1D Correlation in Python/v3
Learn how to perform 1 dimensional correlation between two signals in Python.
See our Version 4 Migration Guide for information about how to upgrade.
New to Plotly?¶
Plotly's Python library is free and open source! Get started by downloading the client and reading the primer.
You can set up Plotly to work in online or offline mode, or in jupyter notebooks.
We also have a quick-reference cheatsheet (new!) to help you get started!
import plotly.plotly as py
import plotly.graph_objs as go
import numpy as np
import pandas as pd
import scipy
from scipy import signal
Correlation Between Saw and Square Wave¶
Similar to convolution, the 1D Cross-Correlation between two functions $f$ and $g$ is a measure of their similarity in terms of the lag of one to another (source). Since we are dealing with arrays of data rather than continuous functions, the cross-correlation is mathematically defined as:
$$ \begin{align*} (f*g)[n] = \sum_{m=-\infty}^{\infty} f^{*}[m]g[n+m] \end{align*} $$where $f^*$ is the complex conjugate
of $f$.
To give us an intuitive glance at what this does, let us look at the cross-correlation between a saw wave and a square wave.
x = np.linspace(0, 20, 50)
saw_y = signal.sawtooth(t=x)
square_y = signal.square(t=x)
convolve_y = signal.convolve(saw_y, square_y)
trace_saw = go.Scatter(
x = x,
y = saw_y,
mode = 'lines',
name = 'Saw',
marker=dict(
color='#57D1C9'
)
)
trace_square = go.Scatter(
x = x,
y = square_y,
mode = 'lines',
name = 'Square',
marker=dict(
color='#ED5485'
)
)
trace_convolution = go.Scatter(
x = x,
y = convolve_y,
mode = 'lines',
name = 'Convolution',
marker=dict(
color='#FFE869'
)
)
data = [trace_saw, trace_square, trace_convolution]
py.iplot(data, filename='1d-convolution-of-saw-and-square')
Correlation Between Saw and Shifted Saw Wave¶
To compare with the plot above, we can plot a saw wave, a phase shifted saw wave and the convolution between the two to see how they correlate along the axis.
x = np.linspace(0, 20, 50)
saw_y = signal.sawtooth(t=x)
square_y = signal.square(t=x)
shifted_saw_y = signal.sawtooth(t=np.linspace(10, 30, 50))
convolve_y = signal.convolve(saw_y, shifted_saw_y)
trace_saw = go.Scatter(
x = x,
y = saw_y,
mode = 'lines',
name = 'Saw',
marker = dict(
color='#FF7844'
),
opacity = 0.8
)
trace_shifted_saw = go.Scatter(
x = x,
y = shifted_saw_y,
mode = 'lines',
name = 'Shifted Saw',
marker = dict(
color='#A64942'
),
opacity = 0.8
)
trace_convolution = go.Scatter(
x = x,
y = convolve_y,
mode = 'lines',
name = 'Convolution',
marker = dict(
color='#53354A'
)
)
data = [trace_saw, trace_shifted_saw, trace_convolution]
py.iplot(data, filename='1d-convolution-of-saw-and-shifted-saw')
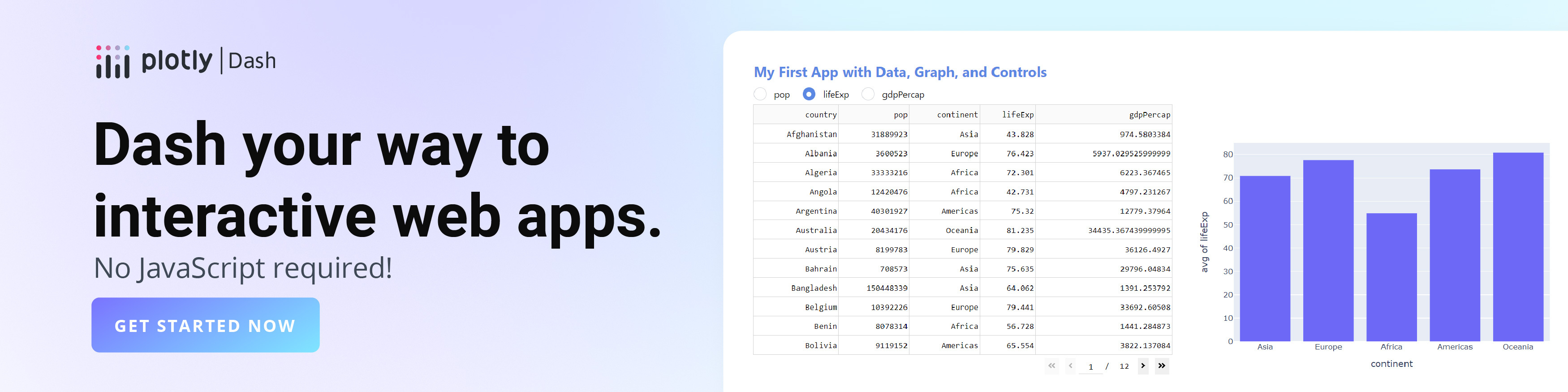