Clustergram in Python
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Default Clustergram¶
A clustergram is a combination heatmap-dendrogram that is commonly used in gene expression data. The hierarchical clustering that is represented by the dendrograms can be used to identify groups of genes with related expression levels. The Dash Bio Clustergram component is a Python-based component that uses plotly.py to generate a figure. It takes as input a two-dimensional numpy array of floating-point values. Imputation of missing data and computation of hierarchical clustering both occur within the component itself. Clusters that meet or exceed a user-defined threshold of similarity comprise single traces in the corresponding dendrogram, and can be highlighted with annotations. The user can specify additional parameters to customize the metrics and methods used to compute parts of the clustering, such as the pairwise distance between observations and the linkage matrix.
import pandas as pd
import dash_bio
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/master/Dash_Bio/Chromosomal/clustergram_brain_cancer.csv')
dash_bio.Clustergram(
data=df,
column_labels=list(df.columns.values),
row_labels=list(df.index),
height=800,
width=700
)
Dendrogram Cluster Colors/Line Widths¶
Change the colors of the dendrogram traces that are used to represent clusters, and configure their line widths.
import pandas as pd
import dash_bio
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/master/Dash_Bio/Chromosomal/clustergram_brain_cancer.csv')
dash_bio.Clustergram(
data=df,
column_labels=list(df.columns.values),
row_labels=list(df.index),
height=800,
width=700,
color_list={
'row': ['#636EFA', '#00CC96', '#19D3F3'],
'col': ['#AB63FA', '#EF553B'],
'bg': '#506784'
},
line_width=2
)
Relative Dendrogram Size¶
Change the relative width and height of, respectively, the row and column dendrograms compared to the width and height of the heatmap.
import pandas as pd
import dash_bio
df = pd.read_csv('https://raw.githubusercontent.com/plotly/datasets/master/Dash_Bio/Chromosomal/clustergram_brain_cancer.csv')
dash_bio.Clustergram(
data=df,
column_labels=list(df.columns.values),
row_labels=list(df.index),
height=800,
width=700,
display_ratio=[0.1, 0.7]
)
Clustergram with Dash¶
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
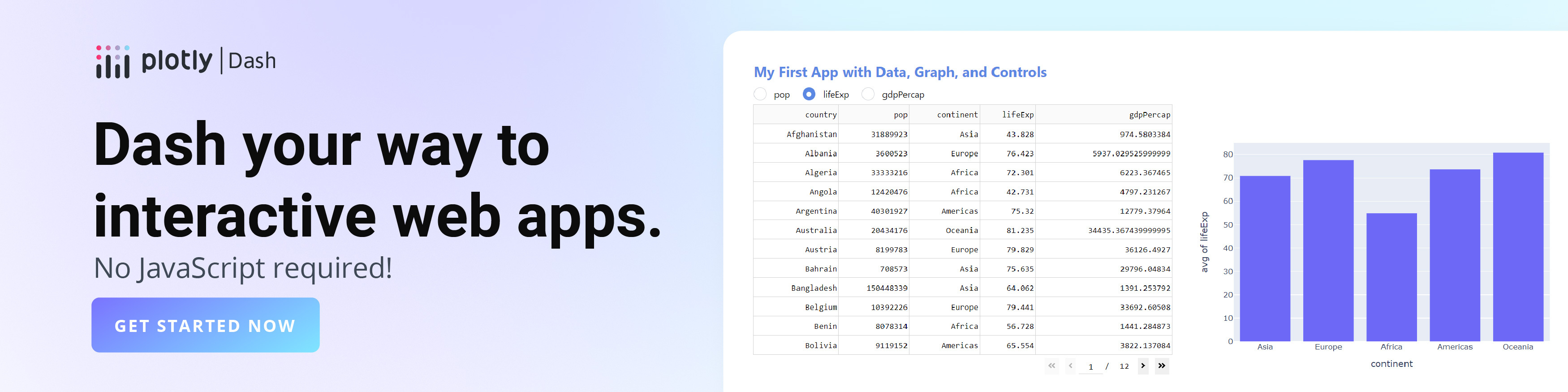