objects to select. | To select ternary objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all ternary objects are selected. | Returns | ------- | generator | Generator that iterates through all of the ternary | objects that satisfy all of the specified selection criteria | | select_xaxes(self, selector=None, row=None, col=None) | Select xaxis subplot objects from a particular subplot cell | and/or xaxis subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | xaxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all xaxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | xaxis and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of xaxis objects to select. | To select xaxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all xaxis objects are selected. | Returns | ------- | generator | Generator that iterates through all of the xaxis | objects that satisfy all of the specified selection criteria | | select_yaxes(self, selector=None, row=None, col=None, secondary_y=None) | Select yaxis subplot objects from a particular subplot cell | and/or yaxis subplot objects that satisfy custom selection | criteria. | | Parameters | ---------- | selector: dict, function, or None (default None) | Dict to use as selection criteria. | yaxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all yaxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | yaxis and those for which the function returned True will | be in the selection. | row, col: int or None (default None) | Subplot row and column index of yaxis objects to select. | To select yaxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all yaxis objects are selected. | secondary_y: boolean or None (default None) | * If True, only select yaxis objects associated with the secondary | y-axis of the subplot. | * If False, only select yaxis objects associated with the primary | y-axis of the subplot. | * If None (the default), do not filter yaxis objects based on | a secondary y-axis condition. | | To select yaxis objects by secondary y-axis, the Figure must | have been created using plotly.subplots.make_subplots. See | the docstring for the specs argument to make_subplots for more | info on creating subplots with secondary y-axes. | Returns | ------- | generator | Generator that iterates through all of the yaxis | objects that satisfy all of the specified selection criteria | | set_subplots(self, rows=None, cols=None, **make_subplots_args) -> 'FigureWidget' | Add subplots to this figure. If the figure already contains subplots, | then this throws an error. Accepts any keyword arguments that | plotly.subplots.make_subplots accepts. | | update(self, dict1=None, overwrite=False, **kwargs) -> 'FigureWidget' | Update the properties of the figure with a dict and/or with | keyword arguments. | | This recursively updates the structure of the figure | object with the values in the input dict / keyword arguments. | | Parameters | ---------- | dict1 : dict | Dictionary of properties to be updated | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | kwargs : | Keyword/value pair of properties to be updated | | Examples | -------- | >>> import plotly.graph_objs as go | >>> fig = go.Figure(data=[{'y': [1, 2, 3]}]) | >>> fig.update(data=[{'y': [4, 5, 6]}]) # doctest: +ELLIPSIS | Figure(...) | >>> fig.to_plotly_json() # doctest: +SKIP | {'data': [{'type': 'scatter', | 'uid': 'e86a7c7a-346a-11e8-8aa8-a0999b0c017b', | 'y': array([4, 5, 6], dtype=int32)}], | 'layout': {}} | | >>> fig = go.Figure(layout={'xaxis': | ... {'color': 'green', | ... 'range': [0, 1]}}) | >>> fig.update({'layout': {'xaxis': {'color': 'pink'}}}) # doctest: +ELLIPSIS | Figure(...) | >>> fig.to_plotly_json() # doctest: +SKIP | {'data': [], | 'layout': {'xaxis': | {'color': 'pink', | 'range': [0, 1]}}} | | Returns | ------- | BaseFigure | Updated figure | | update_annotations(self, patch=None, selector=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all annotations that satisfy the | specified selection criteria | | Parameters | ---------- | patch: dict or None (default None) | Dictionary of property updates to be applied to all annotations that | satisfy the selection criteria. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all annotations are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each annotation and those for which the function returned True | will be in the selection. If an int N, the Nth annotation matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of annotations to select. | To select annotations by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | annotation that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all annotations are selected. | secondary_y: boolean or None (default None) | * If True, only select annotations associated with the secondary | y-axis of the subplot. | * If False, only select annotations associated with the primary | y-axis of the subplot. | * If None (the default), do not filter annotations based on secondary | y-axis. | | To select annotations by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | **kwargs | Additional property updates to apply to each selected annotation. If | a property is specified in both patch and in **kwargs then the | one in **kwargs takes precedence. | | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_coloraxes(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all coloraxis objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | coloraxis objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | coloraxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all coloraxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | coloraxis and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of coloraxis objects to select. | To select coloraxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all coloraxis objects are selected. | **kwargs | Additional property updates to apply to each selected | coloraxis object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_geos(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all geo objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | geo objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | geo objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all geo objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | geo and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of geo objects to select. | To select geo objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all geo objects are selected. | **kwargs | Additional property updates to apply to each selected | geo object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_layout(self, dict1=None, overwrite=False, **kwargs) -> 'FigureWidget' | Update the properties of the figure's layout with a dict and/or with | keyword arguments. | | This recursively updates the structure of the original | layout with the values in the input dict / keyword arguments. | | Parameters | ---------- | dict1 : dict | Dictionary of properties to be updated | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | kwargs : | Keyword/value pair of properties to be updated | | Returns | ------- | BaseFigure | The Figure object that the update_layout method was called on | | update_layout_images(self, patch=None, selector=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all images that satisfy the | specified selection criteria | | Parameters | ---------- | patch: dict or None (default None) | Dictionary of property updates to be applied to all images that | satisfy the selection criteria. