Sunburst Charts in Python
How to make Sunburst Charts.
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Sunburst plots visualize hierarchical data spanning outwards radially from root to leaves. Similar to Icicle charts and Treemaps, the hierarchy is defined by labels
(names
for px.icicle
) and parents
attributes. The root starts from the center and children are added to the outer rings.
Basic Sunburst Plot with plotly.express¶
Plotly Express is the easy-to-use, high-level interface to Plotly, which operates on a variety of types of data and produces easy-to-style figures.
With px.sunburst
, each row of the DataFrame is represented as a sector of the sunburst.
import plotly.express as px
data = dict(
character=["Eve", "Cain", "Seth", "Enos", "Noam", "Abel", "Awan", "Enoch", "Azura"],
parent=["", "Eve", "Eve", "Seth", "Seth", "Eve", "Eve", "Awan", "Eve" ],
value=[10, 14, 12, 10, 2, 6, 6, 4, 4])
fig = px.sunburst(
data,
names='character',
parents='parent',
values='value',
)
fig.show()
Sunburst of a rectangular DataFrame with plotly.express¶
Hierarchical data are often stored as a rectangular dataframe, with different columns corresponding to different levels of the hierarchy. px.sunburst
can take a path
parameter corresponding to a list of columns. Note that id
and parent
should not be provided if path
is given.
import plotly.express as px
df = px.data.tips()
fig = px.sunburst(df, path=['day', 'time', 'sex'], values='total_bill')
fig.show()
Sunburst of a rectangular DataFrame with continuous color argument in px.sunburst¶
If a color
argument is passed, the color of a node is computed as the average of the color values of its children, weighted by their values.
import plotly.express as px
import numpy as np
df = px.data.gapminder().query("year == 2007")
fig = px.sunburst(df, path=['continent', 'country'], values='pop',
color='lifeExp', hover_data=['iso_alpha'],
color_continuous_scale='RdBu',
color_continuous_midpoint=np.average(df['lifeExp'], weights=df['pop']))
fig.show()
Sunburst of a rectangular DataFrame with discrete color argument in px.sunburst¶
When the argument of color
corresponds to non-numerical data, discrete colors are used. If a sector has the same value of the color
column for all its children, then the corresponding color is used, otherwise the first color of the discrete color sequence is used.
import plotly.express as px
df = px.data.tips()
fig = px.sunburst(df, path=['sex', 'day', 'time'], values='total_bill', color='day')
fig.show()
In the example below the color of Saturday
and Sunday
sectors is the same as Dinner
because there are only Dinner entries for Saturday and Sunday. However, for Female -> Friday there are both lunches and dinners, hence the "mixed" color (blue here) is used.
import plotly.express as px
df = px.data.tips()
fig = px.sunburst(df, path=['sex', 'day', 'time'], values='total_bill', color='time')
fig.show()
Using an explicit mapping for discrete colors¶
For more information about discrete colors, see the dedicated page.
import plotly.express as px
df = px.data.tips()
fig = px.sunburst(df, path=['sex', 'day', 'time'], values='total_bill', color='time',
color_discrete_map={'(?)':'black', 'Lunch':'gold', 'Dinner':'darkblue'})
fig.show()
Rectangular data with missing values¶
If the dataset is not fully rectangular, missing values should be supplied as None
. Note that the parents of None
entries must be a leaf, i.e. it cannot have other children than None
(otherwise a ValueError
is raised).
import plotly.express as px
import pandas as pd
vendors = ["A", "B", "C", "D", None, "E", "F", "G", "H", None]
sectors = ["Tech", "Tech", "Finance", "Finance", "Other",
"Tech", "Tech", "Finance", "Finance", "Other"]
regions = ["North", "North", "North", "North", "North",
"South", "South", "South", "South", "South"]
sales = [1, 3, 2, 4, 1, 2, 2, 1, 4, 1]
df = pd.DataFrame(
dict(vendors=vendors, sectors=sectors, regions=regions, sales=sales)
)
print(df)
fig = px.sunburst(df, path=['regions', 'sectors', 'vendors'], values='sales')
fig.show()
Basic Sunburst Plot with go.Sunburst¶
If Plotly Express does not provide a good starting point, it is also possible to use the more generic go.Sunburst
class from plotly.graph_objects
.
