Plotly Streaming in Python/v3
Plotly Streaming
See our Version 4 Migration Guide for information about how to upgrade.
Streaming Support¶
Streaming is no longer supported in Chart Studio Cloud.
Streaming is still available as part of Chart Studio Enterprise. Additionally, Dash supports streaming, as demonstrated by the Dash Wind Streaming example.
Getting Started with Streaming¶
import numpy as np
import plotly.plotly as py
import plotly.tools as tls
import plotly.graph_objs as go
Before you start streaming, you're going to need some stream tokens. You will need one unique stream token for every trace object
you wish to stream to. Thus if you have two traces that you want to plot and stream, you're going to require two unique stream tokens. Notice that more tokens can be added via the settings section of your Plotly profile: https://plotly.com/settings/api
Now in the same way that you set your credentials, as shown in Getting Started, you can add stream tokens to your credentials file.
stream_ids = tls.get_credentials_file()['stream_ids']
print stream_ids
You'll see that stream_ids
will contain a list of the stream tokens we added to the credentials file.
An Example to Get You Started¶
Now that you have some stream tokens to play with, we're going to go over how we're going to put these into action. There are two main objects that will be created and used for streaming:
- Stream Id Object
- Stream link Object
We're going to look at these objects sequentially as we work through our first streaming example. For our first example, we're going to be streaming random data to a single scatter trace, and get something that behaves like the following:
Stream Id Object¶
The Stream Id Object
comes bundled in the graph_objs
package. We can then call help to see the description of this object:
help(go.Stream)
As we can see, the Stream Id Object
is a dictionary-like object that takes two parameters, and has all the methods that are assoicated with dictionaries.
We will need one of these objects for each of trace that we wish to stream data to.
We'll now create a single stream token for our streaming example, which will include one scatter trace.
# Get stream id from stream id list
stream_id = stream_ids[0]
# Make instance of stream id object
stream_1 = go.Stream(
token=stream_id, # link stream id to 'token' key
maxpoints=80 # keep a max of 80 pts on screen
)
The 'maxpoints'
key sets the maxiumum number of points to keep on the plotting surface at any given time.
More over, if you want to avoid the use of these Stream Id Objects
, you can just create a dictionary with at least the token parameter defined, for example:
stream_1 = dict(token=stream_id, maxpoints=60)
Now that we have our Stream Id Object
ready to go, we can set up our plot. We do this in the same way that we would any other plot, the only thing is that we now have to set the stream parameter in our trace object.
# Initialize trace of streaming plot by embedding the unique stream_id
trace1 = go.Scatter(
x=[],
y=[],
mode='lines+markers',
stream=stream_1 # (!) embed stream id, 1 per trace
)
data = go.Data([trace1])
# Add title to layout object
layout = go.Layout(title='Time Series')
# Make a figure object
fig = go.Figure(data=data, layout=layout)
Stream Link Object¶
The Stream Link Object is what will be used to communicate with the Plotly server in order to update the data contained in your trace objects. This object is in the plotly.plotly
object, an can be reference with py.Stream
help(py.Stream) # run help() of the Stream link object
You're going to need to set up one of these stream link objects for each trace you wish to stream data to.
Below we'll set one up for the scatter trace we have in our plot.
# We will provide the stream link object the same token that's associated with the trace we wish to stream to
s = py.Stream(stream_id)
# We then open a connection
s.open()
We can now use the Stream Link object s
in order to stream
data to our plot.
As an example, we will send a time stream and some random numbers:
# (*) Import module keep track and format current time
import datetime
import time
i = 0 # a counter
k = 5 # some shape parameter
# Delay start of stream by 5 sec (time to switch tabs)
time.sleep(5)
while True:
# Current time on x-axis, random numbers on y-axis
x = datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S.%f')
y = (np.cos(k*i/50.)*np.cos(i/50.)+np.random.randn(1))[0]
# Send data to your plot
s.write(dict(x=x, y=y))
# Write numbers to stream to append current data on plot,
# write lists to overwrite existing data on plot
time.sleep(1) # plot a point every second
# Close the stream when done plotting
s.close()
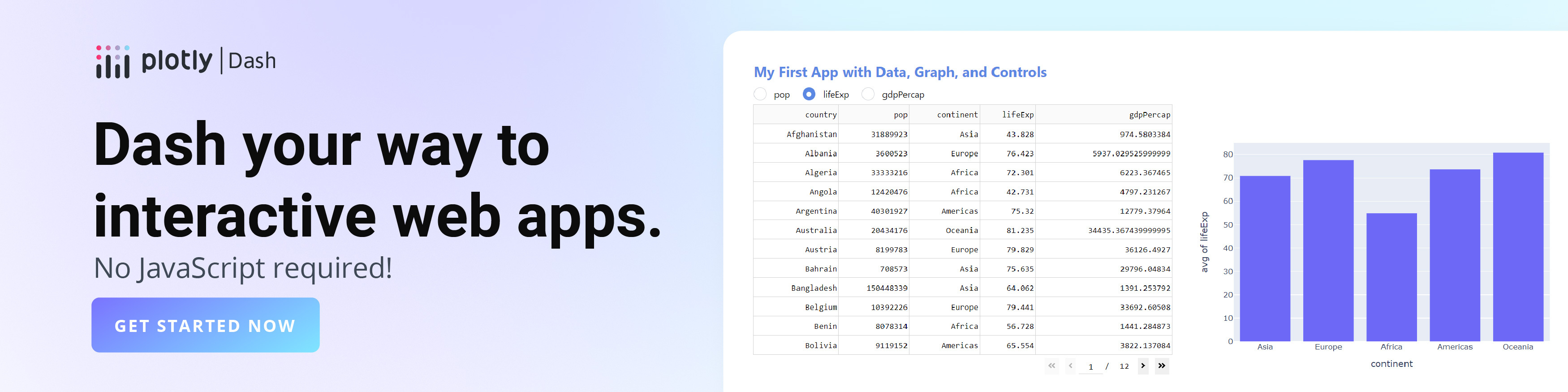