Pandas Plotting Backend in Python
Plotly Express can be used as a Pandas .plot() backend.
New to Plotly?
Plotly is a free and open-source graphing library for Python. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Introduction¶
The popular Pandas data analysis and manipulation tool provides plotting functions on its DataFrame
and Series
objects, which have historically produced matplotlib
plots. Since version 0.25, Pandas has provided a mechanism to use different backends, and as of version 4.8 of plotly
, you can now use a Plotly Express-powered backend for Pandas plotting. This means you can now produce interactive plots directly from a data frame, without even needing to import Plotly.
To activate this backend, you will need to have Plotly installed, and then just need to set pd.options.plotting.backend
to "plotly"
and call .plot()
to get a plotly.graph_objects.Figure
object back, just like if you had called Plotly Express directly:
import pandas as pd
pd.options.plotting.backend = "plotly"
df = pd.DataFrame(dict(a=[1,3,2], b=[3,2,1]))
fig = df.plot()
fig.show()
This functionality wraps Plotly Express and so you can use any of the styling options available to Plotly Express methods. Since what you get back is a regular Figure
object, you can use any of the update mechanisms supported by these objects to apply templates or further customize axes, colors, legends, fonts, hover labels etc. Faceting is also supported.
import pandas as pd
pd.options.plotting.backend = "plotly"
df = pd.DataFrame(dict(a=[1,3,2], b=[3,2,1]))
fig = df.plot(title="Pandas Backend Example", template="simple_white",
labels=dict(index="time", value="money", variable="option"))
fig.update_yaxes(tickprefix="$")
fig.show()
A Note on API Compatibility¶
The Plotly plotting backend for Pandas is not intended to be a drop-in replacement for the default; it does not implement all or even most of the same keyword arguments, such as
subplots=True
etc.
The Plotly plotting backend for Pandas is a more convenient way to invoke certain Plotly Express functions by chaining a .plot()
call without having to import Plotly Express directly. Plotly Express, as of version 4.8 with wide-form data support in addition to its robust long-form data support, implements behaviour for the x
and y
keywords that are very similar to the matplotlib
backend.
In practice, this means that the following two ways of making a chart are identical and support the same additional arguments, because they call the same underlying code:
import pandas as pd
pd.options.plotting.backend = "plotly"
df = pd.DataFrame(dict(a=[1,3,2], b=[3,2,1]))
# using Plotly Express via the Pandas backend
fig1 = df.plot.bar()
fig1.show()
# using Plotly Express directly
import plotly.express as px
fig2 = px.bar(df)
fig2.show()
To achieve a similar effect to subplots=True
, for example, the Plotly Express facet_row
and facet_col
options can be used, the same was as they work when directly calling Plotly Express with wide-form data:
import pandas as pd
pd.options.plotting.backend = "plotly"
df = pd.DataFrame(dict(a=[1,3,2], b=[3,2,1]))
fig = df.plot.bar(facet_row="variable")
fig.show()
Supported Methods¶
The Plotly backend supports the following kind
s of Pandas plots: scatter
, line
, area
, bar
, barh
, hist
and box
, via the call pattern df.plot(kind='scatter')
or df.plot.scatter()
. These delegate to the corresponding Plotly Express functions. In addition, the following are valid options to the kind
argument of df.plot()
: violin
, strip
, funnel
, density_heatmap
, density_contour
and imshow
, even though the call pattern df.plot.violin()
is not supported for these kinds of charts, per the Pandas API.
