Styling Markers in JavaScript
How to style markers in JavaScript.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
In order to make markers distinct, you can add a border to the markers. This can be achieved by adding the line dict to the marker dict. For example, marker:{..., line: {...}}
.
var x = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var y = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var data = [{
x: x,
y: y,
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgb(17, 157, 255)',
size: 20,
line: {
color: 'rgb(231, 99, 250)',
width: 2
}
},
showlegend: false
}, {
x: [2],
y: [4.5],
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgb(17, 157, 255)',
size: 60,
line: {
color: 'rgb(231, 99, 250)',
width: 6
}
},
showlegend: false
}]
Plotly.newPlot('myDiv', data)
Fully opaque, the default setting, is useful for non-overlapping markers. When many points overlap it can be hard to observe density.
var x = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var y = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var data = [{
x: x,
y: y,
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgb(17, 157, 255)',
size: 20,
line: {
color: 'rgb(231, 99, 250)',
width: 2
}
},
showlegend: false
}, {
x: [2,2],
y: [4.25,4.75],
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgb(17, 157, 255)',
size: 60,
line: {
color: 'rgb(231, 99, 250)',
width: 6
}
},
showlegend: false
}]
Plotly.newPlot('myDiv', data)
Setting opacity outside the marker will set the opacity of the trace. Thus, it will allow greater visbility of additional traces but like fully opaque it is hard to distinguish density.
var x = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var y = Array.from({length: 500}, () => Math.random()*(4.5-3)+3);
var x2 = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var y2 = Array.from({length: 500}, () => Math.random()*(6-4.5)+4.5);
var data = [{
x: x,
y: y,
type: 'scatter',
mode: 'markers',
opacity: 0.5,
marker: {
color: 'rgb(17, 157, 255)',
size: 20,
line: {
color: 'rgb(231, 99, 250)',
width: 2
}
},
name: 'Opacity 0.5'
}, {
x: x2,
y: y2,
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgb(17, 157, 255)',
size: 20,
line: {
color: 'rgb(231, 99, 250)',
width: 2
}
},
name: 'Opacity 1.0'
}, {
x: [2,2],
y: [4.25,4.75],
type: 'scatter',
mode: 'markers',
opacity: 0.5,
marker: {
color: 'rgb(17, 157, 255)',
size: 60,
line: {
color: 'rgb(231, 99, 250)',
width: 6
}
},
showlegend: false
}]
Plotly.newPlot('myDiv', data)
To maximise visibility of density, it is recommended to set the opacity inside the marker marker:{opacity:0.5}
. If multiple traces exist with high density, consider using marker opacity in conjunction with trace opacity.
var x = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var y = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var data = [{
x: x,
y: y,
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgb(17, 157, 255)',
opacity: 0.5,
size: 20,
line: {
color: 'rgb(231, 99, 250)',
width: 2
}
},
showlegend: false
}, {
x: [2,2],
y: [4.25,4.75],
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgb(17, 157, 255)',
opacity: 0.5,
size: 60,
line: {
color: 'rgb(231, 99, 250)',
width: 6
}
},
showlegend: false
}]
Plotly.newPlot('myDiv', data)
To maximise visibility of each point, set the color opacity by using alpha: marker:{color: 'rgba(0,0,0,0.5)'}
. Here, the marker line will remain opaque.
var x = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var y = Array.from({length: 500}, () => Math.random()*(6-3)+3);
var data = [{
x: x,
y: y,
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgba(17, 157, 255,0.5)',
size: 20,
line: {
color: 'rgb(231, 99, 250)',
width: 2
}
},
showlegend: false
}, {
x: [2,2],
y: [4.25,4.75],
type: 'scatter',
mode: 'markers',
marker: {
color: 'rgba(17, 157, 255,0.5)',
size: 60,
line: {
color: 'rgb(231, 99, 250)',
width: 6
}
},
showlegend: false
}]
Plotly.newPlot('myDiv', data)
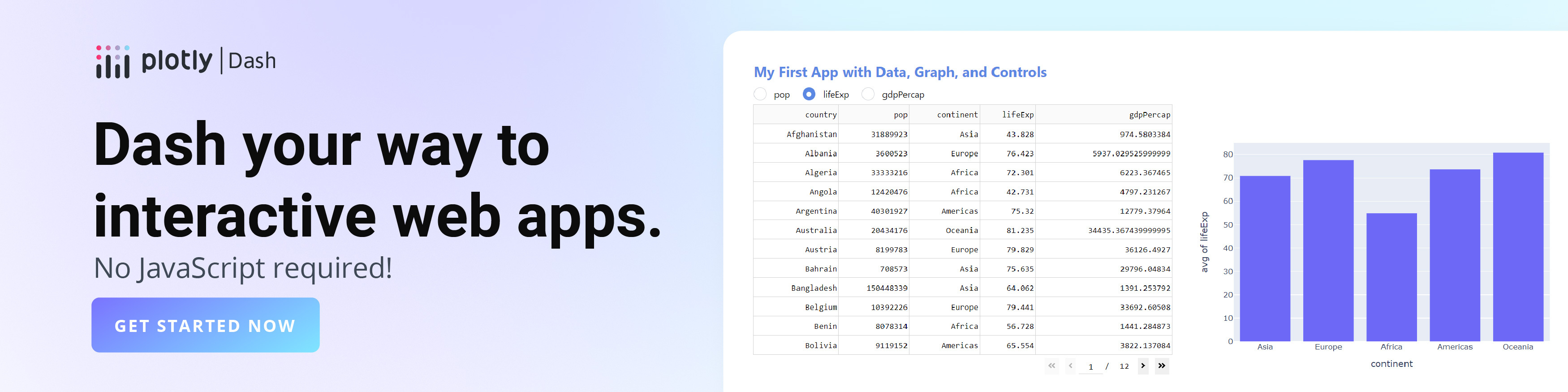