Interactive HTML Export in Python
Plotly allows you to save interactive HTML versions of your figures to your local disk.
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Interactive vs Static Export¶
Plotly figures are interactive when viewed in a web browser: you can hover over data points, pan and zoom axes, and show and hide traces by clicking or double-clicking on the legend. You can export figures either to static image file formats like PNG, JPEG, SVG or PDF or you can export them to HTML files which can be opened in a browser. This page explains how to do the latter.
Saving to an HTML file¶
Any figure can be saved as an HTML file using the write_html
method. These HTML files can be opened in any web browser to access the fully interactive figure.
import plotly.express as px
fig = px.scatter(x=range(10), y=range(10))
fig.write_html("path/to/file.html")
Controlling the size of the HTML file¶
By default, the resulting HTML file is a fully self-contained HTML file which can be uploaded to a web server or shared via email or other file-sharing mechanisms. The downside to this approach is that the file is very large (5Mb+) because it contains an inlined copy of the Plotly.js library required to make the figure interactive. This can be controlled via the include_plotlyjs
argument (see below).
Inserting Plotly Output into HTML using a Jinja2 Template¶
You can insert Plotly output and text related to your data into HTML templates using Jinja2. Use .to_html
to send the HTML to a Python string variable rather than using write_html
to send the HTML to a disk file. Use the full_html=False
option to output just the code necessary to add a figure to a template. We don't want to output a full HTML page, as the template will define the rest of the page's structure — for example, the page's HTML
and BODY
tags. First create an HTML template file containing a Jinja {{ variable }}
. In this example, we customize the HTML in the template file by replacing the Jinja variable {{ fig }}
with our graphic fig
.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" /> <!--It is necessary to use the UTF-8 encoding with plotly graphics to get e.g. negative signs to render correctly -->
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
</head>
<body>
<h1>Here's a Plotly graph!</h1>
{{ fig }}
<p>And here's some text after the graph.</p>
</body>
</html>
Then use the following Python to replace {{ fig }}
in the template with HTML that will display the Plotly figure "fig":
import plotly.express as px
from jinja2 import Template
data_canada = px.data.gapminder().query("country == 'Canada'")
fig = px.bar(data_canada, x='year', y='pop')
output_html_path=r"/path/to/output.html"
input_template_path = r"/path/to/template.html"
plotly_jinja_data = {"fig":fig.to_html(full_html=False)}
#consider also defining the include_plotlyjs parameter to point to an external Plotly.js as described above
with open(output_html_path, "w", encoding="utf-8") as output_file:
with open(input_template_path) as template_file:
j2_template = Template(template_file.read())
output_file.write(j2_template.render(plotly_jinja_data))
HTML export in Dash¶
Dash is the best way to build analytical apps in Python using Plotly figures. To run the app below, run pip install dash
, click "Download" to get the code and run python app.py
.
Get started with the official Dash docs and learn how to effortlessly style & deploy apps like this with Dash Enterprise.
Sign up for Dash Club → Free cheat sheets plus updates from Chris Parmer and Adam Schroeder delivered to your inbox every two months. Includes tips and tricks, community apps, and deep dives into the Dash architecture. Join now.
Full Parameter Documentation¶
import plotly.graph_objects as go
help(go.Figure.write_html)
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
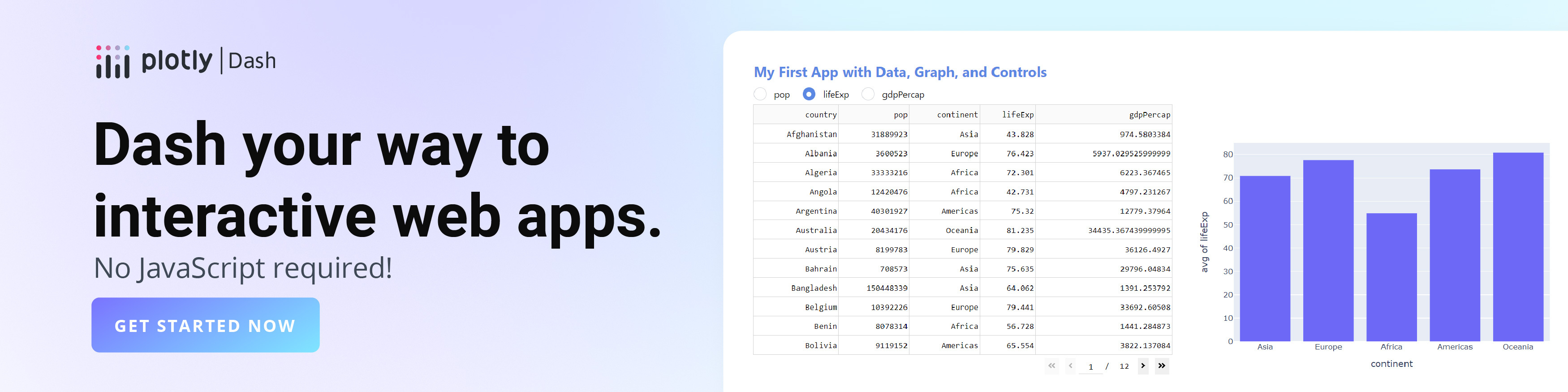