Formatting Ticks in JavaScript
How to format axes ticks in D3.js-based JavaScript charts.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
{
xaxis: {
/* show/hide tick labels (defaults to true) */
showticklabels: boolean,
/* Set the tick mode for the axis "auto" or "linear" or "array" */
tickmode: 'auto',
/* Set the placement of the first tick*/
tick0: '',
/* Set the step in-between ticks*/
dtick: '',
/* Specifies the maximum number of ticks */
nticks: 0,
/* Set the values at which ticks on this axis appear */
tickvals: [ /* */ ],
/* Set the text displayed at the ticks position via tickvals */
ticktext: [ /* */ ],
/* Set the source reference for tickvals */
tickvalssrc: '',
/* Set the source reference for ticktext */
tickvtextsrc: '',
/* Set the tick label formatting rule using d3 formatting mini-languages */
tickformat: '',
/* Set the tickformat per zoom level */
tickformatstops: {
enabled: true,
/* Set the range of the dtick values which describe the zoom level, it is possible to omit "min" or "max" value by passing "null" */
dtickrange: ["min", "max"],
/* dtickformat for described zoom level, the same as "tickformat" */
value: string,
},
/* Set the ticks to display with a prefix: "all" or "first" or "last" or "none" */
showtickprefix: 'all',
tickprefix: string,
/* Set the ticks to display with a suffix: "all" or "first" or "last" or "none" */
showticksuffix: 'all',
ticksuffix: string,
/* Determines a formatting rule for the tick exponents: "none" or "e" or "E" or "power" or "SI" or "B" */
exponentformat: 'B',
}
/* similarly for yaxis */
}
var x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12];
var y = [28.8, 28.5, 37, 56.8, 69.7, 79.7, 78.5, 77.8, 74.1, 62.6, 45.3, 39.9];
var data = [{
x: x,
y: y,
type: 'scatter'
}];
var layout = {
xaxis: {
tickmode: "linear", // If "linear", the placement of the ticks is determined by a starting position `tick0` and a tick step `dtick`
tick0: 0.5,
dtick: 0.75
}
}
Plotly.newPlot('myDiv', data, layout);
var x = ['2000-01', '2000-02', '2000-03', '2000-04', '2000-05', '2000-06', '2000-07', '2000-08', '2000-09', '2000-10', '2000-11', '2000-12', '2001-01'];
var y = [-36.5, -26.6, -43.6, -52.3, -71.5, -81.4, -80.5, -82.2, -76, -67.3, -46.1, -35, -40];
var data = [{
x: x,
y: y,
type: 'scatter'
}];
var layout = {
xaxis: {
tickmode: "linear", // If "linear", the placement of the ticks is determined by a starting position `tick0` and a tick step `dtick`
tick0: '1999-12-15',
dtick: 30 * 24 * 60 * 60 * 1000 // milliseconds
}
}
Plotly.newPlot('myDiv', data, layout);
var x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12];
var y = [28.8, 28.5, 37, 56.8, 69.7, 79.7, 78.5, 77.8, 74.1, 62.6, 45.3, 39.9];
var data = [{
x: x,
y: y,
type: 'scatter'
}];
var layout = {
xaxis: {
tickmode: "array", // If "array", the placement of the ticks is set via `tickvals` and the tick text is `ticktext`.
