Function Reference in JavaScript
Plotly.js function reference. How to create, update, and modify graphs drawn with Plotly's JavaScript Graphing Library.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Common parameters
graphDiv
- The functions documented here all create or modify a plot that is drawn into a
<div>
element on the page, commonly referred to asgraphDiv
orplotDiv
. The first argument to each function on this page is a reference to this element, and it can be either a DOM node, i.e. the output ofdocument.getElementById()
, or a string, in which case it will be treated as theid
of thediv
. A note on sizing: You can either supply height and width in thelayout
object (see below), or give the<div>
a height and width in CSS. data
- The data to be plotted is described in an array usually called
data
, whose elements are trace objects of various types (e.g.scatter
,bar
etc) as documented in the Full Reference. layout
- The layout of the plot – non-data-related visual attributes such as the title, annotations etc – is described in an object usually called
layout
, as documented in/ the Full Reference. config
- High-level configuration options for the plot, such as the scroll/zoom/hover behaviour, is described in an object usually called
config
, as documented here. The difference betweenconfig
andlayout
is thatlayout
relates to the content of the plot, whereasconfig
relates to the context in which the plot is being shown. frames
- Animation frames are described in an object usually called
frames
as per the example here. They can containdata
andlayout
objects, which define any changes to be animated, and atraces
object that defines which traces to animate. Additionally, frames containingname
and/orgroup
attributes can be referenced by Plotly.animate after they are added by Plotly.addFrames
Plotly.newPlot
Draws a new plot in an<div>
element, overwriting any existing plot. To update an existing plot in a <div>
, it is much more efficient to use Plotly.react
than to overwrite it.
After plotting, the
data
or layout
can always be retrieved from the <div>
element in which the plot was drawn:
var graphDiv = document.getElementById('id_of_the_div')
var data = [{
x: [1999, 2000, 2001, 2002],
y: [10, 15, 13, 17],
type: 'scatter'
}];
var layout = {
title: 'Sales Growth',
xaxis: {
title: 'Year',
showgrid: false,
zeroline: false
},
yaxis: {
title: 'Percent',
showline: false
}
};
Plotly.newPlot(graphDiv, data, layout);
...
var dataRetrievedLater = graphDiv.data;
var layoutRetrievedLater = graphDiv.layout;
Plotly.react
Plotly.react
has the same signature as Plotly.newPlot
above, and can be used in its place to create a plot, but when called again on the same <div>
will update it far more efficiently than Plotly.newPlot
, which would destroy and recreate the plot. Plotly.react
is as fast as Plotly.restyle
/Plotly.relayout
documented below.
Important Note: In order to use this method to plot new items in arrays under
data
such as x
or marker.color
etc, these items must either have been added immutably (i.e. the identity of the parent array must have changed) or the value of layout.datarevision
must have changed.
Plotly.restyle
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.An efficient means of changing attributes in the
data
array in an existing plot. When restyling, you may choose to have the specified changes affect as many traces as desired. The update is given as a single object and the traces that are affected are given as a list of traces indices. Note, leaving the trace indices unspecified assumes that you want to restyle all the traces.
// restyle a single trace using attribute strings
var update = {
opacity: 0.4,
'marker.color': 'red'
};
Plotly.restyle(graphDiv, update, 0);
// restyle all traces using attribute strings
var update = {
opacity: 0.4,
'marker.color': 'red'
};
Plotly.restyle(graphDiv, update);
// restyle two traces using attribute strings
var update = {
opacity: 0.4,
'marker.color': 'red'
};
Plotly.restyle(graphDiv, update, [1, 2]);
See the Pen Plotly.restyle by plotly (@plotly) on CodePen.
The above examples have applied values across single or multiple traces. However, you can also specify arrays of values to apply to traces in turn.
// restyle the first trace's marker color 'red' and the second's 'green'
var update = {
'marker.color': ['red', 'green']
};
Plotly.restyle(graphDiv, update, [0, 1])
// alternate between red and green for all traces (note omission of traces)
var update = {
'marker.color': ['red', 'green']
};
Plotly.restyle(graphDiv, update)
See the Pen Plotly.restyle Traces in Turn by plotly (@plotly) on CodePen.
