Lines on Maps in JavaScript
How to draw D3.js-based lines, great circles, and contours on maps in JavaScript. Lines on maps can show distance between geographic points or be contour lines (isolines, isopleths, or isarithms).
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
d3.csv('https://raw.githubusercontent.com/plotly/datasets/master/globe_contours.csv', function(err, rows){
function unpack(rows, key) {
return rows.map(function(row) { return row[key]; });
}
var data = [];
var scl =['rgb(213,62,79)','rgb(244,109,67)','rgb(253,174,97)','rgb(254,224,139)','rgb(255,255,191)','rgb(230,245,152)','rgb(171,221,164)','rgb(102,194,165)','rgb(50,136,189)'];
var allLats = [];
var allLons = [];
for ( var i = 0 ; i < scl.length; i++){
var latHead = 'lat-'+i;
var lonHead = 'lon-'+i;
var lat = unpack(rows, latHead);
var lon = unpack(rows, lonHead);
allLats.push(lat);
allLons.push(lon);
}
for ( var i = 0 ; i < scl.length; i++) {
var current = {
type:'scattergeo',
lon: allLons[i],
lat: allLats[i],
mode: 'lines',
line: {
width: 2,
color: scl[i]
}
}
data.push(current);
};
var layout = {
geo: {
projection: {
type: 'orthographic',
rotation: {
lon: -100,
lat: 40
},
},
showocean: true,
oceancolor: 'rgb(0, 255, 255)',
showland: true,
landcolor: 'rgb(230, 145, 56)',
showlakes: true,
lakecolor: 'rgb(0, 255, 255)',
showcountries: true,
lonaxis: {
showgrid: true,
gridcolor: 'rgb(102, 102, 102)'
},
lataxis: {
showgrid: true,
gridcolor: 'rgb(102, 102, 102)'
}
}
};
Plotly.newPlot("myDiv", data, layout, {showLink: false});
});
var data = [{
type: 'scattergeo',
lat: [ 40.7127, 51.5072 ],
lon: [ -74.0059, 0.1275 ],
mode: 'lines',
line:{
width: 2,
color: 'blue'
}
}];
var layout = {
title: 'London to NYC Great Circle',
showlegend: false,
geo: {
resolution: 50,
showland: true,
showlakes: true,
landcolor: 'rgb(204, 204, 204)',
countrycolor: 'rgb(204, 204, 204)',
lakecolor: 'rgb(255, 255, 255)',
projection: {
type: 'equirectangular'
},
coastlinewidth: 2,
lataxis: {
range: [ 20, 60 ],
showgrid: true,
tickmode: 'linear',
dtick: 10
},
lonaxis:{
range: [-100, 20],
showgrid: true,
tickmode: 'linear',
dtick: 20
}
}
};
Plotly.newPlot('myDiv', data, layout);
d3.csv('https://raw.githubusercontent.com/plotly/datasets/c34aaa0b1b3cddad335173cb7bc0181897201ee6/2011_february_aa_flight_paths.csv', function(err, rows){
function unpack(rows, key) {
return rows.map(function(row) { return row[key]; });}
function getMaxOfArray(numArray) {
return Math.max.apply(null, numArray);
}
var data = [];
var count = unpack(rows, 'cnt');
var startLongitude = unpack(rows, 'start_lon');
var endLongitude = unpack(rows, 'end_lon');
var startLat = unpack(rows, 'start_lat');
var endLat = unpack(rows, 'end_lat');
for ( var i = 0 ; i < count.length; i++ ) {
var opacityValue = count[i]/getMaxOfArray(count);
var result = {
type: 'scattergeo',
locationmode: 'USA-states',
lon: [ startLongitude[i] , endLongitude[i] ],
lat: [ startLat[i] , endLat[i] ],
mode: 'lines',
line: {
width: 1,
color: 'red'
},
opacity: opacityValue
};
data.push(result);
};
var layout = {
title: 'Feb. 2011 American Airline flight paths',
showlegend: false,
geo:{
scope: 'north america',
projection: {
type: 'azimuthal equal area'
},
showland: true,
landcolor: 'rgb(243,243,243)',
countrycolor: 'rgb(204,204,204)'
}
};
Plotly.newPlot("myDiv", data, layout, {showLink: false});
});
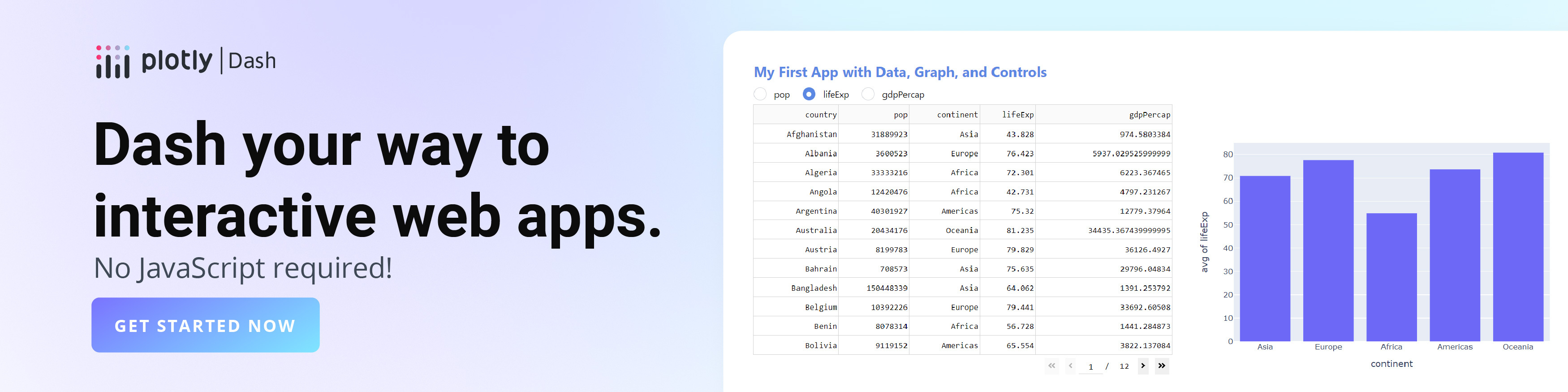