Random Walk in Python/v3
Learn how to use Python to make a Random Walk
See our Version 4 Migration Guide for information about how to upgrade.
The version 4 version of this page is here.
New to Plotly?¶
Plotly's Python library is free and open source! Get started by dowloading the client and reading the primer.
You can set up Plotly to work in online or offline mode, or in jupyter notebooks.
We also have a quick-reference cheatsheet (new!) to help you get started!
import plotly.plotly as py
import plotly.graph_objs as go
from plotly.tools import FigureFactory as FF
import numpy as np
import pandas as pd
import scipy
import random
Tips¶
A random walk
can be thought of as a random process in which a tolken or a marker is randomly moved around some space, that is, a space with a metric used to compute distance. It is more commonly conceptualized in one dimension ($\mathbb{Z}$), two dimensions ($\mathbb{Z}^2$) or three dimensions ($\mathbb{Z}^3$) in Cartesian space, where $\mathbb{Z}$ represents the set of integers. In the visualizations below, we will be using scatter plots as well as a colorscale to denote the time sequence of the walk.
Random Walk in 1D¶
The jitter in the data points along the x and y axes are meant to illuminate where the points are being drawn and what the tendancy of the random walk is.
x = [0]
for j in range(100):
step_x = random.randint(0,1)
if step_x == 1:
x.append(x[j] + 1 + 0.05*np.random.normal())
else:
x.append(x[j] - 1 + 0.05*np.random.normal())
y = [0.05*np.random.normal() for j in range(len(x))]
trace1 = go.Scatter(
x=x,
y=y,
mode='markers',
name='Random Walk in 1D',
marker=dict(
color=[i for i in range(len(x))],
size=7,
colorscale=[[0, 'rgb(178,10,28)'], [0.50, 'rgb(245,160,105)'],
[0.66, 'rgb(245,195,157)'], [1, 'rgb(220,220,220)']],
showscale=True,
)
)
layout = go.Layout(
yaxis=dict(
range=[-1, 1]
)
)
data = [trace1]
fig= go.Figure(data=data, layout=layout)
py.iplot(fig, filename='random-walk-1d')
Random Walk in 2D¶
x = [0]
y = [0]
for j in range(1000):
step_x = random.randint(0,1)
if step_x == 1:
x.append(x[j] + 1 + np.random.normal())
else:
x.append(x[j] - 1 + np.random.normal())
step_y = random.randint(0,1)
if step_y == 1:
y.append(y[j] + 1 + np.random.normal())
else:
y.append(y[j] - 1 + np.random.normal())
trace1 = go.Scatter(
x=x,
y=y,
mode='markers',
name='Random Walk',
marker=dict(
color=[i for i in range(len(x))],
size=8,
colorscale='Greens',
showscale=True
)
)
data = [trace1]
py.iplot(data, filename='random-walk-2d')
Advanced Tip¶
We can formally think of a 1D random walk as a point jumping along the integer number line. Let $Z_i$ be a random variable that takes on the values +1 and -1. Let this random variable represent the steps we take in the random walk in 1D (where +1 means right and -1 means left). Also, as with the above visualizations, let us assume that the probability of moving left and right is just $\frac{1}{2}$. Then, consider the sum
$$ \begin{align*} S_n = \sum_{i=0}^{n}{Z_i} \end{align*} $$where S_n represents the point that the random walk ends up on after n steps have been taken.
To find the expected value
of $S_n$, we can compute it directly. Since each $Z_i$ is independent, we have
but since $Z_i$ takes on the values +1 and -1 then
$$ \begin{align*} \mathbb{E}(Z_i) = 1 \cdot P(Z_i=1) + -1 \cdot P(Z_i=-1) = \frac{1}{2} - \frac{1}{2} = 0 \end{align*} $$Therefore, we expect our random walk to hover around $0$ regardless of how many steps we take in our walk.
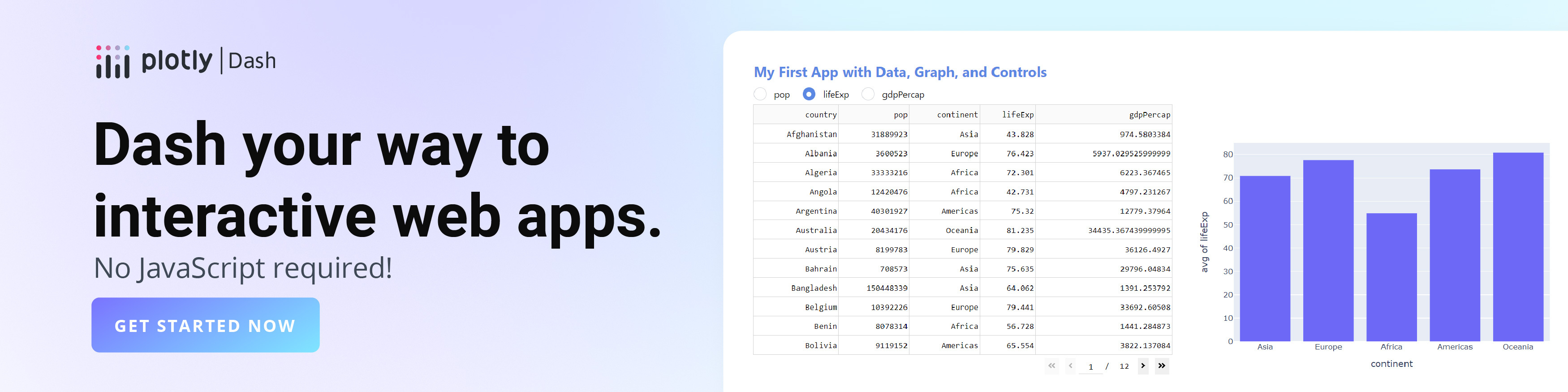