Scatter Plots on Maps in JavaScript
How to make D3.js-based scatter plots on maps in JavaScript. Scatter plots on maps highlight geographic areas and can be colored by value.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
var data = [{
type: 'scattergeo',
mode: 'markers+text',
text: [
'Montreal', 'Toronto', 'Vancouver', 'Calgary', 'Edmonton',
'Ottawa', 'Halifax', 'Victoria', 'Winnepeg', 'Regina'
],
lon: [
-73.57, -79.24, -123.06, -114.1, -113.28,
-75.43, -63.57, -123.21, -97.13, -104.6
],
lat: [
45.5, 43.4, 49.13, 51.1, 53.34, 45.24,
44.64, 48.25, 49.89, 50.45
],
marker: {
size: 7,
color: [
'#bebada', '#fdb462', '#fb8072', '#d9d9d9', '#bc80bd',
'#b3de69', '#8dd3c7', '#80b1d3', '#fccde5', '#ffffb3'
],
line: {
width: 1
}
},
name: 'Canadian cities',
textposition: [
'top right', 'top left', 'top center', 'bottom right', 'top right',
'top left', 'bottom right', 'bottom left', 'top right', 'top right'
],
}];
var layout = {
title: {
text: 'Canadian cities',
font: {
family: 'Droid Serif, serif',
size: 16
}
},
geo: {
scope: 'north america',
resolution: 50,
lonaxis: {
'range': [-130, -55]
},
lataxis: {
'range': [40, 70]
},
showrivers: true,
rivercolor: '#fff',
showlakes: true,
lakecolor: '#fff',
showland: true,
landcolor: '#EAEAAE',
countrycolor: '#d3d3d3',
countrywidth: 1.5,
subunitcolor: '#d3d3d3'
}
};
Plotly.newPlot('myDiv', data, layout);
d3.csv('https://raw.githubusercontent.com/plotly/datasets/master/2011_february_us_airport_traffic.csv', function(err, rows){
function unpack(rows, key) {
return rows.map(function(row) { return row[key]; });
}
var scl = [[0,'rgb(5, 10, 172)'],[0.35,'rgb(40, 60, 190)'],[0.5,'rgb(70, 100, 245)'], [0.6,'rgb(90, 120, 245)'],[0.7,'rgb(106, 137, 247)'],[1,'rgb(220, 220, 220)']];
var data = [{
type:'scattergeo',
locationmode: 'USA-states',
lon: unpack(rows, 'long'),
lat: unpack(rows, 'lat'),
hoverinfor: unpack(rows, 'airport'),
text: unpack(rows, 'airport'),
mode: 'markers',
marker: {
size: 8,
opacity: 0.8,
reversescale: true,
autocolorscale: false,
symbol: 'square',
line: {
width: 1,
color: 'rgb(102,102,102)'
},
colorscale: scl,
cmin: 0,
color: unpack(rows, 'cnt'),
colorbar: {
title: 'Incoming Flights February 2011'
}
}
}];
var layout = {
title: 'Most Trafficked US airports',
colorbar: true,
geo: {
scope: 'usa',
projection: {
type: 'albers usa'
},
showland: true,
landcolor: 'rgb(250,250,250)',
subunitcolor: 'rgb(217,217,217)',
countrycolor: 'rgb(217,217,217)',
countrywidth: 0.5,
subunitwidth: 0.5
}
};
Plotly.newPlot("myDiv", data, layout, {showLink: false});
});
d3.csv('https://raw.githubusercontent.com/plotly/datasets/master/2015_06_30_precipitation.csv', function(err, rows){
function unpack(rows, key) {
return rows.map(function(row) { return row[key]; });
}
scl = [[0, 'rgb(150,0,90)'],[0.125, 'rgb(0, 0, 200)'],[0.25,'rgb(0, 25, 255)'],[0.375,'rgb(0, 152, 255)'],[0.5,'rgb(44, 255, 150)'],[0.625,'rgb(151, 255, 0)'],[0.75,'rgb(255, 234, 0)'],[0.875,'rgb(255, 111, 0)'],[1,'rgb(255, 0, 0)']];
var data = [{
type: 'scattergeo',
mode: 'markers',
text: unpack(rows, 'Globvalue'),
lon: unpack(rows, 'Lon'),
lat: unpack(rows, 'Lat'),
marker: {
color: unpack(rows, 'Globvalue'),
colorscale: scl,
cmin: 0,
cmax: 1.4,
reversescale: true,
opacity: 0.2,
size: 2,
colorbar:{
thickness: 10,
title: {side:
'right'
},
outlinecolor: 'rgba(68,68,68,0)',
ticks: 'outside',
ticklen: 3,
shoticksuffix: 'last',
ticksuffix: 'inches',
dtick: 0.1
}
},
name: 'NA Precipitation'
}];
var layout = {
geo:{
scope: 'north america',
showland: true,
landcolor: 'rgb(212,212,212)',
subunitcolor: 'rgb(255,255,255)',
countrycolor: 'rgb(255,255,255)',
showlakes: true,
lakecolor: 'rgb(255,255,255)',
showsubunits: true,
showcountries: true,
resolution: 50,
projection: {
type: 'conic conformal',
rotation: {
long: -100
}
},
},
longaxis: {
showgrid: true,
gridwidth: 0.5,
range: [ -140.0, -55.0 ],
dtick: 5
},
lataxis: {
showgrid: true,
gridwidth: 0.5,
range: [ 20.0, 60.0 ],
dtick: 5
},
title: 'North America Precipitation',
width: 600,
height: 600
};
Plotly.newPlot('myDiv', data, layout);
});
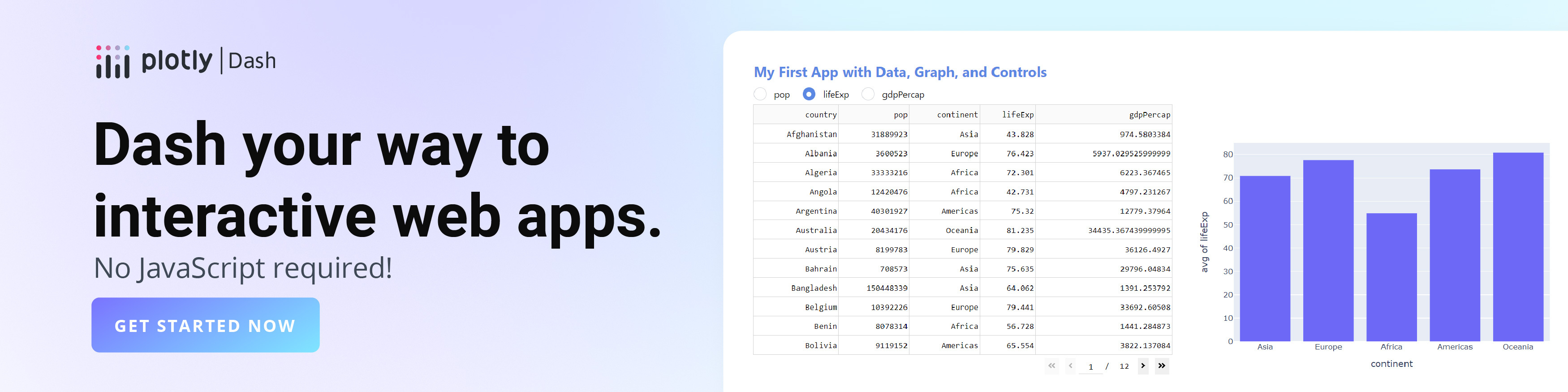