In restyle, arrays are assumed to be used in conjunction with the trace indices provided. Therefore, to apply an array as a value, you need to wrap it in an additional array. For example:
// update the color attribute of the first trace so that the markers within the same trace
// have different colors
var update = {
'marker.color': [['red', 'green']]
}
Plotly.restyle(graphDiv, update, [0])
// update two traces with new z data
var update = {z: [[[1,2,3], [2,1,2], [1,1,1]], [[0,1,1], [0,2,1], [3,2,1]]]};
Plotly.restyle(graphDiv, update, [1, 2])
See the Pen Plotly.restyle Arrays by plotly (@plotly) on CodePen.
The term attribute strings is used above to mean flattened (e.g.,
{marker: {color: 'red'}}
vs. {'marker.color': red}
). When you pass an attribute string to restyle inside the update object, it’s assumed to mean update only this attribute. Therefore, if you wish to replace and entire sub-object, you may simply specify one less level of nesting.
// replace the entire marker object with the one provided
var update = {
marker: {color: 'red'}
};
Plotly.restyle(graphDiv, update, [0])
See the Pen Plotly.restyle Attribute strings by plotly (@plotly) on CodePen.
Finally, you may wish to selectively reset or ignore certain properties when restyling. This may be useful when specifying multiple properties for multiple traces so that you can carefully target what is and is not affected. In general `null` resets a property to the default while `undefined` applies no change to the current state.
// Set the first trace's line to red, the second to the default, and ignore the third
Plotly.restyle(graphDiv, {
'line.color': ['red', null, undefined]
}, [0, 1, 2])
See the Pen null vs. undefined in Plotly.restyle by plotly (@plotly) on CodePen.
Plotly.relayout
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.An efficient means of updating the
layout
object of an existing plot. The call signature and arguments for relayout are similar (but simpler) to restyle. Because there are no indices to deal with, arrays need not be wrapped. Also, no argument specifying applicable trace indices is passed in.
// update only values within nested objects
var update = {
title: 'some new title', // updates the title
'xaxis.range': [0, 5], // updates the xaxis range
'yaxis.range[1]': 15 // updates the end of the yaxis range
};
Plotly.relayout(graphDiv, update)
See the Pen Plotly.relayout by plotly (@plotly) on CodePen.
Plotly.update
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.An efficient means of updating both the
data
array and layout
object in an existing plot, basically a combination of Plotly.restyle
and Plotly.relayout
.
//update the layout and all the traces
var layout_update = {
title: 'some new title', // updates the title
};
var data_update = {
'marker.color': 'red'
};
Plotly.update(graphDiv, data_update, layout_update)
//update the layout and a single trace
var layout_update = {
title: 'some new title', // updates the title
};
var data_update = {
'marker.color': 'red'
};
Plotly.update(graphDiv, data_update, layout_update,0)
//update the layout and two specific traces
var layout_update = {
title: 'some new title', // updates the title
};
var data_update = {
'marker.color': 'red'
};
Plotly.update(graphDiv, data_update, layout_update, [0,2])
See the Pen Plotly.update by plotly (@plotly) on CodePen.
Plotly.validate
Plotly.validate
allows users to validate their input data
array and layout
object. This can be done on the data
array and layout
object passed into Plotly.newPlot
or on an updated graphDiv
with Plotly.validate(graphDiv.data, graphDiv.layout)
.
var data = [{
type: 'bar',
y: [2, 1, 3, 2],
orientation: 'horizontal'
}];
var out = Plotly.validate(data, layout);
console.log(out[0].msg)
// "In data trace 0, key orientation is set to an invalid value (horizontal)"
Plotly.makeTemplate
Plotly.makeTemplate
copies the style information from a figure. It does this by returning a template
object which can be passed to the layout.template
attribute of another figure.