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all images are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each image and those for which the function returned True | will be in the selection. If an int N, the Nth image matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of images to select. | To select images by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | image that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all images are selected. | secondary_y: boolean or None (default None) | * If True, only select images associated with the secondary | y-axis of the subplot. | * If False, only select images associated with the primary | y-axis of the subplot. | * If None (the default), do not filter images based on secondary | y-axis. | | To select images by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | **kwargs | Additional property updates to apply to each selected image. If | a property is specified in both patch and in **kwargs then the | one in **kwargs takes precedence. | | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_legends(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all legend objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | legend objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | legend objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all legend objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | legend and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of legend objects to select. | To select legend objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all legend objects are selected. | **kwargs | Additional property updates to apply to each selected | legend object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_mapboxes(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all mapbox objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | mapbox objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | mapbox objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all mapbox objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | mapbox and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of mapbox objects to select. | To select mapbox objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all mapbox objects are selected. | **kwargs | Additional property updates to apply to each selected | mapbox object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_maps(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all map objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | map objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | map objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all map objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | map and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of map objects to select. | To select map objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all map objects are selected. | **kwargs | Additional property updates to apply to each selected | map object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_polars(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all polar objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | polar objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | polar objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all polar objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | polar and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of polar objects to select. | To select polar objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all polar objects are selected. | **kwargs | Additional property updates to apply to each selected | polar object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_scenes(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all scene objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | scene objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | scene objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all scene objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | scene and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of scene objects to select. | To select scene objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all scene objects are selected. | **kwargs | Additional property updates to apply to each selected | scene object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_selections(self, patch=None, selector=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all selections that satisfy the | specified selection criteria | | Parameters | ---------- | patch: dict or None (default None) | Dictionary of property updates to be applied to all selections that | satisfy the selection criteria. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all selections are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each selection and those for which the function returned True | will be in the selection. If an int N, the Nth selection matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of selections to select. | To select selections by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | selection that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all selections are selected. | secondary_y: boolean or None (default None) | * If True, only select selections associated with the secondary | y-axis of the subplot. | * If False, only select selections associated with the primary | y-axis of the subplot. | * If None (the default), do not filter selections based on secondary | y-axis. | | To select selections by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | **kwargs | Additional property updates to apply to each selected selection. If | a property is specified in both patch and in **kwargs then the | one in **kwargs takes precedence. | | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_shapes(self, patch=None, selector=None, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all shapes that satisfy the | specified selection criteria | | Parameters | ---------- | patch: dict or None (default None) | Dictionary of property updates to be applied to all shapes that | satisfy the selection criteria. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all shapes are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each shape and those for which the function returned True | will be in the selection. If an int N, the Nth shape matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of shapes to select. | To select shapes by row and column, the Figure must have been | created using plotly.subplots.make_subplots. To select only those | shape that are in paper coordinates, set row and col to the | string 'paper'. If None (the default), all shapes are selected. | secondary_y: boolean or None (default None) | * If True, only select shapes associated with the secondary | y-axis of the subplot. | * If False, only select shapes associated with the primary | y-axis of the subplot. | * If None (the default), do not filter shapes based on secondary | y-axis. | | To select shapes by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | **kwargs | Additional property updates to apply to each selected shape. If | a property is specified in both patch and in **kwargs then the | one in **kwargs takes precedence. | | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_smiths(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all smith objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | smith objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | smith objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all smith objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | smith and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of smith objects to select. | To select smith objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all smith objects are selected. | **kwargs | Additional property updates to apply to each selected | smith object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_ternaries(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all ternary objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | ternary objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | ternary objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all ternary objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | ternary and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of ternary objects to select. | To select ternary objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all ternary objects are selected. | **kwargs | Additional property updates to apply to each selected | ternary object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_traces(self, patch=None, selector=None, row=None, col=None, secondary_y=None, overwrite=False, **kwargs) -> 'FigureWidget' | Perform a property update operation on all traces that satisfy the | specified selection criteria | | Parameters | ---------- | patch: dict or None (default None) | Dictionary of property updates to be applied to all traces that | satisfy the selection criteria. | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all traces are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each trace and those for which the function returned True | will be in the selection. If an int N, the Nth trace matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of traces to select. | To select traces by row and column, the Figure must have been | created using plotly.subplots.make_subplots. If None | (the default), all traces are selected. | secondary_y: boolean or None (default None) | * If True, only select traces associated with the secondary | y-axis of the subplot. | * If False, only select traces associated with the primary | y-axis of the subplot. | * If None (the default), do not filter traces based on secondary | y-axis. | | To select traces by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | **kwargs | Additional property updates to apply to each selected trace. If | a property is specified in both patch and in **kwargs then the | one in **kwargs takes precedence. | | Returns | ------- | self | Returns the Figure object that the method was called on | | update_xaxes(self, patch=None, selector=None, overwrite=False, row=None, col=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all xaxis objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | xaxis objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | xaxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all xaxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | xaxis and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of xaxis objects to select. | To select xaxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all xaxis objects are selected. | **kwargs | Additional property updates to apply to each selected | xaxis object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | update_yaxes(self, patch=None, selector=None, overwrite=False, row=None, col=None, secondary_y=None, **kwargs) -> 'FigureWidget' | Perform a property update operation on all yaxis objects | that satisfy the specified selection criteria | | Parameters | ---------- | patch: dict | Dictionary of property updates to be applied to all | yaxis objects that satisfy the selection criteria. | selector: dict, function, or None (default None) | Dict to use as selection criteria. | yaxis objects will be selected if they contain | properties corresponding to all of the dictionary's keys, with | values that exactly match the supplied values. If None | (the default), all yaxis objects are selected. If a | function, it must be a function accepting a single argument and | returning a boolean. The function will be called on each | yaxis and those for which the function returned True will | be in the selection. | overwrite: bool | If True, overwrite existing properties. If False, apply updates | to existing properties recursively, preserving existing | properties that are not specified in the update operation. | row, col: int or None (default None) | Subplot row and column index of yaxis objects to select. | To select yaxis objects by row and column, the Figure | must have been created using plotly.subplots.make_subplots. | If None (the default), all yaxis objects are selected. | secondary_y: boolean or None (default None) | * If True, only select yaxis objects associated with the secondary | y-axis of the subplot. | * If False, only select yaxis objects associated with the primary | y-axis of the subplot. | * If None (the default), do not filter yaxis objects based on | a secondary y-axis condition. | | To select yaxis objects by secondary y-axis, the Figure must | have been created using plotly.subplots.make_subplots. See | the docstring for the specs argument to make_subplots for more | info on creating subplots with secondary y-axes. | **kwargs | Additional property updates to apply to each selected | yaxis object. If a property is specified in | both patch and in **kwargs then the one in **kwargs | takes precedence. | Returns | ------- | self | Returns the FigureWidget object that the method was called on | | ---------------------------------------------------------------------- | Methods inherited from plotly.basewidget.BaseFigureWidget: | | on_edits_completed(self, fn) | Register a function to be called after all pending trace and layout | edit operations have completed | | If there are no pending edit operations then function is called | immediately | | Parameters | ---------- | fn : callable | Function of zero arguments to be called when all pending edit | operations have completed | | ---------------------------------------------------------------------- | Data descriptors inherited from plotly.basewidget.BaseFigureWidget: | | frames | The `frames` property is a tuple of the figure's frame objects | | Returns | ------- | tuple[plotly.graph_objs.Frame] | | ---------------------------------------------------------------------- | Methods inherited from plotly.basedatatypes.BaseFigure: | | __contains__(self, prop) | | __eq__(self, other) | Return self==value. | | __getitem__(self, prop) | | __iter__(self) | | __reduce__(self) | Custom implementation of reduce is used to support deep copying | and pickling | | __repr__(self) | Customize Figure representation when displayed in the | terminal/notebook | | __setattr__(self, prop, value) | Parameters | ---------- | prop : str | The name of a direct child of this object | value | New property value | Returns | ------- | None | | __setitem__(self, prop, value) | | append_trace(self, trace, row, col) | Add a trace to the figure bound to axes at the specified row, | col index. | | A row, col index grid is generated for figures created with | plotly.tools.make_subplots, and can be viewed with the `print_grid` | method | | Parameters | ---------- | trace | The data trace to be bound | row: int | Subplot row index (see Figure.print_grid) | col: int | Subplot column index (see Figure.print_grid) | | Examples | -------- | | >>> from plotly import tools | >>> import plotly.graph_objs as go | >>> # stack two subplots vertically | >>> fig = tools.make_subplots(rows=2) | | This is the format of your plot grid: | [ (1,1) x1,y1 ] | [ (2,1) x2,y2 ] | | >>> fig.append_trace(go.Scatter(x=[1,2,3], y=[2,1,2]), row=1, col=1) | >>> fig.append_trace(go.Scatter(x=[1,2,3], y=[2,1,2]), row=2, col=1) | | batch_animate(self, duration=500, easing='cubic-in-out') | Context manager to animate trace / layout updates | | Parameters | ---------- | duration : number | The duration of the transition, in milliseconds. | If equal to zero, updates are synchronous. | easing : string | The easing function used for the transition. | One of: | - linear | - quad | - cubic | - sin | - exp | - circle | - elastic | - back | - bounce | - linear-in | - quad-in | - cubic-in | - sin-in | - exp-in | - circle-in | - elastic-in | - back-in | - bounce-in | - linear-out | - quad-out | - cubic-out | - sin-out | - exp-out | - circle-out | - elastic-out | - back-out | - bounce-out | - linear-in-out | - quad-in-out | - cubic-in-out | - sin-in-out | - exp-in-out | - circle-in-out | - elastic-in-out | - back-in-out | - bounce-in-out | | Examples | -------- | Suppose we have a figure widget, `fig`, with a single trace. | | >>> import plotly.graph_objs as go | >>> fig = go.FigureWidget(data=[{'y': [3, 4, 2]}]) | | 1) Animate a change in the xaxis and yaxis ranges using default | duration