Configuration Options in JavaScript
How to set the configuration options for figures in JavaScript.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
config
argument sets properties like the mode bar buttons and the interactivity in the chart.
It's the last argument in Plotly.newPlot
calls.
View the full list of configuration options in the plotly.js source code on GitHub .
// mousewheel or two-finger scroll zooms the plot
var trace1 = {
x:['2020-10-04', '2021-11-04', '2023-12-04'],
y: [90, 40, 60],
type: 'scatter'
};
var data = [trace1];
var layout = {
title: 'Scroll and Zoom',
showlegend: false
};
Plotly.newPlot('myDiv', data, layout, {scrollZoom: true});
In editable mode, users can edit the chart title, axis labels and trace names in the legend.
var trace1 = {
x: [0, 1, 2, 3, 4],
y: [1, 5, 3, 7, 5],
mode: 'lines+markers',
type: 'scatter'
};
var trace2 = {
x: [1, 2, 3, 4, 5],
y: [4, 0, 4, 6, 8],
mode: 'lines+markers',
type: 'scatter'
};
var data = [trace1, trace2];
var layout = {title: 'Click Here<br>to Edit Chart Title'};
Plotly.newPlot('myDiv', data, layout, {editable: true});
var trace1 = {
x: [0, 1, 2, 3, 4, 5, 6],
y: [1, 9, 4, 7, 5, 2, 4],
mode: 'markers',
marker: {
size: [20, 40, 25, 10, 60, 90, 30],
}
};
var data = [trace1];
var layout = {
title: 'Create a Static Chart',
showlegend: false
};
Plotly.newPlot('myDiv', data, layout, {staticPlot: true});
var trace1 = {
x: [0, 1, 2, 3, 4, 5, 6],
y: [1, 9, 4, 7, 5, 2, 4],
mode: 'markers',
marker: {
size: [20, 40, 25, 10, 60, 90, 30],
}
};
var data = [trace1];
var layout = {
title: 'Download Chart as SVG instead of PNG',
showlegend: false
};
var config = {
toImageButtonOptions: {
format: 'svg', // one of png, svg, jpeg, webp
filename: 'custom_image',
height: 500,
width: 700,
scale: 1 // Multiply title/legend/axis/canvas sizes by this factor
}
};
Plotly.newPlot('myDiv', data, layout, config);
When users hover over a figure generated with plotly.js
, a modebar
appears in the top-right of the figure. This presents users with several options for interacting with the figure.
By default, the modebar
is only visible while the user is hovering over the chart. If you would like the modebar
to always be visible regardless of whether or not the user is currently hovering over the figure, set the displayModeBar
attribute in the config
of your figure to true
.
var data = [{
y:['Marc', 'Henrietta', 'Jean', 'Claude', 'Jeffrey', 'Jonathan', 'Jennifer', 'Zacharias'],
x: [90, 40, 60, 80, 75, 92, 87, 73],
type: 'bar',
orientation: 'h'}]
var layout = {
title: 'Always Display the Modebar',
showlegend: false}
Plotly.newPlot('myDiv', data, layout, {displayModeBar: true})
When users hover over a figure generated with plotly.js
, a modebar
appears in the top-right of the figure. This presents users with several options for interacting with the figure.
By default, the modebar
is only visible while the user is hovering over the chart. If you would like the modebar
to never be visible, then set the displayModeBar
attribute in the config
of your figure to false
.
