Pie Charts in JavaScript
How to graph D3.js-based pie charts in javascript with D3.js. Examples of pie charts, donut charts and pie chart subplots.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
var data = [{
values: [19, 26, 55],
labels: ['Residential', 'Non-Residential', 'Utility'],
type: 'pie'
}];
var layout = {
height: 400,
width: 500
};
Plotly.newPlot('myDiv', data, layout);
In order to create pie chart subplots, you need to use the domain attribute. domain
allows you to place each trace on a grid of rows and columns defined in the layout or within a rectangle defined by X
and Y
arrays. The example below uses the grid
method (with a 2 x 2 grid defined in the layout) for the first three traces and the X and Y method for the fourth trace.
var allLabels = ['1st', '2nd', '3rd', '4th', '5th'];
var allValues = [
[38, 27, 18, 10, 7],
[28, 26, 21, 15, 10],
[38, 19, 16, 14, 13],
[31, 24, 19, 18, 8]
];
var ultimateColors = [
['rgb(56, 75, 126)', 'rgb(18, 36, 37)', 'rgb(34, 53, 101)', 'rgb(36, 55, 57)', 'rgb(6, 4, 4)'],
['rgb(177, 127, 38)', 'rgb(205, 152, 36)', 'rgb(99, 79, 37)', 'rgb(129, 180, 179)', 'rgb(124, 103, 37)'],
['rgb(33, 75, 99)', 'rgb(79, 129, 102)', 'rgb(151, 179, 100)', 'rgb(175, 49, 35)', 'rgb(36, 73, 147)'],
['rgb(146, 123, 21)', 'rgb(177, 180, 34)', 'rgb(206, 206, 40)', 'rgb(175, 51, 21)', 'rgb(35, 36, 21)']
];
var data = [{
values: allValues[0],
labels: allLabels,
type: 'pie',
name: 'Starry Night',
marker: {
colors: ultimateColors[0]
},
domain: {
row: 0,
column: 0
},
hoverinfo: 'label+percent+name',
textinfo: 'none'
},{
values: allValues[1],
labels: allLabels,
type: 'pie',
name: 'Sunflowers',
marker: {
colors: ultimateColors[1]
},
domain: {
row: 1,
column: 0
},
hoverinfo: 'label+percent+name',
textinfo: 'none'
},{
values: allValues[2],
labels: allLabels,
type: 'pie',
name: 'Irises',
marker: {
colors: ultimateColors[2]
},
domain: {
row: 0,
column: 1
},
hoverinfo: 'label+percent+name',
textinfo: 'none'
},{
values: allValues[3],
labels: allLabels,
type: 'pie',
name: 'The Night Cafe',
marker: {
colors: ultimateColors[3]
},
domain: {
x: [0.52,1],
y: [0, 0.48]
},
hoverinfo: 'label+percent+name',
textinfo: 'none'
}];
var layout = {
height: 400,
width: 500,
grid: {rows: 2, columns: 2}
};
Plotly.newPlot('myDiv', data, layout);
var data = [{
values: [16, 15, 12, 6, 5, 4, 42],
labels: ['US', 'China', 'European Union', 'Russian Federation', 'Brazil', 'India', 'Rest of World' ],
domain: {column: 0},
name: 'GHG Emissions',
hoverinfo: 'label+percent+name',
hole: .4,
type: 'pie'
},{
values: [27, 11, 25, 8, 1, 3, 25],
labels: ['US', 'China', 'European Union', 'Russian Federation', 'Brazil', 'India', 'Rest of World' ],
text: 'CO2',
textposition: 'inside',
domain: {column: 1},
name: 'CO2 Emissions',
hoverinfo: 'label+percent+name',
hole: .4,
type: 'pie'
}];
var layout = {
title: 'Global Emissions 1990-2011',
annotations: [
{
font: {
size: 20
},
showarrow: false,
text: 'GHG',
x: 0.17,
y: 0.5
},
{
font: {
size: 20
},
showarrow: false,
text: 'CO2',
x: 0.82,
y: 0.5
}
],
height: 400,
width: 600,
showlegend: false,
grid: {rows: 1, columns: 2}
};
Plotly.newPlot('myDiv', data, layout);
The following example sets automargin attribute to true, which automatically increases the margin size.
var data = [{
type: "pie",
values: [2, 3, 4, 4],
labels: ["Wages", "Operating expenses", "Cost of sales", "Insurance"],
textinfo: "label+percent",
textposition: "outside",
automargin: true
}]
var layout = {
height: 400,
width: 400,
margin: {"t": 0, "b": 0, "l": 0, "r": 0},
showlegend: false
}
Plotly.newPlot('myDiv', data, layout)
The insidetextorientation
attribute controls the orientation of the text inside chart sectors. When set to auto, text may be oriented in any direction in order to be as big as possible in the middle of a sector. The horizontal option orients text to be parallel with the bottom of the chart, and may make text smaller in order to achieve that goal. The radial option orients text along the radius of the sector. The tangential option orients text perpendicular to the radius of the sector.
var data = [{
type: "pie",
values: [2, 3, 4, 4],
labels: ["Wages", "Operating expenses", "Cost of sales", "Insurance"],
textinfo: "label+percent",
insidetextorientation: "radial"
}]
var layout = [{
height: 700,
width: 700
}]
Plotly.newPlot('myDiv', data, layout)
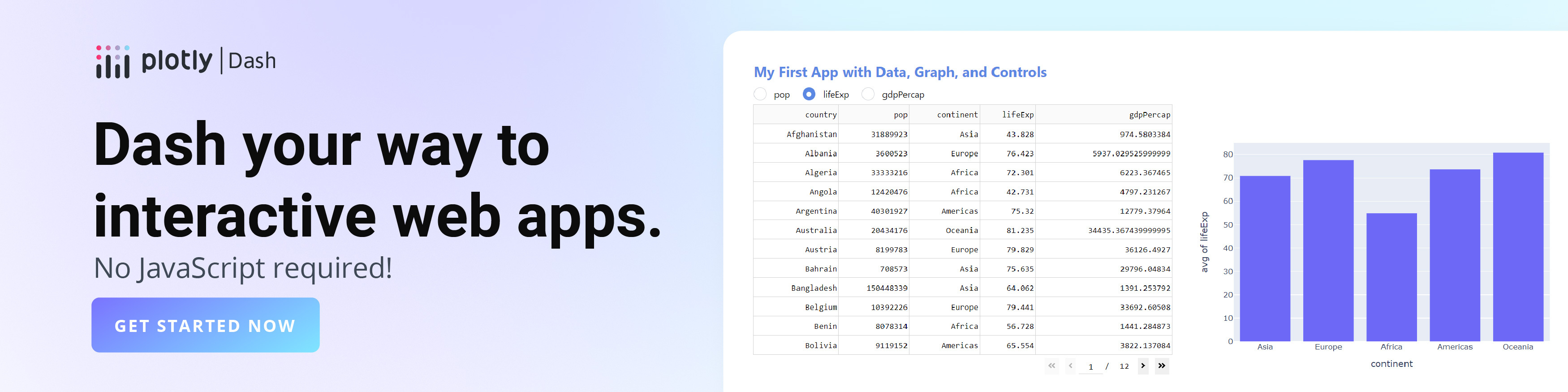