and easing parameters. | | >>> with fig.batch_animate(): | ... fig.layout.xaxis.range = [0, 5] | ... fig.layout.yaxis.range = [0, 10] | | 2) Animate a change in the size and color of the trace's markers | over 2 seconds using the elastic-in-out easing method | | >>> with fig.batch_animate(duration=2000, easing='elastic-in-out'): | ... fig.data[0].marker.color = 'green' | ... fig.data[0].marker.size = 20 | | batch_update(self) | A context manager that batches up trace and layout assignment | operations into a singe plotly_update message that is executed when | the context exits. | | Examples | -------- | For example, suppose we have a figure widget, `fig`, with a single | trace. | | >>> import plotly.graph_objs as go | >>> fig = go.FigureWidget(data=[{'y': [3, 4, 2]}]) | | If we want to update the xaxis range, the yaxis range, and the | marker color, we could do so using a series of three property | assignments as follows: | | >>> fig.layout.xaxis.range = [0, 5] | >>> fig.layout.yaxis.range = [0, 10] | >>> fig.data[0].marker.color = 'green' | | This will work, however it will result in three messages being | sent to the front end (two relayout messages for the axis range | updates followed by one restyle message for the marker color | update). This can cause the plot to appear to stutter as the | three updates are applied incrementally. | | We can avoid this problem by performing these three assignments in a | `batch_update` context as follows: | | >>> with fig.batch_update(): | ... fig.layout.xaxis.range = [0, 5] | ... fig.layout.yaxis.range = [0, 10] | ... fig.data[0].marker.color = 'green' | | Now, these three property updates will be sent to the frontend in a | single update message, and they will be applied by the front end | simultaneously. | | full_figure_for_development(self, warn=True, as_dict=False) | Compute default values for all attributes not specified in the input figure and | returns the output as a "full" figure. This function calls Plotly.js via Kaleido | to populate unspecified attributes. This function is intended for interactive use | during development to learn more about how Plotly.js computes default values and is | not generally necessary or recommended for production use. | | Parameters | ---------- | fig: | Figure object or dict representing a figure | | warn: bool | If False, suppress warnings about not using this in production. | | as_dict: bool | If True, output is a dict with some keys that go.Figure can't parse. | If False, output is a go.Figure with unparseable keys skipped. | | Returns | ------- | plotly.graph_objects.Figure or dict | The full figure | | get_subplot(self, row, col, secondary_y=False) | Return an object representing the subplot at the specified row | and column. May only be used on Figures created using | plotly.tools.make_subplots | | Parameters | ---------- | row: int | 1-based index of subplot row | col: int | 1-based index of subplot column | secondary_y: bool | If True, select the subplot that consists of the x-axis and the | secondary y-axis at the specified row/col. Only valid if the | subplot at row/col is an 2D cartesian subplot that was created | with a secondary y-axis. See the docstring for the specs argument | to make_subplots for more info on creating a subplot with a | secondary y-axis. | Returns | ------- | subplot | * None: if subplot is empty | * plotly.graph_objs.layout.Scene: if subplot type is 'scene' | * plotly.graph_objs.layout.Polar: if subplot type is 'polar' | * plotly.graph_objs.layout.Ternary: if subplot type is 'ternary' | * plotly.graph_objs.layout.Mapbox: if subplot type is 'ternary' | * SubplotDomain namedtuple with `x` and `y` fields: | if subplot type is 'domain'. | - x: length 2 list of the subplot start and stop width | - y: length 2 list of the subplot start and stop height | * SubplotXY namedtuple with `xaxis` and `yaxis` fields: | if subplot type is 'xy'. | - xaxis: plotly.graph_objs.layout.XAxis instance for subplot | - yaxis: plotly.graph_objs.layout.YAxis instance for subplot | | plotly_relayout(self, relayout_data, **kwargs) | Perform a Plotly relayout operation on the figure's layout | | Parameters | ---------- | relayout_data : dict | Dict of layout updates | | dict keys are strings that specify the properties to be updated. | Nested properties are expressed by joining successive keys on | '.' characters (e.g. 'xaxis.range') | | dict values are the values to use to update the layout. | | Returns | ------- | None | | plotly_restyle(self, restyle_data, trace_indexes=None, **kwargs) | Perform a Plotly restyle operation on the figure's traces | | Parameters | ---------- | restyle_data : dict | Dict of trace style updates. | | Keys are strings that specify the properties to be updated. | Nested properties are expressed by joining successive keys on | '.' characters (e.g. 'marker.color'). | | Values may be scalars or lists. When values are scalars, | that scalar value is applied to all traces specified by the | `trace_indexes` parameter. When values are lists, | the restyle operation will cycle through the elements | of the list as it cycles through the traces specified by the | `trace_indexes` parameter. | | Caution: To use plotly_restyle to update a list property (e.g. | the `x` property of the scatter trace), the property value | should be a scalar list containing the list to update with. For | example, the following command would be used to update the 'x' | property of the first trace to the list [1, 2, 3] | | >>> import plotly.graph_objects as go | >>> fig = go.Figure(go.Scatter(x=[2, 4, 6])) | >>> fig.plotly_restyle({'x': [[1, 2, 3]]}, 0) | | trace_indexes : int or list of int | Trace index, or list of trace indexes, that the restyle operation | applies to. Defaults to all trace indexes. | | Returns | ------- | None | | plotly_update(self, restyle_data=None, relayout_data=None, trace_indexes=None, **kwargs) | Perform a Plotly update operation on the figure. | | Note: This operation both mutates and returns the figure | | Parameters | ---------- | restyle_data : dict | Traces update specification. See the docstring for the | `plotly_restyle` method for details | relayout_data : dict | Layout update specification. See the docstring for the | `plotly_relayout` method for details | trace_indexes : | Trace index, or list of trace indexes, that the update operation | applies to. Defaults to all trace indexes. | | Returns | ------- | BaseFigure | None | | pop(self, key, *args) | Remove the value associated with the specified key and return it | | Parameters | ---------- | key: str | Property name | dflt | The default value to return if key was not found in figure | | Returns | ------- | value | The removed value that was previously associated with key | | Raises | ------ | KeyError | If key is not in object and no dflt argument specified | | print_grid(self) | Print a visual layout of the figure's axes arrangement. | This is only valid for figures that are created | with plotly.tools.make_subplots. | | select_traces(self, selector=None, row=None, col=None, secondary_y=None) | Select traces from a particular subplot cell and/or traces | that satisfy custom selection criteria. | | Parameters | ---------- | selector: dict, function, int, str or None (default None) | Dict to use as selection criteria. | Traces will be selected if they contain properties corresponding | to all of the dictionary's keys, with values that exactly match | the supplied values. If None (the default), all traces are | selected. If a function, it must be a function accepting a single | argument and returning a boolean. The function will be called on | each trace and those for which the function returned True | will be in the selection. If an int N, the Nth trace matching row | and col will be selected (N can be negative). If a string S, the selector | is equivalent to dict(type=S). | row, col: int or None (default None) | Subplot row and column index of traces to