var trace1 = {
x:['Zebras', 'Lions', 'Pelicans'],
y: [90, 40, 60],
type: 'bar',
name: 'New York Zoo'
};
var trace2 = {
x:['Zebras', 'Lions', 'Pelicans'],
y: [10, 80, 45],
type: 'bar',
name: 'San Francisco Zoo'
};
var data = [trace1, trace2];
var layout = {
title: 'Hide the Modebar',
showlegend: true
};
Plotly.newPlot('myDiv', data, layout, {displayModeBar: false});
To delete buttons from the modebar, pass an array of strings containing the names of the buttons you want to remove to the modeBarButtonsToRemove
attribute in the figure's configuration object. Note that different chart types have different default modebars. The following is a list of all the modebar buttons and the chart types they are associated with:
- -'2D',
zoom2d
,pan2d
,select2d
,lasso2d
,zoomIn2d
,zoomOut2d
,autoScale2d
,resetScale2d
- -'3D',
zoom3d
,pan3d
,orbitRotation
,tableRotation
,handleDrag3d
,resetCameraDefault3d
,resetCameraLastSave3d
,hoverClosest3d
- -'Cartesian',
hoverClosestCartesian
,hoverCompareCartesian
- -'Geo',
zoomInGeo
,zoomOutGeo
,resetGeo
,hoverClosestGeo
- -'Other',
hoverClosestGl2d
,hoverClosestPie
,toggleHover
,resetViews
,toImage
,sendDataToCloud
,toggleSpikelines
,resetViewMapbox
var data = [{
x:['trees', 'flowers', 'hedges'],
y: [90, 130, 40],
type: 'bar'}]
var layout = {
title: 'Remove Modebar Buttons',
showlegend: false}
Plotly.newPlot('myDiv', data, layout, {modeBarButtonsToRemove: ['toImage']})
The following example shows how to add a button to your modebar, either by using one of the Plotly icons or an arbitrary icon with a custom behaviour.
var icon1 = {
'width': 500,
'height': 600,
'path': 'M224 512c35.32 0 63.97-28.65 63.97-64H160.03c0 35.35 28.65 64 63.97 64zm215.39-149.71c-19.32-20.76-55.47-51.99-55.47-154.29 0-77.7-54.48-139.9-127.94-155.16V32c0-17.67-14.32-32-31.98-32s-31.98 14.33-31.98 32v20.84C118.56 68.1 64.08 130.3 64.08 208c0 102.3-36.15 133.53-55.47 154.29-6 6.45-8.66 14.16-8.61 21.71.11 16.4 12.98 32 32.1 32h383.8c19.12 0 32-15.6 32.1-32 .05-7.55-2.61-15.27-8.61-21.71z'
}
var colors = ['green', 'red', 'blue']
var data = [{
mode: 'lines',
y: [2, 1, 2],
line: {color: colors[0], width: 3, shape: 'spline'}
}]
var layout = {
title: 'add mode bar button with custom icon',
modebardisplay: false}
var config = {
modeBarButtonsToAdd: [
{
name: 'color toggler',
icon: icon1,
click: function(gd) {
var newColor = colors[Math.floor(3 * Math.random())]
Plotly.restyle(gd, 'line.color', newColor)
}},
{
name: 'button1',
icon: Plotly.Icons.pencil,
direction: 'up',
click: function(gd) {alert('button1')
}}],
modeBarButtonsToRemove: ['pan2d','select2d','lasso2d','resetScale2d','zoomOut2d']}
Plotly.newPlot('myDiv', data, layout, config)
Note: showLink
now defaults to false.
var trace1 = {
x: [0, 1, 2, 3, 4, 5, 6],
y: [1, 9, 4, 7, 5, 2, 4],
mode: 'lines+markers',
type: 'scatter'
};
var data = [trace1];
var layout = {
title: 'Display the Edit Chart Link'
};
var config = {
showLink: true,
plotlyServerURL: "https://chart-studio.plotly.com"
};
Plotly.newPlot('myDiv', data, layout, config);
var data = [{
z: [[0, 1, 2, 3, 4, 5, 6],
[1, 9, 4, 7, 5, 2, 4],
[2, 4, 2, 1, 6, 9, 3]],
type: 'heatmap'}]
var layout = {title: 'Customize The Edit Chart Link Text'}
var config = {
showLink: true,
plotlyServerURL: "https://chart-studio.plotly.com",
linkText: 'This text is custom!'
