Dropdown Events in JavaScript
Use Plotly to create custom dropdowns in D3.js-based JavaScript charts.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
function makeTrace(i) {
return {
y: Array.apply(null, Array(10)).map(() => Math.random()),
line: {
shape: 'spline' ,
color: 'red'
},
visible: i === 0,
name: 'Data set ' + i,
};
}
Plotly.newPlot('myDiv', [0, 1, 2, 3].map(makeTrace), {
updatemenus: [{
y: 0.8,
yanchor: 'top',
buttons: [{
method: 'restyle',
args: ['line.color', 'red'],
label: 'red'
}, {
method: 'restyle',
args: ['line.color', 'blue'],
label: 'blue'
}, {
method: 'restyle',
args: ['line.color', 'green'],
label: 'green'
}]
}, {
y: 1,
yanchor: 'top',
buttons: [{
method: 'restyle',
args: ['visible', [true, false, false, false]],
label: 'Data set 0'
}, {
method: 'restyle',
args: ['visible', [false, true, false, false]],
label: 'Data set 1'
}, {
method: 'restyle',
args: ['visible', [false, false, true, false]],
label: 'Data set 2'
}, {
method: 'restyle',
args: ['visible', [false, false, false, true]],
label: 'Data set 3'
}]
}],
});
d3.csv('https://raw.githubusercontent.com/plotly/datasets/master/gapminderDataFiveYear.csv', function(err, rows){
function unpack(rows, key) {
return rows.map(function(row) { return row[key]; });
}
var allCountryNames = unpack(rows, 'country'),
allYear = unpack(rows, 'year'),
allGdp = unpack(rows, 'gdpPercap'),
listofCountries = [],
currentCountry,
currentGdp = [],
currentYear = [];
for (var i = 0; i < allCountryNames.length; i++ ){
if (listofCountries.indexOf(allCountryNames[i]) === -1 ){
listofCountries.push(allCountryNames[i]);
}
}
function getCountryData(chosenCountry) {
currentGdp = [];
currentYear = [];
for (var i = 0 ; i < allCountryNames.length ; i++){
if ( allCountryNames[i] === chosenCountry ) {
currentGdp.push(allGdp[i]);
currentYear.push(allYear[i]);
}
}
};
// Default Country Data
setBubblePlot('Afghanistan');
function setBubblePlot(chosenCountry) {
getCountryData(chosenCountry);
var trace1 = {
x: currentYear,
y: currentGdp,
mode: 'lines+markers',
marker: {
size: 12,
opacity: 0.5
}
};
var data = [trace1];
var layout = {
title:'Line and Scatter Plot',
height: 400,
width: 480
};
Plotly.newPlot('myDiv', data, layout);
};
var innerContainer = document.querySelector('[data-num="0"'),
plotEl = innerContainer.querySelector('.plot'),
countrySelector = innerContainer.querySelector('.countrydata');
function assignOptions(textArray, selector) {
for (var i = 0; i < textArray.length; i++) {
var currentOption = document.createElement('option');
currentOption.text = textArray[i];
selector.appendChild(currentOption);
}
}
assignOptions(listofCountries, countrySelector);
function updateCountry(){
setBubblePlot(countrySelector.value);
}
countrySelector.addEventListener('change', updateCountry, false);
});
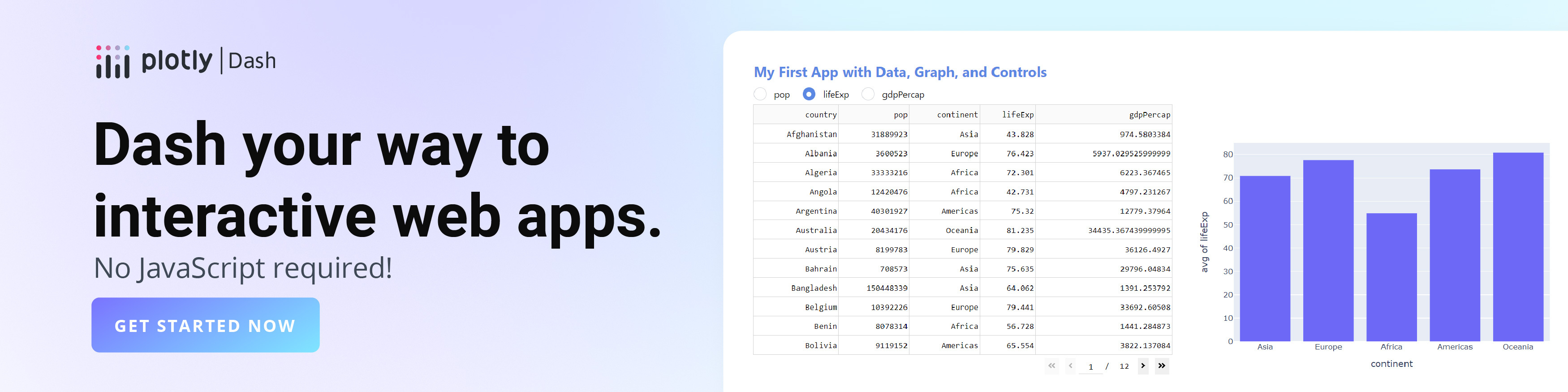