Slider Events in JavaScript
Use Plotly to create custom sliders in D3.js-based JavaScript charts.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
Plotly.newPlot('myDiv', [{
x: [1, 2, 3],
y: [2, 1, 3]
}], {
sliders: [{
pad: {t: 30},
currentvalue: {
xanchor: 'right',
prefix: 'color: ',
font: {
color: '#888',
size: 20
}
},
steps: [{
label: 'red',
method: 'restyle',
args: ['line.color', 'red']
}, {
label: 'green',
method: 'restyle',
args: ['line.color', 'green']
}, {
label: 'blue',
method: 'restyle',
args: ['line.color', 'blue']
}]
}]
});
Plotly.newPlot('myDiv', [{
x: [1, 2, 3],
y: [2, 1, 3]
}], {
sliders: [{
pad: {t: 30},
len: 0.5,
x: 0.5,
currentvalue: {
xanchor: 'right',
prefix: 'color: ',
font: {
color: '#888',
size: 20
}
},
// If all of a component's commands affect a single attribute, the component
// will be bound to the plot and will automatically update to reflect changes.
steps: [{
label: 'red',
method: 'restyle',
args: ['line.color', 'red']
}, {
label: 'green',
method: 'restyle',
args: ['line.color', 'green']
}, {
label: 'blue',
method: 'restyle',
args: ['line.color', 'blue']
}]
}],
updatemenus: [{
pad: {t: 60, r: 30},
type: 'buttons',
xanchor: 'left',
yanchor: 'top',
x: 00,
y: 0,
direction: 'right',
buttons: [{
label: 'red',
method: 'restyle',
args: ['line.color', 'red']
}, {
label: 'green',
method: 'restyle',
args: ['line.color', 'green']
}, {
label: 'blue',
method: 'restyle',
args: ['line.color', 'blue']
}]
}]
});
Plotly.newPlot('myDiv', {
data: [{
x: [1, 2, 3],
y: [2, 1, 3],
line: {
color: 'red',
simplify: false,
}
}],
layout: {
sliders: [{
pad: {t: 30},
x: 0.05,
len: 0.95,
currentvalue: {
xanchor: 'right',
prefix: 'color: ',
font: {
color: '#888',
size: 20
}
},
transition: {duration: 500},
// By default, animate commands are bound to the most recently animated frame:
steps: [{
label: 'red',
method: 'animate',
args: [['red'], {
mode: 'immediate',
frame: {redraw: false, duration: 500},
transition: {duration: 500}
}]
}, {
label: 'green',
method: 'animate',
args: [['green'], {
mode: 'immediate',
frame: {redraw: false, duration: 500},
transition: {duration: 500}
}]
}, {
label: 'blue',
method: 'animate',
args: [['blue'], {
mode: 'immediate',
frame: {redraw: false, duration: 500},
transition: {duration: 500}
}]
}]
}],
updatemenus: [{
type: 'buttons',
showactive: false,
x: 0.05,
y: 0,
xanchor: 'right',
yanchor: 'top',
pad: {t: 60, r: 20},
buttons: [{
label: 'Play',
method: 'animate',
args: [null, {
fromcurrent: true,
frame: {redraw: false, duration: 1000},
transition: {duration: 500}
}]
}]
}]
},
// The slider itself does not contain any notion of timing, so animating a slider
// must be accomplished through a sequence of frames. Here we'll change the color
// and the data of a single trace:
frames: [{
name: 'red',
data: [{
y: [2, 1, 3],
'line.color': 'red'
}]
}, {
name: 'green',
data: [{
y: [3, 2, 1],
'line.color': 'green'}]
}, {
name: 'blue',
data: [{
y: [1, 3, 2],
'line.color': 'blue'}]
}]
});
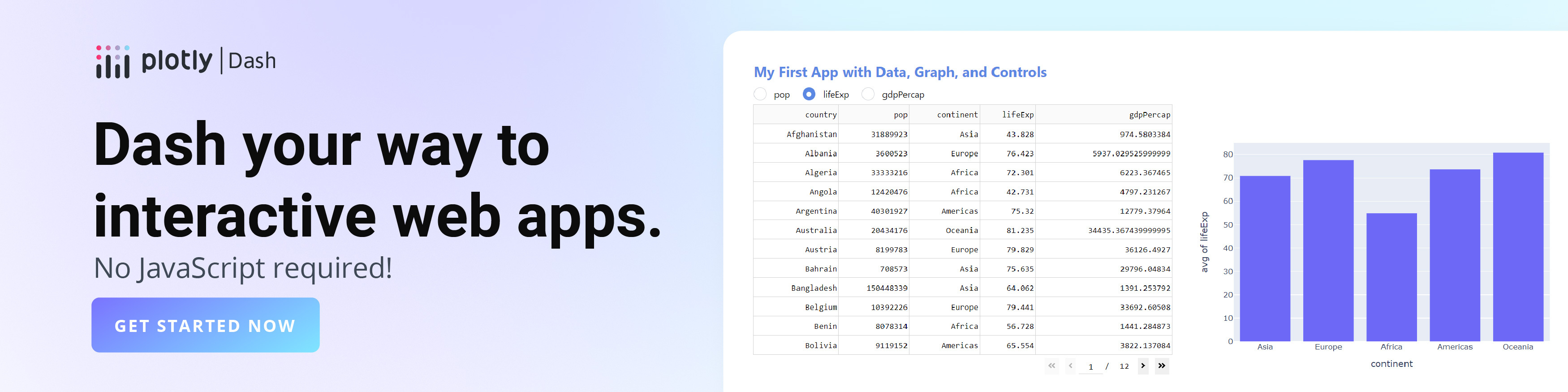