Main arguments:
labels
(names
inpx.sunburst
sincelabels
is reserved for overriding columns names): sets the labels of sunburst sectors.parents
: sets the parent sectors of sunburst sectors. An empty string''
is used for the root node in the hierarchy. In this example, the root is "Eve".values
: sets the values associated with sunburst sectors, determining their width (See thebranchvalues
section below for different modes for setting the width).
import plotly.graph_objects as go
fig =go.Figure(go.Sunburst(
labels=["Eve", "Cain", "Seth", "Enos", "Noam", "Abel", "Awan", "Enoch", "Azura"],
parents=["", "Eve", "Eve", "Seth", "Seth", "Eve", "Eve", "Awan", "Eve" ],
values=[10, 14, 12, 10, 2, 6, 6, 4, 4],
))
# Update layout for tight margin
# See https://plotly.com/python/creating-and-updating-figures/
fig.update_layout(margin = dict(t=0, l=0, r=0, b=0))
fig.show()
Sunburst with Repeated Labels¶
import plotly.graph_objects as go
fig =go.Figure(go.Sunburst(
ids=[
"North America", "Europe", "Australia", "North America - Football", "Soccer",
"North America - Rugby", "Europe - Football", "Rugby",
"Europe - American Football","Australia - Football", "Association",
"Australian Rules", "Autstralia - American Football", "Australia - Rugby",
"Rugby League", "Rugby Union"
],
labels= [
"North<br>America", "Europe", "Australia", "Football", "Soccer", "Rugby",
"Football", "Rugby", "American<br>Football", "Football", "Association",
"Australian<br>Rules", "American<br>Football", "Rugby", "Rugby<br>League",
"Rugby<br>Union"
],
parents=[
"", "", "", "North America", "North America", "North America", "Europe",
"Europe", "Europe","Australia", "Australia - Football", "Australia - Football",
"Australia - Football", "Australia - Football", "Australia - Rugby",
"Australia - Rugby"
],
))
fig.update_layout(margin = dict(t=0, l=0, r=0, b=0))
fig.show()
Branchvalues¶
With branchvalues "total", the value of the parent represents the width of its wedge. In the example below, "Enoch" is 4 and "Awan" is 6 and so Enoch's width is 4/6ths of Awans. With branchvalues "remainder", the parent's width is determined by its own value plus those of its children. So, Enoch's width is 4/10ths of Awan's (4 / (6 + 4)).
Note that this means that the sum of the values of the children cannot exceed the value of their parent when branchvalues is set to "total". When branchvalues is set to "remainder" (the default), children will not take up all of the space below their parent (unless the parent is the root and it has a value of 0).
import plotly.graph_objects as go
fig =go.Figure(go.Sunburst(
labels=[ "Eve", "Cain", "Seth", "Enos", "Noam", "Abel", "Awan", "Enoch", "Azura"],
parents=["", "Eve", "Eve", "Seth", "Seth", "Eve", "Eve", "Awan", "Eve" ],
values=[ 65, 14, 12, 10, 2, 6, 6, 4, 4],
branchvalues="total",
))
fig.update_layout(margin = dict(t=0, l=0, r=0, b=0))
fig.show()
Large Number of Slices¶
This example uses a plotly grid attribute for the subplots. Reference the row and column destination using the domain attribute.
