Sunburst Charts in JavaScript
How to make a D3.js-based sunburst chart in javascript. Visualize hierarchical data spanning outward radially from root to leaves.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
var data = [{
type: "sunburst",
labels: ["Eve", "Cain", "Seth", "Enos", "Noam", "Abel", "Awan", "Enoch", "Azura"],
parents: ["", "Eve", "Eve", "Seth", "Seth", "Eve", "Eve", "Awan", "Eve" ],
values: [10, 14, 12, 10, 2, 6, 6, 4, 4],
outsidetextfont: {size: 20, color: "#377eb8"},
leaf: {opacity: 0.4},
marker: {line: {width: 2}},
}];
var layout = {
margin: {l: 0, r: 0, b: 0, t: 0},
width: 500,
height: 500
};
Plotly.newPlot('myDiv', data, layout);
With branchvalues "total", the value of the parent represents the width of its wedge. In the example below,
"Enoch" is 4 and "Awan" is 6 and so Enoch's width is 4/6ths of Awans. With branchvalues "remainder", the
parent's width is determined by its own value plus those of its children. So, Enoch's width is 4/10ths of
Awan's (4 / (6 + 4)).
Note that this means that the sum of the values of the children cannot exceed the
value of their parent when branchvalues "total". When branchvalues "relative" (the default), children will not take
up all of the space below their parent (unless the parent is the root and it has a value of 0).
var data = [
{
"type": "sunburst",
"labels": ["Eve", "Cain", "Seth", "Enos", "Noam", "Abel", "Awan", "Enoch", "Azura"],
"parents": ["", "Eve", "Eve", "Seth", "Seth", "Eve", "Eve", "Awan", "Eve" ],
"values": [65, 14, 12, 10, 2, 6, 6, 4, 4],
"leaf": {"opacity": 0.4},
"marker": {"line": {"width": 2}},
"branchvalues": 'total'
}];
var layout = {
"margin": {"l": 0, "r": 0, "b": 0, "t": 0},
};
Plotly.newPlot('myDiv', data, layout, {showSendToCloud: true})
myPlot = document.getElementById("myDiv");
var data = [{
type: "sunburst",
ids: [
"North America", "Europe", "Australia", "North America - Football", "Soccer",
"North America - Rugby", "Europe - Football", "Rugby",
"Europe - American Football","Australia - Football", "Association",
"Australian Rules", "Autstralia - American Football", "Australia - Rugby",
"Rugby League", "Rugby Union"
],
labels: [
"North<br>America", "Europe", "Australia", "Football", "Soccer", "Rugby",
"Football", "Rugby", "American<br>Football", "Football", "Association",
"Australian<br>Rules", "American<br>Football", "Rugby", "Rugby<br>League",
"Rugby<br>Union"
],
parents: [
"", "", "", "North America", "North America", "North America", "Europe",
"Europe", "Europe","Australia", "Australia - Football", "Australia - Football",
"Australia - Football", "Australia - Football", "Australia - Rugby",
"Australia - Rugby"
],
outsidetextfont: {size: 20, color: "#377eb8"},
// leaf: {opacity: 0.4},
marker: {line: {width: 2}},
}];
var layout = {
margin: {l: 0, r: 0, b: 0, t:0},
sunburstcolorway:["#636efa","#ef553b","#00cc96"],
};
Plotly.newPlot('myDiv', data, layout);
d3.csv('https://raw.githubusercontent.com/plotly/datasets/master/coffee-flavors.csv', function(err, rows){
function unpack(rows, key) {
return rows.map(function(row) { return row[key]; });
}
var data = [
{
type: "sunburst",
maxdepth: 3,
ids: unpack(rows, 'ids'),
labels: unpack(rows, 'labels'),
parents:unpack(rows, 'parents')
}
];
var layout = {
margin: {l: 0, r: 0, b: 0, t:0},
sunburstcolorway:[
"#636efa","#EF553B","#00cc96","#ab63fa","#19d3f3",
"#e763fa", "#FECB52","#FFA15A","#FF6692","#B6E880"
],
extendsunburstcolorway: true
};
Plotly.newPlot('myDiv', data, layout, {showSendToCloud: true});
})
The insidetextorientation
attribute controls the orientation of the text inside chart sectors. When set to auto, text may be oriented in any direction in order to be as big as possible in the middle of a sector. The horizontal option orients text to be parallel with the bottom of the chart, and may make text smaller in order to achieve that goal. The radial option orients text along the radius of the sector. The tangential option orients text perpendicular to the radius of the sector.
d3.csv('https://raw.githubusercontent.com/plotly/datasets/master/coffee-flavors.csv', function(err, rows){
function unpack(rows, key) {
return rows.map(function(row) {return row[key]})
}
var data = [{
type: "sunburst",
maxdepth: 2,
ids: unpack(rows, 'ids'),
labels: unpack(rows, 'labels'),
parents: unpack(rows, 'parents'),
textposition: 'inside',
insidetextorientation: 'radial'
}]
var layout = {margin: {l: 0, r: 0, b: 0, t:0}}
Plotly.newPlot('myDiv', data, layout)
})
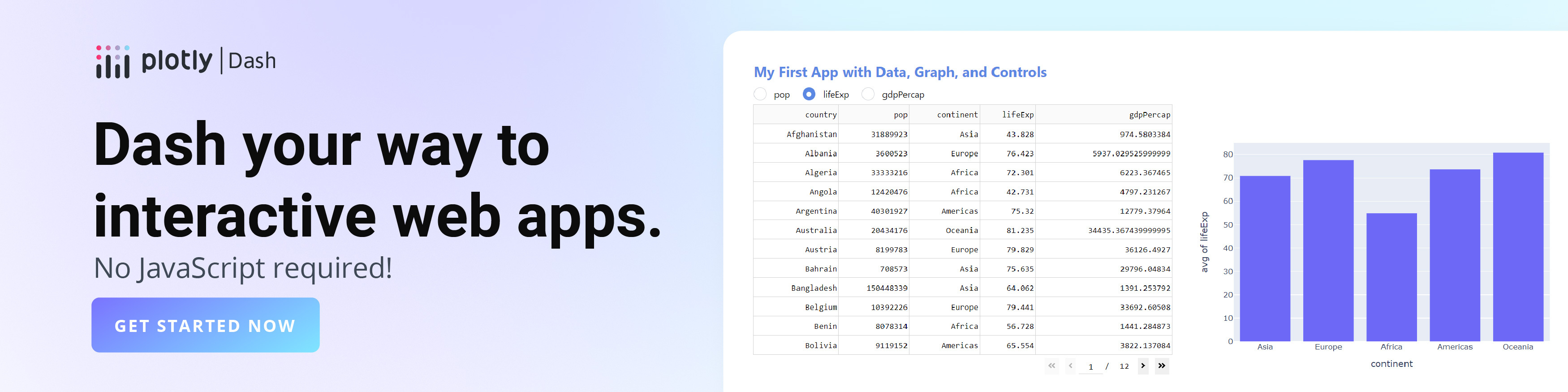