Ternary contours in Python
How to make Ternary Contour Plots in Python with plotly
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Ternary contour plots¶
A ternary contour plots represents isovalue lines of a quantity defined inside a ternary diagram, i.e. as a function of three variables which sum is constant. Coordinates of the ternary plot often correspond to concentrations of three species, and the quantity represented as contours is some property (e.g., physical, chemical, thermodynamical) varying with the composition.
For ternary contour plots, use the figure factory called create_ternary_contour
. The figure factory interpolates between given data points in order to compute the contours.
Below we represent an example from metallurgy, where the mixing enthalpy is represented as a contour plot for aluminum-copper-yttrium (Al-Cu-Y) alloys.
Simple ternary contour plot with plotly¶
import plotly.figure_factory as ff
import numpy as np
Al = np.array([0. , 0. , 0., 0., 1./3, 1./3, 1./3, 2./3, 2./3, 1.])
Cu = np.array([0., 1./3, 2./3, 1., 0., 1./3, 2./3, 0., 1./3, 0.])
Y = 1 - Al - Cu
# synthetic data for mixing enthalpy
# See https://pycalphad.org/docs/latest/examples/TernaryExamples.html
enthalpy = (Al - 0.01) * Cu * (Al - 0.52) * (Cu - 0.48) * (Y - 1)**2
fig = ff.create_ternary_contour(np.array([Al, Y, Cu]), enthalpy,
pole_labels=['Al', 'Y', 'Cu'],
interp_mode='cartesian')
fig.show()
Customized ternary contour plot¶
import plotly.figure_factory as ff
import numpy as np
Al = np.array([0. , 0. , 0., 0., 1./3, 1./3, 1./3, 2./3, 2./3, 1.])
Cu = np.array([0., 1./3, 2./3, 1., 0., 1./3, 2./3, 0., 1./3, 0.])
Y = 1 - Al - Cu
# synthetic data for mixing enthalpy
# See https://pycalphad.org/docs/latest/examples/TernaryExamples.html
enthalpy = 2.e6 * (Al - 0.01) * Cu * (Al - 0.52) * (Cu - 0.48) * (Y - 1)**2 - 5000
fig = ff.create_ternary_contour(np.array([Al, Y, Cu]), enthalpy,
pole_labels=['Al', 'Y', 'Cu'],
interp_mode='cartesian',
ncontours=20,
colorscale='Viridis',
showscale=True,
title='Mixing enthalpy of ternary alloy')
fig.show()
Ternary contour plot with lines only¶
import plotly.figure_factory as ff
import numpy as np
Al = np.array([0. , 0. , 0., 0., 1./3, 1./3, 1./3, 2./3, 2./3, 1.])
Cu = np.array([0., 1./3, 2./3, 1., 0., 1./3, 2./3, 0., 1./3, 0.])
Y = 1 - Al - Cu
# synthetic data for mixing enthalpy
# See https://pycalphad.org/docs/latest/examples/TernaryExamples.html
enthalpy = 2.e6 * (Al - 0.01) * Cu * (Al - 0.52) * (Cu - 0.48) * (Y - 1)**2 - 5000
fig = ff.create_ternary_contour(np.array([Al, Y, Cu]), enthalpy,
pole_labels=['Al', 'Y', 'Cu'],
interp_mode='cartesian',
ncontours=20,
coloring='lines')
fig.show()
Ternary contour plot with data points¶
With showmarkers=True
, data points used to compute the contours are also displayed. They are best visualized for contour lines (no solid coloring). At the moment data points lying on the edges of the diagram are not displayed, this will be improved in future versions.
import plotly.figure_factory as ff
import numpy as np
Al, Cu = np.mgrid[0:1:7j, 0:1:7j]
Al, Cu = Al.ravel(), Cu.ravel()
mask = Al + Cu <= 1
Al, Cu = Al[mask], Cu[mask]
Y = 1 - Al - Cu
enthalpy = (Al - 0.5) * (Cu - 0.5) * (Y - 1)**2
fig = ff.create_ternary_contour(np.array([Al, Y, Cu]), enthalpy,
pole_labels=['Al', 'Y', 'Cu'],
ncontours=20,
coloring='lines',
showmarkers=True)
fig.show()
Interpolation mode¶
Two modes are available in order to interpolate between data points: interpolation in Cartesian space (interp_mode='cartesian'
) or interpolation using the isometric log-ratio transformation (see also preprint), interp_mode='ilr'
. The ilr
transformation preserves metrics in the simplex but is not defined on its edges.
a, b = np.mgrid[0:1:20j, 0:1:20j]
mask = a + b <= 1
a, b = a[mask], b[mask]
coords = np.stack((a, b, 1 - a - b))
value = np.sin(3.2 * np.pi * (a + b)) + np.sin(3 * np.pi * (a - b))
fig = ff.create_ternary_contour(coords, value, ncontours=9)
fig.show()
a, b = np.mgrid[0:1:20j, 0:1:20j]
mask = a + b <= 1
a, b = a[mask], b[mask]
coords = np.stack((a, b, 1 - a - b))
value = np.sin(3.2 * np.pi * (a + b)) + np.sin(3 * np.pi * (a - b))
fig = ff.create_ternary_contour(coords, value, interp_mode='cartesian',
ncontours=9)
fig.show()
Reference¶
For more info on ff.create_ternary_contour()
, see the full function reference
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
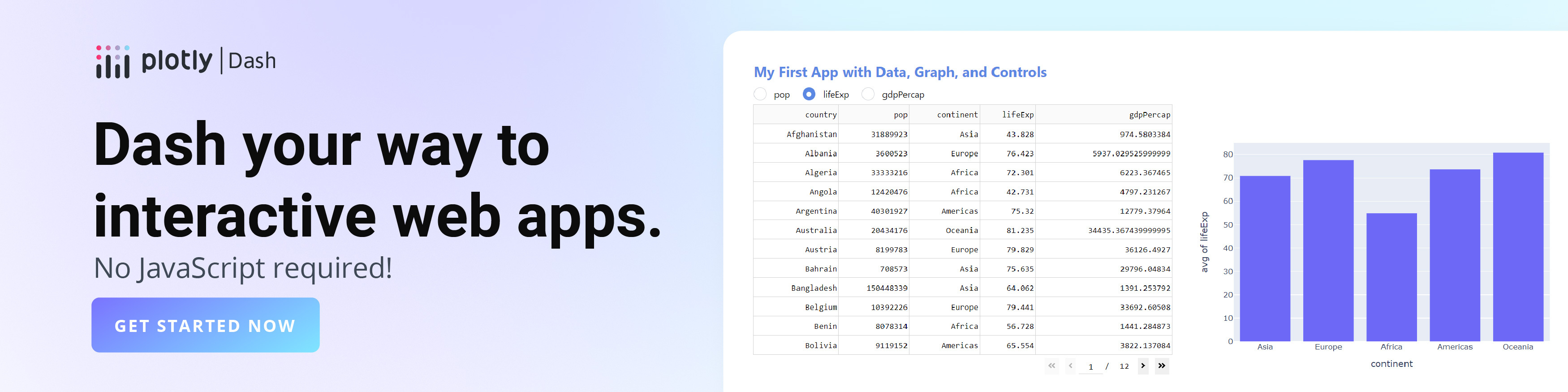