var figure = {
data: [{
type: 'bar',
marker: {color: 'red'},
y: [2, 1, 3, 2],
}],
layout:{
title: 'Quarterly Earnings'
}
};
var template = Plotly.makeTemplate(figure);
var newData = [{
type:'bar',
y:[3,2,5,8]
}]
var layout = {template:template}
Plotly.newPlot(graphDiv,newData,layout)
Plotly.validateTemplate
Plotly.validateTemplate
allows users to Test for consistency between the given figure and a template,
either already included in the figure or given separately. Note that not every issue identified here is necessarily
a problem, it depends on what you're using the template for.
var out = Plotly.validateTemplate(figure, template);
console.log(out[0].msg)
// "The template has 1 traces of type bar but there are none in the data."
Plotly.addTraces
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.This allows you to add new traces to an existing
graphDiv
at any location in its data array. Every graphDiv
object has a data
component which is an array of JSON blobs that each describe one trace. The full list of trace types can be found in the Full Reference.
// add a single trace to an existing graphDiv
Plotly.addTraces(graphDiv, {y: [2,1,2]});
// add two traces
Plotly.addTraces(graphDiv, [{y: [2,1,2]}, {y: [4, 5, 7]}]);
// add a trace at the beginning of the data array
Plotly.addTraces(graphDiv, {y: [1, 5, 7]}, 0);
See the Pen Plotly.addtraces by plotly (@plotly) on CodePen.
Plotly.deleteTraces
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.This allows you to remove traces from an existing
graphDiv
by specifying the indices of the traces to be removed.
// remove the first trace
Plotly.deleteTraces(graphDiv, 0);
// remove the last two traces
Plotly.deleteTraces(graphDiv, [-2, -1]);
See the Pen Plotly.deleteTraces by plotly (@plotly) on CodePen.
Plotly.moveTraces
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.This allows you to reorder traces in an existing
graphDiv
. This will change the ordering of the layering and the legend.
All traces defined in graphDiv
are ordered in an array. They are drawn one by one from first to last. Each time a new layer or trace is drawn to the canvas the new trace is drawn directly over the current canvas, replacing the colors of the traces and background. This algorithm to image stacking/drawing is known as the Painter's Algorithm. As its name implies the Painter's Algorithm is typically the manner in which a painter paints a landscape, starting from objects with the most perspective depth and progressively moving forward and layering over the background objects.
// move the first trace (at index 0) the the end of the data array
Plotly.moveTraces(graphDiv, 0);
// move selected traces (at indices [0, 3, 5]) to the end of the data array
Plotly.moveTraces(graphDiv, [0, 3, 5]);
// move last trace (at index -1) to the beginning of the data array (index 0)
Plotly.moveTraces(graphDiv, -1, 0);
// move selected traces (at indices [1, 4, 5]) to new indices [0, 3, 2]
Plotly.moveTraces(graphDiv, [1, 4, 5], [0, 3, 2]);
See the Pen Plotly.moveTraces by plotly (@plotly) on CodePen.
Plotly.extendTraces
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.This allows you to add data to traces in an existing
graphDiv
.
// extend one trace
Plotly.extendTraces(graphDiv, {y: [[rand()]]}, [0])
// extend multiple traces
Plotly.extendTraces(graphDiv, {y: [[rand()], [rand()]]}, [0, 1])
// extend multiple traces up to a maximum of 10 points per trace
Plotly.extendTraces(graphDiv, {y: [[rand()], [rand()]]}, [0, 1], 10)
See the Pen Plotly.extendTraces by plotly (@plotly) on CodePen.
Plotly.prependTraces
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.This allows you to prepend data to an existing trace
graphDiv
.
// prepend one trace
Plotly.prependTraces(graphDiv, {y: [[rand()]]}, [0])
// prepend multiple traces
Plotly.prependTraces(graphDiv, {y: [[rand()], [rand()]]}, [0, 1])
// prepend multiple traces up to a maximum of 10 points per trace
Plotly.prependTraces(graphDiv, {y: [[rand()], [rand()]]}, [0, 1], 10)
Plotly.addFrames
This function has comparable performance toPlotly.react
and is faster than redrawing the whole plot with Plotly.newPlot
.This allows you to add animation frames to a
graphDiv
. The group
or name
attribute of a frame can
be used by Plotly.animate in place of a frame object (or array of
frame objects).
