Waterfall Charts in JavaScript
How to make a D3.js-based waterfall chart in javascript.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
var data = [
{
name: "2018",
type: "waterfall",
orientation: "v",
measure: [
"relative",
"relative",
"total",
"relative",
"relative",
"total"
],
x: [
"Sales",
"Consulting",
"Net revenue",
"Purchases",
"Other expenses",
"Profit before tax"
],
textposition: "outside",
text: [
"+60",
"+80",
"",
"-40",
"-20",
"Total"
],
y: [
60,
80,
0,
-40,
-20,
0
],
connector: {
line: {
color: "rgb(63, 63, 63)"
}
},
}
];
layout = {
title: {
text: "Profit and loss statement 2018"
},
xaxis: {
type: "category"
},
yaxis: {
type: "linear"
},
autosize: true,
showlegend: true
};
Plotly.newPlot('myDiv', data, layout);
var gd = document.getElementById('myDiv');
var data = [
{
type: "waterfall",
x: [
["2016", "2017", "2017", "2017", "2017", "2018", "2018", "2018", "2018"],
["initial", "q1", "q2", "q3", "total", "q1", "q2", "q3", "total" ]
],
measure: ["absolute", "relative", "relative", "relative", "total", "relative", "relative", "relative", "total"],
y: [1, 2, 3, -1, null, 1, 2, -4, null],
base: 1000
},
{
type: "waterfall",
x: [
["2016", "2017", "2017", "2017", "2017", "2018", "2018", "2018", "2018"],
["initial", "q1", "q2", "q3", "total", "q1", "q2", "q3", "total" ]
],
measure: ["absolute", "relative", "relative", "relative", "total", "relative", "relative", "relative", "total"],
y: [1.1, 2.2, 3.3, -1.1, null, 1.1, 2.2, -4.4, null],
base: 1000
}
];
var layout = {
waterfallgroupgap : 0.5,
xaxis: {
title: "MULTI-CATEGORY",
tickfont: {size: 16},
ticks: "outside"
}
}
Plotly.newPlot('myDiv', data, layout);
var gd = document.getElementById('myDiv');
var data = [
{
name: "2018",
type: "waterfall",
orientation: "h",
measure: [
"relative",
"relative",
"relative",
"relative",
"total",
"relative",
"relative",
"relative",
"relative",
"total",
"relative",
"relative",
"total",
"relative",
"total"
],
y: [
"Sales",
"Consulting",
"Maintenance",
"Other revenue",
"Net revenue",
"Purchases",
"Material expenses",
"Personnel expenses",
"Other expenses",
"Operating profit",
"Investment income",
"Financial income",
"Profit before tax",
"Income tax (15%)",
"Profit after tax"
],
x: [
375,
128,
78,
27,
null,
-327,
-12,
-78,
-12,
null,
32,
89,
null,
-45,
null
],
connector: {
mode: "between",
line: {
width: 4,
color: "rgb(0, 0, 0)",
dash: 0
}
}
}
];
var layout = {title: {
text: "Profit and loss statement 2018<br>waterfall chart displaying positive and negative"
},
yaxis: {
type: "category",
autorange: "reversed"
},
xaxis: {
type: "linear"
},
margin: { l: 150 },
showlegend: true
}
Plotly.newPlot('myDiv', data, layout);
var gd = document.getElementById('myDiv');
var data = [
{
type: "waterfall",
x: [
["2016", "2017", "2017", "2017", "2017", "2018", "2018", "2018", "2018"],
["initial", "q1", "q2", "q3", "total", "q1", "q2", "q3", "total" ]
],
measure: ["absolute", "relative", "relative", "relative", "total", "relative", "relative", "relative", "total"],
y: [10, 20, 30, -10, null, 10, 20, -40, null],
base: 300,
decreasing: { marker: { color: "Maroon" , line:{color : "red", width :2}}},
increasing: { marker: { color: "Teal"} },
totals: { marker: { color: "deep sky blue", line:{color:'blue',width:3}} }
}];
var layout = {title: {
text: "Profit and loss statement"
},
waterfallgap : 0.3,
xaxis: {
title: "",
tickfont: {size: 15},
ticks: "outside"
}
}
Plotly.newPlot('myDiv', data, layout);
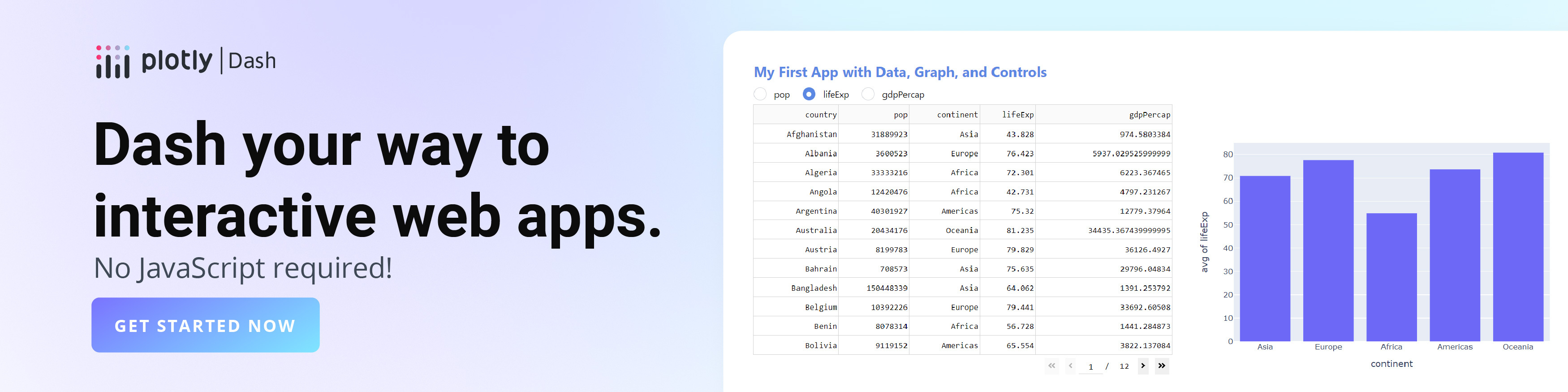