select. | To select traces by row and column, the Figure must have been | created using plotly.subplots.make_subplots. If None | (the default), all traces are selected. | secondary_y: boolean or None (default None) | * If True, only select traces associated with the secondary | y-axis of the subplot. | * If False, only select traces associated with the primary | y-axis of the subplot. | * If None (the default), do not filter traces based on secondary | y-axis. | | To select traces by secondary y-axis, the Figure must have been | created using plotly.subplots.make_subplots. See the docstring | for the specs argument to make_subplots for more info on | creating subplots with secondary y-axes. | Returns | ------- | generator | Generator that iterates through all of the traces that satisfy | all of the specified selection criteria | | show(self, *args, **kwargs) | Show a figure using either the default renderer(s) or the renderer(s) | specified by the renderer argument | | Parameters | ---------- | renderer: str or None (default None) | A string containing the names of one or more registered renderers | (separated by '+' characters) or None. If None, then the default | renderers specified in plotly.io.renderers.default are used. | | validate: bool (default True) | True if the figure should be validated before being shown, | False otherwise. | | width: int or float | An integer or float that determines the number of pixels wide the | plot is. The default is set in plotly.js. | | height: int or float | An integer or float that determines the number of pixels wide the | plot is. The default is set in plotly.js. | | config: dict | A dict of parameters to configure the figure. The defaults are set | in plotly.js. | | Returns | ------- | None | | to_dict(self) | Convert figure to a dictionary | | Note: the dictionary includes the properties explicitly set by the | user, it does not include default values of unspecified properties | | Returns | ------- | dict | | to_html(self, *args, **kwargs) | Convert a figure to an HTML string representation. | | Parameters | ---------- | config: dict or None (default None) | Plotly.js figure config options | auto_play: bool (default=True) | Whether to automatically start the animation sequence on page load | if the figure contains frames. Has no effect if the figure does not | contain frames. | include_plotlyjs: bool or string (default True) | Specifies how the plotly.js library is included/loaded in the output | div string. | | If True, a script tag containing the plotly.js source code (~3MB) | is included in the output. HTML files generated with this option are | fully self-contained and can be used offline. | | If 'cdn', a script tag that references the plotly.js CDN is included | in the output. HTML files generated with this option are about 3MB | smaller than those generated with include_plotlyjs=True, but they | require an active internet connection in order to load the plotly.js | library. | | If 'directory', a script tag is included that references an external | plotly.min.js bundle that is assumed to reside in the same | directory as the HTML file. | | If 'require', Plotly.js is loaded using require.js. This option | assumes that require.js is globally available and that it has been | globally configured to know how to find Plotly.js as 'plotly'. | This option is not advised when full_html=True as it will result | in a non-functional html file. | | If a string that ends in '.js', a script tag is included that | references the specified path. This approach can be used to point | the resulting HTML file to an alternative CDN or local bundle. | | If False, no script tag referencing plotly.js is included. This is | useful when the resulting div string will be placed inside an HTML | document that already loads plotly.js. This option is not advised | when full_html=True as it will result in a non-functional html file. | include_mathjax: bool or string (default False) | Specifies how the MathJax.js library is included in the output html | div string. MathJax is required in order to display labels | with LaTeX typesetting. | | If False, no script tag referencing MathJax.js will be included in the | output. | | If 'cdn', a script tag that references a MathJax CDN location will be | included in the output. HTML div strings generated with this option | will be able to display LaTeX typesetting as long as internet access | is available. | | If a string that ends in '.js', a script tag is included that | references the specified path. This approach can be used to point the | resulting HTML div string to an alternative CDN. | post_script: str or list or None (default None) | JavaScript snippet(s) to be included in the resulting div just after | plot creation. The string(s) may include '{plot_id}' placeholders | that will then be replaced by the `id` of the div element that the | plotly.js figure is associated with. One application for this script | is to install custom plotly.js event handlers. | full_html: bool (default True) | If True, produce a string containing a complete HTML document | starting with an <html> tag. If False, produce a string containing | a single <div> element. | animation_opts: dict or None (default None) | dict of custom animation parameters to be passed to the function | Plotly.animate in Plotly.js. See | https://github.com/plotly/plotly.js/blob/master/src/plots/animation_attributes.js | for available options. Has no effect if the figure does not contain | frames, or auto_play is False. | default_width, default_height: number or str (default '100%') | The default figure width/height to use if the provided figure does not | specify its own layout.width/layout.height property. May be | specified in pixels as an integer (e.g. 500), or as a css width style | string (e.g. '500px', '100%'). | validate: bool (default True) | True if the figure should be validated before being converted to | JSON, False otherwise. | div_id: str (default None) | If provided, this is the value of the id attribute of the div tag. If None, the | id attribute is a UUID. | | Returns | ------- | str | Representation of figure as an HTML div string | | to_image(self, *args, **kwargs) | Convert a figure to a static image bytes string | | Parameters | ---------- | format: str or None | The desired image format. One of | - 'png' | - 'jpg' or 'jpeg' | - 'webp' | - 'svg' | - 'pdf' | - 'eps' (Requires the poppler library to be installed) | | If not specified, will default to `plotly.io.config.default_format` | | width: int or None | The width of the exported image in layout pixels. If the `scale` | property is 1.0, this will also be the width of the exported image | in physical pixels. | | If not specified, will default to `plotly.io.config.default_width` | | height: int or None | The height of the exported image in layout pixels. If the `scale` | property is 1.0, this will also be the height of the exported image | in physical pixels. | | If not specified, will default to `plotly.io.config.default_height` | | scale: int or float or None | The scale factor to use when exporting the figure. A scale factor | larger than 1.0 will increase the image resolution with respect | to the figure's layout pixel dimensions. Whereas as scale factor of | less than 1.0 will decrease the image resolution. | | If not specified, will default to `plotly.io.config.default_scale` | | validate: bool | True if the figure should be validated before being converted to | an image, False otherwise. | | engine: str | Image export engine to use: | - "kaleido": Use Kaleido for image export | - "orca": Use Orca for image export | - "auto" (default): Use Kaleido if installed, otherwise use orca | | Returns | ------- | bytes | The image data | | to_json(self, *args, **kwargs) | Convert a figure to a JSON string representation | | Parameters | ---------- | validate: bool (default True) | True if the figure should be validated before being