tickvals: [1, 3, 5, 7, 9, 11],
ticktext: ['One', 'Three', 'Five', 'Seven', 'Nine', 'Eleven']
}
}
Plotly.newPlot('myDiv', data, layout);
var x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12];
var y = [0.18, 0.38, 0.56, 0.46, 0.59, 0.4, 0.78, 0.77, 0.74, 0.42, 0.45, 0.39];
var data = [{
x: x,
y: y,
type: 'scatter'
}];
var layout = {
yaxis: {
tickformat: '%' // For more formatting types, see: https://github.com/d3/d3-format/blob/master/README.md#locale_format
}
}
Plotly.newPlot('myDiv', data, layout);
d3.csv("https://raw.githubusercontent.com/plotly/datasets/master/finance-charts-apple.csv", function (err, rows) {
function unpack(rows, key) {
return rows.map(function (row) {
return row[key];
});
}
var trace1 = {
type: "scatter",
mode: "lines",
name: 'AAPL High',
x: unpack(rows, 'Date'),
y: unpack(rows, 'AAPL.High'),
line: {
color: '#17BECF'
}
}
var trace2 = {
type: "scatter",
mode: "lines",
name: 'AAPL Low',
x: unpack(rows, 'Date'),
y: unpack(rows, 'AAPL.Low'),
line: {
color: '#7F7F7F'
}
}
var data = [trace1, trace2];
var layout = {
title: 'Time series with custom tickformat',
xaxis: {
tickformat: '%d %B (%a)\n %Y' // For more time formatting types, see: https://github.com/d3/d3-time-format/blob/master/README.md
}
};
Plotly.newPlot('myDiv', data, layout);
})
var gd = document.getElementById('myDiv');
var x = ["2005-01", "2005-02", "2005-03", "2005-04", "2005-05", "2005-06", "2005-07"];
var y = [-20, 10, -5, 0, 5, -10, 20];
var data = [{
x: x,
y: y,
type: 'scatter'
}];
var layout = {
xaxis: {
tickformatstops: [{
"dtickrange": [null, 1000],
"value": "%H:%M:%S.%L ms"
},
{
"dtickrange": [1000, 60000],
"value": "%H:%M:%S s"
},
{
"dtickrange": [60000, 3600000],
"value": "%H:%M m"
},
{
"dtickrange": [3600000, 86400000],
"value": "%H:%M h"
},
{
"dtickrange": [86400000, 604800000],
"value": "%e. %b d"
},
{
"dtickrange": [604800000, "M1"],
"value": "%e. %b w"
},
{
"dtickrange": ["M1", "M12"],
"value": "%b '%y M"
},
{
"dtickrange": ["M12", null],
"value": "%Y Y"
}
]
}
};
Plotly.newPlot("myDiv", data, layout);
var x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12];
var y = [68000, 52000, 60000, 20000, 95000, 40000, 60000, 79000, 74000, 42000, 20000, 90000];
var data = [{
x: x,
y: y,
type: 'scatter'
}];
var layout = {
yaxis: {
showexponent: 'all',
exponentformat: 'e'
}
}
Plotly.newPlot('myDiv', data, layout);
var x = ['2013-02-04', '2013-04-05', '2013-06-06', '2013-08-07', '2013-10-02'];
var y = [1, 4, 3, 6, 2];
var data = [{
x: x,
y: y,
type: 'scatter'
}];
var layout = {
xaxis: {
tickformat: '%a %e %b \n %Y'
}
}
Plotly.newPlot('myDiv', data, layout, {
locale: 'fr' // For more info, see: https://github.com/plotly/plotly.js/blob/master/dist/README.md#to-include-localization and https://github.com/plotly/plotly.js/tree/master/dist
});
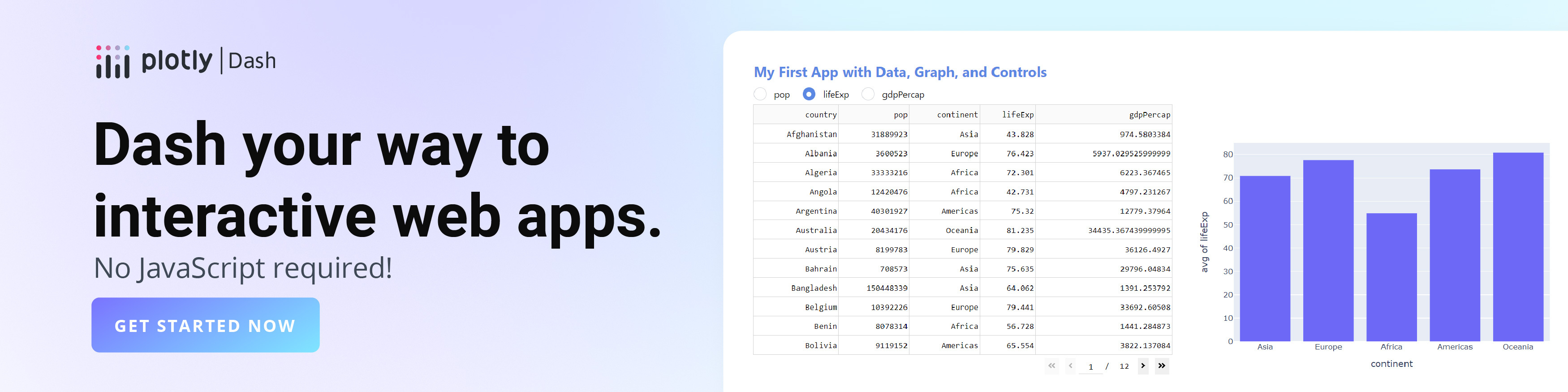