Layout Template Examples in JavaScript
Plotly's template attribute and how to use it with Container arrays.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
The template
attribute of layout
allows a Plotly chart to take it's style and formatting from a template
object. template
s can be generated using Plotly.makeTemplate
or manually. annotaions
, updatemenus
, images
, shapes
and other container array objects in the Plotly layout
are specially handled by the template machinery to provide more flexibility when using these container arrays
in plots derived from these templates.
For more information see https://plotly.com/javascript/reference/layout/#layout-template.
Container array items in a template with a name
attribute will be added to any plot using that template.
We can use this feature to create a template that adds watermarks to our chart by including named image items in images
.
The example below also shows how to make one of these images invisible using the templateitemname
attribute
if you don't want it to display for this specific chart.
var baseLayout = {
title: 'Watermark Template',
// items with a `name` attribute in template.images will be added to any
// plot using this template
images: [{
name: 'watermark_1',
source: "https://raw.githubusercontent.com/michaelbabyn/plot_data/master/benzene.png",
xref: "paper",
yref: "paper",
x: 0.40,
y: 0.9,
sizex: 0.7,
sizey: 0.7,
opacity: 0.1,
layer: "below"
},
{
name: 'watermark_2',
source: "https://raw.githubusercontent.com/michaelbabyn/plot_data/master/naphthalene.png",
xref: "paper",
yref: "paper",
x: .75,
y: 0.3,
sizex: 0.25,
sizey: 0.25,
sizing: "stretch",
opacity: 0.2,
layer: "below"
}],
showlegend: false
};
var template = {data: {}, layout: baseLayout};
var data = [{
x: [0, 1, 2, 3, 4, 5],
y: [2, 4, 3, 0, 5, 6],
}];
var layoutUsingTemplate = {
template: template,
images: [
{
// set the second watermark in the template to be invisible
templateitemname: 'watermark_2',
visible: false
}
]
};
Plotly.newPlot("myDiv", data, layoutUsingTemplate);
A container item in your new plot with the attribute templateitemname
matching one of the named
container items in the template will inherit attributes from item with the corresponding name.
If an item in the plot using the template has the templateitemname
attribute but there is no
corresponding template container item by the same name, it will be marked as invisible in your new plot.
var x = [0, 1, 2, 3, 4, 5];
var y = [2, 4, 3, 0, 5, 6];
var baseData = [{
mode: 'lines',
error_y: {visible: true, width: 0},
line: {color: 'teal'}
}];
var baseLayout = {
title: 'Template Title',
annotations: [{
text: 'First point',
name:'first',
yref: 'y', xref: 'x',
ay: 40, ax: 30,
font: {size: 16}
}],
showlegend: false
};
// use Plotly.makeTemplate to generate the template object
var template = Plotly.makeTemplate({data: baseData, layout: baseLayout});
var data = [{
x: x,
y: y,
}];
var annotations = [
// plotly will look for an annotation with `name` matching `templateitemname`
// and use insert that annotation into the new plot.
{
templateitemname:'first',
x: x[0],
y: y[0],
},
{
templateitemname: 'fourth', //since there is no template item with this name,
//this annotation will be set to invisible.
text: 'Fourth point',
x: x[3],
y: y[3],
showarrow: true,
yref: 'y', xref: 'x',
}
];
var layoutWithTemplate = {template: template, annotations: annotations};
Plotly.newPlot("myDiv", data, layoutWithTemplate);
Add an attribute called annotationdefaults
to your template to set a default annotation object. Each
item in the plot using the template without a templateitemname
attribute will have the default applied
to it. annotationdefaults
can be manually added to a template or, if makeTemplate is used, the first un-named
item in annotations will be used as the default.
Note, this behaviour works for all container array objects. E.g for images
, you would create imagedefaults
in
your layout containing the default image item.
var x = [0, 1, 2, 3, 4, 5];
var y = [2, 4, 3, 0, 5, 6];
var baseData = [{
mode: 'lines',
error_y: {visible: true, width: 0},
line: {color: 'teal'}
}];
var baseLayout = {
// Plotly.makeTemplate will use the first annotation without a `name` attribute
// in the annotations array as the annotationdefaults for the template.
annotations: [
{
text: 'DEFAULT ANNOTATION',
x: 0.1,
y: 1.1,
yref: 'paper', xref: 'paper',
showarrow: false,
font: {color:'teal', size: 14}
}
],
showlegend: false
};
// use Plotly.makeTemplate to generate the template object
var template = Plotly.makeTemplate({data: baseData, layout: baseLayout});
var data = [{
x: x,
y: y
}];
var annotations = [
{}, // An empty annotation object will copy annotationdefaults
{
text: 'Third point',
x: x[2],
y: y[2],
showarrow: true,
yref: 'y', xref: 'x',
font: {size: 20} // since there is no font.color attribute for this object,
// it will use the annotationdefaults' color
}
];
var layoutWithTemplate = {template: template, annotations: annotations};
Plotly.newPlot("myDiv", data, layoutWithTemplate);
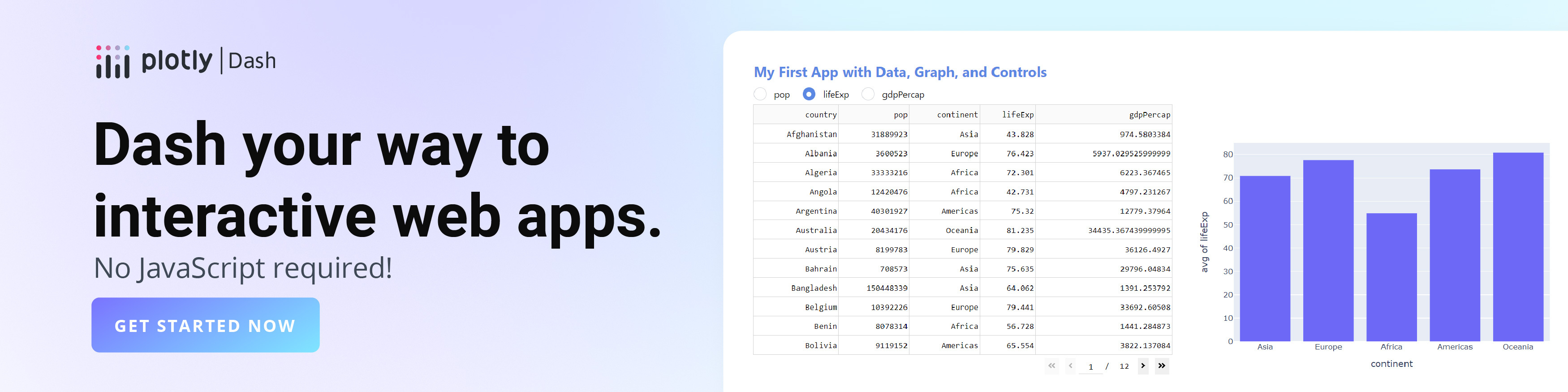