};
Plotly.newPlot('myDiv', data, layout, config)
var data = [{
values: [19, 26, 55],
labels: ['Residential', 'Non-Residential', 'Utility'],
type: 'pie'
}];
var layout = {
title: 'Show Edit in Chart Studio Modebar Button'
};
var config = {
showEditInChartStudio: true,
plotlyServerURL: "https://chart-studio.plotly.com"
};
Plotly.newPlot('myDiv', data, layout, config);
Load and register a non-default locale by adding <script src="https://cdn.plot.ly/plotly-locale-YOUR-LOCALE-latest.js"></script>
to your HTML after the plotly.js tag and then reference the locale in the config
. For Example, the codepen example below has
<script src="https://cdn.plot.ly/plotly-locale-fr-latest.js"></script>
in its HTML. For more information and a list of available locales, see
https://github.com/plotly/plotly.js/blob/master/dist/README.md#to-include-localization
var trace1 = {
type: "scatter",
mode: "lines",
x: ['2018-01-01', '2018-08-31'],
y: [10, 5],
line: {color: '#17BECF'}
};
var trace2 = {
type: "scatter",
mode: "lines",
x: ['2018-01-01', '2018-08-31'],
y: [3,7],
line: {color: '#7F7F7F'}
};
var data = [trace1,trace2];
var layout = {
title: 'Custom Locale',
};
var config = {locale: 'fr'};
Plotly.newPlot('myDiv', data, layout, config);
var trace1 = {
x:['trees', 'flowers', 'hedges'],
y: [90, 130, 40],
type: 'bar'
};
var data = [trace1];
var layout = {
title: 'Hide the Plotly Logo on the Modebar',
showlegend: false
};
Plotly.newPlot('myDiv', data, layout, {displaylogo: false});
var trace1 = {
type: 'bar',
x: [1, 2, 3, 4],
y: [5, 10, 2, 8],
marker: {
color: '#C8A2C8',
line: {
width: 2.5
}
}
};
var data = [ trace1 ];
var layout = {
title: 'Responsive to window size!',
font: {size: 18}
};
Plotly.newPlot('myDiv', data, layout, {responsive: true});
Sets the maximum delay between two consecutive clicks to be interpreted as a double-click in ms. This is the time interval between first mousedown, and' second mouseup. The default timing is 300 ms (less than half a second). This setting propagates to all on-subplot double clicks, (except for geo and mapbox).
var data = [{
type: "bar",
y: [3, 5, 3, 2],
x: ["2019-09-02", "2019-10-10", "2019-11-12", "2019-12-22"]
}];
var layout = {xaxis: {type: 'date'}};
var config = {doubleClickDelay: 1000}
Plotly.newPlot("myDiv", data, layout, config)
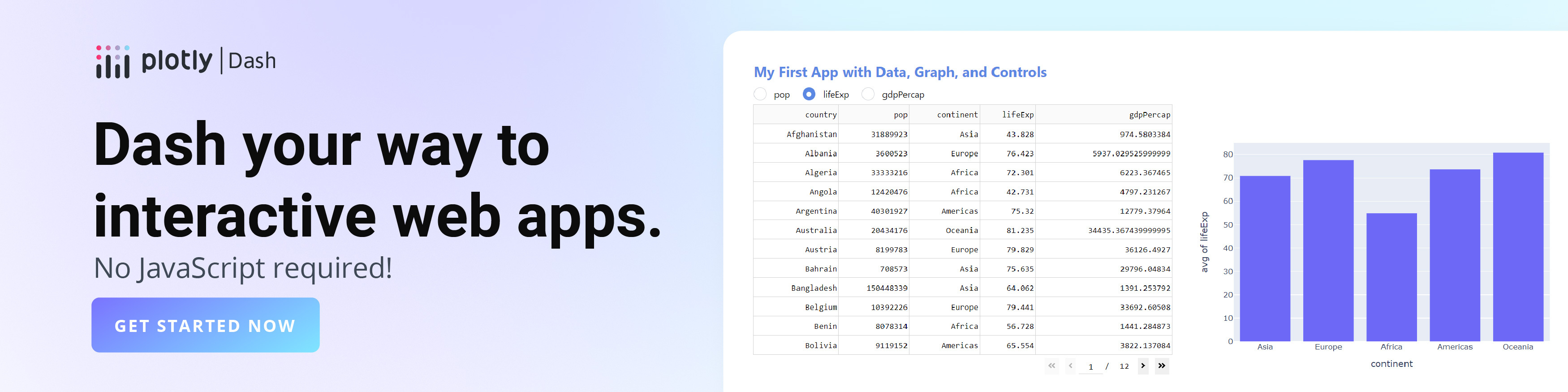