3D Axes in Python
How to format axes of 3d plots in Python with Plotly.
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Range of axes¶
3D figures have an attribute in layout
called scene
, which contains
attributes such as xaxis
, yaxis
and zaxis
parameters, in order to
set the range, title, ticks, color etc. of the axes.
For creating 3D charts, see this page.
Set range
on an axis to manually configure a range for that axis. If you don't set range
, it's automatically calculated. In this example, we set a range
on xaxis
, yaxis
, and zaxis
.
import plotly.graph_objects as go
import numpy as np
np.random.seed(1)
N = 70
fig = go.Figure(data=[go.Mesh3d(x=(70*np.random.randn(N)),
y=(55*np.random.randn(N)),
z=(40*np.random.randn(N)),
opacity=0.5,
color='rgba(244,22,100,0.6)'
)])
fig.update_layout(
scene = dict(
xaxis = dict(nticks=4, range=[-100,100],),
yaxis = dict(nticks=4, range=[-50,100],),
zaxis = dict(nticks=4, range=[-100,100],),),
width=700,
margin=dict(r=20, l=10, b=10, t=10))
fig.show()
Setting only a Lower or Upper Bound for Range¶
New in 5.17
You can also set just a lower or upper bound for range
. In this case, autorange is used for the other bound. In this example, we apply autorange to the lower bound of the yaxis
and the upper bound of zaxis
by setting them to None
.
import plotly.graph_objects as go
import numpy as np
np.random.seed(1)
N = 70
fig = go.Figure(data=[go.Mesh3d(x=(70*np.random.randn(N)),
y=(55*np.random.randn(N)),
z=(40*np.random.randn(N)),
opacity=0.5,
color='rgba(244,22,100,0.6)'
)])
fig.update_layout(
scene = dict(
xaxis = dict(nticks=4, range=[-100,100],),
yaxis = dict(nticks=4, range=[None, 100],),
zaxis = dict(nticks=4, range=[-100, None],),),
width=700,
margin=dict(r=20, l=10, b=10, t=10))
fig.show()
Fixed Ratio Axes¶
import plotly.graph_objects as go
from plotly.subplots import make_subplots
import numpy as np
N = 50
fig = make_subplots(rows=2, cols=2,
specs=[[{'is_3d': True}, {'is_3d': True}],
[{'is_3d': True}, {'is_3d': True}]],
print_grid=False)
for i in [1,2]:
for j in [1,2]:
fig.append_trace(
go.Mesh3d(
x=(60*np.random.randn(N)),
y=(25*np.random.randn(N)),
z=(40*np.random.randn(N)),
opacity=0.5,
),
row=i, col=j)
fig.update_layout(width=700, margin=dict(r=10, l=10, b=10, t=10))
# fix the ratio in the top left subplot to be a cube
fig.update_layout(scene_aspectmode='cube')
# manually force the z-axis to appear twice as big as the other two
fig.update_layout(scene2_aspectmode='manual',
scene2_aspectratio=dict(x=1, y=1, z=2))
# draw axes in proportion to the proportion of their ranges
fig.update_layout(scene3_aspectmode='data')
# automatically produce something that is well proportioned using 'data' as the default
fig.update_layout(scene4_aspectmode='auto')
