Text Template in JavaScript
How to use D3.js-based text template in Plotly.js.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
To show an arbitrary text in your chart you can use texttemplate, which is a template string used for rendering the information, and will override textinfo.
var data = [{
type: "pie",
values: [2, 5, 3, 2.5],
labels: ["R", "Python", "Java Script", "Matlab"],
texttemplate: "%{label}: %{value} (%{percent})",
textposition: "inside"
}];
Plotly.newPlot("myDiv", data)
The following example uses textfont to customize the added text.
var data = [{
type: "scatterternary",
a: [3, 2, 5],
b: [2, 5, 2],
c: [5, 2, 2],
mode: "markers+text",
text: ["A", "B", "C"],
texttemplate: "%{text}<br>(%{a:.2f}, %{b:.2f}, %{c:.2f})",
textposition: "bottom center",
textfont:{'family': "Times", 'size': [18, 21, 20], 'color': ["IndianRed", "MediumPurple", "DarkOrange"]}
}];
Plotly.newPlot("myDiv", data)
The following example displays how to show date by setting axis.type in funnel charts.
var data = [{
type: 'funnel',
name: 'Montreal',
orientation: "h",
y: ["2018-01-01", "2018-07-01", "2019-01-01", "2020-01-01"],
x: [100, 60, 40, 20],
textposition: "inside",
texttemplate: "%{label}"
},{
type: "funnel",
name: 'Vancouver',
orientation: "h",
y: ["2018-01-01", "2018-07-01", "2019-01-01", "2020-01-01"],
x: [90, 70, 50, 10],
textposition: "inside",
textinfo: "label"}]
var layout = {yaxis: {type: 'date'}}
Plotly.newPlot("myDiv", data, layout)
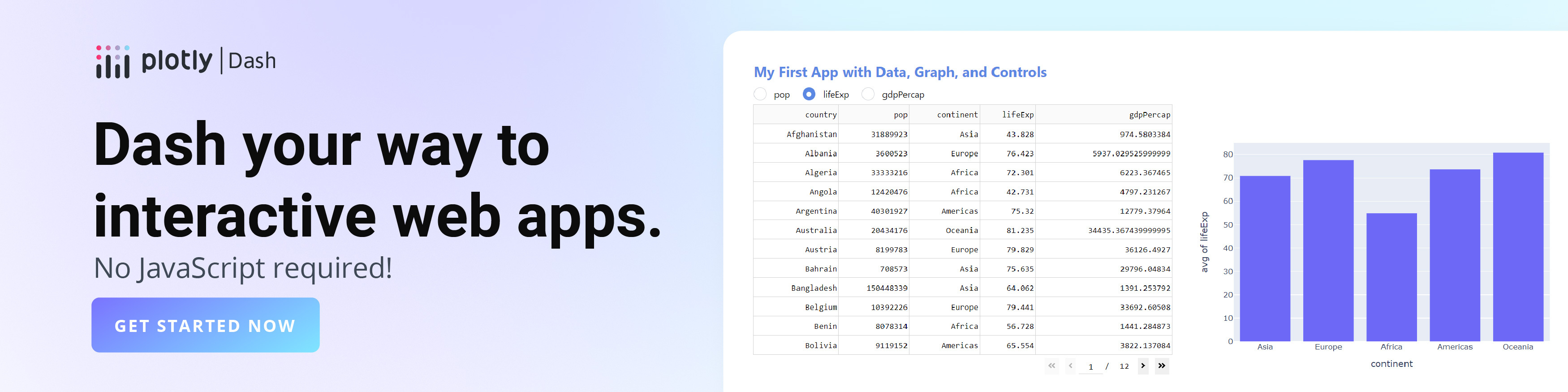