Radar Charts in Python
How to make radar charts in Python with Plotly.
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
A Radar Chart (also known as a spider plot or star plot) displays multivariate data in the form of a two-dimensional chart of quantitative variables represented on axes originating from the center. The relative position and angle of the axes is typically uninformative. It is equivalent to a parallel coordinates plot with the axes arranged radially.
For a Radar Chart, use a polar chart with categorical angular variables, with px.line_polar
, or with go.Scatterpolar
. See more examples of polar charts.
Radar Chart with Plotly Express¶
Plotly Express is the easy-to-use, high-level interface to Plotly, which operates on a variety of types of data and produces easy-to-style figures.
Use line_close=True
for closed lines.
import plotly.express as px
import pandas as pd
df = pd.DataFrame(dict(
r=[1, 5, 2, 2, 3],
theta=['processing cost','mechanical properties','chemical stability',
'thermal stability', 'device integration']))
fig = px.line_polar(df, r='r', theta='theta', line_close=True)
fig.show()
For a filled line in a Radar Chart, update the figure created with px.line_polar
with fig.update_traces
.
import plotly.express as px
import pandas as pd
df = pd.DataFrame(dict(
r=[1, 5, 2, 2, 3],
theta=['processing cost','mechanical properties','chemical stability',
'thermal stability', 'device integration']))
fig = px.line_polar(df, r='r', theta='theta', line_close=True)
fig.update_traces(fill='toself')
fig.show()
Basic Radar Chart with go.Scatterpolar¶
import plotly.graph_objects as go
fig = go.Figure(data=go.Scatterpolar(
r=[1, 5, 2, 2, 3],
theta=['processing cost','mechanical properties','chemical stability', 'thermal stability',
'device integration'],
fill='toself'
))
fig.update_layout(
polar=dict(
radialaxis=dict(
visible=True
),
),
showlegend=False
)
fig.show()
Multiple Trace Radar Chart¶
import plotly.graph_objects as go
categories = ['processing cost','mechanical properties','chemical stability',
'thermal stability', 'device integration']
fig = go.Figure()
fig.add_trace(go.Scatterpolar(
r=[1, 5, 2, 2, 3],
theta=categories,
fill='toself',
name='Product A'
))
fig.add_trace(go.Scatterpolar(
r=[4, 3, 2.5, 1, 2],
theta=categories,
fill='toself',
name='Product B'
))
fig.update_layout(
polar=dict(
radialaxis=dict(
visible=True,
range=[0, 5]
)),
showlegend=False
)
fig.show()
Reference¶
See function reference for px.(line_polar)
or https://plotly.com/python/reference/scatterpolar/ for more information and chart attribute options!
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
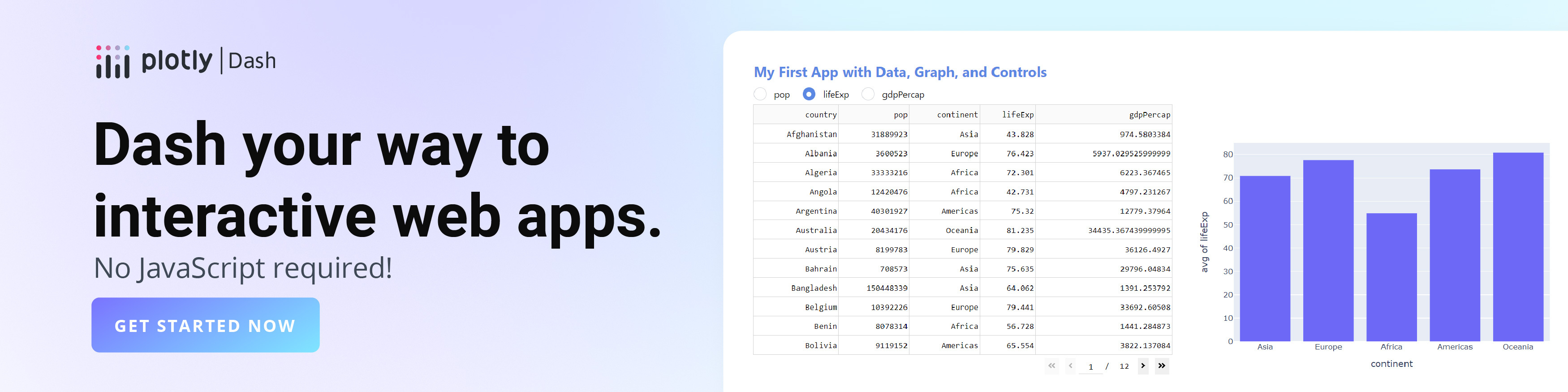