Tile Density Heatmap in JavaScript
How to make a tile-based density heatmap in JavaScript. A density heatmap uses a variable binding expression to display population density.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
var data = [
{type: "densitymap", lon: [10, 20, 30], lat: [15, 25, 35], z: [1, 3, 2],
radius: 50, colorbar: {y: 1, yanchor: 'top', len: 0.45}},
{type: 'densitymap', lon: [-10, -20, -30], lat: [15, 25, 35],
radius: [50, 100, 10], colorbar: {y: 0, yanchor: 'bottom', len: 0.45}
}];
var layout = {map: {style: 'light', center: {lat: 20}}, width: 600, height: 400};
Plotly.newPlot('myDiv', data, layout);
Click to copy
© CARTO, © OpenStreetMap contributors
d3.csv('https://raw.githubusercontent.com/plotly/datasets/master/earthquakes-23k.csv',
function(err, rows){function unpack(rows, key) {return rows.map(function(row){ return row[key];
})};
var data = [{
lon: unpack(rows, 'Longitude'), lat: unpack(rows, 'Latitude'), radius:10,
z: unpack(rows, 'Magnitude'), type: "densitymap", coloraxis: 'coloraxis',
hoverinfo: 'skip'}];
var layout = {
map: {center: {lon: 60, lat: 30}, style: "outdoors", zoom: 2},
coloraxis: {colorscale: "Viridis"}, title: {text: "Earthquake Magnitude"},
width: 600, height: 400, margin: {t: 30, b: 0}};
Plotly.newPlot('myDiv', data, layout);
})
Click to copy
var data = [{type: 'densitymapbox', lon: [10, 20, 30], lat: [15, 25, 35], z: [1, 3, 2]}];
var layout = {width: 600, height: 400, mapbox: {style: 'https://tiles.stadiamaps.com/styles/stamen_watercolor.json?api_key=YOUR-API-KEY'}};
Plotly.newPlot('myDiv', data, layout);
Click to copy
Mapbox traces are deprecated and may be removed in a future version of Plotly.js.
Earlier examples use traces that render with Maplibre GL JS.
These traces were introduced in Plotly.js 2.35.0 and replace Mapbox-based tile maps,
which are now deprecated. Here's one of the earlier examples written using the Mapbox-based densitymapbox
trace.
var data = [
{type: "densitymapbox", lon: [10, 20, 30], lat: [15, 25, 35], z: [1, 3, 2],
radius: 50, colorbar: {y: 1, yanchor: 'top', len: 0.45}},
{type: 'densitymapbox', lon: [-10, -20, -30], lat: [15, 25, 35],
radius: [50, 100, 10], colorbar: {y: 0, yanchor: 'bottom', len: 0.45}
}];
var layout = {mapbox: {style: 'light', center: {lat: 20}}, width: 600, height: 400};
var config = {mapboxAccessToken: "your access token"};
Plotly.newPlot('myDiv', data, layout, config);
Click to copy
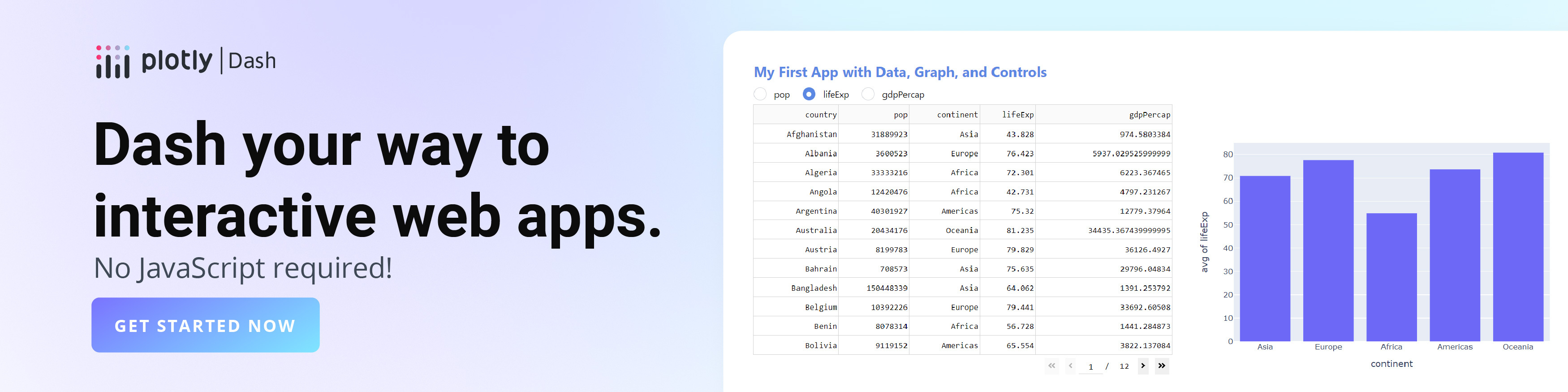