Contour Plots in JavaScript
How to make a D3.js-based contour plot in javascript. Examples of contour plots of matrices with subplots, custom color-scales, and smoothing.
New to Plotly?
Plotly is a free and open-source graphing library for JavaScript. We recommend you read our Getting Started guide for the latest installation or upgrade instructions, then move on to our Plotly Fundamentals tutorials or dive straight in to some Basic Charts tutorials.
var size = 100, x = new Array(size), y = new Array(size), z = new Array(size), i, j;
for(var i = 0; i < size; i++) {
x[i] = y[i] = -2 * Math.PI + 4 * Math.PI * i / size;
z[i] = new Array(size);
}
for(var i = 0; i < size; i++) {
for(j = 0; j < size; j++) {
var r2 = x[i]*x[i] + y[j]*y[j];
z[i][j] = Math.sin(x[i]) * Math.cos(y[j]) * Math.sin(r2) / Math.log(r2+1);
}
}
var data = [ {
z: z,
x: x,
y: y,
type: 'contour'
}
];
Plotly.newPlot('myDiv', data);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5.0, 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour'
}
];
var layout = {
title: 'Basic Contour Plot'
}
Plotly.newPlot('myDiv', data, layout);
var data = [{
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
x: [-9, -6, -5 , -3, -1],
y: [0, 1, 4, 5, 7],
type: 'contour'
}];
var layout = {
title: 'Setting the X and Y Coordinates in a Contour Plot'
};
Plotly.newPlot('myDiv', data, layout);
var data = [{
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
colorscale: 'Jet',
}];
var layout = {
title: 'Colorscale for Contour Plot'
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
colorscale: 'Jet',
autocontour: false,
contours: {
start: 0,
end: 8,
size: 2
}
}];
var layout = {
title: 'Customizing Size and Range of Contours'
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
colorscale: 'Jet',
dx: 10,
x0: 5,
dy: 10,
y0: 10
}];
var layout = {
title: 'Customizing Spacing Between X and Y Axis Ticks'
};
Plotly.newPlot('myDiv', data, layout);
var trace1 = {
z: [[null, null, null, 12, 13, 14, 15, 16],
[null, 1, null, 11, null, null, null, 17],
[null, 2, 6, 7, null, null, null, 18],
[null, 3, null, 8, null, null, null, 19],
[5, 4, 10, 9, null, null, null, 20],
[null, null, null, 27, null, null, null, 21],
[null, null, null, 26, 25, 24, 23, 22]],
type: 'contour',
showscale: false,
xaxis: 'x1',
yaxis: 'y1'
};
var trace2 = {
z: [[null, null, null, 12, 13, 14, 15, 16],
[null, 1, null, 11, null, null, null, 17],
[null, 2, 6, 7, null, null, null, 18],
[null, 3, null, 8, null, null, null, 19],
[5, 4, 10, 9, null, null, null, 20],
[null, null, null, 27, null, null, null, 21],
[null, null, null, 26, 25, 24, 23, 22]],
connectgaps: true,
type: 'contour',
showscale: false,
xaxis: 'x2',
yaxis: 'y2'
};
var trace3 = {
z: [[null, null, null, 12, 13, 14, 15, 16],
[null, 1, null, 11, null, null, null, 17],
[null, 2, 6, 7, null, null, null, 18],
[null, 3, null, 8, null, null, null, 19],
[5, 4, 10, 9, null, null, null, 20],
[null, null, null, 27, null, null, null, 21],
[null, null, null, 26, 25, 24, 23, 22]],
zsmooth: 'best',
type: 'heatmap',
showscale: false,
xaxis: 'x3',
yaxis: 'y3'
};
var trace4 = {
z: [[null, null, null, 12, 13, 14, 15, 16],
[null, 1, null, 11, null, null, null, 17],
[null, 2, 6, 7, null, null, null, 18],
[null, 3, null, 8, null, null, null, 19],
[5, 4, 10, 9, null, null, null, 20],
