Updating Plotly Graphs in Python/v3
How to update your graphs in Python with the fileopt parameter.
See our Version 4 Migration Guide for information about how to upgrade.
New to Plotly?¶
Plotly's Python library is free and open source! Get started by downloading the client and reading the primer.
You can set up Plotly to work in online or offline mode, or in jupyter notebooks.
We also have a quick-reference cheatsheet (new!) to help you get started!
Version Check¶
Plotly's python package is updated frequently. Run pip install plotly --upgrade
to use the latest version.
import plotly
plotly.__version__
Overwriting existing graphs and updating a graph at its unique URL¶
By default, Plotly will overwrite files made with the same filename. For example, if a graph named 'my plot' already exists in your account, then it will be overwritten with this new version and the URL of the graph will persist.
import plotly.plotly as py
import plotly.graph_objs as go
data = [
go.Scatter(
x=[1, 2],
y=[3, 4]
)
]
plot_url = py.plot(data, filename='my plot')
Saving to a folder¶
Filenames that contain "/"
be treated as a Plotly directory and will be saved to your Plotly account in a folder tree. For example, to save your graphs to the folder my-graphs
use the filename = "my-graphs/my plot"
(if it doesn't already exist it will be created)
import plotly.plotly as py
import plotly.graph_objs as go
data = [
go.Scatter(
x=[1, 2],
y=[3, 4]
)
]
plot_url = py.plot(data, filename='my-graphs/my plot')
Creating new files¶
With fileopt='new'
, Plotly will always create a new file. If a file with the same name already exists, then Plotly will append a '(1)' to the end of the filename, e.g. new plot (1)
and create a unique URL.
import plotly.plotly as py
import plotly.graph_objs as go
data = [
go.Scatter(
x=[1, 2],
y=[3, 4]
)
]
plot_url = py.plot(data, filename='new plot', fileopt='new')
Extending traces in an existing graph¶
To extend existing traces with your new data, use fileopt='extend'
.
import plotly.plotly as py
import plotly.graph_objs as go
trace0 = go.Scatter(
x=[1, 2],
y=[1, 2]
)
trace1 = go.Scatter(
x=[1, 2],
y=[2, 3]
)
trace2 = go.Scatter(
x=[1, 2],
y=[3, 4]
)
data = [trace0, trace1, trace2]
# Take 1: if there is no data in the plot, 'extend' will create new traces.
plot_url = py.plot(data, filename='extend plot', fileopt='extend')
Then, extend the traces with more data.
import plotly.plotly as py
import plotly.graph_objs as go
trace0 = go.Scatter(
x=[3, 4],
y=[2, 1]
)
trace1 = go.Scatter(
x=[3, 4],
y=[3, 2]
)
trace2 = go.Scatter(
x=[3, 4],
y=[4, 3]
)
data = [trace0, trace1, trace2]
# Take 2: extend the traces on the plot with the data in the order supplied.
plot_url = py.plot(data, filename='extend plot', fileopt='extend')
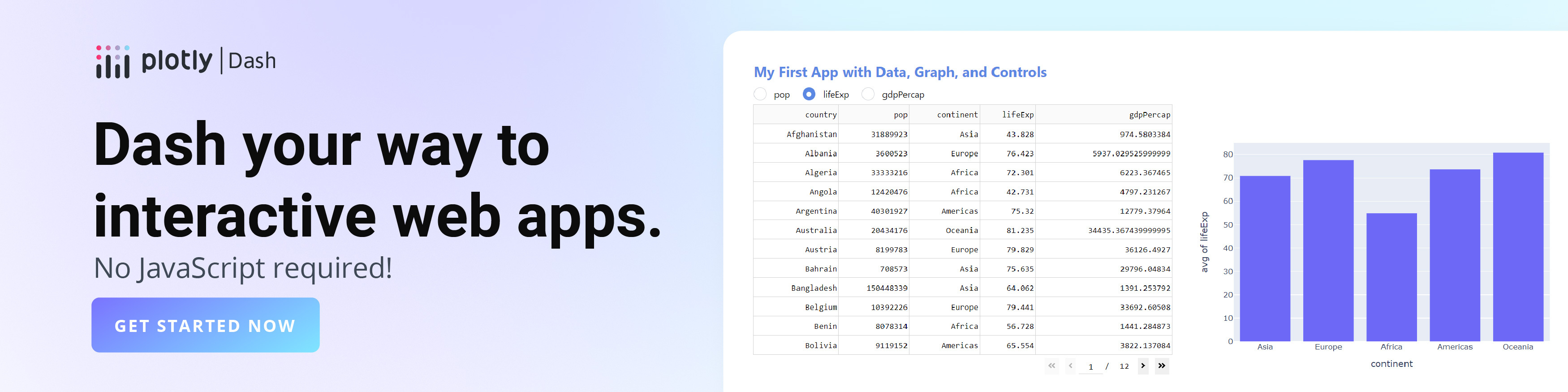