fig.show()
Set Axes Title¶
import plotly.graph_objects as go
import numpy as np
# Define random surface
N = 50
fig = go.Figure()
fig.add_trace(go.Mesh3d(x=(60*np.random.randn(N)),
y=(25*np.random.randn(N)),
z=(40*np.random.randn(N)),
opacity=0.5,
color='yellow'
))
fig.add_trace(go.Mesh3d(x=(70*np.random.randn(N)),
y=(55*np.random.randn(N)),
z=(30*np.random.randn(N)),
opacity=0.5,
color='pink'
))
fig.update_layout(scene = dict(
xaxis_title='X AXIS TITLE',
yaxis_title='Y AXIS TITLE',
zaxis_title='Z AXIS TITLE'),
width=700,
margin=dict(r=20, b=10, l=10, t=10))
fig.show()
Ticks Formatting¶
import plotly.graph_objects as go
import numpy as np
# Define random surface
N = 50
fig = go.Figure(data=[go.Mesh3d(x=(60*np.random.randn(N)),
y=(25*np.random.randn(N)),
z=(40*np.random.randn(N)),
opacity=0.5,
color='rgba(100,22,200,0.5)'
)])
# Different types of customized ticks
fig.update_layout(scene = dict(
xaxis = dict(
ticktext= ['TICKS','MESH','PLOTLY','PYTHON'],
tickvals= [0,50,75,-50]),
yaxis = dict(
nticks=5, tickfont=dict(
color='green',
size=12,
family='Old Standard TT, serif',),
ticksuffix='#'),
zaxis = dict(
nticks=4, ticks='outside',
tick0=0, tickwidth=4),),
width=700,
margin=dict(r=10, l=10, b=10, t=10)
)
fig.show()
Background and Grid Color¶
import plotly.graph_objects as go
import numpy as np
N = 50
fig = go.Figure(data=[go.Mesh3d(x=(30*np.random.randn(N)),
y=(25*np.random.randn(N)),
z=(30*np.random.randn(N)),
opacity=0.5,)])
# xaxis.backgroundcolor is used to set background color
fig.update_layout(scene = dict(
xaxis = dict(
backgroundcolor="rgb(200, 200, 230)",
gridcolor="white",
showbackground=True,
zerolinecolor="white",),
yaxis = dict(
backgroundcolor="rgb(230, 200,230)",
gridcolor="white",
showbackground=True,
zerolinecolor="white"),
zaxis = dict(
backgroundcolor="rgb(230, 230,200)",
gridcolor="white",
showbackground=True,
zerolinecolor="white",),),
width=700,
margin=dict(
r=10, l=10,
b=10, t=10)
)
fig.show()
Disabling tooltip spikes¶
By default, guidelines originating from the tooltip point are drawn. It is possible to disable this behaviour with the showspikes
parameter. In this example we only keep the z
spikes (projection of the tooltip on the x-y
plane). Hover on the data to show this behaviour.
import plotly.graph_objects as go
import numpy as np
N = 50
fig = go.Figure(data=[go.Mesh3d(x=(30*np.random.randn(N)),
y=(25*np.random.randn(N)),
z=(30*np.random.randn(N)),
opacity=0.5,)])
fig.update_layout(scene=dict(xaxis_showspikes=False,
yaxis_showspikes=False))
fig.show()
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
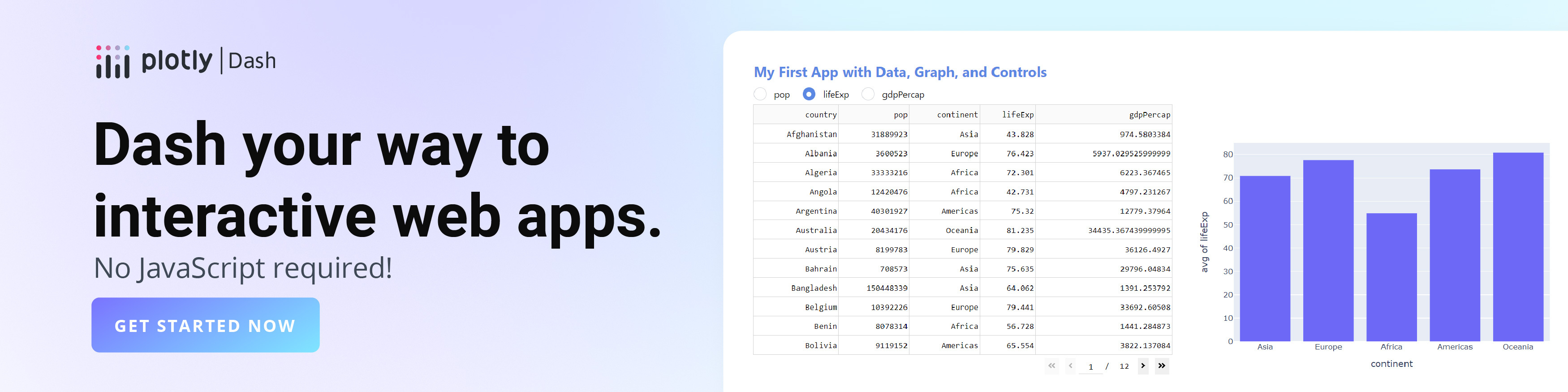