import plotly.graph_objects as go
import pandas as pd
df1 = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/718417069ead87650b90472464c7565dc8c2cb1c/sunburst-coffee-flavors-complete.csv')
df2 = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/718417069ead87650b90472464c7565dc8c2cb1c/coffee-flavors.csv')
fig = go.Figure()
fig.add_trace(go.Sunburst(
ids=df1.ids,
labels=df1.labels,
parents=df1.parents,
domain=dict(column=0)
))
fig.add_trace(go.Sunburst(
ids=df2.ids,
labels=df2.labels,
parents=df2.parents,
domain=dict(column=1),
maxdepth=2
))
fig.update_layout(
grid= dict(columns=2, rows=1),
margin = dict(t=0, l=0, r=0, b=0)
)
fig.show()
Controlling text orientation inside sunburst sectors¶
The insidetextorientation
attribute controls the orientation of text inside sectors. With
"auto" the texts may automatically be rotated to fit with the maximum size inside the slice. Using "horizontal" (resp. "radial", "tangential") forces text to be horizontal (resp. radial or tangential). Note that plotly
may reduce the font size in order to fit the text with the requested orientation.
For a figure fig
created with plotly express, use fig.update_traces(insidetextorientation='...')
to change the text orientation.
import plotly.graph_objects as go
import pandas as pd
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/718417069ead87650b90472464c7565dc8c2cb1c/coffee-flavors.csv')
fig = go.Figure()
fig.add_trace(go.Sunburst(
ids=df.ids,
labels=df.labels,
parents=df.parents,
domain=dict(column=1),
maxdepth=2,
insidetextorientation='radial'
))
fig.update_layout(
margin = dict(t=10, l=10, r=10, b=10)
)
fig.show()
Controlling text fontsize with uniformtext¶
If you want all the text labels to have the same size, you can use the uniformtext
layout parameter. The minsize
attribute sets the font size, and the mode
attribute sets what happens for labels which cannot fit with the desired fontsize: either hide
them or show
them with overflow.
Note: animated transitions are currently not implemented when uniformtext
is used.
import plotly.graph_objects as go
import pandas as pd
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/718417069ead87650b90472464c7565dc8c2cb1c/sunburst-coffee-flavors-complete.csv')
fig = go.Figure(go.Sunburst(
ids = df.ids,
labels = df.labels,
parents = df.parents))
fig.update_layout(uniformtext=dict(minsize=10, mode='hide'))
fig.show()
import plotly.graph_objects as go
fig = go.Figure(
go.Sunburst(
labels=["Eve", "Cain", "Seth", "Enos", "Noam", "Abel", "Awan", "Enoch", "Azura"],
parents=["", "Eve", "Eve", "Seth", "Seth", "Eve", "Eve", "Awan", "Eve"],
values=[65, 14, 12, 10, 2, 6, 6, 4, 4],
branchvalues="total",
textfont_size=16,
marker=dict(
pattern=dict(
shape=["", "/", "/", ".", ".", "/", "/", ".", "/"], solidity=0.9
)
),
)
)
fig.update_layout(margin=dict(t=0, l=0, r=0, b=0))
fig.show()
Sunburst chart with a continuous colorscale¶
The example below visualizes a breakdown of sales (corresponding to sector width) and call success rate (corresponding to sector color) by region, county and salesperson level. For example, when exploring the data you can see that although the East region is behaving poorly, the Tyler county is still above average -- however, its performance is reduced by the poor success rate of salesperson GT.
In the right subplot which has a maxdepth
of two levels, click on a sector to see its breakdown to lower levels.
import plotly.graph_objects as go
from plotly.subplots import make_subplots
import pandas as pd
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/master/sales_success.csv')
print(df.head())
levels = ['salesperson', 'county', 'region'] # levels used for the hierarchical chart
color_columns = ['sales', 'calls']
value_column = 'calls'
def build_hierarchical_dataframe(df, levels, value_column, color_columns=None):
"""
Build a hierarchy of levels for Sunburst or Treemap charts.
Levels are given starting from the bottom to the top of the hierarchy,
ie the last level corresponds to the root.