converted to | JSON, False otherwise. | | pretty: bool (default False) | True if JSON representation should be pretty-printed, False if | representation should be as compact as possible. | | remove_uids: bool (default True) | True if trace UIDs should be omitted from the JSON representation | | engine: str (default None) | The JSON encoding engine to use. One of: | - "json" for an encoder based on the built-in Python json module | - "orjson" for a fast encoder the requires the orjson package | If not specified, the default encoder is set to the current value of | plotly.io.json.config.default_encoder. | | Returns | ------- | str | Representation of figure as a JSON string | | to_ordered_dict(self, skip_uid=True) | | to_plotly_json(self) | Convert figure to a JSON representation as a Python dict | | Note: May include some JSON-invalid data types, use the `PlotlyJSONEncoder` util | or the `to_json` method to encode to a string. | | Returns | ------- | dict | | write_html(self, *args, **kwargs) | Write a figure to an HTML file representation | | Parameters | ---------- | file: str or writeable | A string representing a local file path or a writeable object | (e.g. a pathlib.Path object or an open file descriptor) | config: dict or None (default None) | Plotly.js figure config options | auto_play: bool (default=True) | Whether to automatically start the animation sequence on page load | if the figure contains frames. Has no effect if the figure does not | contain frames. | include_plotlyjs: bool or string (default True) | Specifies how the plotly.js library is included/loaded in the output | div string. | | If True, a script tag containing the plotly.js source code (~3MB) | is included in the output. HTML files generated with this option are | fully self-contained and can be used offline. | | If 'cdn', a script tag that references the plotly.js CDN is included | in the output. HTML files generated with this option are about 3MB | smaller than those generated with include_plotlyjs=True, but they | require an active internet connection in order to load the plotly.js | library. | | If 'directory', a script tag is included that references an external | plotly.min.js bundle that is assumed to reside in the same | directory as the HTML file. If `file` is a string to a local file | path and `full_html` is True, then the plotly.min.js bundle is copied | into the directory of the resulting HTML file. If a file named | plotly.min.js already exists in the output directory then this file | is left unmodified and no copy is performed. HTML files generated | with this option can be used offline, but they require a copy of | the plotly.min.js bundle in the same directory. This option is | useful when many figures will be saved as HTML files in the same | directory because the plotly.js source code will be included only | once per output directory, rather than once per output file. | | If 'require', Plotly.js is loaded using require.js. This option | assumes that require.js is globally available and that it has been | globally configured to know how to find Plotly.js as 'plotly'. | This option is not advised when full_html=True as it will result | in a non-functional html file. | | If a string that ends in '.js', a script tag is included that | references the specified path. This approach can be used to point | the resulting HTML file to an alternative CDN or local bundle. | | If False, no script tag referencing plotly.js is included. This is | useful when the resulting div string will be placed inside an HTML | document that already loads plotly.js. This option is not advised | when full_html=True as it will result in a non-functional html file. | | include_mathjax: bool or string (default False) | Specifies how the MathJax.js library is included in the output html | div string. MathJax is required in order to display labels | with LaTeX typesetting. | | If False, no script tag referencing MathJax.js will be included in the | output. | | If 'cdn', a script tag that references a MathJax CDN location will be | included in the output. HTML div strings generated with this option | will be able to display LaTeX typesetting as long as internet access | is available. | | If a string that ends in '.js', a script tag is included that | references the specified path. This approach can be used to point the | resulting HTML div string to an alternative CDN. | post_script: str or list or None (default None) | JavaScript snippet(s) to be included in the resulting div just after | plot creation. The string(s) may include '{plot_id}' placeholders | that will then be replaced by the `id` of the div element that the | plotly.js figure is associated with. One application for this script | is to install custom plotly.js event handlers. | full_html: bool (default True) | If True, produce a string containing a complete HTML document | starting with an <html> tag. If False, produce a string containing | a single <div> element. | animation_opts: dict or None (default None) | dict of custom animation parameters to be passed to the function | Plotly.animate in Plotly.js. See | https://github.com/plotly/plotly.js/blob/master/src/plots/animation_attributes.js | for available options. Has no effect if the figure does not contain | frames, or auto_play is False. | default_width, default_height: number or str (default '100%') | The default figure width/height to use if the provided figure does not | specify its own layout.width/layout.height property. May be | specified in pixels as an integer (e.g. 500), or as a css width style | string (e.g. '500px', '100%'). | validate: bool (default True) | True if the figure should be validated before being converted to | JSON, False otherwise. | auto_open: bool (default True) | If True, open the saved file in a web browser after saving. | This argument only applies if `full_html` is True. | div_id: str (default None) | If provided, this is the value of the id attribute of the div tag. If None, the | id attribute is a UUID. | | Returns | ------- | None | | write_image(self, *args, **kwargs) | Convert a figure to a static image and write it to a file or writeable | object | | Parameters | ---------- | file: str or writeable | A string representing a local file path or a writeable object | (e.g. a pathlib.Path object or an open file descriptor) | | format: str or None | The desired image format. One of | - 'png' | - 'jpg' or 'jpeg' | - 'webp' | - 'svg' | - 'pdf' | - 'eps' (Requires the poppler library to be installed) | | If not specified and `file` is a string then this will default to the | file extension. If not specified and `file` is not a string then this | will default to `plotly.io.config.default_format` | | width: int or None | The width of the exported image in layout pixels. If the `scale` | property is 1.0, this will also be the width of the exported image | in physical pixels. | | If not specified, will default to `plotly.io.config.default_width` | | height: int or None | The height of the exported image in layout pixels. If the `scale` | property is 1.0, this will also be the height of the exported image | in physical pixels. | | If not specified, will default to `plotly.io.config.default_height` | | scale: int or float or None | The scale factor to use when exporting the figure. A scale factor | larger than 1.0 will increase the image resolution with respect | to the figure's layout pixel dimensions. Whereas as scale factor of | less than 1.0 will decrease the image resolution. | | If not specified, will default to `plotly.io.config.default_scale` | | validate: bool | True if the figure should be validated before being converted to | an image, False otherwise. | | engine: str | Image export engine to use: | - "kaleido": Use Kaleido for image export | - "orca": Use Orca for image export | - "auto" (default): Use Kaleido if installed, otherwise use orca | Returns | ------- | None | | write_json(self, *args, **kwargs) | Convert