[null, null, null, 27, null, null, null, 21],
[null, null, null, 26, 25, 24, 23, 22]],
zsmooth: 'best',
type: 'heatmap',
showscale: false,
connectgaps: true,
xaxis: 'x4',
yaxis: 'y4'
};
var data = [trace1, trace2, trace3, trace4];
var layout = {
title: 'Connect the Gaps Between Null Values in the Z Matrix',
xaxis: {domain: [0, 0.45],
anchor: 'y1'},
yaxis: {domain: [0.55, 1],
anchor: 'x1'},
xaxis2: {domain: [0.55, 1],
anchor: 'y2'},
yaxis2: {domain: [0.55, 1],
anchor: 'x2'},
xaxis3: {domain: [0, 0.45],
anchor: 'y3'},
yaxis3: {domain: [0, 0.45],
anchor: 'x3'},
xaxis4: {domain: [0.55, 1],
anchor: 'y4'},
yaxis4: {domain: [0, 0.45],
anchor: 'x4'}
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[2, 4, 7, 12, 13, 14, 15, 16],
[3, 1, 6, 11, 12, 13, 16, 17],
[4, 2, 7, 7, 11, 14, 17, 18],
[5, 3, 8, 8, 13, 15, 18, 19],
[7, 4, 10, 9, 16, 18, 20, 19],
[9, 10, 5, 27, 23, 21, 21, 21],
[11, 14, 17, 26, 25, 24, 23, 22]],
type: 'contour',
line:{
smoothing: 0
},
xaxis: 'x1',
yaxis: 'y1'
},
{
z: [[2, 4, 7, 12, 13, 14, 15, 16],
[3, 1, 6, 11, 12, 13, 16, 17],
[4, 2, 7, 7, 11, 14, 17, 18],
[5, 3, 8, 8, 13, 15, 18, 19],
[7, 4, 10, 9, 16, 18, 20, 19],
[9, 10, 5, 27, 23, 21, 21, 21],
[11, 14, 17, 26, 25, 24, 23, 22]],
type: 'contour',
line:{
smoothing: 0.85
},
xaxis: 'x2',
yaxis: 'y2'
}];
var layout = {
title: 'Smoothing Contour Lines',
xaxis: {domain: [0, 0.45],
anchor: 'y1'},
yaxis: {domain: [0, 1],
anchor: 'x1'},
xaxis2: {domain: [0.55, 1],
anchor: 'y2'},
yaxis2: {domain: [0, 1],
anchor: 'x2'}
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
contours: {
coloring: 'heatmap'
}
}];
var layout = {
title: 'Smooth Contour Coloring'
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
colorscale: 'Jet',
contours:{
coloring: 'lines'
}
}];
var layout = {
title: 'Contour Lines'
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5.0, 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
contours: {
coloring: 'heatmap',
showlabels: true,
labelfont: {
family: 'Raleway',
size: 12,
color: 'white',
}
}
}];
var layout = {
title: 'Contour with Labels'
}
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
colorscale: [[0, 'rgb(166,206,227)'], [0.25, 'rgb(31,120,180)'], [0.45, 'rgb(178,223,138)'], [0.65, 'rgb(51,160,44)'], [0.85, 'rgb(251,154,153)'], [1, 'rgb(227,26,28)']]
}
];
var layout = {
title: 'Custom Contour Plot Colorscale'
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
colorbar:{
title: 'Color Bar Title',
titleside: 'right',
titlefont: {
size: 14,
family: 'Arial, sans-serif'
}
}
}];
var layout = {
title: 'Colorbar with a Title'
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
colorbar:{
thickness: 75,
thicknessmode: 'pixels',
len: 0.9,
lenmode: 'fraction',
outlinewidth: 0
}
}];
var layout = {
title: 'Colorbar Size for Contour Plots'
};
Plotly.newPlot('myDiv', data, layout);
var data = [ {
z: [[10, 10.625, 12.5, 15.625, 20],
[5.625, 6.25, 8.125, 11.25, 15.625],
[2.5, 3.125, 5., 8.125, 12.5],
[0.625, 1.25, 3.125, 6.25, 10.625],
[0, 0.625, 2.5, 5.625, 10]],
type: 'contour',
colorbar:{
ticks: 'outside',
dtick: 1,
tickwidth: 2,
ticklen: 10,
tickcolor: 'grey',
showticklabels: true,
tickfont: {
size: 15
},
xpad: 50
}
}];
var layout = {
title: 'Styling Color Bar Ticks for Contour Plots'
};
Plotly.newPlot('myDiv', data, layout);
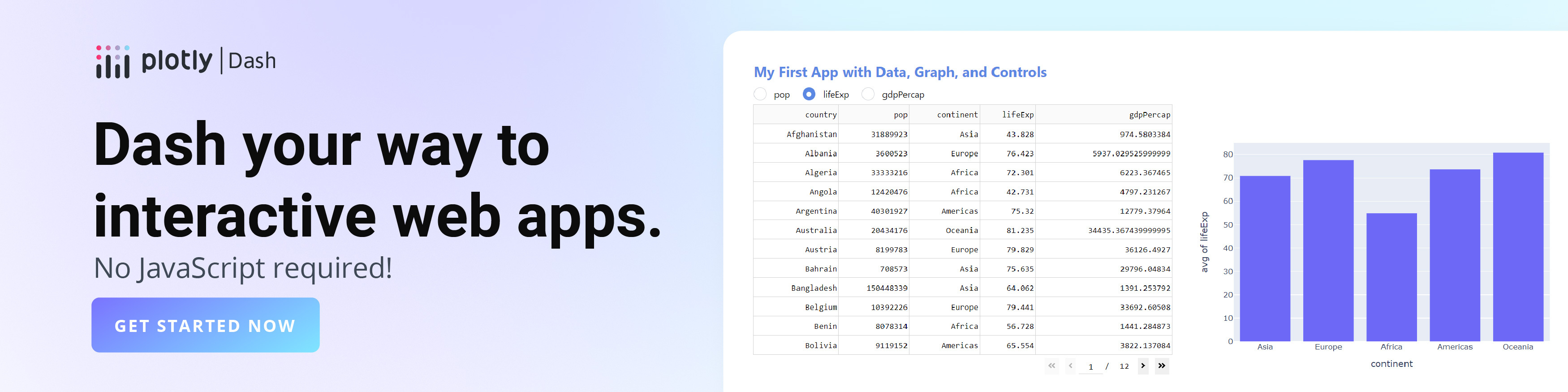