"""
df_list = []
for i, level in enumerate(levels):
df_tree = pd.DataFrame(columns=['id', 'parent', 'value', 'color'])
dfg = df.groupby(levels[i:]).sum()
dfg = dfg.reset_index()
df_tree['id'] = dfg[level].copy()
if i < len(levels) - 1:
df_tree['parent'] = dfg[levels[i+1]].copy()
else:
df_tree['parent'] = 'total'
df_tree['value'] = dfg[value_column]
df_tree['color'] = dfg[color_columns[0]] / dfg[color_columns[1]]
df_list.append(df_tree)
total = pd.Series(dict(id='total', parent='',
value=df[value_column].sum(),
color=df[color_columns[0]].sum() / df[color_columns[1]].sum()), name=0)
df_list.append(total)
df_all_trees = pd.concat(df_list, ignore_index=True)
return df_all_trees
df_all_trees = build_hierarchical_dataframe(df, levels, value_column, color_columns)
average_score = df['sales'].sum() / df['calls'].sum()
fig = make_subplots(1, 2, specs=[[{"type": "domain"}, {"type": "domain"}]],)
fig.add_trace(go.Sunburst(
labels=df_all_trees['id'],
parents=df_all_trees['parent'],
values=df_all_trees['value'],
branchvalues='total',
marker=dict(
colors=df_all_trees['color'],
colorscale='RdBu',
cmid=average_score),
hovertemplate='<b>%{label} </b> <br> Sales: %{value}<br> Success rate: %{color:.2f}',
name=''
), 1, 1)
fig.add_trace(go.Sunburst(
labels=df_all_trees['id'],
parents=df_all_trees['parent'],
values=df_all_trees['value'],
branchvalues='total',
marker=dict(
colors=df_all_trees['color'],
colorscale='RdBu',
cmid=average_score),
hovertemplate='<b>%{label} </b> <br> Sales: %{value}<br> Success rate: %{color:.2f}',
maxdepth=2
), 1, 2)
fig.update_layout(margin=dict(t=10, b=10, r=10, l=10))
fig.show()
Reference¶
See function reference for px.sunburst()
or https://plotly.com/python/reference/sunburst/ for more information and chart attribute options!
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
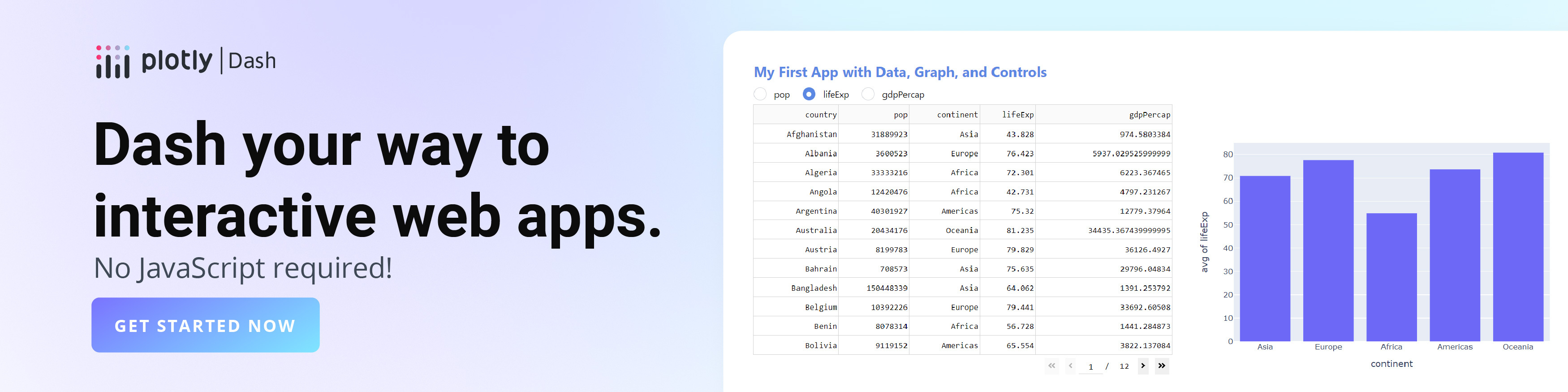