import pandas as pd
import numpy as np
pd.options.plotting.backend = "plotly"
np.random.seed(1)
df = pd.DataFrame(dict(
a=np.random.normal(loc=1, scale=2, size=100),
b=np.random.normal(loc=2, scale=1, size=100)
))
fig = df.plot.scatter(x="a", y="b")
fig.show()
import pandas as pd
pd.options.plotting.backend = "plotly"
df = pd.DataFrame(dict(a=[1,3,2], b=[3,2,1]))
fig = df.plot.line()
fig.show()
import pandas as pd
pd.options.plotting.backend = "plotly"
df = pd.DataFrame(dict(a=[1,3,2], b=[3,2,1]))
fig = df.plot.area()
fig.show()
import pandas as pd
pd.options.plotting.backend = "plotly"
df = pd.DataFrame(dict(a=[1,3,2], b=[3,2,1]))
fig = df.plot.bar()
fig.show()
import pandas as pd
pd.options.plotting.backend = "plotly"
df = pd.DataFrame(dict(a=[1,3,2], b=[3,2,1]))
fig = df.plot.barh()
fig.show()
import pandas as pd
import numpy as np
pd.options.plotting.backend = "plotly"
np.random.seed(1)
df = pd.DataFrame(dict(
a=np.random.normal(loc=1, scale=2, size=100),
b=np.random.normal(loc=2, scale=1, size=100)
))
fig = df.plot.hist()
fig.show()
import pandas as pd
import numpy as np
pd.options.plotting.backend = "plotly"
np.random.seed(1)
df = pd.DataFrame(dict(
a=np.random.normal(loc=1, scale=2, size=100),
b=np.random.normal(loc=2, scale=1, size=100)
))
fig = df.plot.box()
fig.show()
Series
and DataFrame
functions: hist
and boxplot
¶
The Pandas plotting API also exposes .hist()
on DataFrame
s and Series
objects, and .boxplot()
on DataFrames
, which can also be used with the Plotly backend.
import pandas as pd
import numpy as np
pd.options.plotting.backend = "plotly"
np.random.seed(1)
df = pd.DataFrame(dict(
a=np.random.normal(loc=1, scale=2, size=100),
b=np.random.normal(loc=2, scale=1, size=100)
))
fig = df.boxplot()
fig.show()
What about Cufflinks?¶
There also exists an independent third-party wrapper library around Plotly called Cufflinks, which provides similar functionality (with an API closer to that of Pandas' default matplotlib
backend) by adding a .iplot()
method to Pandas dataframes, as it was developed before Pandas supported configurable backends. Issues and questions regarding Cufflinks should be raised in the Cufflinks repository.
What About Dash?¶
Dash is an open-source framework for building analytical applications, with no Javascript required, and it is tightly integrated with the Plotly graphing library.
Learn about how to install Dash at https://dash.plot.ly/installation.
Everywhere in this page that you see fig.show()
, you can display the same figure in a Dash application by passing it to the figure
argument of the Graph
component from the built-in dash_core_components
package like this:
import plotly.graph_objects as go # or plotly.express as px
fig = go.Figure() # or any Plotly Express function e.g. px.bar(...)
# fig.add_trace( ... )
# fig.update_layout( ... )
from dash import Dash, dcc, html
app = Dash()
app.layout = html.Div([
dcc.Graph(figure=fig)
])
app.run_server(debug=True, use_reloader=False) # Turn off reloader if inside Jupyter
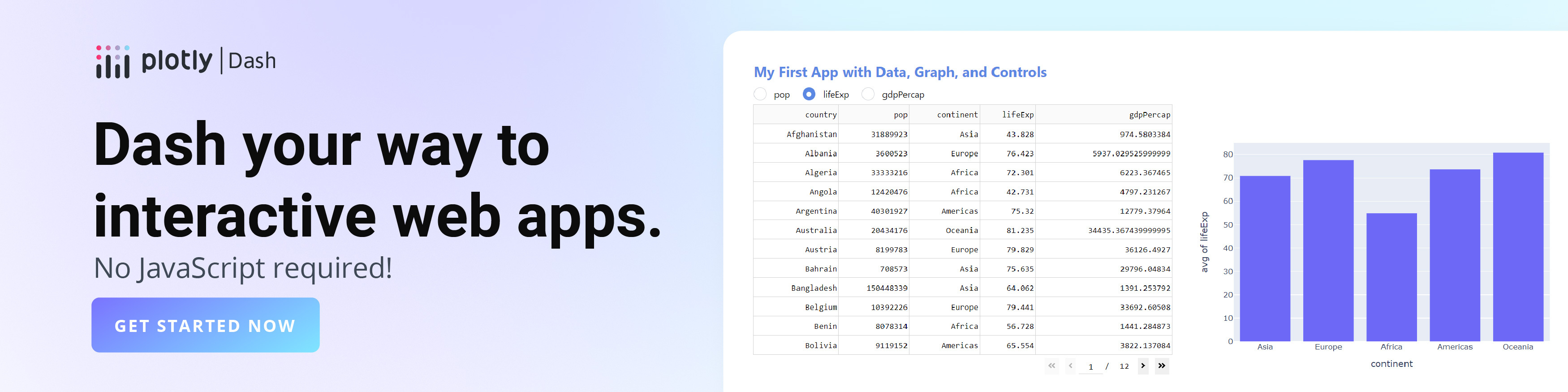