a figure to JSON and write it to a file or writeable | object | | Parameters | ---------- | file: str or writeable | A string representing a local file path or a writeable object | (e.g. an open file descriptor) | | pretty: bool (default False) | True if JSON representation should be pretty-printed, False if | representation should be as compact as possible. | | remove_uids: bool (default True) | True if trace UIDs should be omitted from the JSON representation | | engine: str (default None) | The JSON encoding engine to use. One of: | - "json" for an encoder based on the built-in Python json module | - "orjson" for a fast encoder the requires the orjson package | If not specified, the default encoder is set to the current value of | plotly.io.json.config.default_encoder. | | Returns | ------- | None | | ---------------------------------------------------------------------- | Data descriptors inherited from plotly.basedatatypes.BaseFigure: | | __dict__ | dictionary for instance variables (if defined) | | __weakref__ | list of weak references to the object (if defined) | | data | The `data` property is a tuple of the figure's trace objects | | Returns | ------- | tuple[BaseTraceType] | | layout | The `layout` property of the figure | | Returns | ------- | plotly.graph_objs.Layout | | ---------------------------------------------------------------------- | Data and other attributes inherited from plotly.basedatatypes.BaseFigure: | | __hash__ = None | | ---------------------------------------------------------------------- | Methods inherited from ipywidgets.widgets.domwidget.DOMWidget: | | add_class(self, className) | Adds a class to the top level element of the widget. | | Doesn't add the class if it already exists. | | remove_class(self, className) | Removes a class from the top level element of the widget. | | Doesn't remove the class if it doesn't exist. | | ---------------------------------------------------------------------- | Methods inherited from ipywidgets.widgets.widget.Widget: | | __del__(self) | Object disposal | | add_traits(self, **traits) | Dynamically add trait attributes to the Widget. | | close(self) | Close method. | | Closes the underlying comm. | When the comm is closed, all of the widget views are automatically | removed from the front-end. | | get_state(self, key=None, drop_defaults=False) | Gets the widget state, or a piece of it. | | Parameters | ---------- | key : unicode or iterable (optional) | A single property's name or iterable of property names to get. | | Returns | ------- | state : dict of states | metadata : dict | metadata for each field: {key: metadata} | | get_view_spec(self) | | hold_sync(self) | Hold syncing any state until the outermost context manager exits | | notify_change(self, change) | Called when a property has changed. | | on_displayed(self, callback, remove=False) | (Un)Register a widget displayed callback. | | Parameters | ---------- | callback: method handler | Must have a signature of:: | | callback(widget, **kwargs) | | kwargs from display are passed through without modification. | remove: bool | True if the callback should be unregistered. | | on_msg(self, callback, remove=False) | (Un)Register a custom msg receive callback. | | Parameters | ---------- | callback: callable | callback will be passed three arguments when a message arrives:: | | callback(widget, content, buffers) | | remove: bool | True if the callback should be unregistered. | | open(self) | Open a comm to the frontend if one isn't already open. | | send(self, content, buffers=None) | Sends a custom msg to the widget model in the front-end. | | Parameters | ---------- | content : dict | Content of the message to send. | buffers : list of binary buffers | Binary buffers to send with message | | send_state(self, key=None) | Sends the widget state, or a piece of it, to the front-end, if it exists. | | Parameters | ---------- | key : unicode, or iterable (optional) | A single property's name or iterable of property names to sync with the front-end. | | set_state(self, sync_data) | Called when a state is received from the front-end. | | ---------------------------------------------------------------------- | Class methods inherited from ipywidgets.widgets.widget.Widget: | | close_all() from traitlets.traitlets.MetaHasTraits | | handle_control_comm_opened(comm, msg) from traitlets.traitlets.MetaHasTraits | Class method, called when the comm-open message on the | "jupyter.widget.control" comm channel is received | | ---------------------------------------------------------------------- | Static methods inherited from ipywidgets.widgets.widget.Widget: | | get_manager_state(drop_defaults=False, widgets=None) | Returns the full state for a widget manager for embedding | | :param drop_defaults: when True, it will not include default value | :param widgets: list with widgets to include in the state (or all widgets when None) | :return: | | handle_comm_opened(comm, msg) | Static method, called when a widget is constructed. | | on_widget_constructed(callback) | Registers a callback to be called when a widget is constructed. | | The callback must have the following signature: | callback(widget) | | ---------------------------------------------------------------------- | Readonly properties inherited from ipywidgets.widgets.widget.Widget: | | model_id | Gets the model id of this widget. | | If a Comm doesn't exist yet, a Comm will be created automagically. | | ---------------------------------------------------------------------- | Data descriptors inherited from ipywidgets.widgets.widget.Widget: | | comm | | keys | The traits which are synced. | | ---------------------------------------------------------------------- | Data and other attributes inherited from ipywidgets.widgets.widget.Widget: | | widget_types = <ipywidgets.widgets.widget.WidgetRegistry object> | | widgets = {'acdaa288edd443648bc48bd441898692': FigureWidget({ | 'dat... | | ---------------------------------------------------------------------- | Data descriptors inherited from ipywidgets.widgets.widget.LoggingHasTraits: | | log | | ---------------------------------------------------------------------- | Methods inherited from traitlets.traitlets.HasTraits: | | __getstate__(self) -> 'dict[str, t.Any]' | | __setstate__(self, state: 'dict[str, t.Any]') -> 'None' | | has_trait(self, name: 'str') -> 'bool' | Returns True if the object has a trait with the specified name. | | hold_trait_notifications(self) -> 't.Any' | Context manager for bundling trait change notifications and cross | validation. | | Use this when doing multiple trait assignments (init, config), to avoid | race conditions in trait notifiers requesting other trait values. | All trait notifications will fire after all values have been assigned. | | observe(self, handler: 't.Callable[..., t.Any]', names: 'Sentinel | str | t.Iterable[Sentinel | str]' = traitlets.All, type: 'Sentinel | str' = 'change') -> 'None' | Setup a handler to be called when a trait changes. | | This is used to setup dynamic notifications of trait changes. | | Parameters | ---------- | handler : callable | A callable that is called when a trait changes. Its | signature should be ``handler(change)``, where ``change`` is a | dictionary. The change dictionary at least holds a 'type' key. | * ``type``: the type of notification. | Other keys may be passed depending on the value of 'type'. In the | case where type is 'change', we also have the following keys: | * ``owner`` : the HasTraits instance | * ``old`` : the old value of the modified trait attribute | * ``new`` : the new value of the modified trait attribute | * ``name`` : the name of the modified trait attribute. | names : list, str, All | If names is All, the handler will apply to all traits. If a list | of str, handler will apply to all names in the list. If a | str, the handler will apply just to that name. | type : str, All (default: 'change') | The type of