Click Events in JavaScript
How to bind callback functions to click events in D3.js-based JavaScript charts.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
{
points: [{
curveNumber: 2, // index in data of the trace associated with the selected point
pointNumber: 2, // index of the selected point
x: 5, // x value
y: 600, // y value
data: {/* */}, // ref to the trace as sent to Plotly.newPlot associated with the selected point
fullData: {/* */}, // ref to the trace including all the defaults
xaxis: {/* */}, // ref to x-axis object (i.e layout.xaxis) associated with the selected point
yaxis: {/* */} // ref to y-axis object " "
}, {
/* similarly for other selected points */
}]
}
var myPlot = document.getElementById('myDiv'),
N = 16,
x = d3.range(N),
y = d3.range(N).map( d3.random.normal() ),
data = [ { x:x, y:y, type:'scatter',
mode:'markers', marker:{size:16} } ],
layout = {
hovermode:'closest',
title:'Click on Points'
};
Plotly.newPlot('myDiv', data, layout);
myPlot.on('plotly_click', function(data){
var pts = '';
for(var i=0; i < data.points.length; i++){
pts = 'x = '+data.points[i].x +'\ny = '+
data.points[i].y.toPrecision(4) + '\n\n';
}
alert('Closest point clicked:\n\n'+pts);
});
var myPlot = document.getElementById('myDiv'),
N = 100,
x = d3.range(N),
y1 = d3.range(N).map( d3.random.normal() ),
y2 = d3.range(N).map( d3.random.normal(-2) ),
y3 = d3.range(N).map( d3.random.normal(2) ),
trace1 = { x:x, y:y1, type:'scatter', mode:'lines', name:'Jeff' },
trace2 = { x:x, y:y2, type:'scatter', mode:'lines', name:'Terren' },
trace3 = { x:x, y:y3, type:'scatter', mode:'lines', name:'Arthur' },
data = [ trace1, trace2, trace3 ],
layout = {
hovermode:'closest',
title:'Click on Points to add an Annotation on it'
};
Plotly.newPlot('myDiv', data, layout);
myPlot.on('plotly_click', function(data){
var pts = '';
for(var i=0; i < data.points.length; i++){
annotate_text = 'x = '+data.points[i].x +
'y = '+data.points[i].y.toPrecision(4);
annotation = {
text: annotate_text,
x: data.points[i].x,
y: parseFloat(data.points[i].y.toPrecision(4))
}
annotations = self.layout.annotations || [];
annotations.push(annotation);
Plotly.relayout('myDiv',{annotations: annotations})
}
});
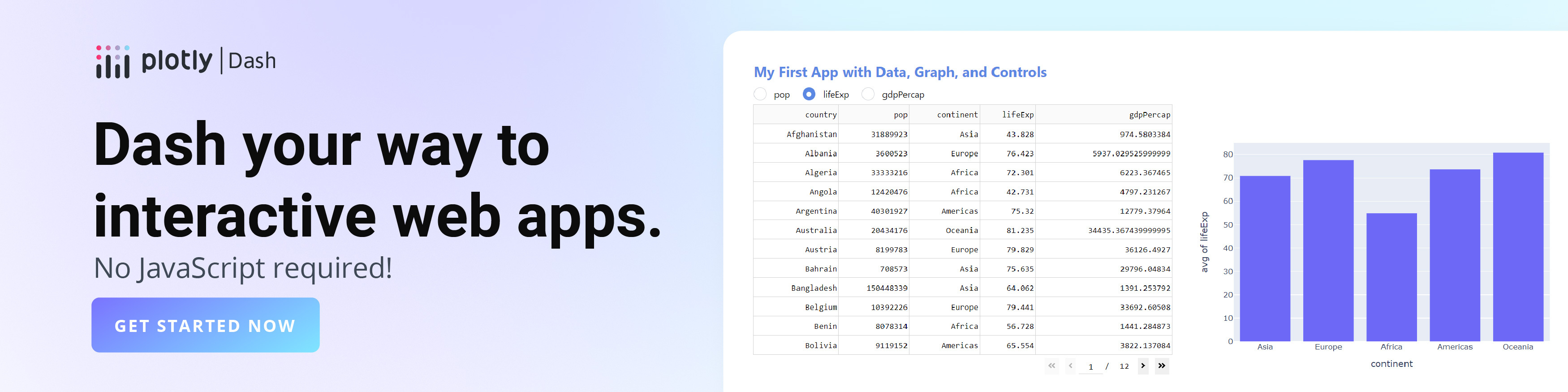