See example here.
Plotly.animate
Add dynamic behaviour to plotly graphs withPlotly.animate
.
Plotly.newPlot('graph', [{
x: [1, 2, 3],
y: [0, 0.5, 1],
line: {simplify: false},
}]);
function randomize() {
Plotly.animate('graph', {
data: [{y: [Math.random(), Math.random(), Math.random()]}],
traces: [0],
layout: {}
}, {
transition: {
duration: 500,
easing: 'cubic-in-out'
},
frame: {
duration: 500
}
})
}
See the Pen Plotly.animate by plotly (@plotly) on CodePen.
Plotly.purge
Usingpurge
will clear the div, and remove any Plotly plots that have been placed in it.
// purge will be used on the div that you wish clear of Plotly plots
Plotly.purge(graphDiv);
See the Pen Plotly.purge by plotly (@plotly) on CodePen.
Plotly.toImage
toImage
will generate a promise to an image of the plot in data URL format.
// Plotly.toImage will turn the plot in the given div into a data URL string
// toImage takes the div as the first argument and an object specifying image properties as the other
Plotly.toImage(graphDiv, {format: 'png', width: 800, height: 600}).then(function(dataUrl) {
// use the dataUrl
})
See the Pen Plotly.toImage by plotly (@plotly) on CodePen.
Plotly.downloadImage
downloadImage
will trigger a request to download the image of a Plotly plot.
// downloadImage will accept the div as the first argument and an object specifying image properties as the other
Plotly.downloadImage(graphDiv, {format: 'png', width: 800, height: 600, filename: 'newplot'});
See the Pen Plotly.toImage by plotly (@plotly) on CodePen.
Using events
Plots emit events prefixed withplotly_
when clicked or hovered over, and event handlers can be bound to events using the on
method that is exposed by the plot div object. For more information and examples of how to use Plotly events see: https://plotly.com/javascript/plotlyjs-events/.
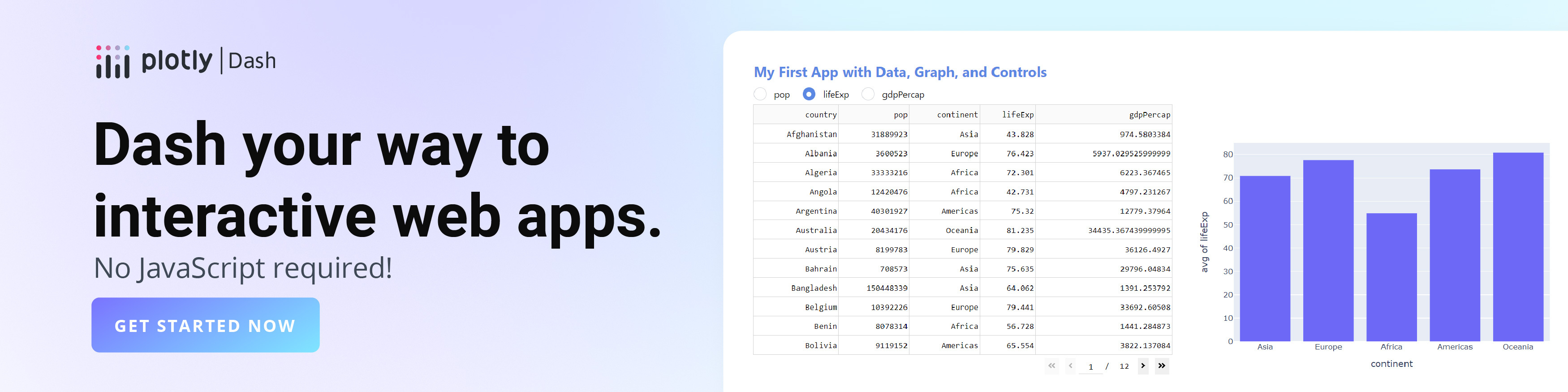