notification to filter by. If equal to All, then all | notifications are passed to the observe handler. | | on_trait_change(self, handler: 'EventHandler | None' = None, name: 'Sentinel | str | None' = None, remove: 'bool' = False) -> 'None' | DEPRECATED: Setup a handler to be called when a trait changes. | | This is used to setup dynamic notifications of trait changes. | | Static handlers can be created by creating methods on a HasTraits | subclass with the naming convention '_[traitname]_changed'. Thus, | to create static handler for the trait 'a', create the method | _a_changed(self, name, old, new) (fewer arguments can be used, see | below). | | If `remove` is True and `handler` is not specified, all change | handlers for the specified name are uninstalled. | | Parameters | ---------- | handler : callable, None | A callable that is called when a trait changes. Its | signature can be handler(), handler(name), handler(name, new), | handler(name, old, new), or handler(name, old, new, self). | name : list, str, None | If None, the handler will apply to all traits. If a list | of str, handler will apply to all names in the list. If a | str, the handler will apply just to that name. | remove : bool | If False (the default), then install the handler. If True | then unintall it. | | set_trait(self, name: 'str', value: 't.Any') -> 'None' | Forcibly sets trait attribute, including read-only attributes. | | setup_instance(*args: 't.Any', **kwargs: 't.Any') -> 'None' | This is called **before** self.__init__ is called. | | trait_defaults(self, *names: 'str', **metadata: 't.Any') -> 'dict[str, t.Any] | Sentinel' | Return a trait's default value or a dictionary of them | | Notes | ----- | Dynamically generated default values may | depend on the current state of the object. | | trait_has_value(self, name: 'str') -> 'bool' | Returns True if the specified trait has a value. | | This will return false even if ``getattr`` would return a | dynamically generated default value. These default values | will be recognized as existing only after they have been | generated. | | Example | | .. code-block:: python | | class MyClass(HasTraits): | i = Int() | | | mc = MyClass() | assert not mc.trait_has_value("i") | mc.i # generates a default value | assert mc.trait_has_value("i") | | trait_metadata(self, traitname: 'str', key: 'str', default: 't.Any' = None) -> 't.Any' | Get metadata values for trait by key. | | trait_names(self, **metadata: 't.Any') -> 'list[str]' | Get a list of all the names of this class' traits. | | trait_values(self, **metadata: 't.Any') -> 'dict[str, t.Any]' | A ``dict`` of trait names and their values. | | The metadata kwargs allow functions to be passed in which | filter traits based on metadata values. The functions should | take a single value as an argument and return a boolean. If | any function returns False, then the trait is not included in | the output. If a metadata key doesn't exist, None will be passed | to the function. | | Returns | ------- | A ``dict`` of trait names and their values. | | Notes | ----- | Trait values are retrieved via ``getattr``, any exceptions raised | by traits or the operations they may trigger will result in the | absence of a trait value in the result ``dict``. | | traits(self, **metadata: 't.Any') -> 'dict[str, TraitType[t.Any, t.Any]]' | Get a ``dict`` of all the traits of this class. The dictionary | is keyed on the name and the values are the TraitType objects. | | The TraitTypes returned don't know anything about the values | that the various HasTrait's instances are holding. | | The metadata kwargs allow functions to be passed in which | filter traits based on metadata values. The functions should | take a single value as an argument and return a boolean. If | any function returns False, then the trait is not included in | the output. If a metadata key doesn't exist, None will be passed | to the function. | | unobserve(self, handler: 't.Callable[..., t.Any]', names: 'Sentinel | str | t.Iterable[Sentinel | str]' = traitlets.All, type: 'Sentinel | str' = 'change') -> 'None' | Remove a trait change handler. | | This is used to unregister handlers to trait change notifications. | | Parameters | ---------- | handler : callable | The callable called when a trait attribute changes. | names : list, str, All (default: All) | The names of the traits for which the specified handler should be | uninstalled. If names is All, the specified handler is uninstalled | from the list of notifiers corresponding to all changes. | type : str or All (default: 'change') | The type of notification to filter by. If All, the specified handler | is uninstalled from the list of notifiers corresponding to all types. | | unobserve_all(self, name: 'str | t.Any' = traitlets.All) -> 'None' | Remove trait change handlers of any type for the specified name. | If name is not specified, removes all trait notifiers. | | ---------------------------------------------------------------------- | Class methods inherited from traitlets.traitlets.HasTraits: | | class_own_trait_events(name: 'str') -> 'dict[str, EventHandler]' from traitlets.traitlets.MetaHasTraits | Get a dict of all event handlers defined on this class, not a parent. | | Works like ``event_handlers``, except for excluding traits from parents. | | class_own_traits(**metadata: 't.Any') -> 'dict[str, TraitType[t.Any, t.Any]]' from traitlets.traitlets.MetaHasTraits | Get a dict of all the traitlets defined on this class, not a parent. | | Works like `class_traits`, except for excluding traits from parents. | | class_trait_names(**metadata: 't.Any') -> 'list[str]' from traitlets.traitlets.MetaHasTraits | Get a list of all the names of this class' traits. | | This method is just like the :meth:`trait_names` method, | but is unbound. | | class_traits(**metadata: 't.Any') -> 'dict[str, TraitType[t.Any, t.Any]]' from traitlets.traitlets.MetaHasTraits | Get a ``dict`` of all the traits of this class. The dictionary | is keyed on the name and the values are the TraitType objects. | | This method is just like the :meth:`traits` method, but is unbound. | | The TraitTypes returned don't know anything about the values | that the various HasTrait's instances are holding. | | The metadata kwargs allow functions to be passed in which | filter traits based on metadata values. The functions should | take a single value as an argument and return a boolean. If | any function returns False, then the trait is not included in | the output. If a metadata key doesn't exist, None will be passed | to the function. | | trait_events(name: 'str | None' = None) -> 'dict[str, EventHandler]' from traitlets.traitlets.MetaHasTraits | Get a ``dict`` of all the event handlers of this class. | | Parameters | ---------- | name : str (default: None) | The name of a trait of this class. If name is ``None`` then all | the event handlers of this class will be returned instead. | | Returns | ------- | The event handlers associated with a trait name, or all event handlers. | | ---------------------------------------------------------------------- | Readonly properties inherited from traitlets.traitlets.HasTraits: | | cross_validation_lock | A contextmanager for running a block with our cross validation lock set | to True. | | At the end of the block, the lock's value is restored to its value | prior to entering the block. | | ---------------------------------------------------------------------- | Data and other attributes inherited from traitlets.traitlets.HasTraits: | | __annotations__ = {'_all_trait_default_generators': 'dict[str, t.Any]'... | | ---------------------------------------------------------------------- | Static methods inherited from traitlets.traitlets.HasDescriptors: | | __new__(*args: 't.Any', **kwargs: 't.Any') -> 't.Any' | Create and return a new object. See help(type) for accurate signature.
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
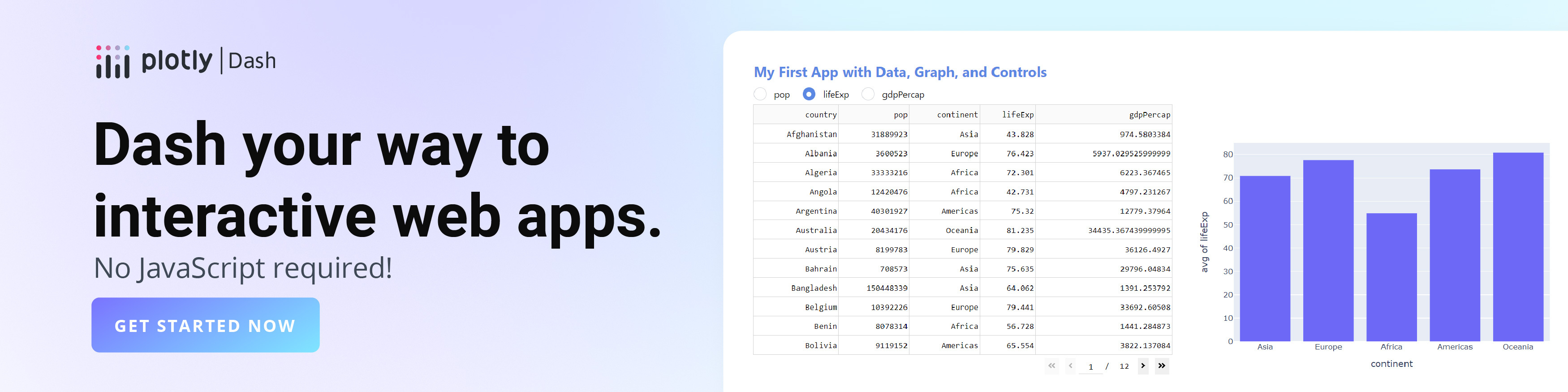